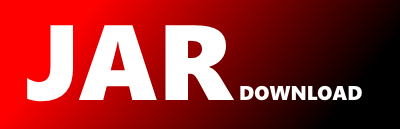
tw.teddysoft.ezspec.keyword.Step Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ezspec-core Show documentation
Show all versions of ezspec-core Show documentation
ezspec is a framework for adopting Gherkin in Java testing.
The newest version!
package tw.teddysoft.ezspec.keyword;
import tw.teddysoft.ezspec.keyword.visitor.SpecificationElement;
import tw.teddysoft.ezspec.keyword.visitor.SpecificationElementVisitor;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
import java.util.function.Consumer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* {@code Step} is a class for representing Gherkin steps.
*
* @author Teddy Chen
* @since 1.0
*/
public abstract class Step implements SpecificationElement {
public static final boolean ContinuousAfterFailure = true;
public static final boolean TerminateAfterFailure = false;
private String description;
private Result result;
private Consumer callback;
private final boolean continuousAfterFailure;
/**
* Instantiates a new Step.
*
* @param description the step description
* @param callback the step definition
*/
public Step(String description, Consumer callback){
this(description, false, callback);
}
/**
* Instantiates a new Step.
*
* @param description the step description
* @param continuous the parameter for deciding to continue executing
* the next step after this step failed
* @param callback the step definition
*/
public Step(String description, boolean continuous, Consumer callback){
if (description.endsWith("\n")) {
description = description.substring(0, description.length() - 1);
}
this.description = description.trim();
this.continuousAfterFailure = continuous;
this.callback = callback;
this.result = Result.Pending(null);
}
public boolean isContinuousAfterFailure() {
return continuousAfterFailure;
}
public Consumer getCallback() {
return callback;
}
public String description(){
return description;
}
public Result getResult() {
return result;
}
public void setResult(Result result) {
this.result = result;
}
public abstract String getName();
static List parseArguments(String description){
List arguments = new LinkedList<>();
String regx = "\\s\\$\\{([^}]+)\\}|\\s\\$([\\S^{]+)";
Pattern pattern = Pattern.compile(regx);
Matcher matcher = pattern.matcher(" " + description);
while (matcher.find()) {
arguments.add(new Argument(matcher.group(0)));
}
return arguments;
}
/**
* Erase table string in step description.
*
* @param description the description
* @return the string
*/
public static String eraseTable(String description){
String lines[] = description.split("\\r?\\n");
StringBuilder sb = new StringBuilder();
Arrays.stream(lines).forEach(line ->{
if (!line.startsWith("|") && !line.startsWith("<|") && !line.startsWith(">"))
sb.append(line);
});
return sb.toString();
}
static String eraseReservedWords(String description){
description = description + " ";
String regxValueArgument = "\\s$([^{])([\\S]+)|\\s\\$([^{])([\\S]?)";
Pattern pattern = Pattern.compile(regxValueArgument);
Matcher matcher = pattern.matcher(description);
int removedCharCount = 0;
while (matcher.find()) {
String value = " " + matcher.group(0).substring(2);
description = description.substring(0, matcher.start() - removedCharCount) + value + description.substring(matcher.end() - removedCharCount);
removedCharCount++;
}
String regxKeyValueArgument = "\\s\\$\\{([^}]+)\\}";
pattern = Pattern.compile(regxKeyValueArgument);
matcher = pattern.matcher(description);
String regxKeyValueSplitter = "(\\=|\\:)";
while (matcher.find()) {
String data = matcher.group(1);
if (null != data){
String value = data.split(regxKeyValueSplitter)[1];
description = description.replace(matcher.group(0).trim(), value);
}
}
return description.trim();
}
@Override
public void accept(SpecificationElementVisitor visitor){
visitor.visit(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy