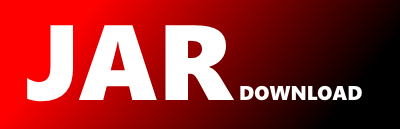
uk.ac.liv.pgb.analytica.lib.wrappedr.RSourceScanner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analytica-lib Show documentation
Show all versions of analytica-lib Show documentation
Library allowing generation of plots and data from proteomics and
metabolomics mass spectrometry data through the use of R and java
methods.
The newest version!
package uk.ac.liv.pgb.analytica.lib.wrappedr;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.LinkedList;
import java.util.List;
/**
* Manages the R source file and manifest files, both bundled and user provided.
* @author sperkins
*/
public final class RSourceScanner {
/**
* The singleton instance of this class.
*/
private static final RSourceScanner INSTANCE = new RSourceScanner();
/**
* The R source files, with any manifests, that have been successfully scanned.
*/
private final List scannedFiles = new LinkedList<>();
/**
* Gets the singleton instance of this class.
* @return The singleton instance of this class.
*/
public static RSourceScanner getInstance() {
return INSTANCE;
}
/**
* Gets the R source files, with any manifests, that have been successfully scanned.
* @return The R source files successfully scanned.
*/
public List getScannedFiles() {
return this.scannedFiles;
}
/**
* Private constructor to prevent external instantiation.
*/
private RSourceScanner() {
scanPackedResources();
//scanLiveSources():
}
/**
* Scan sources and manifest files in user directories.
*/
private void scanLiveSources() {
}
/**
* Scan sources and manifest files packed with the application.
*/
private void scanPackedResources() {
// Read the file contains an index of other files in the resources folder to read.
InputStream indexStream = this.getClass().getClassLoader().getResourceAsStream("R/index.txt");
if (indexStream == null) {
System.out.println("Index of R source files not found in resource folder.");
return;
}
// Create temporary directory for R source files.
File temporaryDir;
try {
temporaryDir = Files.createTempDirectory("wrapperR-sources").toFile();
} catch (IOException io) {
System.out.println("Error creating the temporary folder: embedded R source code will be unaccessible.");
return;
}
List indexContent = readStream(indexStream);
indexContent.stream().forEach(fileStub -> {
String sourceFile = fileStub.trim() + ".R";
String manifestFile = fileStub.trim() + ".mf";
if (!sourceFile.isEmpty() && !manifestFile.isEmpty()) {
File scannedSource = scanResource(sourceFile, temporaryDir);
File scannedManifest = scanResource(manifestFile, temporaryDir);
if (scannedSource != null && scannedSource.exists()) {
RSourceFile sourceObject = new RSourceFile();
sourceObject.setSourceFile(scannedSource);
if (scannedManifest != null && scannedManifest.exists()) {
RManifest manifest = new RManifest();
manifest.fillFromFile(scannedManifest);
sourceObject.setManifest(manifest);
}
scannedFiles.add(sourceObject);
}
}
});
temporaryDir.deleteOnExit();
}
/**
* Scans a single R resource and copies it to a reachable location.
* @param resourceName The resource name.
* @param tmpDir The directory to copy the resource to.
* @return The location of the copied resource.
*/
private File scanResource(final String resourceName, final File tmpDir) {
InputStream stream = this.getClass().getClassLoader().getResourceAsStream("R/" + resourceName);
if (stream == null) {
System.out.println("R source file or manifest file not found in resource folder: " + resourceName);
return null;
}
List lines = readStream(stream);
File tmpFile = null;
try {
tmpFile = Files.createFile(Paths.get(tmpDir.getAbsolutePath(), resourceName)).toFile();
} catch (IOException io) {
System.out.println("Error creating the temporary file: embedded R source code or manifest for this file will be inaccessible.");
return null;
}
tmpFile.deleteOnExit();
try (BufferedWriter writer = Files.newBufferedWriter(tmpFile.toPath())) {
for (String line : lines) {
writer.write(line);
writer.newLine();
}
} catch (IOException io) {
System.out.println("Error writing temporary R source file.");
return null;
}
return tmpFile;
}
/**
* Reads an input stream into a list.
* @param stream The input stream.
* @return The list created from the input stream.
*/
private List readStream(final InputStream stream) {
List lines = new LinkedList<>();
try (BufferedReader reader = new BufferedReader(new InputStreamReader(stream, StandardCharsets.UTF_8))) {
String line = null;
while ((line = reader.readLine()) != null) {
lines.add(line);
}
} catch (IOException ex) {
System.out.println("Could not read stream!");
}
return lines;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy