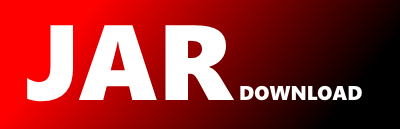
net.jradius.dictionary.vsa_shiva.VSADictionaryImpl Maven / Gradle / Ivy
// DO NOT EDIT THIS FILE DIRECTLY! - AUTOMATICALLY GENERATED
// Generated by: class net.jradius.freeradius.RadiusDictionary
// Generated on: Fri, 29 Nov 2013 13:24:47 +0000
package net.jradius.dictionary.vsa_shiva;
import java.util.Map;
import net.jradius.packet.attribute.VSADictionary;
/**
* Dictionary for package net.jradius.dictionary.vsa_shiva
* @author class net.jradius.freeradius.RadiusDictionary
*/
public class VSADictionaryImpl implements VSADictionary
{
public String getVendorName() { return "Shiva"; }
public void loadAttributes(Map> map)
{
map.put(new Long(1L), Attr_ShivaUserAttributes.class);
map.put(new Long(30L), Attr_ShivaCompression.class);
map.put(new Long(31L), Attr_ShivaDialbackDelay.class);
map.put(new Long(32L), Attr_ShivaCallDurnTrap.class);
map.put(new Long(33L), Attr_ShivaBandwidthTrap.class);
map.put(new Long(34L), Attr_ShivaMinimumCall.class);
map.put(new Long(35L), Attr_ShivaDefaultHost.class);
map.put(new Long(36L), Attr_ShivaMenuName.class);
map.put(new Long(37L), Attr_ShivaUserFlags.class);
map.put(new Long(38L), Attr_ShivaTermtype.class);
map.put(new Long(39L), Attr_ShivaBreakKey.class);
map.put(new Long(40L), Attr_ShivaFwdKey.class);
map.put(new Long(41L), Attr_ShivaBakKey.class);
map.put(new Long(42L), Attr_ShivaDialTimeout.class);
map.put(new Long(43L), Attr_ShivaLATPort.class);
map.put(new Long(44L), Attr_ShivaMaxVCs.class);
map.put(new Long(45L), Attr_ShivaDHCPLeasetime.class);
map.put(new Long(46L), Attr_ShivaLATGroups.class);
map.put(new Long(60L), Attr_ShivaRTCTimestamp.class);
map.put(new Long(61L), Attr_ShivaCircuitType.class);
map.put(new Long(90L), Attr_ShivaCalledNumber.class);
map.put(new Long(91L), Attr_ShivaCallingNumber.class);
map.put(new Long(92L), Attr_ShivaCustomerId.class);
map.put(new Long(93L), Attr_ShivaTypeOfService.class);
map.put(new Long(94L), Attr_ShivaLinkSpeed.class);
map.put(new Long(95L), Attr_ShivaLinksInBundle.class);
map.put(new Long(96L), Attr_ShivaCompressionType.class);
map.put(new Long(97L), Attr_ShivaLinkProtocol.class);
map.put(new Long(98L), Attr_ShivaNetworkProtocols.class);
map.put(new Long(99L), Attr_ShivaSessionId.class);
map.put(new Long(100L), Attr_ShivaDisconnectReason.class);
map.put(new Long(101L), Attr_ShivaAcctServSwitch.class);
map.put(new Long(102L), Attr_ShivaEventFlags.class);
map.put(new Long(103L), Attr_ShivaFunction.class);
map.put(new Long(104L), Attr_ShivaConnectReason.class);
}
public void loadAttributesNames(Map> map)
{
map.put(Attr_ShivaUserAttributes.NAME, Attr_ShivaUserAttributes.class);
map.put(Attr_ShivaCompression.NAME, Attr_ShivaCompression.class);
map.put(Attr_ShivaDialbackDelay.NAME, Attr_ShivaDialbackDelay.class);
map.put(Attr_ShivaCallDurnTrap.NAME, Attr_ShivaCallDurnTrap.class);
map.put(Attr_ShivaBandwidthTrap.NAME, Attr_ShivaBandwidthTrap.class);
map.put(Attr_ShivaMinimumCall.NAME, Attr_ShivaMinimumCall.class);
map.put(Attr_ShivaDefaultHost.NAME, Attr_ShivaDefaultHost.class);
map.put(Attr_ShivaMenuName.NAME, Attr_ShivaMenuName.class);
map.put(Attr_ShivaUserFlags.NAME, Attr_ShivaUserFlags.class);
map.put(Attr_ShivaTermtype.NAME, Attr_ShivaTermtype.class);
map.put(Attr_ShivaBreakKey.NAME, Attr_ShivaBreakKey.class);
map.put(Attr_ShivaFwdKey.NAME, Attr_ShivaFwdKey.class);
map.put(Attr_ShivaBakKey.NAME, Attr_ShivaBakKey.class);
map.put(Attr_ShivaDialTimeout.NAME, Attr_ShivaDialTimeout.class);
map.put(Attr_ShivaLATPort.NAME, Attr_ShivaLATPort.class);
map.put(Attr_ShivaMaxVCs.NAME, Attr_ShivaMaxVCs.class);
map.put(Attr_ShivaDHCPLeasetime.NAME, Attr_ShivaDHCPLeasetime.class);
map.put(Attr_ShivaLATGroups.NAME, Attr_ShivaLATGroups.class);
map.put(Attr_ShivaRTCTimestamp.NAME, Attr_ShivaRTCTimestamp.class);
map.put(Attr_ShivaCircuitType.NAME, Attr_ShivaCircuitType.class);
map.put(Attr_ShivaCalledNumber.NAME, Attr_ShivaCalledNumber.class);
map.put(Attr_ShivaCallingNumber.NAME, Attr_ShivaCallingNumber.class);
map.put(Attr_ShivaCustomerId.NAME, Attr_ShivaCustomerId.class);
map.put(Attr_ShivaTypeOfService.NAME, Attr_ShivaTypeOfService.class);
map.put(Attr_ShivaLinkSpeed.NAME, Attr_ShivaLinkSpeed.class);
map.put(Attr_ShivaLinksInBundle.NAME, Attr_ShivaLinksInBundle.class);
map.put(Attr_ShivaCompressionType.NAME, Attr_ShivaCompressionType.class);
map.put(Attr_ShivaLinkProtocol.NAME, Attr_ShivaLinkProtocol.class);
map.put(Attr_ShivaNetworkProtocols.NAME, Attr_ShivaNetworkProtocols.class);
map.put(Attr_ShivaSessionId.NAME, Attr_ShivaSessionId.class);
map.put(Attr_ShivaDisconnectReason.NAME, Attr_ShivaDisconnectReason.class);
map.put(Attr_ShivaAcctServSwitch.NAME, Attr_ShivaAcctServSwitch.class);
map.put(Attr_ShivaEventFlags.NAME, Attr_ShivaEventFlags.class);
map.put(Attr_ShivaFunction.NAME, Attr_ShivaFunction.class);
map.put(Attr_ShivaConnectReason.NAME, Attr_ShivaConnectReason.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy