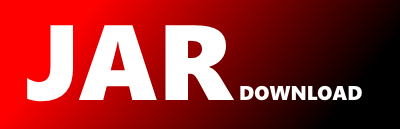
uk.ac.liv.pgb.analytica.lib.TaskManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analytica-lib Show documentation
Show all versions of analytica-lib Show documentation
Library allowing generation of plots and data from proteomics and
metabolomics mass spectrometry data through the use of R and java
methods.
The newest version!
package uk.ac.liv.pgb.analytica.lib;
import java.io.File;
import java.util.LinkedList;
import java.util.List;
import java.util.Optional;
import uk.ac.liv.pgb.analytica.lib.wrappedr.RSourceFile;
import uk.ac.liv.pgb.analytica.lib.wrappedr.RSourceScanner;
/**
* Manages all available tasks.
* @author sperkins
*/
public final class TaskManager {
/**
* The singleton instance of this class.
*/
private static final TaskManager INSTANCE = new TaskManager();
/**
* The singleton instance for managing R source files.
*/
private final RSourceScanner rSources = RSourceScanner.getInstance();
/**
* Tasks that have been registered.
*/
private final List registeredTasks = new LinkedList<>();
/**
* The graphics policy that has been registered.
*/
private GraphicsPolicy graphicsPolicy = null;
/**
* Private constructor to prevent external instantiation.
*/
private TaskManager() {
registerRTasks();
}
/**
* Gets the singleton instance of this class.
* @return The singleton instance of this class.
*/
public static TaskManager getInstance() {
return INSTANCE;
}
/**
* Registers the given graphics policy with this class.
* @param policy The graphics policy.
*/
public void registerGraphicsPolicy(final GraphicsPolicy policy) {
this.graphicsPolicy = policy;
}
/**
* Finds a task given the name.
* @param taskName The task name.
* @return The found task.
*/
public Task findTask(final String taskName) {
Optional taskOptional = registeredTasks.stream().filter(p -> p.getName().equalsIgnoreCase(taskName)).findFirst();
return taskOptional.isPresent() ? taskOptional.get() : null;
}
/**
* Executes the given task with the given file.
* @param task The task.
* @param file The input file.
*/
public void executeTask(final Task task, final File file) {
task.execute(file, graphicsPolicy != null ? graphicsPolicy : new GraphicsPolicy());
}
/**
* Registers all available R tasks from the R source scanner.
*/
private void registerRTasks() {
List sources = rSources.getScannedFiles();
for (RSourceFile source : sources) {
if (source.hasManifest()) {
RTaskWithManifest task = new RTaskWithManifest(source);
registeredTasks.add(task);
} else {
RTaskWithoutManifest task = new RTaskWithoutManifest();
registeredTasks.add(task);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy