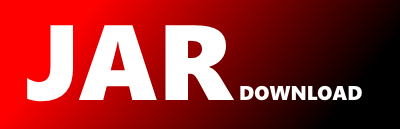
commonMain.kotlinx.collections.atomic.MutableAtomicList.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx-collections-atomic Show documentation
Show all versions of kotlinx-collections-atomic Show documentation
A Kotlin Multiplatform Mutable Collections that are thread safe
package kotlinx.collections.atomic
import kotlinx.atomicfu.AtomicRef
import kotlinx.atomicfu.atomic
internal class MutableAtomicList(
override val atomic: AtomicRef>
) : MutableAtomicCollection>, MutableList {
constructor(list: MutableList) : this(atomic(list))
override fun doAction(run: MutableList.() -> T): T {
val list = atomic.value.toMutableList()
val res = list.run()
atomic.lazySet(list)
return res
}
override fun get(index: Int): E = atomic.value[index]
override fun indexOf(element: E): Int = atomic.value.indexOf(element)
override fun lastIndexOf(element: E): Int = atomic.value.lastIndexOf(element)
override fun listIterator(): MutableListIterator = atomic.value.listIterator()
override fun listIterator(index: Int): MutableListIterator = atomic.value.listIterator(index)
override fun set(index: Int, element: E): E = atomic.value.set(index, element)
override fun subList(fromIndex: Int, toIndex: Int): MutableList = atomic.value.subList(fromIndex, toIndex)
override fun add(index: Int, element: E) = doAction { add(index, element) }
override fun addAll(index: Int, elements: Collection): Boolean = doAction { addAll(index, elements) }
override fun removeAt(index: Int): E = doAction { removeAt(index) }
override fun clear() {
atomic.lazySet(mutableListOf())
}
override val size: Int
get() = atomic.value.size
override fun contains(element: E): Boolean = atomic.value.contains(element)
override fun containsAll(elements: Collection): Boolean = atomic.value.containsAll(elements)
override fun isEmpty(): Boolean = atomic.value.isEmpty()
override fun iterator(): MutableIterator = atomic.value.iterator()
override fun add(element: E): Boolean = doAction { add(element) }
override fun addAll(elements: Collection): Boolean = doAction { addAll(elements) }
override fun remove(element: E): Boolean = doAction { remove(element) }
override fun removeAll(elements: Collection): Boolean = doAction { removeAll(elements) }
override fun retainAll(elements: Collection): Boolean = doAction { retainAll(elements) }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy