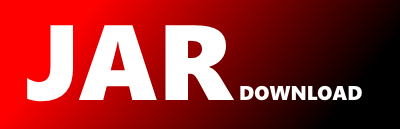
uk.ac.liv.pgb.analytica.lib.wrappedr.CommandExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analytica-lib Show documentation
Show all versions of analytica-lib Show documentation
Library allowing generation of plots and data from proteomics and
metabolomics mass spectrometry data through the use of R and java
methods.
The newest version!
package uk.ac.liv.pgb.analytica.lib.wrappedr;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* Manages execution of an executable/command.
* @author sperkins
*/
public final class CommandExecutor {
/**
* The executable/command to be executed.
*/
private final File executable;
/**
* The error message produced by the executable/command.
*/
private String errorMessage;
/**
* The standard output of the executable/command.
*/
private List standardOutput = new ArrayList<>();
/**
* The standard error of the executable/command.
*/
private List standardError = new ArrayList<>();
/**
* Creates an instance of this class using the given executable/command.
* @param executable The executable/command to execute.
*/
public CommandExecutor(final File executable) {
this.executable = executable;
if (this.executable == null) {
throw new RuntimeException("No executable command provided.");
}
}
/**
* Executes an executable/command given some arguments.
* @param arguments The arguments to the executable/command.
* @return True if no exception occurred and the return value was normal.
* @throws InterruptedException Thrown if there was an error waiting for the executable/command to finish.
*/
public boolean execute(final List arguments) throws InterruptedException {
// Put the path to the executable at the front of the list of arguments.
arguments.add(0, executable.getAbsolutePath());
// Create the process builder with the list.
ProcessBuilder processBuilder = new ProcessBuilder(arguments);
Process process = null;
try {
process = processBuilder.start();
InputStream outputStream = process.getInputStream();
readStream(outputStream, standardOutput);
InputStream errorStream = process.getErrorStream();
readStream(errorStream, standardError);
process.waitFor();
} catch (IOException e) {
return false;
}
int exitValue = process.exitValue();
return exitValue == 0;
}
/**
* Reads an input stream into a list.
* @param stream The input stream.
* @param streamOutput The list to read the stream into.
* @throws IOException Thrown if there is an error reading the stream.
*/
private static void readStream(final InputStream stream, final List streamOutput) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(stream, StandardCharsets.UTF_8));
String line;
while ((line = reader.readLine()) != null) {
streamOutput.add(line);
}
}
/**
* Executes an executable/command with no arguments.
* @return True if no exception occurred.
* @throws InterruptedException Thrown if there was an error waiting for the executable/command to finish.
* @deprecated This method should not be used anymore, developers should instead provide an empty list to execute().
*/
@Deprecated
public boolean callExe() throws InterruptedException {
return execute(Collections.emptyList());
}
/**
* Gets the standard error reported by the executable/command.
* @return The standard error.
*/
public List getError() {
return standardError;
}
/**
* Gets the standard output reported by the command/executable.
* @return The standard output.
*/
public List getOutput() {
return standardOutput;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy