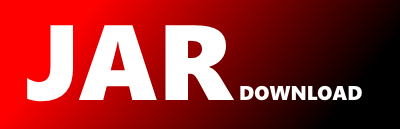
uk.ac.sussex.gdsc.smlm.function.gaussian.NbFixedGaussian2DFunction Maven / Gradle / Ivy
Show all versions of gdsc-smlm Show documentation
/*-
* #%L
* Genome Damage and Stability Centre SMLM Package
*
* Software for single molecule localisation microscopy (SMLM)
* %%
* Copyright (C) 2011 - 2023 Alex Herbert
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
package uk.ac.sussex.gdsc.smlm.function.gaussian;
/**
* Evaluates a 2-dimensional Gaussian function for a configured number of peaks.
*
* The single parameter x in the {@link #eval(int, double[])} function is assumed to be a linear
* index into 2-dimensional data. The dimensions of the data must be specified to allow unpacking to
* coordinates.
*
*
Data should be packed in descending dimension order, e.g. Y,X : Index for [x,y] = MaxX*y + x.
*/
public class NbFixedGaussian2DFunction extends FixedGaussian2DFunction {
/**
* Constructor.
*
* @param numberOfPeaks The number of peaks
* @param maxx The maximum x value of the 2-dimensional data (used to unpack a linear index into
* coordinates)
* @param maxy The maximum y value of the 2-dimensional data (used to unpack a linear index into
* coordinates)
*/
public NbFixedGaussian2DFunction(int numberOfPeaks, int maxx, int maxy) {
super(numberOfPeaks, maxx, maxy);
}
@Override
public Gaussian2DFunction copy() {
return new NbFixedGaussian2DFunction(numberOfPeaks, maxx, maxy);
}
/**
* Evaluates a 2-dimensional fixed circular Gaussian function for multiple peaks.
*
*
{@inheritDoc}
*/
@Override
public double eval(final int x, final double[] dyda) {
// Track the position of the parameters
int apos = 0;
int dydapos = 0;
// First parameter is the background level
double y = params[BACKGROUND];
// Unpack the predictor into the dimensions
final int x1 = x / maxx;
final int x0 = x % maxx;
for (int j = 0; j < numberOfPeaks; j++) {
y += gaussian(x0, x1, dyda, apos, dydapos, peakFactors[j]);
apos += PARAMETERS_PER_PEAK;
dydapos += GRADIENT_PARAMETERS_PER_PEAK;
}
return y;
}
@Override
public boolean evaluatesBackground() {
return false;
}
}