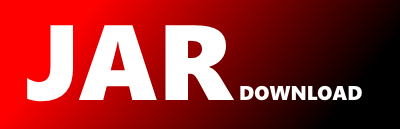
tw.teddysoft.ezspec.keyword.ScenarioEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ezspec-core Show documentation
Show all versions of ezspec-core Show documentation
ezspec is a framework for adopting Gherkin in Java testing.
The newest version!
package tw.teddysoft.ezspec.keyword;
import tw.teddysoft.ezspec.keyword.table.Row;
import tw.teddysoft.ezspec.keyword.table.Table;
import java.util.*;
/**
* {@code ScenarioEnvironment} is a class for passing variables between steps
* in scenario.
*
* @author Teddy Chen
* @since 1.0
*/
public class ScenarioEnvironment{
/**
* The constant INPUT_KEY is the key of the current executing example table.
*/
public static final String INPUT_KEY = "$INPUT";
/**
* The constant ANONYMOUS_TABLE_KEY is the key of the last table
* in step description.
*/
public static final String ANONYMOUS_TABLE_KEY = "$ANONYMOUS_TABLE";
/**
* The constant ARGUMENTS_KEY is the key of the last argument
* in step description.
*/
public static final String ARGUMENTS_KEY = "$ARGUMENTS";
/**
* The constant HISTORICAL_ARGUMENTS_KEY is the key of the past arguments
* in step description.
*/
public static final String HISTORICAL_ARGUMENTS_KEY = "$HISTORICAL_ARGUMENTS";
private int executionCount;
private final Map context;
private ScenarioEnvironment() {
context = new HashMap<>();
context.put(ARGUMENTS_KEY, new ArrayList());
context.put(HISTORICAL_ARGUMENTS_KEY, new ArrayList());
executionCount = 0;
}
private ScenarioEnvironment(ScenarioEnvironment env) {
this();
historicalArguments().addAll(env.getHistoricalArgs());
put(INPUT_KEY, env.getInput());
setAnonymousTable(env.get(ANONYMOUS_TABLE_KEY, Table.class));
for (Map.Entry entry : env.context.entrySet()) {
switch (entry.getKey()) {
case INPUT_KEY, ANONYMOUS_TABLE_KEY, ARGUMENTS_KEY, HISTORICAL_ARGUMENTS_KEY:
break;
default: {
context.put(entry.getKey(), entry.getValue());
break;
}
}
}
}
/**
* Clone scenario environment.
*
* @param env the env
* @return the scenario environment
*/
public static ScenarioEnvironment clone(ScenarioEnvironment env) {
return new ScenarioEnvironment(env);
}
public static ScenarioEnvironment create() {
return new ScenarioEnvironment();
}
public ScenarioEnvironment setInput(Table table) {
put(INPUT_KEY, table);
return this;
}
public int getExecutionCount() {
return executionCount;
}
public void setExecutionCount(int executionCount) {
this.executionCount = executionCount;
}
public Table getInput() {
return get(INPUT_KEY, Table.class);
}
public void addContext(ScenarioEnvironment runtime){
context.putAll(runtime.context);
}
public ScenarioEnvironment setAnonymousTable(Table table) {
return put(ANONYMOUS_TABLE_KEY, table);
}
public Table table() {
if (!context.containsKey(ANONYMOUS_TABLE_KEY)) {
throw new RuntimeException("No anonymous table in the scenario.");
}
return get(ANONYMOUS_TABLE_KEY, Table.class);
}
public Row lastRow() {
if (!context.containsKey(ANONYMOUS_TABLE_KEY)) {
throw new RuntimeException("No anonymous table in the scenario.");
}
return get(ANONYMOUS_TABLE_KEY, Table.class).row(this.table().rows().size() - 1);
}
public Row row(int index) {
if (!context.containsKey(ANONYMOUS_TABLE_KEY)) {
throw new RuntimeException("No anonymous table in the scenario.");
}
return get(ANONYMOUS_TABLE_KEY, Table.class).row(index);
}
public Row row(String firstColumn) {
if (!context.containsKey(ANONYMOUS_TABLE_KEY)) {
throw new RuntimeException("No anonymous table in the scenario.");
}
return get(ANONYMOUS_TABLE_KEY, Table.class).row(firstColumn);
}
public ScenarioEnvironment put(String key, Object value) {
context.put(key, value);
return this;
}
public String gets(String key) {
return (String) context.getOrDefault(key, "");
}
public int geti(String key) {
return Integer.valueOf(gets(key));
}
public Table gett(String key) {
return get(key, Table.class);
}
public T get(String key, Class cls) {
return (T) context.get(key);
}
public List getArgs(){
return Collections.unmodifiableList(arguments());
}
public List getHistoricalArgs(){
return Collections.unmodifiableList(historicalArguments());
}
public boolean hasArgument(){
return !arguments().isEmpty();
}
public String getArg(int index){
return arguments().get(index).value();
}
public int getArgi(int index){
return Integer.valueOf(arguments().get(index).value().replace(",", ""));
}
public double getArgi(String key){
String str = getArg(key);
return Integer.valueOf(str.replace(",", ""));
}
public double getArgd(String key){
String str = getArg(key);
if (str.endsWith("%")){
str = str.replaceAll("%", "");
return Double.parseDouble(str) / 100.0;
}
return Double.parseDouble(str.replace(",", ""));
}
public double getArgd(int index){
String str = arguments().get(index).value();
if (str.endsWith("%")){
str = str.replaceAll("%", "");
return Double.parseDouble(str) / 100.0;
}
return Double.parseDouble(str.replace(",", ""));
}
public String getHistoricalArg(int i){
return historicalArguments().get(i).value();
}
public String getArg(String key){
return arguments().stream().filter(x -> x.key().equals(key)).findFirst().get().value();
}
public String getHistoricalArg(String key){
return historicalArguments().stream().filter(x -> x.key().equals(key)).findFirst().get().value();
}
private List arguments(){
return ((List) context.get(ARGUMENTS_KEY));
}
private List historicalArguments(){
return ((List) context.get(HISTORICAL_ARGUMENTS_KEY));
}
void setArguments(List args) {
arguments().clear();
arguments().addAll(args);
historicalArguments().addAll(args);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy