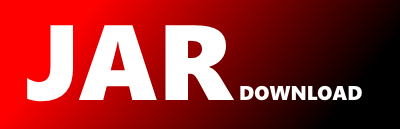
commonTest.ListConcurrencyTest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx-collections-atomic Show documentation
Show all versions of kotlinx-collections-atomic Show documentation
A Kotlin Multiplatform Mutable Collections that are thread safe
import expect.*
import kotlinx.collections.atomic.mutableAtomicListOf
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.runTest
import kotlinx.coroutines.withContext
import kotlin.test.Test
class ListConcurrencyTest {
val numbers: MutableList = mutableAtomicListOf(1)
@Test
fun should_add_items_to_list() {
numbers.add(1)
}
@Test
fun should_add_items_in_different_threads() = runTest {
val chars: MutableList = mutableAtomicListOf('A')
withContext(Dispatchers.Default) {
chars.add('B')
}
withContext(Dispatchers.Unconfined) {
chars.add('C')
}
expect(chars).toContain('A', 'B', 'C')
}
@Test
fun should_add_multiple_items_in_different_threads() = runTest {
val chars: MutableList = mutableAtomicListOf('A')
withContext(Dispatchers.Default) {
chars.addAll(listOf('B'))
}
withContext(Dispatchers.Unconfined) {
chars.addAll(listOf('C'))
}
expect(chars).toContain('A', 'B', 'C')
}
@Test
fun should_remove_items_in_any_thread() = runTest {
val chars: MutableList = mutableAtomicListOf('A', 'B', 'C')
withContext(Dispatchers.Default) {
chars.remove('B')
}
withContext(Dispatchers.Unconfined) {
chars.remove('C')
}
expect(chars).toContain('A')
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy