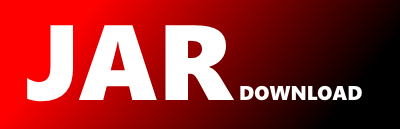
tw.teddysoft.ezspec.extension.junit5.JunitUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ezspec-core Show documentation
Show all versions of ezspec-core Show documentation
ezspec is a framework for adopting Gherkin in Java testing.
The newest version!
package tw.teddysoft.ezspec.extension.junit5;
import org.junit.jupiter.params.provider.Arguments;
import tw.teddysoft.ezspec.keyword.table.Header;
import tw.teddysoft.ezspec.keyword.table.Row;
import tw.teddysoft.ezspec.keyword.table.Table;
import java.lang.reflect.Constructor;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Stream;
import static java.lang.String.format;
/**
* {@code JunitUtils} is a class for parsing values in example using Junit5.
*
* @author Teddy Chen
* @since 1.0
*/
public class JunitUtils {
/**
* Construct table by parsing rows from argumentProvider.
*
* @param header the header of the table
* @param argumentProvider the argument for example execution
* @return the table
*/
public static Table constructTable(List header, Stream extends Arguments> argumentProvider) {
var rows = parseRowsFromJunit5ArgumentsProvider(Header.valueOf(header), argumentProvider);
return new Table(Header.valueOf(header), rows);
}
/**
* Parse rows from arguments provider list.
*
* @param header the header of the table
* @param provideArguments the argument for example execution
* @return the list
*/
public static List parseRowsFromJunit5ArgumentsProvider(Header header, Stream extends Arguments> provideArguments) {
List> rowsData = new ArrayList<>();
try {
var exampleStreams = new ArrayList<>(Arrays.asList(provideArguments));
for (var exampleStream : exampleStreams) {
exampleStream.forEach(x -> {
rowsData.add(new ArrayList<>());
Arrays.stream(x.get()).toList().forEach(each -> {
rowsData.get(rowsData.size() - 1).add(each.toString());
});
});
}
} catch (Exception e) {
throw new RuntimeException(e);
}
List rows = new ArrayList<>();
for (int i = 0; i < rowsData.size(); i++) {
rows.add(new Row(header, rowsData.get(i), i));
}
return rows;
}
public static Arguments getArgument(Class extends Junit5Examples> cls, String exampleName) {
try {
Class> clazz = Class.forName(cls.getTypeName());
Constructor> constructor = clazz.getDeclaredConstructor();
constructor.setAccessible(true);
Junit5Examples instance = (Junit5Examples) constructor.newInstance();
for (var arguments : instance.provideArguments(null).toList()) {
var args = arguments.get();
for (var arg : args) {
if (arg.equals(exampleName)) {
return arguments;
}
}
}
} catch (Exception e) {
throw new RuntimeException(e);
}
throw new RuntimeException(format("Example '%s' not found.", exampleName));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy