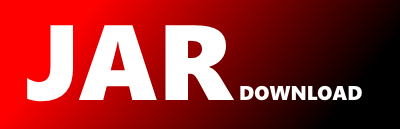
commonMain.kotlinx.collections.atomic.MutableAtomicMap.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx-collections-atomic Show documentation
Show all versions of kotlinx-collections-atomic Show documentation
A Kotlin Multiplatform Mutable Collections that are thread safe
package kotlinx.collections.atomic
import kotlinx.atomicfu.atomic
internal class MutableAtomicMap(value: MutableMap = mutableMapOf()) : MutableMap {
private val atomic = atomic(value)
override val size: Int
get() = atomic.value.size
override fun containsKey(key: K): Boolean = atomic.value.containsKey(key)
override fun containsValue(value: V): Boolean = atomic.value.containsValue(value)
override fun get(key: K): V? = atomic.value[key]
override fun isEmpty(): Boolean = atomic.value.isEmpty()
override val entries: MutableSet>
get() = atomic.value.entries
override val keys: MutableSet
get() = atomic.value.keys
override val values: MutableCollection
get() = atomic.value.values
override fun clear() {
atomic.lazySet(mutableMapOf())
}
private inline fun doAction(builder: MutableMap.() -> R): R {
val map = atomic.value
val newMap = mutableMapOf().apply { putAll(map) }
val res = newMap.builder()
atomic.lazySet(newMap)
return res
}
@OptIn(ExperimentalStdlibApi::class)
override fun put(key: K, value: V): V? = doAction { put(key, value) }
override fun putAll(from: Map) = doAction { putAll(from) }
override fun remove(key: K): V? = doAction { remove(key) }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy