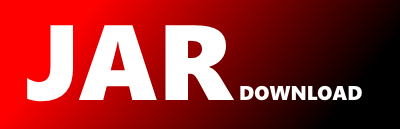
uk.ac.liv.pgb.analytica.lib.RTaskWithManifest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analytica-lib Show documentation
Show all versions of analytica-lib Show documentation
Library allowing generation of plots and data from proteomics and
metabolomics mass spectrometry data through the use of R and java
methods.
The newest version!
package uk.ac.liv.pgb.analytica.lib;
import java.io.File;
import java.io.IOException;
import java.util.Optional;
import uk.ac.liv.pgb.analytica.lib.wrappedr.RDataTransformations;
import uk.ac.liv.pgb.analytica.lib.wrappedr.RRuntime;
import uk.ac.liv.pgb.analytica.lib.wrappedr.RSourceFile;
import uk.ac.liv.pgb.analytica.lib.wrappedr.converters.RDataConverter;
/**
* Wraps a piece of R code that has a manifest describing what to do with it.
* @author sperkins
*/
public final class RTaskWithManifest implements Task {
/**
* The R source object.
*/
private final RSourceFile source;
/**
* Creates an instance of the class with the given R source object.
* @param source The R source object.
*/
public RTaskWithManifest(final RSourceFile source) {
this.source = source;
}
/**
* Gets the name of associated with this task.
* @return The task name.
*/
@Override
public String getName() {
return source.getManifest().getTaskName();
}
/**
* Checks whether the given file is compatible with this task.
* @param file Input file.
* @return True if the given file is compatible with this task.
*/
@Override
public boolean compatibleWith(final File file) {
return RDataTransformations.getInstance().getConverters().stream().anyMatch(conv -> {
if (source.getManifest().getTaskSupportedFormats().stream().anyMatch(rTaskFormat -> {
return conv.getSupportedOutput().equalsIgnoreCase(rTaskFormat);
})) {
return conv.getSupportedInputs().stream().anyMatch(format -> {
return file.getName().toUpperCase().endsWith(format);
});
}
return false;
});
}
/**
* Executes this R task with the given file and graphics policy.
* @param file The input file.
* @param policy The graphics policy.
* @return The execution result describing the output of the task.
*/
@Override
public TaskExecutionResult execute(final File file, final GraphicsPolicy policy) {
// Get the RRuntime early, so that we fail fast if there is a problem with it.
RRuntime runtime = RRuntime.getInstance();
// Get a converter for the file for have read in that converts to a format that the task supports.
Optional converter = RDataTransformations.getInstance().getConverters().stream().filter(conv -> {
if (source.getManifest().getTaskSupportedFormats().stream().anyMatch(rTaskFormat -> {
return conv.getSupportedOutput().equalsIgnoreCase(rTaskFormat);
})) {
return conv.getSupportedInputs().stream().anyMatch(format -> {
return file.getName().toUpperCase().endsWith(format);
});
}
return false;
}).findAny();
// If we didn't find a converter, we can't do anything more. Return a result reflecting this.
if (!converter.isPresent()) {
return TaskExecutionResult.error("The selected task requires a format that can not be produced by the supplied file.", null);
}
// Need to create a temp file for our data in the right format.
// If there is an exception, return an appropriate result.
File tmpDataFile;
try {
tmpDataFile = File.createTempFile("analytics_", "_tmp_data.csv");
} catch (IOException ex) {
return TaskExecutionResult.error("Could not create the temporary data file.", ex);
}
// Convert the input file to a format that the R script can parse.
converter.get().convert(file, tmpDataFile);
// Install/load our required packages.
source.getManifest().getTaskDependencies().forEach(p -> {
runtime.loadPackageDependency(p);
});
// Load in our R code file.
runtime.source(source.getSourceFile());
// Check our graphics policy. If it is to write a file, we will do that.
if (policy.isToFileDevice()) {
// Build the output file name.
StringBuilder outPathBuilder = new StringBuilder();
outPathBuilder.append(file.getParentFile().getAbsolutePath());
outPathBuilder.append(File.separator);
String prefix = policy.getOutputFilePrefix();
outPathBuilder.append(prefix != null ? prefix : "analytica_");
outPathBuilder.append(this.getName()).append("_");
outPathBuilder.append(file.getName().replaceAll("\\.[^\\.]+$", "")).append("_");
outPathBuilder.append(".png");
runtime.loadFileGraphicsDevice(new File(outPathBuilder.toString()));
} else {
// Load in our java graphics device. This (or subsequently displaying graphics)
// should fail when not called from the GUI as the graphics device will not exist.
throw new UnsupportedOperationException("Not yet implemented!");
}
// Execute our function.
runtime.executeFunction(source.getManifest().getFunctionName(), tmpDataFile.getAbsolutePath());
// Close graphics device, regardless of what it is.
// Note: this may be wrong for a java graphics device.
runtime.closeGraphicsDevice();
return TaskExecutionResult.success("Executed R function: " + source.getManifest().getFunctionName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy