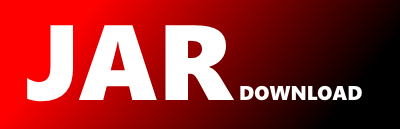
uk.ac.liv.pgb.analytica.lib.wrappedr.RManifest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analytica-lib Show documentation
Show all versions of analytica-lib Show documentation
Library allowing generation of plots and data from proteomics and
metabolomics mass spectrometry data through the use of R and java
methods.
The newest version!
package uk.ac.liv.pgb.analytica.lib.wrappedr;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.LinkedList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Describes the information contained in an R manifest file.
* @author sperkins
*/
public final class RManifest {
/**
* Pattern used to find entries in the manifest file.
*/
private static final Pattern KEY_VALUE_PATTERN = Pattern.compile("(\\w+):\\{(.*)\\}");
/**
* The number of expected parameters in a value when the key is 'MA' (see below).
*/
private static final int ARGUMENT_PARAMETER_DATA_LENGTH = 3;
/**
* Dependencies described in the manifest file.
*/
private List packageDependencies = new LinkedList<>();
/**
* The task name as described in the manifest file.
*/
private String taskName;
/**
* The task pretty name as described in the manifest file.
*/
private String taskPrettyName;
/**
* The task description as described in the manifest file.
*/
private String taskDescription;
/**
* The supported input formats as described in the manifest file.
*/
private List supportedFormats = new LinkedList<>();
/**
* The task's function name as described in the manifest file.
*/
private String functionName;
/**
* The task's mandatory arguments as described in the manifest file.
*/
private List mandatoryArguments = new LinkedList<>();
/**
* Gets the name of the task.
* @return The name of the task.
*/
public String getTaskName() {
return this.taskName;
}
/**
* Gets the pretty name of the task.
* @return The pretty name of the task.
*/
public String getTaskPrettyName() {
return this.taskPrettyName;
}
/**
* Gets the description of the task.
* @return The description of the task.
*/
public String getTaskDescription() {
return this.taskDescription;
}
/**
* Gets the task's supported input formats.
* @return The task's supported input formats.
*/
public List getTaskSupportedFormats() {
return this.supportedFormats;
}
/**
* Gets the task's package dependencies.
* @return The task's package dependencies.
*/
public List getTaskDependencies() {
return this.packageDependencies;
}
/**
* Gets the task's function name.
* @return The task's function name.
*/
public String getFunctionName() {
return this.functionName;
}
/**
* Reads data from an R manifest file into this instance.
* @param file The R manifest file.
*/
public void fillFromFile(final File file) {
try (BufferedReader reader = Files.newBufferedReader(Paths.get(file.getAbsolutePath()))) {
String line = null;
while ((line = reader.readLine()) != null) {
String trimmed = line.trim();
Matcher matcher = KEY_VALUE_PATTERN.matcher(trimmed);
if (matcher.matches()) {
String parameter = matcher.group(1);
String data = matcher.group(2);
if (data != null && !data.isEmpty()) {
String[] parameterData = data.split(",");
switch (parameter) {
case "DP":
for (String datum : parameterData) {
String dependency = datum != null ? datum.trim() : "";
if (dependency.isEmpty()) {
continue;
}
packageDependencies.add(dependency);
}
break;
case "TN":
taskName = parameterData[0];
break;
case "TPN":
taskPrettyName = parameterData[0];
break;
case "TD":
taskDescription = parameterData[0];
break;
case "SF":
for (String datum : parameterData) {
String format = datum != null ? datum.trim() : "";
if (format.isEmpty()) {
continue;
}
supportedFormats.add(format);
}
break;
case "FN":
functionName = parameterData[0];
break;
case "MA":
if (parameterData.length != ARGUMENT_PARAMETER_DATA_LENGTH) {
break;
}
Argument argument = new Argument();
argument.setArgumentName(parameterData[0]);
argument.setArgumentPrettyName(parameterData[1]);
argument.setArgumentDescription(parameterData[2]);
mandatoryArguments.add(argument);
break;
default:
break;
}
}
}
}
} catch (IOException ex) {
System.out.println("Error reading manifest: manifest will not be used.");
}
}
/**
* Information about an argument.
*/
private static class Argument {
/**
* Gets the argument name.
* @return The argument name.
*/
public String getArgumentName() {
return argumentName;
}
/**
* Sets the argument name.
* @param argumentName The argument name.
*/
public void setArgumentName(final String argumentName) {
this.argumentName = argumentName;
}
/**
* Gets the argument pretty name.
* @return The argument pretty name.
*/
public String getArgumentPrettyName() {
return argumentPrettyName;
}
/**
* Sets the argument pretty name.
* @param argumentPrettyName The argument pretty name.
*/
public void setArgumentPrettyName(final String argumentPrettyName) {
this.argumentPrettyName = argumentPrettyName;
}
/**
* Gets the argument description..
* @return The argument description..
*/
public String getArgumentDescription() {
return argumentDescription;
}
/**
* Sets the argument description.
* @param argumentDescription The argument description.
*/
public void setArgumentDescription(final String argumentDescription) {
this.argumentDescription = argumentDescription;
}
/**
* The argument name.
*/
private String argumentName;
/**
* The argument pretty name.
*/
private String argumentPrettyName;
/**
* The argument description.
*/
private String argumentDescription;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy