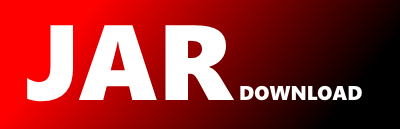
tw.teddysoft.ezspec.keyword.Rule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ezspec-core Show documentation
Show all versions of ezspec-core Show documentation
ezspec is a framework for adopting Gherkin in Java testing.
The newest version!
package tw.teddysoft.ezspec.keyword;
import tw.teddysoft.ezspec.keyword.visitor.SpecificationElement;
import tw.teddysoft.ezspec.keyword.visitor.SpecificationElementVisitor;
import java.util.ArrayList;
import java.util.List;
/**
* {@code Rule} is a class for representing a branch of the feature.
*
* @author Teddy Chen
* @since 1.0
*/
public class Rule implements SpecificationElement {
public static final String KEYWORD = "Rule";
private final String name;
private final List scenarios;
private String description;
private Background background;
private final Feature feature;
/**
* Instantiates a new Rule.
*
* @param name the name of rule
* @param feature the feature this rule belongs to
*/
Rule(String name, Feature feature){
this(name, "", feature);
}
Rule(String name, String description, Feature feature){
this.name = name;
this.description = description;
scenarios = new ArrayList<>();
background = Background.DEFAULT;
this.feature = feature;
}
/**
* Sets the description of rule.
*
* @param description the description
* @return the rule
*/
public Rule description(String description){
this.description = description;
return this;
}
/**
* A factory to create a scenario.
*
* @return the scenario
*/
public Scenario newScenario() {
return newScenario(Scenario.getEnclosingMethodName());
}
/**
* Creates a scenario in rule.
*
* @param name the name of scenario
* @return the scenario
*/
public Scenario newScenario(String name) {
var existingScenario = scenarios.stream().filter(x -> x.getName().equals(name)).findFirst();
if (existingScenario.isPresent()){
return existingScenario.get();
}
var scenario = new RuntimeScenario(name, background, this);
scenarios.add(scenario);
return scenario;
}
/**
* A factory to create a scenario outline.
*
* @return the scenario outline
*/
public ScenarioOutline newScenarioOutline() {
return newScenarioOutline(Scenario.getEnclosingMethodName(), "");
}
/**
* A factory to create a scenario outline.
*
* @param name the name of scenario outline
* @return the scenario outline
*/
public ScenarioOutline newScenarioOutline(String name) {
return newScenarioOutline(name, "");
}
/**
* A factory to create a scenario outline.
*
* @param name the name of scenario outline
* @param description the description of scenario outline
* @return the scenario outline
*/
public ScenarioOutline newScenarioOutline(String name, String description) {
var existingScenarioOutline = scenarios.stream().filter(x -> x.getName().equals(name)).findFirst();
if (existingScenarioOutline.isPresent()){
return (ScenarioOutline) existingScenarioOutline.get();
}
var scenarioOutline = new ScenarioOutline(name, description, background, this);
scenarios.add(scenarioOutline);
return scenarioOutline;
}
/**
* Creates a background to rule.
*
* @return the background
*/
public Background newBackground() {
background = new Background(Scenario.getEnclosingMethodName(), this);
return background;
}
/**
* Creates a background to rule.
*
* @param name the name of background
* @return the background
*/
public Background newBackground(String name) {
background = new Background(name, this);
return background;
}
void setBackground(Background background) {
this.background = background;
}
/**
* Gets scenarios.
*
* @return the scenarios
*/
public List getScenarios() {
return scenarios;
}
/**
* Gets rule name.
*
* @return the name of rule
*/
public String getName() {
return name ;
}
/**
* Gets rule description.
*
* @return the description of rule
*/
public String description(){
return description;
}
/**
* Gets last scenario.
*
* @return the scenario
*/
public Scenario lastScenario() {
return scenarios.get(scenarios.size() - 1);
}
/**
* Gets last scenario outline.
*
* @return the scenario outline
*/
public ScenarioOutline lastScenarioOutline() {
var scenarioOutlines = scenarios.stream().filter(x-> x instanceof ScenarioOutline).toList();
return (ScenarioOutline) scenarioOutlines.get(scenarioOutlines.size() - 1);
}
/**
* Rule text string including rule name, rule description and background.
*
* @return the string
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
if (!name.isEmpty())
sb.append(KEYWORD).append(": ").append(name).append("\n");
if (null != description && !description.isEmpty())
sb.append(description).append("\n");
if (null != background && Background.DEFAULT != background){
sb.append(Background.KEYWORD).append(": ").append(background).append("\n");
}
scenarios.forEach(scenario -> sb.append(scenario.toString()).append("\n"));
return sb.toString();
}
/**
* Empty rule.
*
* @return the empty rule
*/
public static Rule Empty(){
return new EmptyRule();
}
/**
* Gets background.
*
* @return the background
*/
public Background getBackground() {
return background;
}
@Override
public void accept(SpecificationElementVisitor visitor) {
if (!name.isEmpty())
visitor.visit(this);
background.accept(visitor);
scenarios.forEach(scenario -> {
scenario.accept(visitor);
});
}
public Feature getFeature(){
return feature;
};
public void removeScenario(Scenario scenario) {
scenarios.removeIf( x-> x.getName().equals(scenario.getName()));
}
public void addScenario(Scenario scenario) {
scenarios.add(scenario);
}
/**
* {@code EmptyRule} is a class for representing an empty rule.
*/
public static class EmptyRule extends Rule {
private EmptyRule() {
super("Empty Rule", null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy