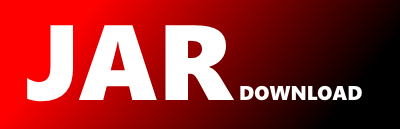
tw.teddysoft.ezspec.keyword.Result Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ezspec-core Show documentation
Show all versions of ezspec-core Show documentation
ezspec is a framework for adopting Gherkin in Java testing.
The newest version!
package tw.teddysoft.ezspec.keyword;
import java.util.Arrays;
import java.util.Objects;
/**
* {@code Result} is a class for presenting step status.
*
* @author Teddy Chen
* @since 1.0
*/
public class Result {
private StepExecutionOutcome executionOutcome;
private Throwable error;
public static Result Success(){
return new Result(StepExecutionOutcome.Success, null);
}
public static Result Failure(Throwable error){
Objects.requireNonNull(error, "error");
return new Result(StepExecutionOutcome.Failure, error);
}
public static Result Skip() {
return new Result(StepExecutionOutcome.Skipped, null);
}
public static Result Pending(Throwable error) {
return new Result(StepExecutionOutcome.Pending, error);
}
private Result(StepExecutionOutcome outcome, Throwable error) {
this.executionOutcome = outcome;
this.error = error;
}
public String getStackTrace(){
StringBuilder sb = new StringBuilder();
if (null != getException()){
Arrays.stream(getException().getStackTrace()).forEach(x -> {
if (!sb.isEmpty()) sb.append("\n");
sb.append(x.toString());
});
}
return sb.toString();
}
public StepExecutionOutcome getExecutionOutcome() {
return executionOutcome;
}
public boolean isSuccess() {
return executionOutcome.equals(StepExecutionOutcome.Success);
}
public boolean isFailure() {
return executionOutcome.equals(StepExecutionOutcome.Failure);
}
public boolean isSkipped() {
return executionOutcome.equals(StepExecutionOutcome.Skipped);
}
public boolean isPending() {
return executionOutcome.equals(StepExecutionOutcome.Pending);
}
@Override
public String toString(){
return executionOutcome.name();
}
public Throwable getException(){
return error;
}
public String getFailureMessage(){
return switch (executionOutcome){
case Failure -> {
StringBuilder sb = new StringBuilder(doGetFailureMessage());
sb.append("[").append(getException().getMessage().replace("expected: ", "anticipated: ")).append("]");
yield sb.toString();
}
case Pending -> {
StringBuilder sb = new StringBuilder();
if (null != getException() && null != getException().getMessage())
sb.append("[").append(getException().getMessage().replace("expected: ", "anticipated: ")).append("]");
yield sb.toString();
}
default -> "";
};
}
private String doGetFailureMessage(){
StringBuilder sb = new StringBuilder();
int stackIndex = 0;
StackTraceElement stackTrace [] = getException().getStackTrace();
for(int i = 0; i < stackTrace.length; i++){
if (stackTrace[i].toString().startsWith("tw.teddysoft.ezspec.scenario.RuntimeScenario.invokeStep")){
stackIndex = i - 1;
}
}
sb.append(stackTrace[stackIndex].toString());
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy