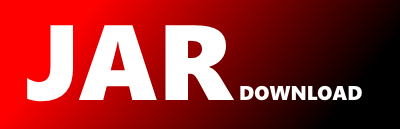
io.netty.handler.codec.http.router.BadClientSilencer Maven / Gradle / Ivy
package io.netty.handler.codec.http.router;
import io.netty.channel.ChannelHandler.Sharable;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
/**
* This handler should be put at the last position of the inbound pipeline to
* catch all exceptions caused by bad client (closed connection, malformed request etc.)
* and server processing.
*
* By default exceptions are logged to stderr. You may need to override
* onUnknownMessage, onBadClient, and onBadServer to log to more suitable places.
*/
@Sharable
public class BadClientSilencer extends SimpleChannelInboundHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy