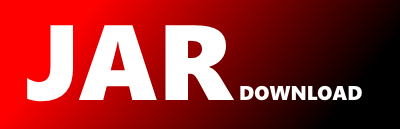
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: uk/ac/sussex/gdsc/smlm/tsf/tsf.proto
package uk.ac.sussex.gdsc.smlm.tsf;
public final class TSFProtos {
private TSFProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code TSF.FitMode}
*/
public enum FitMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ONEAXIS = 0;
*/
ONEAXIS(0),
/**
* TWOAXIS = 1;
*/
TWOAXIS(1),
/**
* TWOAXISANDTHETA = 2;
*/
TWOAXISANDTHETA(2),
;
/**
* ONEAXIS = 0;
*/
public static final int ONEAXIS_VALUE = 0;
/**
* TWOAXIS = 1;
*/
public static final int TWOAXIS_VALUE = 1;
/**
* TWOAXISANDTHETA = 2;
*/
public static final int TWOAXISANDTHETA_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static FitMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static FitMode forNumber(int value) {
switch (value) {
case 0: return ONEAXIS;
case 1: return TWOAXIS;
case 2: return TWOAXISANDTHETA;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
FitMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public FitMode findValueByNumber(int number) {
return FitMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.getDescriptor().getEnumTypes().get(0);
}
private static final FitMode[] VALUES = values();
public static FitMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private FitMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TSF.FitMode)
}
/**
* Protobuf enum {@code TSF.ThetaUnits}
*/
public enum ThetaUnits
implements com.google.protobuf.ProtocolMessageEnum {
/**
* DEGREES = 0;
*/
DEGREES(0),
/**
* RADIANS = 1;
*/
RADIANS(1),
;
/**
* DEGREES = 0;
*/
public static final int DEGREES_VALUE = 0;
/**
* RADIANS = 1;
*/
public static final int RADIANS_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ThetaUnits valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ThetaUnits forNumber(int value) {
switch (value) {
case 0: return DEGREES;
case 1: return RADIANS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ThetaUnits> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ThetaUnits findValueByNumber(int number) {
return ThetaUnits.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.getDescriptor().getEnumTypes().get(1);
}
private static final ThetaUnits[] VALUES = values();
public static ThetaUnits valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ThetaUnits(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TSF.ThetaUnits)
}
/**
* Protobuf enum {@code TSF.IntensityUnits}
*/
public enum IntensityUnits
implements com.google.protobuf.ProtocolMessageEnum {
/**
* COUNTS = 0;
*/
COUNTS(0),
/**
* PHOTONS = 1;
*/
PHOTONS(1),
;
/**
* COUNTS = 0;
*/
public static final int COUNTS_VALUE = 0;
/**
* PHOTONS = 1;
*/
public static final int PHOTONS_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IntensityUnits valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static IntensityUnits forNumber(int value) {
switch (value) {
case 0: return COUNTS;
case 1: return PHOTONS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
IntensityUnits> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public IntensityUnits findValueByNumber(int number) {
return IntensityUnits.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.getDescriptor().getEnumTypes().get(2);
}
private static final IntensityUnits[] VALUES = values();
public static IntensityUnits valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private IntensityUnits(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TSF.IntensityUnits)
}
/**
* Protobuf enum {@code TSF.LocationUnits}
*/
public enum LocationUnits
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NM = 0;
*/
NM(0),
/**
* UM = 1;
*/
UM(1),
/**
* PIXELS = 2;
*/
PIXELS(2),
;
/**
* NM = 0;
*/
public static final int NM_VALUE = 0;
/**
* UM = 1;
*/
public static final int UM_VALUE = 1;
/**
* PIXELS = 2;
*/
public static final int PIXELS_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LocationUnits valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static LocationUnits forNumber(int value) {
switch (value) {
case 0: return NM;
case 1: return UM;
case 2: return PIXELS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LocationUnits> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LocationUnits findValueByNumber(int number) {
return LocationUnits.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.getDescriptor().getEnumTypes().get(3);
}
private static final LocationUnits[] VALUES = values();
public static LocationUnits valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private LocationUnits(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TSF.LocationUnits)
}
/**
*
* The camera type
*
*
* Protobuf enum {@code TSF.CameraType}
*/
public enum CameraType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* CCD = 0;
*/
CCD(0),
/**
* EMCCD = 1;
*/
EMCCD(1),
/**
* SCMOS = 2;
*/
SCMOS(2),
;
/**
* CCD = 0;
*/
public static final int CCD_VALUE = 0;
/**
* EMCCD = 1;
*/
public static final int EMCCD_VALUE = 1;
/**
* SCMOS = 2;
*/
public static final int SCMOS_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CameraType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static CameraType forNumber(int value) {
switch (value) {
case 0: return CCD;
case 1: return EMCCD;
case 2: return SCMOS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CameraType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CameraType findValueByNumber(int number) {
return CameraType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.getDescriptor().getEnumTypes().get(4);
}
private static final CameraType[] VALUES = values();
public static CameraType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private CameraType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TSF.CameraType)
}
public interface FluorophoreTypeOrBuilder extends
// @@protoc_insertion_point(interface_extends:TSF.FluorophoreType)
com.google.protobuf.MessageOrBuilder {
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return The id.
*/
int getId();
/**
* optional string description = 2;
* @return Whether the description field is set.
*/
boolean hasDescription();
/**
* optional string description = 2;
* @return The description.
*/
java.lang.String getDescription();
/**
* optional string description = 2;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
* optional bool is_fiducial = 3;
* @return Whether the isFiducial field is set.
*/
boolean hasIsFiducial();
/**
* optional bool is_fiducial = 3;
* @return The isFiducial.
*/
boolean getIsFiducial();
}
/**
* Protobuf type {@code TSF.FluorophoreType}
*/
public static final class FluorophoreType extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:TSF.FluorophoreType)
FluorophoreTypeOrBuilder {
private static final long serialVersionUID = 0L;
// Use FluorophoreType.newBuilder() to construct.
private FluorophoreType(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FluorophoreType() {
description_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FluorophoreType();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_FluorophoreType_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_FluorophoreType_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private int id_ = 0;
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
* optional string description = 2;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string description = 2;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
}
}
/**
* optional string description = 2;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IS_FIDUCIAL_FIELD_NUMBER = 3;
private boolean isFiducial_ = false;
/**
* optional bool is_fiducial = 3;
* @return Whether the isFiducial field is set.
*/
@java.lang.Override
public boolean hasIsFiducial() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool is_fiducial = 3;
* @return The isFiducial.
*/
@java.lang.Override
public boolean getIsFiducial() {
return isFiducial_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, isFiducial_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, isFiducial_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType)) {
return super.equals(obj);
}
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType other = (uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription()
.equals(other.getDescription())) return false;
}
if (hasIsFiducial() != other.hasIsFiducial()) return false;
if (hasIsFiducial()) {
if (getIsFiducial()
!= other.getIsFiducial()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasIsFiducial()) {
hash = (37 * hash) + IS_FIDUCIAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsFiducial());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TSF.FluorophoreType}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:TSF.FluorophoreType)
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_FluorophoreType_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_FluorophoreType_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder.class);
}
// Construct using uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = 0;
description_ = "";
isFiducial_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_FluorophoreType_descriptor;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType getDefaultInstanceForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.getDefaultInstance();
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType build() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType buildPartial() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType result = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.isFiducial_ = isFiducial_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType) {
return mergeFrom((uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType other) {
if (other == uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasIsFiducial()) {
setIsFiducial(other.getIsFiducial());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
id_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
description_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
isFiducial_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int id_ ;
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Key for Spot.fluorophore_type.
*
*
* required int32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* optional string description = 2;
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string description = 2;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string description = 2;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string description = 2;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string description = 2;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string description = 2;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private boolean isFiducial_ ;
/**
* optional bool is_fiducial = 3;
* @return Whether the isFiducial field is set.
*/
@java.lang.Override
public boolean hasIsFiducial() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool is_fiducial = 3;
* @return The isFiducial.
*/
@java.lang.Override
public boolean getIsFiducial() {
return isFiducial_;
}
/**
* optional bool is_fiducial = 3;
* @param value The isFiducial to set.
* @return This builder for chaining.
*/
public Builder setIsFiducial(boolean value) {
isFiducial_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional bool is_fiducial = 3;
* @return This builder for chaining.
*/
public Builder clearIsFiducial() {
bitField0_ = (bitField0_ & ~0x00000004);
isFiducial_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:TSF.FluorophoreType)
}
// @@protoc_insertion_point(class_scope:TSF.FluorophoreType)
private static final uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType();
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FluorophoreType parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ROIOrBuilder extends
// @@protoc_insertion_point(interface_extends:TSF.ROI)
com.google.protobuf.MessageOrBuilder {
/**
* required int32 x = 1;
* @return Whether the x field is set.
*/
boolean hasX();
/**
* required int32 x = 1;
* @return The x.
*/
int getX();
/**
* required int32 y = 2;
* @return Whether the y field is set.
*/
boolean hasY();
/**
* required int32 y = 2;
* @return The y.
*/
int getY();
/**
* required int32 x_width = 3;
* @return Whether the xWidth field is set.
*/
boolean hasXWidth();
/**
* required int32 x_width = 3;
* @return The xWidth.
*/
int getXWidth();
/**
* required int32 y_width = 4;
* @return Whether the yWidth field is set.
*/
boolean hasYWidth();
/**
* required int32 y_width = 4;
* @return The yWidth.
*/
int getYWidth();
}
/**
*
* ROI in pixels, should be consistent with nr pixels given in SpotList
*
*
* Protobuf type {@code TSF.ROI}
*/
public static final class ROI extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:TSF.ROI)
ROIOrBuilder {
private static final long serialVersionUID = 0L;
// Use ROI.newBuilder() to construct.
private ROI(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ROI() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ROI();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_ROI_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_ROI_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder.class);
}
private int bitField0_;
public static final int X_FIELD_NUMBER = 1;
private int x_ = 0;
/**
* required int32 x = 1;
* @return Whether the x field is set.
*/
@java.lang.Override
public boolean hasX() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required int32 x = 1;
* @return The x.
*/
@java.lang.Override
public int getX() {
return x_;
}
public static final int Y_FIELD_NUMBER = 2;
private int y_ = 0;
/**
* required int32 y = 2;
* @return Whether the y field is set.
*/
@java.lang.Override
public boolean hasY() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required int32 y = 2;
* @return The y.
*/
@java.lang.Override
public int getY() {
return y_;
}
public static final int X_WIDTH_FIELD_NUMBER = 3;
private int xWidth_ = 0;
/**
* required int32 x_width = 3;
* @return Whether the xWidth field is set.
*/
@java.lang.Override
public boolean hasXWidth() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* required int32 x_width = 3;
* @return The xWidth.
*/
@java.lang.Override
public int getXWidth() {
return xWidth_;
}
public static final int Y_WIDTH_FIELD_NUMBER = 4;
private int yWidth_ = 0;
/**
* required int32 y_width = 4;
* @return Whether the yWidth field is set.
*/
@java.lang.Override
public boolean hasYWidth() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* required int32 y_width = 4;
* @return The yWidth.
*/
@java.lang.Override
public int getYWidth() {
return yWidth_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasX()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasY()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasXWidth()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasYWidth()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, x_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, y_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, xWidth_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, yWidth_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, x_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, y_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, xWidth_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, yWidth_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI)) {
return super.equals(obj);
}
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI other = (uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI) obj;
if (hasX() != other.hasX()) return false;
if (hasX()) {
if (getX()
!= other.getX()) return false;
}
if (hasY() != other.hasY()) return false;
if (hasY()) {
if (getY()
!= other.getY()) return false;
}
if (hasXWidth() != other.hasXWidth()) return false;
if (hasXWidth()) {
if (getXWidth()
!= other.getXWidth()) return false;
}
if (hasYWidth() != other.hasYWidth()) return false;
if (hasYWidth()) {
if (getYWidth()
!= other.getYWidth()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasX()) {
hash = (37 * hash) + X_FIELD_NUMBER;
hash = (53 * hash) + getX();
}
if (hasY()) {
hash = (37 * hash) + Y_FIELD_NUMBER;
hash = (53 * hash) + getY();
}
if (hasXWidth()) {
hash = (37 * hash) + X_WIDTH_FIELD_NUMBER;
hash = (53 * hash) + getXWidth();
}
if (hasYWidth()) {
hash = (37 * hash) + Y_WIDTH_FIELD_NUMBER;
hash = (53 * hash) + getYWidth();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ROI in pixels, should be consistent with nr pixels given in SpotList
*
*
* Protobuf type {@code TSF.ROI}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:TSF.ROI)
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_ROI_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_ROI_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder.class);
}
// Construct using uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
x_ = 0;
y_ = 0;
xWidth_ = 0;
yWidth_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_ROI_descriptor;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI getDefaultInstanceForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance();
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI build() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI buildPartial() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI result = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.x_ = x_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.y_ = y_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.xWidth_ = xWidth_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.yWidth_ = yWidth_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI) {
return mergeFrom((uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI other) {
if (other == uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance()) return this;
if (other.hasX()) {
setX(other.getX());
}
if (other.hasY()) {
setY(other.getY());
}
if (other.hasXWidth()) {
setXWidth(other.getXWidth());
}
if (other.hasYWidth()) {
setYWidth(other.getYWidth());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasX()) {
return false;
}
if (!hasY()) {
return false;
}
if (!hasXWidth()) {
return false;
}
if (!hasYWidth()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
x_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
y_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
xWidth_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
yWidth_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int x_ ;
/**
* required int32 x = 1;
* @return Whether the x field is set.
*/
@java.lang.Override
public boolean hasX() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required int32 x = 1;
* @return The x.
*/
@java.lang.Override
public int getX() {
return x_;
}
/**
* required int32 x = 1;
* @param value The x to set.
* @return This builder for chaining.
*/
public Builder setX(int value) {
x_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required int32 x = 1;
* @return This builder for chaining.
*/
public Builder clearX() {
bitField0_ = (bitField0_ & ~0x00000001);
x_ = 0;
onChanged();
return this;
}
private int y_ ;
/**
* required int32 y = 2;
* @return Whether the y field is set.
*/
@java.lang.Override
public boolean hasY() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required int32 y = 2;
* @return The y.
*/
@java.lang.Override
public int getY() {
return y_;
}
/**
* required int32 y = 2;
* @param value The y to set.
* @return This builder for chaining.
*/
public Builder setY(int value) {
y_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required int32 y = 2;
* @return This builder for chaining.
*/
public Builder clearY() {
bitField0_ = (bitField0_ & ~0x00000002);
y_ = 0;
onChanged();
return this;
}
private int xWidth_ ;
/**
* required int32 x_width = 3;
* @return Whether the xWidth field is set.
*/
@java.lang.Override
public boolean hasXWidth() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* required int32 x_width = 3;
* @return The xWidth.
*/
@java.lang.Override
public int getXWidth() {
return xWidth_;
}
/**
* required int32 x_width = 3;
* @param value The xWidth to set.
* @return This builder for chaining.
*/
public Builder setXWidth(int value) {
xWidth_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* required int32 x_width = 3;
* @return This builder for chaining.
*/
public Builder clearXWidth() {
bitField0_ = (bitField0_ & ~0x00000004);
xWidth_ = 0;
onChanged();
return this;
}
private int yWidth_ ;
/**
* required int32 y_width = 4;
* @return Whether the yWidth field is set.
*/
@java.lang.Override
public boolean hasYWidth() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* required int32 y_width = 4;
* @return The yWidth.
*/
@java.lang.Override
public int getYWidth() {
return yWidth_;
}
/**
* required int32 y_width = 4;
* @param value The yWidth to set.
* @return This builder for chaining.
*/
public Builder setYWidth(int value) {
yWidth_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* required int32 y_width = 4;
* @return This builder for chaining.
*/
public Builder clearYWidth() {
bitField0_ = (bitField0_ & ~0x00000008);
yWidth_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:TSF.ROI)
}
// @@protoc_insertion_point(class_scope:TSF.ROI)
private static final uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI();
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ROI parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SpotListOrBuilder extends
// @@protoc_insertion_point(interface_extends:TSF.SpotList)
com.google.protobuf.GeneratedMessageV3.
ExtendableMessageOrBuilder {
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return Whether the applicationId field is set.
*/
boolean hasApplicationId();
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return The applicationId.
*/
int getApplicationId();
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return Whether the filepath field is set.
*/
boolean hasFilepath();
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return The filepath.
*/
java.lang.String getFilepath();
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return The bytes for filepath.
*/
com.google.protobuf.ByteString
getFilepathBytes();
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return Whether the uid field is set.
*/
boolean hasUid();
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return The uid.
*/
long getUid();
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return Whether the nrPixelsX field is set.
*/
boolean hasNrPixelsX();
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return The nrPixelsX.
*/
int getNrPixelsX();
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return Whether the nrPixelsY field is set.
*/
boolean hasNrPixelsY();
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return The nrPixelsY.
*/
int getNrPixelsY();
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return Whether the pixelSize field is set.
*/
boolean hasPixelSize();
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return The pixelSize.
*/
float getPixelSize();
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return Whether the nrSpots field is set.
*/
boolean hasNrSpots();
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return The nrSpots.
*/
long getNrSpots();
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return Whether the boxSize field is set.
*/
boolean hasBoxSize();
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return The boxSize.
*/
int getBoxSize();
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return Whether the nrChannels field is set.
*/
boolean hasNrChannels();
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return The nrChannels.
*/
int getNrChannels();
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return Whether the nrFrames field is set.
*/
boolean hasNrFrames();
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return The nrFrames.
*/
int getNrFrames();
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return Whether the nrSlices field is set.
*/
boolean hasNrSlices();
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return The nrSlices.
*/
int getNrSlices();
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return Whether the nrPos field is set.
*/
boolean hasNrPos();
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return The nrPos.
*/
int getNrPos();
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
java.util.List
getFluorophoreTypesList();
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType getFluorophoreTypes(int index);
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
int getFluorophoreTypesCount();
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
java.util.List extends uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder>
getFluorophoreTypesOrBuilderList();
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder getFluorophoreTypesOrBuilder(
int index);
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return Whether the locationUnits field is set.
*/
boolean hasLocationUnits();
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return The locationUnits.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits getLocationUnits();
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return Whether the intensityUnits field is set.
*/
boolean hasIntensityUnits();
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return The intensityUnits.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits getIntensityUnits();
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return Whether the thetaUnits field is set.
*/
boolean hasThetaUnits();
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return The thetaUnits.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits getThetaUnits();
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return Whether the fitMode field is set.
*/
boolean hasFitMode();
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return The fitMode.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode getFitMode();
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return Whether the isTrack field is set.
*/
boolean hasIsTrack();
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return The isTrack.
*/
boolean getIsTrack();
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return A list containing the ecf.
*/
java.util.List getEcfList();
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return The count of ecf.
*/
int getEcfCount();
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @param index The index of the element to return.
* @return The ecf at the given index.
*/
double getEcf(int index);
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return A list containing the qe.
*/
java.util.List getQeList();
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return The count of qe.
*/
int getQeCount();
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @param index The index of the element to return.
* @return The qe at the given index.
*/
double getQe(int index);
/**
* optional .TSF.ROI roi = 29;
* @return Whether the roi field is set.
*/
boolean hasRoi();
/**
* optional .TSF.ROI roi = 29;
* @return The roi.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI getRoi();
/**
* optional .TSF.ROI roi = 29;
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder getRoiOrBuilder();
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return Whether the source field is set.
*/
boolean hasSource();
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return The source.
*/
java.lang.String getSource();
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return The bytes for source.
*/
com.google.protobuf.ByteString
getSourceBytes();
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return Whether the configuration field is set.
*/
boolean hasConfiguration();
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return The configuration.
*/
java.lang.String getConfiguration();
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return The bytes for configuration.
*/
com.google.protobuf.ByteString
getConfigurationBytes();
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return Whether the gain field is set.
*/
boolean hasGain();
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return The gain.
*/
double getGain();
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return Whether the exposureTime field is set.
*/
boolean hasExposureTime();
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return The exposureTime.
*/
double getExposureTime();
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return Whether the readNoise field is set.
*/
boolean hasReadNoise();
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return The readNoise.
*/
double getReadNoise();
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return Whether the bias field is set.
*/
boolean hasBias();
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return The bias.
*/
double getBias();
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return Whether the cameraType field is set.
*/
boolean hasCameraType();
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return The cameraType.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType getCameraType();
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return Whether the pSF field is set.
*/
boolean hasPSF();
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return The pSF.
*/
java.lang.String getPSF();
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return The bytes for pSF.
*/
com.google.protobuf.ByteString
getPSFBytes();
}
/**
* Protobuf type {@code TSF.SpotList}
*/
public static final class SpotList extends
com.google.protobuf.GeneratedMessageV3.ExtendableMessage<
SpotList> implements
// @@protoc_insertion_point(message_implements:TSF.SpotList)
SpotListOrBuilder {
private static final long serialVersionUID = 0L;
// Use SpotList.newBuilder() to construct.
private SpotList(com.google.protobuf.GeneratedMessageV3.ExtendableBuilder builder) {
super(builder);
}
private SpotList() {
applicationId_ = 1;
name_ = "";
filepath_ = "";
fluorophoreTypes_ = java.util.Collections.emptyList();
locationUnits_ = 0;
intensityUnits_ = 0;
thetaUnits_ = 0;
fitMode_ = 0;
ecf_ = emptyDoubleList();
qe_ = emptyDoubleList();
source_ = "";
configuration_ = "";
cameraType_ = 0;
pSF_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SpotList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_SpotList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_SpotList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.Builder.class);
}
private int bitField0_;
public static final int APPLICATION_ID_FIELD_NUMBER = 1;
private int applicationId_ = 1;
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return Whether the applicationId field is set.
*/
@java.lang.Override
public boolean hasApplicationId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return The applicationId.
*/
@java.lang.Override
public int getApplicationId() {
return applicationId_;
}
public static final int NAME_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILEPATH_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object filepath_ = "";
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return Whether the filepath field is set.
*/
@java.lang.Override
public boolean hasFilepath() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return The filepath.
*/
@java.lang.Override
public java.lang.String getFilepath() {
java.lang.Object ref = filepath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
filepath_ = s;
}
return s;
}
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return The bytes for filepath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFilepathBytes() {
java.lang.Object ref = filepath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filepath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UID_FIELD_NUMBER = 4;
private long uid_ = 0L;
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return Whether the uid field is set.
*/
@java.lang.Override
public boolean hasUid() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return The uid.
*/
@java.lang.Override
public long getUid() {
return uid_;
}
public static final int NR_PIXELS_X_FIELD_NUMBER = 5;
private int nrPixelsX_ = 0;
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return Whether the nrPixelsX field is set.
*/
@java.lang.Override
public boolean hasNrPixelsX() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return The nrPixelsX.
*/
@java.lang.Override
public int getNrPixelsX() {
return nrPixelsX_;
}
public static final int NR_PIXELS_Y_FIELD_NUMBER = 6;
private int nrPixelsY_ = 0;
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return Whether the nrPixelsY field is set.
*/
@java.lang.Override
public boolean hasNrPixelsY() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return The nrPixelsY.
*/
@java.lang.Override
public int getNrPixelsY() {
return nrPixelsY_;
}
public static final int PIXEL_SIZE_FIELD_NUMBER = 7;
private float pixelSize_ = 0F;
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return Whether the pixelSize field is set.
*/
@java.lang.Override
public boolean hasPixelSize() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return The pixelSize.
*/
@java.lang.Override
public float getPixelSize() {
return pixelSize_;
}
public static final int NR_SPOTS_FIELD_NUMBER = 8;
private long nrSpots_ = 0L;
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return Whether the nrSpots field is set.
*/
@java.lang.Override
public boolean hasNrSpots() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return The nrSpots.
*/
@java.lang.Override
public long getNrSpots() {
return nrSpots_;
}
public static final int BOX_SIZE_FIELD_NUMBER = 17;
private int boxSize_ = 0;
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return Whether the boxSize field is set.
*/
@java.lang.Override
public boolean hasBoxSize() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return The boxSize.
*/
@java.lang.Override
public int getBoxSize() {
return boxSize_;
}
public static final int NR_CHANNELS_FIELD_NUMBER = 18;
private int nrChannels_ = 0;
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return Whether the nrChannels field is set.
*/
@java.lang.Override
public boolean hasNrChannels() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return The nrChannels.
*/
@java.lang.Override
public int getNrChannels() {
return nrChannels_;
}
public static final int NR_FRAMES_FIELD_NUMBER = 19;
private int nrFrames_ = 0;
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return Whether the nrFrames field is set.
*/
@java.lang.Override
public boolean hasNrFrames() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return The nrFrames.
*/
@java.lang.Override
public int getNrFrames() {
return nrFrames_;
}
public static final int NR_SLICES_FIELD_NUMBER = 20;
private int nrSlices_ = 0;
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return Whether the nrSlices field is set.
*/
@java.lang.Override
public boolean hasNrSlices() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return The nrSlices.
*/
@java.lang.Override
public int getNrSlices() {
return nrSlices_;
}
public static final int NR_POS_FIELD_NUMBER = 21;
private int nrPos_ = 0;
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return Whether the nrPos field is set.
*/
@java.lang.Override
public boolean hasNrPos() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return The nrPos.
*/
@java.lang.Override
public int getNrPos() {
return nrPos_;
}
public static final int FLUOROPHORE_TYPES_FIELD_NUMBER = 26;
@SuppressWarnings("serial")
private java.util.List fluorophoreTypes_;
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
@java.lang.Override
public java.util.List getFluorophoreTypesList() {
return fluorophoreTypes_;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
@java.lang.Override
public java.util.List extends uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder>
getFluorophoreTypesOrBuilderList() {
return fluorophoreTypes_;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
@java.lang.Override
public int getFluorophoreTypesCount() {
return fluorophoreTypes_.size();
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType getFluorophoreTypes(int index) {
return fluorophoreTypes_.get(index);
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder getFluorophoreTypesOrBuilder(
int index) {
return fluorophoreTypes_.get(index);
}
public static final int LOCATION_UNITS_FIELD_NUMBER = 22;
private int locationUnits_ = 0;
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return Whether the locationUnits field is set.
*/
@java.lang.Override public boolean hasLocationUnits() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return The locationUnits.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits getLocationUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.forNumber(locationUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.NM : result;
}
public static final int INTENSITY_UNITS_FIELD_NUMBER = 23;
private int intensityUnits_ = 0;
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return Whether the intensityUnits field is set.
*/
@java.lang.Override public boolean hasIntensityUnits() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return The intensityUnits.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits getIntensityUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.forNumber(intensityUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.COUNTS : result;
}
public static final int THETA_UNITS_FIELD_NUMBER = 27;
private int thetaUnits_ = 0;
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return Whether the thetaUnits field is set.
*/
@java.lang.Override public boolean hasThetaUnits() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return The thetaUnits.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits getThetaUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits.forNumber(thetaUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits.DEGREES : result;
}
public static final int FIT_MODE_FIELD_NUMBER = 24;
private int fitMode_ = 0;
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return Whether the fitMode field is set.
*/
@java.lang.Override public boolean hasFitMode() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return The fitMode.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode getFitMode() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode.forNumber(fitMode_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode.ONEAXIS : result;
}
public static final int IS_TRACK_FIELD_NUMBER = 25;
private boolean isTrack_ = false;
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return Whether the isTrack field is set.
*/
@java.lang.Override
public boolean hasIsTrack() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return The isTrack.
*/
@java.lang.Override
public boolean getIsTrack() {
return isTrack_;
}
public static final int ECF_FIELD_NUMBER = 28;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.DoubleList ecf_;
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return A list containing the ecf.
*/
@java.lang.Override
public java.util.List
getEcfList() {
return ecf_;
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return The count of ecf.
*/
public int getEcfCount() {
return ecf_.size();
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @param index The index of the element to return.
* @return The ecf at the given index.
*/
public double getEcf(int index) {
return ecf_.getDouble(index);
}
public static final int QE_FIELD_NUMBER = 30;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.DoubleList qe_;
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return A list containing the qe.
*/
@java.lang.Override
public java.util.List
getQeList() {
return qe_;
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return The count of qe.
*/
public int getQeCount() {
return qe_.size();
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @param index The index of the element to return.
* @return The qe at the given index.
*/
public double getQe(int index) {
return qe_.getDouble(index);
}
public static final int ROI_FIELD_NUMBER = 29;
private uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI roi_;
/**
* optional .TSF.ROI roi = 29;
* @return Whether the roi field is set.
*/
@java.lang.Override
public boolean hasRoi() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
* optional .TSF.ROI roi = 29;
* @return The roi.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI getRoi() {
return roi_ == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance() : roi_;
}
/**
* optional .TSF.ROI roi = 29;
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder getRoiOrBuilder() {
return roi_ == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance() : roi_;
}
public static final int SOURCE_FIELD_NUMBER = 1501;
@SuppressWarnings("serial")
private volatile java.lang.Object source_ = "";
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return Whether the source field is set.
*/
@java.lang.Override
public boolean hasSource() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return The source.
*/
@java.lang.Override
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
source_ = s;
}
return s;
}
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return The bytes for source.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONFIGURATION_FIELD_NUMBER = 1502;
@SuppressWarnings("serial")
private volatile java.lang.Object configuration_ = "";
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return Whether the configuration field is set.
*/
@java.lang.Override
public boolean hasConfiguration() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return The configuration.
*/
@java.lang.Override
public java.lang.String getConfiguration() {
java.lang.Object ref = configuration_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
configuration_ = s;
}
return s;
}
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return The bytes for configuration.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getConfigurationBytes() {
java.lang.Object ref = configuration_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configuration_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GAIN_FIELD_NUMBER = 1503;
private double gain_ = 0D;
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return Whether the gain field is set.
*/
@java.lang.Override
public boolean hasGain() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return The gain.
*/
@java.lang.Override
public double getGain() {
return gain_;
}
public static final int EXPOSURE_TIME_FIELD_NUMBER = 1504;
private double exposureTime_ = 0D;
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return Whether the exposureTime field is set.
*/
@java.lang.Override
public boolean hasExposureTime() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return The exposureTime.
*/
@java.lang.Override
public double getExposureTime() {
return exposureTime_;
}
public static final int READ_NOISE_FIELD_NUMBER = 1505;
private double readNoise_ = 0D;
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return Whether the readNoise field is set.
*/
@java.lang.Override
public boolean hasReadNoise() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return The readNoise.
*/
@java.lang.Override
public double getReadNoise() {
return readNoise_;
}
public static final int BIAS_FIELD_NUMBER = 1506;
private double bias_ = 0D;
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return Whether the bias field is set.
*/
@java.lang.Override
public boolean hasBias() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return The bias.
*/
@java.lang.Override
public double getBias() {
return bias_;
}
public static final int CAMERA_TYPE_FIELD_NUMBER = 1509;
private int cameraType_ = 0;
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return Whether the cameraType field is set.
*/
@java.lang.Override public boolean hasCameraType() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return The cameraType.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType getCameraType() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType.forNumber(cameraType_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType.CCD : result;
}
public static final int PSF_FIELD_NUMBER = 1510;
@SuppressWarnings("serial")
private volatile java.lang.Object pSF_ = "";
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return Whether the pSF field is set.
*/
@java.lang.Override
public boolean hasPSF() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return The pSF.
*/
@java.lang.Override
public java.lang.String getPSF() {
java.lang.Object ref = pSF_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pSF_ = s;
}
return s;
}
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return The bytes for pSF.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPSFBytes() {
java.lang.Object ref = pSF_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pSF_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasApplicationId()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getFluorophoreTypesCount(); i++) {
if (!getFluorophoreTypes(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRoi()) {
if (!getRoi().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (!extensionsAreInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.ExtendableMessage.ExtensionWriter
extensionWriter = newExtensionWriter();
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, applicationId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, filepath_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(4, uid_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(5, nrPixelsX_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt32(6, nrPixelsY_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeFloat(7, pixelSize_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt64(8, nrSpots_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeInt32(17, boxSize_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeInt32(18, nrChannels_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeInt32(19, nrFrames_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeInt32(20, nrSlices_);
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeInt32(21, nrPos_);
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeEnum(22, locationUnits_);
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeEnum(23, intensityUnits_);
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeEnum(24, fitMode_);
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeBool(25, isTrack_);
}
for (int i = 0; i < fluorophoreTypes_.size(); i++) {
output.writeMessage(26, fluorophoreTypes_.get(i));
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeEnum(27, thetaUnits_);
}
for (int i = 0; i < ecf_.size(); i++) {
output.writeDouble(28, ecf_.getDouble(i));
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeMessage(29, getRoi());
}
for (int i = 0; i < qe_.size(); i++) {
output.writeDouble(30, qe_.getDouble(i));
}
if (((bitField0_ & 0x00080000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1501, source_);
}
if (((bitField0_ & 0x00100000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1502, configuration_);
}
if (((bitField0_ & 0x00200000) != 0)) {
output.writeDouble(1503, gain_);
}
if (((bitField0_ & 0x00400000) != 0)) {
output.writeDouble(1504, exposureTime_);
}
if (((bitField0_ & 0x00800000) != 0)) {
output.writeDouble(1505, readNoise_);
}
if (((bitField0_ & 0x01000000) != 0)) {
output.writeDouble(1506, bias_);
}
if (((bitField0_ & 0x02000000) != 0)) {
output.writeEnum(1509, cameraType_);
}
if (((bitField0_ & 0x04000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1510, pSF_);
}
extensionWriter.writeUntil(2048, output);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, applicationId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, filepath_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, uid_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, nrPixelsX_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, nrPixelsY_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(7, pixelSize_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, nrSpots_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(17, boxSize_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(18, nrChannels_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(19, nrFrames_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(20, nrSlices_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(21, nrPos_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, locationUnits_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(23, intensityUnits_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(24, fitMode_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(25, isTrack_);
}
for (int i = 0; i < fluorophoreTypes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, fluorophoreTypes_.get(i));
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(27, thetaUnits_);
}
{
int dataSize = 0;
dataSize = 8 * getEcfList().size();
size += dataSize;
size += 2 * getEcfList().size();
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(29, getRoi());
}
{
int dataSize = 0;
dataSize = 8 * getQeList().size();
size += dataSize;
size += 2 * getQeList().size();
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1501, source_);
}
if (((bitField0_ & 0x00100000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1502, configuration_);
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1503, gain_);
}
if (((bitField0_ & 0x00400000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1504, exposureTime_);
}
if (((bitField0_ & 0x00800000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1505, readNoise_);
}
if (((bitField0_ & 0x01000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1506, bias_);
}
if (((bitField0_ & 0x02000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1509, cameraType_);
}
if (((bitField0_ & 0x04000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1510, pSF_);
}
size += extensionsSerializedSize();
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList)) {
return super.equals(obj);
}
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList other = (uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList) obj;
if (hasApplicationId() != other.hasApplicationId()) return false;
if (hasApplicationId()) {
if (getApplicationId()
!= other.getApplicationId()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasFilepath() != other.hasFilepath()) return false;
if (hasFilepath()) {
if (!getFilepath()
.equals(other.getFilepath())) return false;
}
if (hasUid() != other.hasUid()) return false;
if (hasUid()) {
if (getUid()
!= other.getUid()) return false;
}
if (hasNrPixelsX() != other.hasNrPixelsX()) return false;
if (hasNrPixelsX()) {
if (getNrPixelsX()
!= other.getNrPixelsX()) return false;
}
if (hasNrPixelsY() != other.hasNrPixelsY()) return false;
if (hasNrPixelsY()) {
if (getNrPixelsY()
!= other.getNrPixelsY()) return false;
}
if (hasPixelSize() != other.hasPixelSize()) return false;
if (hasPixelSize()) {
if (java.lang.Float.floatToIntBits(getPixelSize())
!= java.lang.Float.floatToIntBits(
other.getPixelSize())) return false;
}
if (hasNrSpots() != other.hasNrSpots()) return false;
if (hasNrSpots()) {
if (getNrSpots()
!= other.getNrSpots()) return false;
}
if (hasBoxSize() != other.hasBoxSize()) return false;
if (hasBoxSize()) {
if (getBoxSize()
!= other.getBoxSize()) return false;
}
if (hasNrChannels() != other.hasNrChannels()) return false;
if (hasNrChannels()) {
if (getNrChannels()
!= other.getNrChannels()) return false;
}
if (hasNrFrames() != other.hasNrFrames()) return false;
if (hasNrFrames()) {
if (getNrFrames()
!= other.getNrFrames()) return false;
}
if (hasNrSlices() != other.hasNrSlices()) return false;
if (hasNrSlices()) {
if (getNrSlices()
!= other.getNrSlices()) return false;
}
if (hasNrPos() != other.hasNrPos()) return false;
if (hasNrPos()) {
if (getNrPos()
!= other.getNrPos()) return false;
}
if (!getFluorophoreTypesList()
.equals(other.getFluorophoreTypesList())) return false;
if (hasLocationUnits() != other.hasLocationUnits()) return false;
if (hasLocationUnits()) {
if (locationUnits_ != other.locationUnits_) return false;
}
if (hasIntensityUnits() != other.hasIntensityUnits()) return false;
if (hasIntensityUnits()) {
if (intensityUnits_ != other.intensityUnits_) return false;
}
if (hasThetaUnits() != other.hasThetaUnits()) return false;
if (hasThetaUnits()) {
if (thetaUnits_ != other.thetaUnits_) return false;
}
if (hasFitMode() != other.hasFitMode()) return false;
if (hasFitMode()) {
if (fitMode_ != other.fitMode_) return false;
}
if (hasIsTrack() != other.hasIsTrack()) return false;
if (hasIsTrack()) {
if (getIsTrack()
!= other.getIsTrack()) return false;
}
if (!getEcfList()
.equals(other.getEcfList())) return false;
if (!getQeList()
.equals(other.getQeList())) return false;
if (hasRoi() != other.hasRoi()) return false;
if (hasRoi()) {
if (!getRoi()
.equals(other.getRoi())) return false;
}
if (hasSource() != other.hasSource()) return false;
if (hasSource()) {
if (!getSource()
.equals(other.getSource())) return false;
}
if (hasConfiguration() != other.hasConfiguration()) return false;
if (hasConfiguration()) {
if (!getConfiguration()
.equals(other.getConfiguration())) return false;
}
if (hasGain() != other.hasGain()) return false;
if (hasGain()) {
if (java.lang.Double.doubleToLongBits(getGain())
!= java.lang.Double.doubleToLongBits(
other.getGain())) return false;
}
if (hasExposureTime() != other.hasExposureTime()) return false;
if (hasExposureTime()) {
if (java.lang.Double.doubleToLongBits(getExposureTime())
!= java.lang.Double.doubleToLongBits(
other.getExposureTime())) return false;
}
if (hasReadNoise() != other.hasReadNoise()) return false;
if (hasReadNoise()) {
if (java.lang.Double.doubleToLongBits(getReadNoise())
!= java.lang.Double.doubleToLongBits(
other.getReadNoise())) return false;
}
if (hasBias() != other.hasBias()) return false;
if (hasBias()) {
if (java.lang.Double.doubleToLongBits(getBias())
!= java.lang.Double.doubleToLongBits(
other.getBias())) return false;
}
if (hasCameraType() != other.hasCameraType()) return false;
if (hasCameraType()) {
if (cameraType_ != other.cameraType_) return false;
}
if (hasPSF() != other.hasPSF()) return false;
if (hasPSF()) {
if (!getPSF()
.equals(other.getPSF())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
if (!getExtensionFields().equals(other.getExtensionFields()))
return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasApplicationId()) {
hash = (37 * hash) + APPLICATION_ID_FIELD_NUMBER;
hash = (53 * hash) + getApplicationId();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasFilepath()) {
hash = (37 * hash) + FILEPATH_FIELD_NUMBER;
hash = (53 * hash) + getFilepath().hashCode();
}
if (hasUid()) {
hash = (37 * hash) + UID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUid());
}
if (hasNrPixelsX()) {
hash = (37 * hash) + NR_PIXELS_X_FIELD_NUMBER;
hash = (53 * hash) + getNrPixelsX();
}
if (hasNrPixelsY()) {
hash = (37 * hash) + NR_PIXELS_Y_FIELD_NUMBER;
hash = (53 * hash) + getNrPixelsY();
}
if (hasPixelSize()) {
hash = (37 * hash) + PIXEL_SIZE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getPixelSize());
}
if (hasNrSpots()) {
hash = (37 * hash) + NR_SPOTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNrSpots());
}
if (hasBoxSize()) {
hash = (37 * hash) + BOX_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getBoxSize();
}
if (hasNrChannels()) {
hash = (37 * hash) + NR_CHANNELS_FIELD_NUMBER;
hash = (53 * hash) + getNrChannels();
}
if (hasNrFrames()) {
hash = (37 * hash) + NR_FRAMES_FIELD_NUMBER;
hash = (53 * hash) + getNrFrames();
}
if (hasNrSlices()) {
hash = (37 * hash) + NR_SLICES_FIELD_NUMBER;
hash = (53 * hash) + getNrSlices();
}
if (hasNrPos()) {
hash = (37 * hash) + NR_POS_FIELD_NUMBER;
hash = (53 * hash) + getNrPos();
}
if (getFluorophoreTypesCount() > 0) {
hash = (37 * hash) + FLUOROPHORE_TYPES_FIELD_NUMBER;
hash = (53 * hash) + getFluorophoreTypesList().hashCode();
}
if (hasLocationUnits()) {
hash = (37 * hash) + LOCATION_UNITS_FIELD_NUMBER;
hash = (53 * hash) + locationUnits_;
}
if (hasIntensityUnits()) {
hash = (37 * hash) + INTENSITY_UNITS_FIELD_NUMBER;
hash = (53 * hash) + intensityUnits_;
}
if (hasThetaUnits()) {
hash = (37 * hash) + THETA_UNITS_FIELD_NUMBER;
hash = (53 * hash) + thetaUnits_;
}
if (hasFitMode()) {
hash = (37 * hash) + FIT_MODE_FIELD_NUMBER;
hash = (53 * hash) + fitMode_;
}
if (hasIsTrack()) {
hash = (37 * hash) + IS_TRACK_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsTrack());
}
if (getEcfCount() > 0) {
hash = (37 * hash) + ECF_FIELD_NUMBER;
hash = (53 * hash) + getEcfList().hashCode();
}
if (getQeCount() > 0) {
hash = (37 * hash) + QE_FIELD_NUMBER;
hash = (53 * hash) + getQeList().hashCode();
}
if (hasRoi()) {
hash = (37 * hash) + ROI_FIELD_NUMBER;
hash = (53 * hash) + getRoi().hashCode();
}
if (hasSource()) {
hash = (37 * hash) + SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSource().hashCode();
}
if (hasConfiguration()) {
hash = (37 * hash) + CONFIGURATION_FIELD_NUMBER;
hash = (53 * hash) + getConfiguration().hashCode();
}
if (hasGain()) {
hash = (37 * hash) + GAIN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getGain()));
}
if (hasExposureTime()) {
hash = (37 * hash) + EXPOSURE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getExposureTime()));
}
if (hasReadNoise()) {
hash = (37 * hash) + READ_NOISE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getReadNoise()));
}
if (hasBias()) {
hash = (37 * hash) + BIAS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getBias()));
}
if (hasCameraType()) {
hash = (37 * hash) + CAMERA_TYPE_FIELD_NUMBER;
hash = (53 * hash) + cameraType_;
}
if (hasPSF()) {
hash = (37 * hash) + PSF_FIELD_NUMBER;
hash = (53 * hash) + getPSF().hashCode();
}
hash = hashFields(hash, getExtensionFields());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TSF.SpotList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.ExtendableBuilder<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList, Builder> implements
// @@protoc_insertion_point(builder_implements:TSF.SpotList)
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_SpotList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_SpotList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.Builder.class);
}
// Construct using uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFluorophoreTypesFieldBuilder();
getRoiFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
applicationId_ = 1;
name_ = "";
filepath_ = "";
uid_ = 0L;
nrPixelsX_ = 0;
nrPixelsY_ = 0;
pixelSize_ = 0F;
nrSpots_ = 0L;
boxSize_ = 0;
nrChannels_ = 0;
nrFrames_ = 0;
nrSlices_ = 0;
nrPos_ = 0;
if (fluorophoreTypesBuilder_ == null) {
fluorophoreTypes_ = java.util.Collections.emptyList();
} else {
fluorophoreTypes_ = null;
fluorophoreTypesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00002000);
locationUnits_ = 0;
intensityUnits_ = 0;
thetaUnits_ = 0;
fitMode_ = 0;
isTrack_ = false;
ecf_ = emptyDoubleList();
qe_ = emptyDoubleList();
roi_ = null;
if (roiBuilder_ != null) {
roiBuilder_.dispose();
roiBuilder_ = null;
}
source_ = "";
configuration_ = "";
gain_ = 0D;
exposureTime_ = 0D;
readNoise_ = 0D;
bias_ = 0D;
cameraType_ = 0;
pSF_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_SpotList_descriptor;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList getDefaultInstanceForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.getDefaultInstance();
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList build() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList buildPartial() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList result = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList result) {
if (fluorophoreTypesBuilder_ == null) {
if (((bitField0_ & 0x00002000) != 0)) {
fluorophoreTypes_ = java.util.Collections.unmodifiableList(fluorophoreTypes_);
bitField0_ = (bitField0_ & ~0x00002000);
}
result.fluorophoreTypes_ = fluorophoreTypes_;
} else {
result.fluorophoreTypes_ = fluorophoreTypesBuilder_.build();
}
if (((bitField0_ & 0x00080000) != 0)) {
ecf_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00080000);
}
result.ecf_ = ecf_;
if (((bitField0_ & 0x00100000) != 0)) {
qe_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00100000);
}
result.qe_ = qe_;
}
private void buildPartial0(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.applicationId_ = applicationId_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.filepath_ = filepath_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.uid_ = uid_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.nrPixelsX_ = nrPixelsX_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.nrPixelsY_ = nrPixelsY_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.pixelSize_ = pixelSize_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.nrSpots_ = nrSpots_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.boxSize_ = boxSize_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.nrChannels_ = nrChannels_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.nrFrames_ = nrFrames_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.nrSlices_ = nrSlices_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.nrPos_ = nrPos_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.locationUnits_ = locationUnits_;
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.intensityUnits_ = intensityUnits_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.thetaUnits_ = thetaUnits_;
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.fitMode_ = fitMode_;
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.isTrack_ = isTrack_;
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.roi_ = roiBuilder_ == null
? roi_
: roiBuilder_.build();
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.source_ = source_;
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.configuration_ = configuration_;
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.gain_ = gain_;
to_bitField0_ |= 0x00200000;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.exposureTime_ = exposureTime_;
to_bitField0_ |= 0x00400000;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.readNoise_ = readNoise_;
to_bitField0_ |= 0x00800000;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.bias_ = bias_;
to_bitField0_ |= 0x01000000;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.cameraType_ = cameraType_;
to_bitField0_ |= 0x02000000;
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.pSF_ = pSF_;
to_bitField0_ |= 0x04000000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList) {
return mergeFrom((uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList other) {
if (other == uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList.getDefaultInstance()) return this;
if (other.hasApplicationId()) {
setApplicationId(other.getApplicationId());
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasFilepath()) {
filepath_ = other.filepath_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasUid()) {
setUid(other.getUid());
}
if (other.hasNrPixelsX()) {
setNrPixelsX(other.getNrPixelsX());
}
if (other.hasNrPixelsY()) {
setNrPixelsY(other.getNrPixelsY());
}
if (other.hasPixelSize()) {
setPixelSize(other.getPixelSize());
}
if (other.hasNrSpots()) {
setNrSpots(other.getNrSpots());
}
if (other.hasBoxSize()) {
setBoxSize(other.getBoxSize());
}
if (other.hasNrChannels()) {
setNrChannels(other.getNrChannels());
}
if (other.hasNrFrames()) {
setNrFrames(other.getNrFrames());
}
if (other.hasNrSlices()) {
setNrSlices(other.getNrSlices());
}
if (other.hasNrPos()) {
setNrPos(other.getNrPos());
}
if (fluorophoreTypesBuilder_ == null) {
if (!other.fluorophoreTypes_.isEmpty()) {
if (fluorophoreTypes_.isEmpty()) {
fluorophoreTypes_ = other.fluorophoreTypes_;
bitField0_ = (bitField0_ & ~0x00002000);
} else {
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.addAll(other.fluorophoreTypes_);
}
onChanged();
}
} else {
if (!other.fluorophoreTypes_.isEmpty()) {
if (fluorophoreTypesBuilder_.isEmpty()) {
fluorophoreTypesBuilder_.dispose();
fluorophoreTypesBuilder_ = null;
fluorophoreTypes_ = other.fluorophoreTypes_;
bitField0_ = (bitField0_ & ~0x00002000);
fluorophoreTypesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFluorophoreTypesFieldBuilder() : null;
} else {
fluorophoreTypesBuilder_.addAllMessages(other.fluorophoreTypes_);
}
}
}
if (other.hasLocationUnits()) {
setLocationUnits(other.getLocationUnits());
}
if (other.hasIntensityUnits()) {
setIntensityUnits(other.getIntensityUnits());
}
if (other.hasThetaUnits()) {
setThetaUnits(other.getThetaUnits());
}
if (other.hasFitMode()) {
setFitMode(other.getFitMode());
}
if (other.hasIsTrack()) {
setIsTrack(other.getIsTrack());
}
if (!other.ecf_.isEmpty()) {
if (ecf_.isEmpty()) {
ecf_ = other.ecf_;
bitField0_ = (bitField0_ & ~0x00080000);
} else {
ensureEcfIsMutable();
ecf_.addAll(other.ecf_);
}
onChanged();
}
if (!other.qe_.isEmpty()) {
if (qe_.isEmpty()) {
qe_ = other.qe_;
bitField0_ = (bitField0_ & ~0x00100000);
} else {
ensureQeIsMutable();
qe_.addAll(other.qe_);
}
onChanged();
}
if (other.hasRoi()) {
mergeRoi(other.getRoi());
}
if (other.hasSource()) {
source_ = other.source_;
bitField0_ |= 0x00400000;
onChanged();
}
if (other.hasConfiguration()) {
configuration_ = other.configuration_;
bitField0_ |= 0x00800000;
onChanged();
}
if (other.hasGain()) {
setGain(other.getGain());
}
if (other.hasExposureTime()) {
setExposureTime(other.getExposureTime());
}
if (other.hasReadNoise()) {
setReadNoise(other.getReadNoise());
}
if (other.hasBias()) {
setBias(other.getBias());
}
if (other.hasCameraType()) {
setCameraType(other.getCameraType());
}
if (other.hasPSF()) {
pSF_ = other.pSF_;
bitField0_ |= 0x20000000;
onChanged();
}
this.mergeExtensionFields(other);
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasApplicationId()) {
return false;
}
for (int i = 0; i < getFluorophoreTypesCount(); i++) {
if (!getFluorophoreTypes(i).isInitialized()) {
return false;
}
}
if (hasRoi()) {
if (!getRoi().isInitialized()) {
return false;
}
}
if (!extensionsAreInitialized()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
applicationId_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
name_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
filepath_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 32: {
uid_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
nrPixelsX_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
case 48: {
nrPixelsY_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case 48
case 61: {
pixelSize_ = input.readFloat();
bitField0_ |= 0x00000040;
break;
} // case 61
case 64: {
nrSpots_ = input.readInt64();
bitField0_ |= 0x00000080;
break;
} // case 64
case 136: {
boxSize_ = input.readInt32();
bitField0_ |= 0x00000100;
break;
} // case 136
case 144: {
nrChannels_ = input.readInt32();
bitField0_ |= 0x00000200;
break;
} // case 144
case 152: {
nrFrames_ = input.readInt32();
bitField0_ |= 0x00000400;
break;
} // case 152
case 160: {
nrSlices_ = input.readInt32();
bitField0_ |= 0x00000800;
break;
} // case 160
case 168: {
nrPos_ = input.readInt32();
bitField0_ |= 0x00001000;
break;
} // case 168
case 176: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(22, tmpRaw);
} else {
locationUnits_ = tmpRaw;
bitField0_ |= 0x00004000;
}
break;
} // case 176
case 184: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(23, tmpRaw);
} else {
intensityUnits_ = tmpRaw;
bitField0_ |= 0x00008000;
}
break;
} // case 184
case 192: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(24, tmpRaw);
} else {
fitMode_ = tmpRaw;
bitField0_ |= 0x00020000;
}
break;
} // case 192
case 200: {
isTrack_ = input.readBool();
bitField0_ |= 0x00040000;
break;
} // case 200
case 210: {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType m =
input.readMessage(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.PARSER,
extensionRegistry);
if (fluorophoreTypesBuilder_ == null) {
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.add(m);
} else {
fluorophoreTypesBuilder_.addMessage(m);
}
break;
} // case 210
case 216: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(27, tmpRaw);
} else {
thetaUnits_ = tmpRaw;
bitField0_ |= 0x00010000;
}
break;
} // case 216
case 225: {
double v = input.readDouble();
ensureEcfIsMutable();
ecf_.addDouble(v);
break;
} // case 225
case 226: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureEcfIsMutable();
while (input.getBytesUntilLimit() > 0) {
ecf_.addDouble(input.readDouble());
}
input.popLimit(limit);
break;
} // case 226
case 234: {
input.readMessage(
getRoiFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00200000;
break;
} // case 234
case 241: {
double v = input.readDouble();
ensureQeIsMutable();
qe_.addDouble(v);
break;
} // case 241
case 242: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureQeIsMutable();
while (input.getBytesUntilLimit() > 0) {
qe_.addDouble(input.readDouble());
}
input.popLimit(limit);
break;
} // case 242
case 12010: {
source_ = input.readBytes();
bitField0_ |= 0x00400000;
break;
} // case 12010
case 12018: {
configuration_ = input.readBytes();
bitField0_ |= 0x00800000;
break;
} // case 12018
case 12025: {
gain_ = input.readDouble();
bitField0_ |= 0x01000000;
break;
} // case 12025
case 12033: {
exposureTime_ = input.readDouble();
bitField0_ |= 0x02000000;
break;
} // case 12033
case 12041: {
readNoise_ = input.readDouble();
bitField0_ |= 0x04000000;
break;
} // case 12041
case 12049: {
bias_ = input.readDouble();
bitField0_ |= 0x08000000;
break;
} // case 12049
case 12072: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1509, tmpRaw);
} else {
cameraType_ = tmpRaw;
bitField0_ |= 0x10000000;
}
break;
} // case 12072
case 12082: {
pSF_ = input.readBytes();
bitField0_ |= 0x20000000;
break;
} // case 12082
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int applicationId_ = 1;
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return Whether the applicationId field is set.
*/
@java.lang.Override
public boolean hasApplicationId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return The applicationId.
*/
@java.lang.Override
public int getApplicationId() {
return applicationId_;
}
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @param value The applicationId to set.
* @return This builder for chaining.
*/
public Builder setApplicationId(int value) {
applicationId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* UID for the application that generated these data
* Request a UID from nico at cmp.ucsf.edu or use 1
*
*
* required int32 application_id = 1 [default = 1];
* @return This builder for chaining.
*/
public Builder clearApplicationId() {
bitField0_ = (bitField0_ & ~0x00000001);
applicationId_ = 1;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* name identifying the original dataset
*
*
* optional string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object filepath_ = "";
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return Whether the filepath field is set.
*/
public boolean hasFilepath() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return The filepath.
*/
public java.lang.String getFilepath() {
java.lang.Object ref = filepath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
filepath_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return The bytes for filepath.
*/
public com.google.protobuf.ByteString
getFilepathBytes() {
java.lang.Object ref = filepath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filepath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @param value The filepath to set.
* @return This builder for chaining.
*/
public Builder setFilepath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
filepath_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @return This builder for chaining.
*/
public Builder clearFilepath() {
filepath_ = getDefaultInstance().getFilepath();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* path to the image data used to generate these spot data
*
*
* optional string filepath = 3;
* @param value The bytes for filepath to set.
* @return This builder for chaining.
*/
public Builder setFilepathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
filepath_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private long uid_ ;
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return Whether the uid field is set.
*/
@java.lang.Override
public boolean hasUid() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return The uid.
*/
@java.lang.Override
public long getUid() {
return uid_;
}
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @param value The uid to set.
* @return This builder for chaining.
*/
public Builder setUid(long value) {
uid_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Unique ID, can be used by application to link to original data
*
*
* optional int64 uid = 4;
* @return This builder for chaining.
*/
public Builder clearUid() {
bitField0_ = (bitField0_ & ~0x00000008);
uid_ = 0L;
onChanged();
return this;
}
private int nrPixelsX_ ;
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return Whether the nrPixelsX field is set.
*/
@java.lang.Override
public boolean hasNrPixelsX() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return The nrPixelsX.
*/
@java.lang.Override
public int getNrPixelsX() {
return nrPixelsX_;
}
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @param value The nrPixelsX to set.
* @return This builder for chaining.
*/
public Builder setNrPixelsX(int value) {
nrPixelsX_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* nr pixels in x of original data
*
*
* optional int32 nr_pixels_x = 5;
* @return This builder for chaining.
*/
public Builder clearNrPixelsX() {
bitField0_ = (bitField0_ & ~0x00000010);
nrPixelsX_ = 0;
onChanged();
return this;
}
private int nrPixelsY_ ;
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return Whether the nrPixelsY field is set.
*/
@java.lang.Override
public boolean hasNrPixelsY() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return The nrPixelsY.
*/
@java.lang.Override
public int getNrPixelsY() {
return nrPixelsY_;
}
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @param value The nrPixelsY to set.
* @return This builder for chaining.
*/
public Builder setNrPixelsY(int value) {
nrPixelsY_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* nr pixels in y of original data
*
*
* optional int32 nr_pixels_y = 6;
* @return This builder for chaining.
*/
public Builder clearNrPixelsY() {
bitField0_ = (bitField0_ & ~0x00000020);
nrPixelsY_ = 0;
onChanged();
return this;
}
private float pixelSize_ ;
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return Whether the pixelSize field is set.
*/
@java.lang.Override
public boolean hasPixelSize() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return The pixelSize.
*/
@java.lang.Override
public float getPixelSize() {
return pixelSize_;
}
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @param value The pixelSize to set.
* @return This builder for chaining.
*/
public Builder setPixelSize(float value) {
pixelSize_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* pixel size in nanometer
*
*
* optional float pixel_size = 7;
* @return This builder for chaining.
*/
public Builder clearPixelSize() {
bitField0_ = (bitField0_ & ~0x00000040);
pixelSize_ = 0F;
onChanged();
return this;
}
private long nrSpots_ ;
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return Whether the nrSpots field is set.
*/
@java.lang.Override
public boolean hasNrSpots() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return The nrSpots.
*/
@java.lang.Override
public long getNrSpots() {
return nrSpots_;
}
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @param value The nrSpots to set.
* @return This builder for chaining.
*/
public Builder setNrSpots(long value) {
nrSpots_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* number of spots in this data set
*
*
* optional int64 nr_spots = 8;
* @return This builder for chaining.
*/
public Builder clearNrSpots() {
bitField0_ = (bitField0_ & ~0x00000080);
nrSpots_ = 0L;
onChanged();
return this;
}
private int boxSize_ ;
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return Whether the boxSize field is set.
*/
@java.lang.Override
public boolean hasBoxSize() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return The boxSize.
*/
@java.lang.Override
public int getBoxSize() {
return boxSize_;
}
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @param value The boxSize to set.
* @return This builder for chaining.
*/
public Builder setBoxSize(int value) {
boxSize_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* size (in pixels) of rectangular box used in Gaussian fitting
*
*
* optional int32 box_size = 17;
* @return This builder for chaining.
*/
public Builder clearBoxSize() {
bitField0_ = (bitField0_ & ~0x00000100);
boxSize_ = 0;
onChanged();
return this;
}
private int nrChannels_ ;
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return Whether the nrChannels field is set.
*/
@java.lang.Override
public boolean hasNrChannels() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return The nrChannels.
*/
@java.lang.Override
public int getNrChannels() {
return nrChannels_;
}
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @param value The nrChannels to set.
* @return This builder for chaining.
*/
public Builder setNrChannels(int value) {
nrChannels_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Nr of channels in the original data set
*
*
* optional int32 nr_channels = 18;
* @return This builder for chaining.
*/
public Builder clearNrChannels() {
bitField0_ = (bitField0_ & ~0x00000200);
nrChannels_ = 0;
onChanged();
return this;
}
private int nrFrames_ ;
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return Whether the nrFrames field is set.
*/
@java.lang.Override
public boolean hasNrFrames() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return The nrFrames.
*/
@java.lang.Override
public int getNrFrames() {
return nrFrames_;
}
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @param value The nrFrames to set.
* @return This builder for chaining.
*/
public Builder setNrFrames(int value) {
nrFrames_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* Nr of frames in the original data set
*
*
* optional int32 nr_frames = 19;
* @return This builder for chaining.
*/
public Builder clearNrFrames() {
bitField0_ = (bitField0_ & ~0x00000400);
nrFrames_ = 0;
onChanged();
return this;
}
private int nrSlices_ ;
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return Whether the nrSlices field is set.
*/
@java.lang.Override
public boolean hasNrSlices() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return The nrSlices.
*/
@java.lang.Override
public int getNrSlices() {
return nrSlices_;
}
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @param value The nrSlices to set.
* @return This builder for chaining.
*/
public Builder setNrSlices(int value) {
nrSlices_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* Nr of slices in the original data set
*
*
* optional int32 nr_slices = 20;
* @return This builder for chaining.
*/
public Builder clearNrSlices() {
bitField0_ = (bitField0_ & ~0x00000800);
nrSlices_ = 0;
onChanged();
return this;
}
private int nrPos_ ;
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return Whether the nrPos field is set.
*/
@java.lang.Override
public boolean hasNrPos() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return The nrPos.
*/
@java.lang.Override
public int getNrPos() {
return nrPos_;
}
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @param value The nrPos to set.
* @return This builder for chaining.
*/
public Builder setNrPos(int value) {
nrPos_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* Nr of positions in the original data set
*
*
* optional int32 nr_pos = 21;
* @return This builder for chaining.
*/
public Builder clearNrPos() {
bitField0_ = (bitField0_ & ~0x00001000);
nrPos_ = 0;
onChanged();
return this;
}
private java.util.List fluorophoreTypes_ =
java.util.Collections.emptyList();
private void ensureFluorophoreTypesIsMutable() {
if (!((bitField0_ & 0x00002000) != 0)) {
fluorophoreTypes_ = new java.util.ArrayList(fluorophoreTypes_);
bitField0_ |= 0x00002000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder> fluorophoreTypesBuilder_;
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public java.util.List getFluorophoreTypesList() {
if (fluorophoreTypesBuilder_ == null) {
return java.util.Collections.unmodifiableList(fluorophoreTypes_);
} else {
return fluorophoreTypesBuilder_.getMessageList();
}
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public int getFluorophoreTypesCount() {
if (fluorophoreTypesBuilder_ == null) {
return fluorophoreTypes_.size();
} else {
return fluorophoreTypesBuilder_.getCount();
}
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType getFluorophoreTypes(int index) {
if (fluorophoreTypesBuilder_ == null) {
return fluorophoreTypes_.get(index);
} else {
return fluorophoreTypesBuilder_.getMessage(index);
}
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder setFluorophoreTypes(
int index, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType value) {
if (fluorophoreTypesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.set(index, value);
onChanged();
} else {
fluorophoreTypesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder setFluorophoreTypes(
int index, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder builderForValue) {
if (fluorophoreTypesBuilder_ == null) {
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.set(index, builderForValue.build());
onChanged();
} else {
fluorophoreTypesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder addFluorophoreTypes(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType value) {
if (fluorophoreTypesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.add(value);
onChanged();
} else {
fluorophoreTypesBuilder_.addMessage(value);
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder addFluorophoreTypes(
int index, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType value) {
if (fluorophoreTypesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.add(index, value);
onChanged();
} else {
fluorophoreTypesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder addFluorophoreTypes(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder builderForValue) {
if (fluorophoreTypesBuilder_ == null) {
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.add(builderForValue.build());
onChanged();
} else {
fluorophoreTypesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder addFluorophoreTypes(
int index, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder builderForValue) {
if (fluorophoreTypesBuilder_ == null) {
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.add(index, builderForValue.build());
onChanged();
} else {
fluorophoreTypesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder addAllFluorophoreTypes(
java.lang.Iterable extends uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType> values) {
if (fluorophoreTypesBuilder_ == null) {
ensureFluorophoreTypesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fluorophoreTypes_);
onChanged();
} else {
fluorophoreTypesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder clearFluorophoreTypes() {
if (fluorophoreTypesBuilder_ == null) {
fluorophoreTypes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
} else {
fluorophoreTypesBuilder_.clear();
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public Builder removeFluorophoreTypes(int index) {
if (fluorophoreTypesBuilder_ == null) {
ensureFluorophoreTypesIsMutable();
fluorophoreTypes_.remove(index);
onChanged();
} else {
fluorophoreTypesBuilder_.remove(index);
}
return this;
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder getFluorophoreTypesBuilder(
int index) {
return getFluorophoreTypesFieldBuilder().getBuilder(index);
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder getFluorophoreTypesOrBuilder(
int index) {
if (fluorophoreTypesBuilder_ == null) {
return fluorophoreTypes_.get(index); } else {
return fluorophoreTypesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public java.util.List extends uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder>
getFluorophoreTypesOrBuilderList() {
if (fluorophoreTypesBuilder_ != null) {
return fluorophoreTypesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fluorophoreTypes_);
}
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder addFluorophoreTypesBuilder() {
return getFluorophoreTypesFieldBuilder().addBuilder(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.getDefaultInstance());
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder addFluorophoreTypesBuilder(
int index) {
return getFluorophoreTypesFieldBuilder().addBuilder(
index, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.getDefaultInstance());
}
/**
*
* Fluorophore type characterizations. If you use the fluorophore type field,
* you must add a FluorophoreType message for each used id.
*
*
* repeated .TSF.FluorophoreType fluorophore_types = 26;
*/
public java.util.List
getFluorophoreTypesBuilderList() {
return getFluorophoreTypesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder>
getFluorophoreTypesFieldBuilder() {
if (fluorophoreTypesBuilder_ == null) {
fluorophoreTypesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreType.Builder, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FluorophoreTypeOrBuilder>(
fluorophoreTypes_,
((bitField0_ & 0x00002000) != 0),
getParentForChildren(),
isClean());
fluorophoreTypes_ = null;
}
return fluorophoreTypesBuilder_;
}
private int locationUnits_ = 0;
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return Whether the locationUnits field is set.
*/
@java.lang.Override public boolean hasLocationUnits() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return The locationUnits.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits getLocationUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.forNumber(locationUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.NM : result;
}
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @param value The locationUnits to set.
* @return This builder for chaining.
*/
public Builder setLocationUnits(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
locationUnits_ = value.getNumber();
onChanged();
return this;
}
/**
*
* If units will always be the same for all spots, then use these units tags,
* otherwise use the unit tags with each spot
*
*
* optional .TSF.LocationUnits location_units = 22;
* @return This builder for chaining.
*/
public Builder clearLocationUnits() {
bitField0_ = (bitField0_ & ~0x00004000);
locationUnits_ = 0;
onChanged();
return this;
}
private int intensityUnits_ = 0;
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return Whether the intensityUnits field is set.
*/
@java.lang.Override public boolean hasIntensityUnits() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return The intensityUnits.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits getIntensityUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.forNumber(intensityUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.COUNTS : result;
}
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @param value The intensityUnits to set.
* @return This builder for chaining.
*/
public Builder setIntensityUnits(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
intensityUnits_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .TSF.IntensityUnits intensity_units = 23;
* @return This builder for chaining.
*/
public Builder clearIntensityUnits() {
bitField0_ = (bitField0_ & ~0x00008000);
intensityUnits_ = 0;
onChanged();
return this;
}
private int thetaUnits_ = 0;
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return Whether the thetaUnits field is set.
*/
@java.lang.Override public boolean hasThetaUnits() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return The thetaUnits.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits getThetaUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits.forNumber(thetaUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits.DEGREES : result;
}
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @param value The thetaUnits to set.
* @return This builder for chaining.
*/
public Builder setThetaUnits(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ThetaUnits value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
thetaUnits_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .TSF.ThetaUnits theta_units = 27;
* @return This builder for chaining.
*/
public Builder clearThetaUnits() {
bitField0_ = (bitField0_ & ~0x00010000);
thetaUnits_ = 0;
onChanged();
return this;
}
private int fitMode_ = 0;
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return Whether the fitMode field is set.
*/
@java.lang.Override public boolean hasFitMode() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return The fitMode.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode getFitMode() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode.forNumber(fitMode_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode.ONEAXIS : result;
}
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @param value The fitMode to set.
* @return This builder for chaining.
*/
public Builder setFitMode(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.FitMode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
fitMode_ = value.getNumber();
onChanged();
return this;
}
/**
*
* If fitmode will always be the same for all spots, then use this fitmode
* otherwise use the fitmode with each spot
*
*
* optional .TSF.FitMode fit_mode = 24;
* @return This builder for chaining.
*/
public Builder clearFitMode() {
bitField0_ = (bitField0_ & ~0x00020000);
fitMode_ = 0;
onChanged();
return this;
}
private boolean isTrack_ ;
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return Whether the isTrack field is set.
*/
@java.lang.Override
public boolean hasIsTrack() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return The isTrack.
*/
@java.lang.Override
public boolean getIsTrack() {
return isTrack_;
}
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @param value The isTrack to set.
* @return This builder for chaining.
*/
public Builder setIsTrack(boolean value) {
isTrack_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* flag indicating whether this is a sequence of spot data in consecutive
* time frames thought to originate from the same entity
*
*
* optional bool is_track = 25 [default = false];
* @return This builder for chaining.
*/
public Builder clearIsTrack() {
bitField0_ = (bitField0_ & ~0x00040000);
isTrack_ = false;
onChanged();
return this;
}
private com.google.protobuf.Internal.DoubleList ecf_ = emptyDoubleList();
private void ensureEcfIsMutable() {
if (!((bitField0_ & 0x00080000) != 0)) {
ecf_ = mutableCopy(ecf_);
bitField0_ |= 0x00080000;
}
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return A list containing the ecf.
*/
public java.util.List
getEcfList() {
return ((bitField0_ & 0x00080000) != 0) ?
java.util.Collections.unmodifiableList(ecf_) : ecf_;
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return The count of ecf.
*/
public int getEcfCount() {
return ecf_.size();
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @param index The index of the element to return.
* @return The ecf at the given index.
*/
public double getEcf(int index) {
return ecf_.getDouble(index);
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @param index The index to set the value at.
* @param value The ecf to set.
* @return This builder for chaining.
*/
public Builder setEcf(
int index, double value) {
ensureEcfIsMutable();
ecf_.setDouble(index, value);
onChanged();
return this;
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @param value The ecf to add.
* @return This builder for chaining.
*/
public Builder addEcf(double value) {
ensureEcfIsMutable();
ecf_.addDouble(value);
onChanged();
return this;
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @param values The ecf to add.
* @return This builder for chaining.
*/
public Builder addAllEcf(
java.lang.Iterable extends java.lang.Double> values) {
ensureEcfIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ecf_);
onChanged();
return this;
}
/**
*
* The electron conversion factor (camera gain), defined as
* # of electrons per pixel / # of counts per pixel
* The ecf can be different for different channels (which can
* happen when separate cameras are used for separate channels),
* therefore provide the ecf for each channel in the channel order
*
*
* repeated double ecf = 28;
* @return This builder for chaining.
*/
public Builder clearEcf() {
ecf_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00080000);
onChanged();
return this;
}
private com.google.protobuf.Internal.DoubleList qe_ = emptyDoubleList();
private void ensureQeIsMutable() {
if (!((bitField0_ & 0x00100000) != 0)) {
qe_ = mutableCopy(qe_);
bitField0_ |= 0x00100000;
}
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return A list containing the qe.
*/
public java.util.List
getQeList() {
return ((bitField0_ & 0x00100000) != 0) ?
java.util.Collections.unmodifiableList(qe_) : qe_;
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return The count of qe.
*/
public int getQeCount() {
return qe_.size();
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @param index The index of the element to return.
* @return The qe at the given index.
*/
public double getQe(int index) {
return qe_.getDouble(index);
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @param index The index to set the value at.
* @param value The qe to set.
* @return This builder for chaining.
*/
public Builder setQe(
int index, double value) {
ensureQeIsMutable();
qe_.setDouble(index, value);
onChanged();
return this;
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @param value The qe to add.
* @return This builder for chaining.
*/
public Builder addQe(double value) {
ensureQeIsMutable();
qe_.addDouble(value);
onChanged();
return this;
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @param values The qe to add.
* @return This builder for chaining.
*/
public Builder addAllQe(
java.lang.Iterable extends java.lang.Double> values) {
ensureQeIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, qe_);
onChanged();
return this;
}
/**
*
* The quantum efficiency can be used to calculate the number
* of photons that hit the sensor, rather than the number of
* electrons that were derived from them
* Since this number is wavelength dependent, provide the QE
* for each fluorophore type (in the fluorophore type order)
* See the description of the field channel in the Spot message below
*
*
* repeated double qe = 30;
* @return This builder for chaining.
*/
public Builder clearQe() {
qe_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
private uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI roi_;
private com.google.protobuf.SingleFieldBuilderV3<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder> roiBuilder_;
/**
* optional .TSF.ROI roi = 29;
* @return Whether the roi field is set.
*/
public boolean hasRoi() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
* optional .TSF.ROI roi = 29;
* @return The roi.
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI getRoi() {
if (roiBuilder_ == null) {
return roi_ == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance() : roi_;
} else {
return roiBuilder_.getMessage();
}
}
/**
* optional .TSF.ROI roi = 29;
*/
public Builder setRoi(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI value) {
if (roiBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
roi_ = value;
} else {
roiBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
* optional .TSF.ROI roi = 29;
*/
public Builder setRoi(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder builderForValue) {
if (roiBuilder_ == null) {
roi_ = builderForValue.build();
} else {
roiBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
* optional .TSF.ROI roi = 29;
*/
public Builder mergeRoi(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI value) {
if (roiBuilder_ == null) {
if (((bitField0_ & 0x00200000) != 0) &&
roi_ != null &&
roi_ != uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance()) {
getRoiBuilder().mergeFrom(value);
} else {
roi_ = value;
}
} else {
roiBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
* optional .TSF.ROI roi = 29;
*/
public Builder clearRoi() {
bitField0_ = (bitField0_ & ~0x00200000);
roi_ = null;
if (roiBuilder_ != null) {
roiBuilder_.dispose();
roiBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .TSF.ROI roi = 29;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder getRoiBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getRoiFieldBuilder().getBuilder();
}
/**
* optional .TSF.ROI roi = 29;
*/
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder getRoiOrBuilder() {
if (roiBuilder_ != null) {
return roiBuilder_.getMessageOrBuilder();
} else {
return roi_ == null ?
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.getDefaultInstance() : roi_;
}
}
/**
* optional .TSF.ROI roi = 29;
*/
private com.google.protobuf.SingleFieldBuilderV3<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder>
getRoiFieldBuilder() {
if (roiBuilder_ == null) {
roiBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROI.Builder, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.ROIOrBuilder>(
getRoi(),
getParentForChildren(),
isClean());
roi_ = null;
}
return roiBuilder_;
}
private java.lang.Object source_ = "";
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return Whether the source field is set.
*/
public boolean hasSource() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return The source.
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
source_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return The bytes for source.
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @param value The source to set.
* @return This builder for chaining.
*/
public Builder setSource(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
source_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @return This builder for chaining.
*/
public Builder clearSource() {
source_ = getDefaultInstance().getSource();
bitField0_ = (bitField0_ & ~0x00400000);
onChanged();
return this;
}
/**
*
* Source of the results (can be a serialised object)
*
*
* optional string source = 1501;
* @param value The bytes for source to set.
* @return This builder for chaining.
*/
public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
source_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
private java.lang.Object configuration_ = "";
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return Whether the configuration field is set.
*/
public boolean hasConfiguration() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return The configuration.
*/
public java.lang.String getConfiguration() {
java.lang.Object ref = configuration_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
configuration_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return The bytes for configuration.
*/
public com.google.protobuf.ByteString
getConfigurationBytes() {
java.lang.Object ref = configuration_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configuration_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @param value The configuration to set.
* @return This builder for chaining.
*/
public Builder setConfiguration(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
configuration_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @return This builder for chaining.
*/
public Builder clearConfiguration() {
configuration_ = getDefaultInstance().getConfiguration();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
return this;
}
/**
*
* Configuration used for fitting (can be a serialised object)
*
*
* optional string configuration = 1502;
* @param value The bytes for configuration to set.
* @return This builder for chaining.
*/
public Builder setConfigurationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
configuration_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
private double gain_ ;
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return Whether the gain field is set.
*/
@java.lang.Override
public boolean hasGain() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return The gain.
*/
@java.lang.Override
public double getGain() {
return gain_;
}
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @param value The gain to set.
* @return This builder for chaining.
*/
public Builder setGain(double value) {
gain_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* The system gain to convert counts to photons (units=count/photon)
*
*
* optional double gain = 1503;
* @return This builder for chaining.
*/
public Builder clearGain() {
bitField0_ = (bitField0_ & ~0x01000000);
gain_ = 0D;
onChanged();
return this;
}
private double exposureTime_ ;
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return Whether the exposureTime field is set.
*/
@java.lang.Override
public boolean hasExposureTime() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return The exposureTime.
*/
@java.lang.Override
public double getExposureTime() {
return exposureTime_;
}
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @param value The exposureTime to set.
* @return This builder for chaining.
*/
public Builder setExposureTime(double value) {
exposureTime_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* The exposure time for a single frame (units=millisecond)
*
*
* optional double exposure_time = 1504;
* @return This builder for chaining.
*/
public Builder clearExposureTime() {
bitField0_ = (bitField0_ & ~0x02000000);
exposureTime_ = 0D;
onChanged();
return this;
}
private double readNoise_ ;
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return Whether the readNoise field is set.
*/
@java.lang.Override
public boolean hasReadNoise() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return The readNoise.
*/
@java.lang.Override
public double getReadNoise() {
return readNoise_;
}
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @param value The readNoise to set.
* @return This builder for chaining.
*/
public Builder setReadNoise(double value) {
readNoise_ = value;
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
* The camera read noise for a pixel (units=count)
*
*
* optional double read_noise = 1505;
* @return This builder for chaining.
*/
public Builder clearReadNoise() {
bitField0_ = (bitField0_ & ~0x04000000);
readNoise_ = 0D;
onChanged();
return this;
}
private double bias_ ;
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return Whether the bias field is set.
*/
@java.lang.Override
public boolean hasBias() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return The bias.
*/
@java.lang.Override
public double getBias() {
return bias_;
}
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @param value The bias to set.
* @return This builder for chaining.
*/
public Builder setBias(double value) {
bias_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
* The camera bias (units=counts)
*
*
* optional double bias = 1506;
* @return This builder for chaining.
*/
public Builder clearBias() {
bitField0_ = (bitField0_ & ~0x08000000);
bias_ = 0D;
onChanged();
return this;
}
private int cameraType_ = 0;
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return Whether the cameraType field is set.
*/
@java.lang.Override public boolean hasCameraType() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return The cameraType.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType getCameraType() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType.forNumber(cameraType_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType.CCD : result;
}
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @param value The cameraType to set.
* @return This builder for chaining.
*/
public Builder setCameraType(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.CameraType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x10000000;
cameraType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The camera type
*
*
* optional .TSF.CameraType camera_type = 1509;
* @return This builder for chaining.
*/
public Builder clearCameraType() {
bitField0_ = (bitField0_ & ~0x10000000);
cameraType_ = 0;
onChanged();
return this;
}
private java.lang.Object pSF_ = "";
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return Whether the pSF field is set.
*/
public boolean hasPSF() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return The pSF.
*/
public java.lang.String getPSF() {
java.lang.Object ref = pSF_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pSF_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return The bytes for pSF.
*/
public com.google.protobuf.ByteString
getPSFBytes() {
java.lang.Object ref = pSF_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pSF_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @param value The pSF to set.
* @return This builder for chaining.
*/
public Builder setPSF(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
pSF_ = value;
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @return This builder for chaining.
*/
public Builder clearPSF() {
pSF_ = getDefaultInstance().getPSF();
bitField0_ = (bitField0_ & ~0x20000000);
onChanged();
return this;
}
/**
*
* PSF used for fitting (can be a serialised object)
*
*
* optional string PSF = 1510;
* @param value The bytes for pSF to set.
* @return This builder for chaining.
*/
public Builder setPSFBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
pSF_ = value;
bitField0_ |= 0x20000000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:TSF.SpotList)
}
// @@protoc_insertion_point(class_scope:TSF.SpotList)
private static final uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList();
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SpotList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SpotOrBuilder extends
// @@protoc_insertion_point(interface_extends:TSF.Spot)
com.google.protobuf.GeneratedMessageV3.
ExtendableMessageOrBuilder {
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return Whether the molecule field is set.
*/
boolean hasMolecule();
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return The molecule.
*/
int getMolecule();
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return Whether the channel field is set.
*/
boolean hasChannel();
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return The channel.
*/
int getChannel();
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return Whether the frame field is set.
*/
boolean hasFrame();
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return The frame.
*/
int getFrame();
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return Whether the slice field is set.
*/
boolean hasSlice();
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return The slice.
*/
int getSlice();
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return Whether the pos field is set.
*/
boolean hasPos();
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return The pos.
*/
int getPos();
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return Whether the fluorophoreType field is set.
*/
boolean hasFluorophoreType();
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return The fluorophoreType.
*/
int getFluorophoreType();
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return Whether the cluster field is set.
*/
boolean hasCluster();
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return The cluster.
*/
int getCluster();
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return Whether the locationUnits field is set.
*/
boolean hasLocationUnits();
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return The locationUnits.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits getLocationUnits();
/**
* required float x = 7;
* @return Whether the x field is set.
*/
boolean hasX();
/**
* required float x = 7;
* @return The x.
*/
float getX();
/**
* required float y = 8;
* @return Whether the y field is set.
*/
boolean hasY();
/**
* required float y = 8;
* @return The y.
*/
float getY();
/**
* optional float z = 9;
* @return Whether the z field is set.
*/
boolean hasZ();
/**
* optional float z = 9;
* @return The z.
*/
float getZ();
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return Whether the intensityUnits field is set.
*/
boolean hasIntensityUnits();
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return The intensityUnits.
*/
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits getIntensityUnits();
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return Whether the intensity field is set.
*/
boolean hasIntensity();
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return The intensity.
*/
float getIntensity();
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return Whether the background field is set.
*/
boolean hasBackground();
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return The background.
*/
float getBackground();
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return Whether the width field is set.
*/
boolean hasWidth();
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return The width.
*/
float getWidth();
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return Whether the a field is set.
*/
boolean hasA();
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return The a.
*/
float getA();
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return Whether the theta field is set.
*/
boolean hasTheta();
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return The theta.
*/
float getTheta();
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return Whether the xOriginal field is set.
*/
boolean hasXOriginal();
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return The xOriginal.
*/
float getXOriginal();
/**
* optional float y_original = 102;
* @return Whether the yOriginal field is set.
*/
boolean hasYOriginal();
/**
* optional float y_original = 102;
* @return The yOriginal.
*/
float getYOriginal();
/**
* optional float z_original = 103;
* @return Whether the zOriginal field is set.
*/
boolean hasZOriginal();
/**
* optional float z_original = 103;
* @return The zOriginal.
*/
float getZOriginal();
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return Whether the xPrecision field is set.
*/
boolean hasXPrecision();
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return The xPrecision.
*/
float getXPrecision();
/**
* optional float y_precision = 105;
* @return Whether the yPrecision field is set.
*/
boolean hasYPrecision();
/**
* optional float y_precision = 105;
* @return The yPrecision.
*/
float getYPrecision();
/**
* optional float z_precision = 106;
* @return Whether the zPrecision field is set.
*/
boolean hasZPrecision();
/**
* optional float z_precision = 106;
* @return The zPrecision.
*/
float getZPrecision();
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return Whether the xPosition field is set.
*/
boolean hasXPosition();
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return The xPosition.
*/
int getXPosition();
/**
* optional int32 y_position = 108;
* @return Whether the yPosition field is set.
*/
boolean hasYPosition();
/**
* optional int32 y_position = 108;
* @return The yPosition.
*/
int getYPosition();
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return Whether the error field is set.
*/
boolean hasError();
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return The error.
*/
double getError();
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return Whether the noise field is set.
*/
boolean hasNoise();
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return The noise.
*/
float getNoise();
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return Whether the endFrame field is set.
*/
boolean hasEndFrame();
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return The endFrame.
*/
int getEndFrame();
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return Whether the originalValue field is set.
*/
boolean hasOriginalValue();
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return The originalValue.
*/
float getOriginalValue();
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return A list containing the paramStdDevs.
*/
java.util.List getParamStdDevsList();
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return The count of paramStdDevs.
*/
int getParamStdDevsCount();
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @param index The index of the element to return.
* @return The paramStdDevs at the given index.
*/
float getParamStdDevs(int index);
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return Whether the meanIntensity field is set.
*/
boolean hasMeanIntensity();
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return The meanIntensity.
*/
float getMeanIntensity();
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return Whether the category field is set.
*/
boolean hasCategory();
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return The category.
*/
int getCategory();
}
/**
* Protobuf type {@code TSF.Spot}
*/
public static final class Spot extends
com.google.protobuf.GeneratedMessageV3.ExtendableMessage<
Spot> implements
// @@protoc_insertion_point(message_implements:TSF.Spot)
SpotOrBuilder {
private static final long serialVersionUID = 0L;
// Use Spot.newBuilder() to construct.
private Spot(com.google.protobuf.GeneratedMessageV3.ExtendableBuilder builder) {
super(builder);
}
private Spot() {
locationUnits_ = 0;
intensityUnits_ = 0;
paramStdDevs_ = emptyFloatList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Spot();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_Spot_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_Spot_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.Builder.class);
}
private int bitField0_;
public static final int MOLECULE_FIELD_NUMBER = 1;
private int molecule_ = 0;
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return Whether the molecule field is set.
*/
@java.lang.Override
public boolean hasMolecule() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return The molecule.
*/
@java.lang.Override
public int getMolecule() {
return molecule_;
}
public static final int CHANNEL_FIELD_NUMBER = 2;
private int channel_ = 0;
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return Whether the channel field is set.
*/
@java.lang.Override
public boolean hasChannel() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return The channel.
*/
@java.lang.Override
public int getChannel() {
return channel_;
}
public static final int FRAME_FIELD_NUMBER = 3;
private int frame_ = 0;
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return Whether the frame field is set.
*/
@java.lang.Override
public boolean hasFrame() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return The frame.
*/
@java.lang.Override
public int getFrame() {
return frame_;
}
public static final int SLICE_FIELD_NUMBER = 4;
private int slice_ = 0;
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return Whether the slice field is set.
*/
@java.lang.Override
public boolean hasSlice() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return The slice.
*/
@java.lang.Override
public int getSlice() {
return slice_;
}
public static final int POS_FIELD_NUMBER = 5;
private int pos_ = 0;
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return Whether the pos field is set.
*/
@java.lang.Override
public boolean hasPos() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return The pos.
*/
@java.lang.Override
public int getPos() {
return pos_;
}
public static final int FLUOROPHORE_TYPE_FIELD_NUMBER = 19;
private int fluorophoreType_ = 0;
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return Whether the fluorophoreType field is set.
*/
@java.lang.Override
public boolean hasFluorophoreType() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return The fluorophoreType.
*/
@java.lang.Override
public int getFluorophoreType() {
return fluorophoreType_;
}
public static final int CLUSTER_FIELD_NUMBER = 20;
private int cluster_ = 0;
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return Whether the cluster field is set.
*/
@java.lang.Override
public boolean hasCluster() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return The cluster.
*/
@java.lang.Override
public int getCluster() {
return cluster_;
}
public static final int LOCATION_UNITS_FIELD_NUMBER = 17;
private int locationUnits_ = 0;
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return Whether the locationUnits field is set.
*/
@java.lang.Override public boolean hasLocationUnits() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return The locationUnits.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits getLocationUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.forNumber(locationUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.NM : result;
}
public static final int X_FIELD_NUMBER = 7;
private float x_ = 0F;
/**
* required float x = 7;
* @return Whether the x field is set.
*/
@java.lang.Override
public boolean hasX() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* required float x = 7;
* @return The x.
*/
@java.lang.Override
public float getX() {
return x_;
}
public static final int Y_FIELD_NUMBER = 8;
private float y_ = 0F;
/**
* required float y = 8;
* @return Whether the y field is set.
*/
@java.lang.Override
public boolean hasY() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* required float y = 8;
* @return The y.
*/
@java.lang.Override
public float getY() {
return y_;
}
public static final int Z_FIELD_NUMBER = 9;
private float z_ = 0F;
/**
* optional float z = 9;
* @return Whether the z field is set.
*/
@java.lang.Override
public boolean hasZ() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional float z = 9;
* @return The z.
*/
@java.lang.Override
public float getZ() {
return z_;
}
public static final int INTENSITY_UNITS_FIELD_NUMBER = 18;
private int intensityUnits_ = 0;
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return Whether the intensityUnits field is set.
*/
@java.lang.Override public boolean hasIntensityUnits() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return The intensityUnits.
*/
@java.lang.Override public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits getIntensityUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.forNumber(intensityUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.COUNTS : result;
}
public static final int INTENSITY_FIELD_NUMBER = 10;
private float intensity_ = 0F;
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return Whether the intensity field is set.
*/
@java.lang.Override
public boolean hasIntensity() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return The intensity.
*/
@java.lang.Override
public float getIntensity() {
return intensity_;
}
public static final int BACKGROUND_FIELD_NUMBER = 11;
private float background_ = 0F;
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return Whether the background field is set.
*/
@java.lang.Override
public boolean hasBackground() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return The background.
*/
@java.lang.Override
public float getBackground() {
return background_;
}
public static final int WIDTH_FIELD_NUMBER = 12;
private float width_ = 0F;
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return Whether the width field is set.
*/
@java.lang.Override
public boolean hasWidth() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return The width.
*/
@java.lang.Override
public float getWidth() {
return width_;
}
public static final int A_FIELD_NUMBER = 13;
private float a_ = 0F;
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return Whether the a field is set.
*/
@java.lang.Override
public boolean hasA() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return The a.
*/
@java.lang.Override
public float getA() {
return a_;
}
public static final int THETA_FIELD_NUMBER = 14;
private float theta_ = 0F;
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return Whether the theta field is set.
*/
@java.lang.Override
public boolean hasTheta() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return The theta.
*/
@java.lang.Override
public float getTheta() {
return theta_;
}
public static final int X_ORIGINAL_FIELD_NUMBER = 101;
private float xOriginal_ = 0F;
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return Whether the xOriginal field is set.
*/
@java.lang.Override
public boolean hasXOriginal() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return The xOriginal.
*/
@java.lang.Override
public float getXOriginal() {
return xOriginal_;
}
public static final int Y_ORIGINAL_FIELD_NUMBER = 102;
private float yOriginal_ = 0F;
/**
* optional float y_original = 102;
* @return Whether the yOriginal field is set.
*/
@java.lang.Override
public boolean hasYOriginal() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
* optional float y_original = 102;
* @return The yOriginal.
*/
@java.lang.Override
public float getYOriginal() {
return yOriginal_;
}
public static final int Z_ORIGINAL_FIELD_NUMBER = 103;
private float zOriginal_ = 0F;
/**
* optional float z_original = 103;
* @return Whether the zOriginal field is set.
*/
@java.lang.Override
public boolean hasZOriginal() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
* optional float z_original = 103;
* @return The zOriginal.
*/
@java.lang.Override
public float getZOriginal() {
return zOriginal_;
}
public static final int X_PRECISION_FIELD_NUMBER = 104;
private float xPrecision_ = 0F;
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return Whether the xPrecision field is set.
*/
@java.lang.Override
public boolean hasXPrecision() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return The xPrecision.
*/
@java.lang.Override
public float getXPrecision() {
return xPrecision_;
}
public static final int Y_PRECISION_FIELD_NUMBER = 105;
private float yPrecision_ = 0F;
/**
* optional float y_precision = 105;
* @return Whether the yPrecision field is set.
*/
@java.lang.Override
public boolean hasYPrecision() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
* optional float y_precision = 105;
* @return The yPrecision.
*/
@java.lang.Override
public float getYPrecision() {
return yPrecision_;
}
public static final int Z_PRECISION_FIELD_NUMBER = 106;
private float zPrecision_ = 0F;
/**
* optional float z_precision = 106;
* @return Whether the zPrecision field is set.
*/
@java.lang.Override
public boolean hasZPrecision() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
* optional float z_precision = 106;
* @return The zPrecision.
*/
@java.lang.Override
public float getZPrecision() {
return zPrecision_;
}
public static final int X_POSITION_FIELD_NUMBER = 107;
private int xPosition_ = 0;
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return Whether the xPosition field is set.
*/
@java.lang.Override
public boolean hasXPosition() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return The xPosition.
*/
@java.lang.Override
public int getXPosition() {
return xPosition_;
}
public static final int Y_POSITION_FIELD_NUMBER = 108;
private int yPosition_ = 0;
/**
* optional int32 y_position = 108;
* @return Whether the yPosition field is set.
*/
@java.lang.Override
public boolean hasYPosition() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
* optional int32 y_position = 108;
* @return The yPosition.
*/
@java.lang.Override
public int getYPosition() {
return yPosition_;
}
public static final int ERROR_FIELD_NUMBER = 1500;
private double error_ = 0D;
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return Whether the error field is set.
*/
@java.lang.Override
public boolean hasError() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return The error.
*/
@java.lang.Override
public double getError() {
return error_;
}
public static final int NOISE_FIELD_NUMBER = 1501;
private float noise_ = 0F;
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return Whether the noise field is set.
*/
@java.lang.Override
public boolean hasNoise() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return The noise.
*/
@java.lang.Override
public float getNoise() {
return noise_;
}
public static final int END_FRAME_FIELD_NUMBER = 1503;
private int endFrame_ = 0;
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return Whether the endFrame field is set.
*/
@java.lang.Override
public boolean hasEndFrame() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return The endFrame.
*/
@java.lang.Override
public int getEndFrame() {
return endFrame_;
}
public static final int ORIGINAL_VALUE_FIELD_NUMBER = 1504;
private float originalValue_ = 0F;
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return Whether the originalValue field is set.
*/
@java.lang.Override
public boolean hasOriginalValue() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return The originalValue.
*/
@java.lang.Override
public float getOriginalValue() {
return originalValue_;
}
public static final int PARAM_STD_DEVS_FIELD_NUMBER = 1505;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.FloatList paramStdDevs_;
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return A list containing the paramStdDevs.
*/
@java.lang.Override
public java.util.List
getParamStdDevsList() {
return paramStdDevs_;
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return The count of paramStdDevs.
*/
public int getParamStdDevsCount() {
return paramStdDevs_.size();
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @param index The index of the element to return.
* @return The paramStdDevs at the given index.
*/
public float getParamStdDevs(int index) {
return paramStdDevs_.getFloat(index);
}
public static final int MEAN_INTENSITY_FIELD_NUMBER = 1506;
private float meanIntensity_ = 0F;
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return Whether the meanIntensity field is set.
*/
@java.lang.Override
public boolean hasMeanIntensity() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return The meanIntensity.
*/
@java.lang.Override
public float getMeanIntensity() {
return meanIntensity_;
}
public static final int CATEGORY_FIELD_NUMBER = 1507;
private int category_ = 0;
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return Whether the category field is set.
*/
@java.lang.Override
public boolean hasCategory() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return The category.
*/
@java.lang.Override
public int getCategory() {
return category_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasMolecule()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasChannel()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasFrame()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasX()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasY()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasIntensity()) {
memoizedIsInitialized = 0;
return false;
}
if (!extensionsAreInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.ExtendableMessage.ExtensionWriter
extensionWriter = newExtensionWriter();
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, molecule_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, channel_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, frame_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, slice_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(5, pos_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeFloat(7, x_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeFloat(8, y_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeFloat(9, z_);
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeFloat(10, intensity_);
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeFloat(11, background_);
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeFloat(12, width_);
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeFloat(13, a_);
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeFloat(14, theta_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeEnum(17, locationUnits_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeEnum(18, intensityUnits_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt32(19, fluorophoreType_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(20, cluster_);
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeFloat(101, xOriginal_);
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeFloat(102, yOriginal_);
}
if (((bitField0_ & 0x00080000) != 0)) {
output.writeFloat(103, zOriginal_);
}
if (((bitField0_ & 0x00100000) != 0)) {
output.writeFloat(104, xPrecision_);
}
if (((bitField0_ & 0x00200000) != 0)) {
output.writeFloat(105, yPrecision_);
}
if (((bitField0_ & 0x00400000) != 0)) {
output.writeFloat(106, zPrecision_);
}
if (((bitField0_ & 0x00800000) != 0)) {
output.writeInt32(107, xPosition_);
}
if (((bitField0_ & 0x01000000) != 0)) {
output.writeInt32(108, yPosition_);
}
if (((bitField0_ & 0x02000000) != 0)) {
output.writeDouble(1500, error_);
}
if (((bitField0_ & 0x04000000) != 0)) {
output.writeFloat(1501, noise_);
}
if (((bitField0_ & 0x08000000) != 0)) {
output.writeInt32(1503, endFrame_);
}
if (((bitField0_ & 0x10000000) != 0)) {
output.writeFloat(1504, originalValue_);
}
for (int i = 0; i < paramStdDevs_.size(); i++) {
output.writeFloat(1505, paramStdDevs_.getFloat(i));
}
if (((bitField0_ & 0x20000000) != 0)) {
output.writeFloat(1506, meanIntensity_);
}
if (((bitField0_ & 0x40000000) != 0)) {
output.writeInt32(1507, category_);
}
extensionWriter.writeUntil(2048, output);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, molecule_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, channel_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, frame_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, slice_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, pos_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(7, x_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(8, y_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(9, z_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(10, intensity_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(11, background_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(12, width_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(13, a_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(14, theta_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(17, locationUnits_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(18, intensityUnits_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(19, fluorophoreType_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(20, cluster_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(101, xOriginal_);
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(102, yOriginal_);
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(103, zOriginal_);
}
if (((bitField0_ & 0x00100000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(104, xPrecision_);
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(105, yPrecision_);
}
if (((bitField0_ & 0x00400000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(106, zPrecision_);
}
if (((bitField0_ & 0x00800000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(107, xPosition_);
}
if (((bitField0_ & 0x01000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(108, yPosition_);
}
if (((bitField0_ & 0x02000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1500, error_);
}
if (((bitField0_ & 0x04000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(1501, noise_);
}
if (((bitField0_ & 0x08000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1503, endFrame_);
}
if (((bitField0_ & 0x10000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(1504, originalValue_);
}
{
int dataSize = 0;
dataSize = 4 * getParamStdDevsList().size();
size += dataSize;
size += 2 * getParamStdDevsList().size();
}
if (((bitField0_ & 0x20000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(1506, meanIntensity_);
}
if (((bitField0_ & 0x40000000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1507, category_);
}
size += extensionsSerializedSize();
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot)) {
return super.equals(obj);
}
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot other = (uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot) obj;
if (hasMolecule() != other.hasMolecule()) return false;
if (hasMolecule()) {
if (getMolecule()
!= other.getMolecule()) return false;
}
if (hasChannel() != other.hasChannel()) return false;
if (hasChannel()) {
if (getChannel()
!= other.getChannel()) return false;
}
if (hasFrame() != other.hasFrame()) return false;
if (hasFrame()) {
if (getFrame()
!= other.getFrame()) return false;
}
if (hasSlice() != other.hasSlice()) return false;
if (hasSlice()) {
if (getSlice()
!= other.getSlice()) return false;
}
if (hasPos() != other.hasPos()) return false;
if (hasPos()) {
if (getPos()
!= other.getPos()) return false;
}
if (hasFluorophoreType() != other.hasFluorophoreType()) return false;
if (hasFluorophoreType()) {
if (getFluorophoreType()
!= other.getFluorophoreType()) return false;
}
if (hasCluster() != other.hasCluster()) return false;
if (hasCluster()) {
if (getCluster()
!= other.getCluster()) return false;
}
if (hasLocationUnits() != other.hasLocationUnits()) return false;
if (hasLocationUnits()) {
if (locationUnits_ != other.locationUnits_) return false;
}
if (hasX() != other.hasX()) return false;
if (hasX()) {
if (java.lang.Float.floatToIntBits(getX())
!= java.lang.Float.floatToIntBits(
other.getX())) return false;
}
if (hasY() != other.hasY()) return false;
if (hasY()) {
if (java.lang.Float.floatToIntBits(getY())
!= java.lang.Float.floatToIntBits(
other.getY())) return false;
}
if (hasZ() != other.hasZ()) return false;
if (hasZ()) {
if (java.lang.Float.floatToIntBits(getZ())
!= java.lang.Float.floatToIntBits(
other.getZ())) return false;
}
if (hasIntensityUnits() != other.hasIntensityUnits()) return false;
if (hasIntensityUnits()) {
if (intensityUnits_ != other.intensityUnits_) return false;
}
if (hasIntensity() != other.hasIntensity()) return false;
if (hasIntensity()) {
if (java.lang.Float.floatToIntBits(getIntensity())
!= java.lang.Float.floatToIntBits(
other.getIntensity())) return false;
}
if (hasBackground() != other.hasBackground()) return false;
if (hasBackground()) {
if (java.lang.Float.floatToIntBits(getBackground())
!= java.lang.Float.floatToIntBits(
other.getBackground())) return false;
}
if (hasWidth() != other.hasWidth()) return false;
if (hasWidth()) {
if (java.lang.Float.floatToIntBits(getWidth())
!= java.lang.Float.floatToIntBits(
other.getWidth())) return false;
}
if (hasA() != other.hasA()) return false;
if (hasA()) {
if (java.lang.Float.floatToIntBits(getA())
!= java.lang.Float.floatToIntBits(
other.getA())) return false;
}
if (hasTheta() != other.hasTheta()) return false;
if (hasTheta()) {
if (java.lang.Float.floatToIntBits(getTheta())
!= java.lang.Float.floatToIntBits(
other.getTheta())) return false;
}
if (hasXOriginal() != other.hasXOriginal()) return false;
if (hasXOriginal()) {
if (java.lang.Float.floatToIntBits(getXOriginal())
!= java.lang.Float.floatToIntBits(
other.getXOriginal())) return false;
}
if (hasYOriginal() != other.hasYOriginal()) return false;
if (hasYOriginal()) {
if (java.lang.Float.floatToIntBits(getYOriginal())
!= java.lang.Float.floatToIntBits(
other.getYOriginal())) return false;
}
if (hasZOriginal() != other.hasZOriginal()) return false;
if (hasZOriginal()) {
if (java.lang.Float.floatToIntBits(getZOriginal())
!= java.lang.Float.floatToIntBits(
other.getZOriginal())) return false;
}
if (hasXPrecision() != other.hasXPrecision()) return false;
if (hasXPrecision()) {
if (java.lang.Float.floatToIntBits(getXPrecision())
!= java.lang.Float.floatToIntBits(
other.getXPrecision())) return false;
}
if (hasYPrecision() != other.hasYPrecision()) return false;
if (hasYPrecision()) {
if (java.lang.Float.floatToIntBits(getYPrecision())
!= java.lang.Float.floatToIntBits(
other.getYPrecision())) return false;
}
if (hasZPrecision() != other.hasZPrecision()) return false;
if (hasZPrecision()) {
if (java.lang.Float.floatToIntBits(getZPrecision())
!= java.lang.Float.floatToIntBits(
other.getZPrecision())) return false;
}
if (hasXPosition() != other.hasXPosition()) return false;
if (hasXPosition()) {
if (getXPosition()
!= other.getXPosition()) return false;
}
if (hasYPosition() != other.hasYPosition()) return false;
if (hasYPosition()) {
if (getYPosition()
!= other.getYPosition()) return false;
}
if (hasError() != other.hasError()) return false;
if (hasError()) {
if (java.lang.Double.doubleToLongBits(getError())
!= java.lang.Double.doubleToLongBits(
other.getError())) return false;
}
if (hasNoise() != other.hasNoise()) return false;
if (hasNoise()) {
if (java.lang.Float.floatToIntBits(getNoise())
!= java.lang.Float.floatToIntBits(
other.getNoise())) return false;
}
if (hasEndFrame() != other.hasEndFrame()) return false;
if (hasEndFrame()) {
if (getEndFrame()
!= other.getEndFrame()) return false;
}
if (hasOriginalValue() != other.hasOriginalValue()) return false;
if (hasOriginalValue()) {
if (java.lang.Float.floatToIntBits(getOriginalValue())
!= java.lang.Float.floatToIntBits(
other.getOriginalValue())) return false;
}
if (!getParamStdDevsList()
.equals(other.getParamStdDevsList())) return false;
if (hasMeanIntensity() != other.hasMeanIntensity()) return false;
if (hasMeanIntensity()) {
if (java.lang.Float.floatToIntBits(getMeanIntensity())
!= java.lang.Float.floatToIntBits(
other.getMeanIntensity())) return false;
}
if (hasCategory() != other.hasCategory()) return false;
if (hasCategory()) {
if (getCategory()
!= other.getCategory()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
if (!getExtensionFields().equals(other.getExtensionFields()))
return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMolecule()) {
hash = (37 * hash) + MOLECULE_FIELD_NUMBER;
hash = (53 * hash) + getMolecule();
}
if (hasChannel()) {
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getChannel();
}
if (hasFrame()) {
hash = (37 * hash) + FRAME_FIELD_NUMBER;
hash = (53 * hash) + getFrame();
}
if (hasSlice()) {
hash = (37 * hash) + SLICE_FIELD_NUMBER;
hash = (53 * hash) + getSlice();
}
if (hasPos()) {
hash = (37 * hash) + POS_FIELD_NUMBER;
hash = (53 * hash) + getPos();
}
if (hasFluorophoreType()) {
hash = (37 * hash) + FLUOROPHORE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getFluorophoreType();
}
if (hasCluster()) {
hash = (37 * hash) + CLUSTER_FIELD_NUMBER;
hash = (53 * hash) + getCluster();
}
if (hasLocationUnits()) {
hash = (37 * hash) + LOCATION_UNITS_FIELD_NUMBER;
hash = (53 * hash) + locationUnits_;
}
if (hasX()) {
hash = (37 * hash) + X_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getX());
}
if (hasY()) {
hash = (37 * hash) + Y_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getY());
}
if (hasZ()) {
hash = (37 * hash) + Z_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getZ());
}
if (hasIntensityUnits()) {
hash = (37 * hash) + INTENSITY_UNITS_FIELD_NUMBER;
hash = (53 * hash) + intensityUnits_;
}
if (hasIntensity()) {
hash = (37 * hash) + INTENSITY_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getIntensity());
}
if (hasBackground()) {
hash = (37 * hash) + BACKGROUND_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getBackground());
}
if (hasWidth()) {
hash = (37 * hash) + WIDTH_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getWidth());
}
if (hasA()) {
hash = (37 * hash) + A_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getA());
}
if (hasTheta()) {
hash = (37 * hash) + THETA_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getTheta());
}
if (hasXOriginal()) {
hash = (37 * hash) + X_ORIGINAL_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getXOriginal());
}
if (hasYOriginal()) {
hash = (37 * hash) + Y_ORIGINAL_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getYOriginal());
}
if (hasZOriginal()) {
hash = (37 * hash) + Z_ORIGINAL_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getZOriginal());
}
if (hasXPrecision()) {
hash = (37 * hash) + X_PRECISION_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getXPrecision());
}
if (hasYPrecision()) {
hash = (37 * hash) + Y_PRECISION_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getYPrecision());
}
if (hasZPrecision()) {
hash = (37 * hash) + Z_PRECISION_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getZPrecision());
}
if (hasXPosition()) {
hash = (37 * hash) + X_POSITION_FIELD_NUMBER;
hash = (53 * hash) + getXPosition();
}
if (hasYPosition()) {
hash = (37 * hash) + Y_POSITION_FIELD_NUMBER;
hash = (53 * hash) + getYPosition();
}
if (hasError()) {
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getError()));
}
if (hasNoise()) {
hash = (37 * hash) + NOISE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getNoise());
}
if (hasEndFrame()) {
hash = (37 * hash) + END_FRAME_FIELD_NUMBER;
hash = (53 * hash) + getEndFrame();
}
if (hasOriginalValue()) {
hash = (37 * hash) + ORIGINAL_VALUE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getOriginalValue());
}
if (getParamStdDevsCount() > 0) {
hash = (37 * hash) + PARAM_STD_DEVS_FIELD_NUMBER;
hash = (53 * hash) + getParamStdDevsList().hashCode();
}
if (hasMeanIntensity()) {
hash = (37 * hash) + MEAN_INTENSITY_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getMeanIntensity());
}
if (hasCategory()) {
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getCategory();
}
hash = hashFields(hash, getExtensionFields());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TSF.Spot}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.ExtendableBuilder<
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot, Builder> implements
// @@protoc_insertion_point(builder_implements:TSF.Spot)
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.SpotOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_Spot_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_Spot_fieldAccessorTable
.ensureFieldAccessorsInitialized(
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.class, uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.Builder.class);
}
// Construct using uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
molecule_ = 0;
channel_ = 0;
frame_ = 0;
slice_ = 0;
pos_ = 0;
fluorophoreType_ = 0;
cluster_ = 0;
locationUnits_ = 0;
x_ = 0F;
y_ = 0F;
z_ = 0F;
intensityUnits_ = 0;
intensity_ = 0F;
background_ = 0F;
width_ = 0F;
a_ = 0F;
theta_ = 0F;
xOriginal_ = 0F;
yOriginal_ = 0F;
zOriginal_ = 0F;
xPrecision_ = 0F;
yPrecision_ = 0F;
zPrecision_ = 0F;
xPosition_ = 0;
yPosition_ = 0;
error_ = 0D;
noise_ = 0F;
endFrame_ = 0;
originalValue_ = 0F;
paramStdDevs_ = emptyFloatList();
meanIntensity_ = 0F;
category_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.internal_static_TSF_Spot_descriptor;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot getDefaultInstanceForType() {
return uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.getDefaultInstance();
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot build() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot buildPartial() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot result = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot result) {
if (((bitField0_ & 0x20000000) != 0)) {
paramStdDevs_.makeImmutable();
bitField0_ = (bitField0_ & ~0x20000000);
}
result.paramStdDevs_ = paramStdDevs_;
}
private void buildPartial0(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.molecule_ = molecule_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.channel_ = channel_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.frame_ = frame_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.slice_ = slice_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.pos_ = pos_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.fluorophoreType_ = fluorophoreType_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.cluster_ = cluster_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.locationUnits_ = locationUnits_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.x_ = x_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.y_ = y_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.z_ = z_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.intensityUnits_ = intensityUnits_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.intensity_ = intensity_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.background_ = background_;
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.width_ = width_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.a_ = a_;
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.theta_ = theta_;
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.xOriginal_ = xOriginal_;
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.yOriginal_ = yOriginal_;
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.zOriginal_ = zOriginal_;
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.xPrecision_ = xPrecision_;
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.yPrecision_ = yPrecision_;
to_bitField0_ |= 0x00200000;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.zPrecision_ = zPrecision_;
to_bitField0_ |= 0x00400000;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.xPosition_ = xPosition_;
to_bitField0_ |= 0x00800000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.yPosition_ = yPosition_;
to_bitField0_ |= 0x01000000;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.error_ = error_;
to_bitField0_ |= 0x02000000;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.noise_ = noise_;
to_bitField0_ |= 0x04000000;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.endFrame_ = endFrame_;
to_bitField0_ |= 0x08000000;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.originalValue_ = originalValue_;
to_bitField0_ |= 0x10000000;
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.meanIntensity_ = meanIntensity_;
to_bitField0_ |= 0x20000000;
}
if (((from_bitField0_ & 0x80000000) != 0)) {
result.category_ = category_;
to_bitField0_ |= 0x40000000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot) {
return mergeFrom((uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot other) {
if (other == uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot.getDefaultInstance()) return this;
if (other.hasMolecule()) {
setMolecule(other.getMolecule());
}
if (other.hasChannel()) {
setChannel(other.getChannel());
}
if (other.hasFrame()) {
setFrame(other.getFrame());
}
if (other.hasSlice()) {
setSlice(other.getSlice());
}
if (other.hasPos()) {
setPos(other.getPos());
}
if (other.hasFluorophoreType()) {
setFluorophoreType(other.getFluorophoreType());
}
if (other.hasCluster()) {
setCluster(other.getCluster());
}
if (other.hasLocationUnits()) {
setLocationUnits(other.getLocationUnits());
}
if (other.hasX()) {
setX(other.getX());
}
if (other.hasY()) {
setY(other.getY());
}
if (other.hasZ()) {
setZ(other.getZ());
}
if (other.hasIntensityUnits()) {
setIntensityUnits(other.getIntensityUnits());
}
if (other.hasIntensity()) {
setIntensity(other.getIntensity());
}
if (other.hasBackground()) {
setBackground(other.getBackground());
}
if (other.hasWidth()) {
setWidth(other.getWidth());
}
if (other.hasA()) {
setA(other.getA());
}
if (other.hasTheta()) {
setTheta(other.getTheta());
}
if (other.hasXOriginal()) {
setXOriginal(other.getXOriginal());
}
if (other.hasYOriginal()) {
setYOriginal(other.getYOriginal());
}
if (other.hasZOriginal()) {
setZOriginal(other.getZOriginal());
}
if (other.hasXPrecision()) {
setXPrecision(other.getXPrecision());
}
if (other.hasYPrecision()) {
setYPrecision(other.getYPrecision());
}
if (other.hasZPrecision()) {
setZPrecision(other.getZPrecision());
}
if (other.hasXPosition()) {
setXPosition(other.getXPosition());
}
if (other.hasYPosition()) {
setYPosition(other.getYPosition());
}
if (other.hasError()) {
setError(other.getError());
}
if (other.hasNoise()) {
setNoise(other.getNoise());
}
if (other.hasEndFrame()) {
setEndFrame(other.getEndFrame());
}
if (other.hasOriginalValue()) {
setOriginalValue(other.getOriginalValue());
}
if (!other.paramStdDevs_.isEmpty()) {
if (paramStdDevs_.isEmpty()) {
paramStdDevs_ = other.paramStdDevs_;
bitField0_ = (bitField0_ & ~0x20000000);
} else {
ensureParamStdDevsIsMutable();
paramStdDevs_.addAll(other.paramStdDevs_);
}
onChanged();
}
if (other.hasMeanIntensity()) {
setMeanIntensity(other.getMeanIntensity());
}
if (other.hasCategory()) {
setCategory(other.getCategory());
}
this.mergeExtensionFields(other);
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasMolecule()) {
return false;
}
if (!hasChannel()) {
return false;
}
if (!hasFrame()) {
return false;
}
if (!hasX()) {
return false;
}
if (!hasY()) {
return false;
}
if (!hasIntensity()) {
return false;
}
if (!extensionsAreInitialized()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
molecule_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
channel_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
frame_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
slice_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
pos_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
case 61: {
x_ = input.readFloat();
bitField0_ |= 0x00000100;
break;
} // case 61
case 69: {
y_ = input.readFloat();
bitField0_ |= 0x00000200;
break;
} // case 69
case 77: {
z_ = input.readFloat();
bitField0_ |= 0x00000400;
break;
} // case 77
case 85: {
intensity_ = input.readFloat();
bitField0_ |= 0x00001000;
break;
} // case 85
case 93: {
background_ = input.readFloat();
bitField0_ |= 0x00002000;
break;
} // case 93
case 101: {
width_ = input.readFloat();
bitField0_ |= 0x00004000;
break;
} // case 101
case 109: {
a_ = input.readFloat();
bitField0_ |= 0x00008000;
break;
} // case 109
case 117: {
theta_ = input.readFloat();
bitField0_ |= 0x00010000;
break;
} // case 117
case 136: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(17, tmpRaw);
} else {
locationUnits_ = tmpRaw;
bitField0_ |= 0x00000080;
}
break;
} // case 136
case 144: {
int tmpRaw = input.readEnum();
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits tmpValue =
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(18, tmpRaw);
} else {
intensityUnits_ = tmpRaw;
bitField0_ |= 0x00000800;
}
break;
} // case 144
case 152: {
fluorophoreType_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case 152
case 160: {
cluster_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case 160
case 813: {
xOriginal_ = input.readFloat();
bitField0_ |= 0x00020000;
break;
} // case 813
case 821: {
yOriginal_ = input.readFloat();
bitField0_ |= 0x00040000;
break;
} // case 821
case 829: {
zOriginal_ = input.readFloat();
bitField0_ |= 0x00080000;
break;
} // case 829
case 837: {
xPrecision_ = input.readFloat();
bitField0_ |= 0x00100000;
break;
} // case 837
case 845: {
yPrecision_ = input.readFloat();
bitField0_ |= 0x00200000;
break;
} // case 845
case 853: {
zPrecision_ = input.readFloat();
bitField0_ |= 0x00400000;
break;
} // case 853
case 856: {
xPosition_ = input.readInt32();
bitField0_ |= 0x00800000;
break;
} // case 856
case 864: {
yPosition_ = input.readInt32();
bitField0_ |= 0x01000000;
break;
} // case 864
case 12001: {
error_ = input.readDouble();
bitField0_ |= 0x02000000;
break;
} // case 12001
case 12013: {
noise_ = input.readFloat();
bitField0_ |= 0x04000000;
break;
} // case 12013
case 12024: {
endFrame_ = input.readInt32();
bitField0_ |= 0x08000000;
break;
} // case 12024
case 12037: {
originalValue_ = input.readFloat();
bitField0_ |= 0x10000000;
break;
} // case 12037
case 12045: {
float v = input.readFloat();
ensureParamStdDevsIsMutable();
paramStdDevs_.addFloat(v);
break;
} // case 12045
case 12042: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureParamStdDevsIsMutable();
while (input.getBytesUntilLimit() > 0) {
paramStdDevs_.addFloat(input.readFloat());
}
input.popLimit(limit);
break;
} // case 12042
case 12053: {
meanIntensity_ = input.readFloat();
bitField0_ |= 0x40000000;
break;
} // case 12053
case 12056: {
category_ = input.readInt32();
bitField0_ |= 0x80000000;
break;
} // case 12056
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int molecule_ ;
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return Whether the molecule field is set.
*/
@java.lang.Override
public boolean hasMolecule() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return The molecule.
*/
@java.lang.Override
public int getMolecule() {
return molecule_;
}
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @param value The molecule to set.
* @return This builder for chaining.
*/
public Builder setMolecule(int value) {
molecule_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Identifier for a physical molecule. Multiple localizations will be considered
* to be generated by the same molecule if they share the same molecule ID.
* Typically, a molecule generates up to one localization per frame.
* If you don't implement tracking, assign a different molecule ID to
* each localization.
* The difference between molecules and clusters is that a cluster is a group
* of physical molecules, e.g. a raft on a membrane. When you are tracking to
* link your localizations, you're looking at a molecule, and when you're
* thresholding on the nearest neighbor distance, it's typically a cluster.
* If you are using both cluster and molecule, localizations with the same
* molecule ID should have the same cluster ID.
* Molecule IDs must be globally unique across clusters.
*
*
* required int32 molecule = 1;
* @return This builder for chaining.
*/
public Builder clearMolecule() {
bitField0_ = (bitField0_ & ~0x00000001);
molecule_ = 0;
onChanged();
return this;
}
private int channel_ ;
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return Whether the channel field is set.
*/
@java.lang.Override
public boolean hasChannel() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return The channel.
*/
@java.lang.Override
public int getChannel() {
return channel_;
}
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @param value The channel to set.
* @return This builder for chaining.
*/
public Builder setChannel(int value) {
channel_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Identifier for an input channel. Input channels represent different optical
* paths, often with different spectral characteristics. For example, in a
* biplane setup, you'd have two channels, one for each camera. Channels are
* 1-based.
* Please note that channels and fluorophore type are subtly different: channel
* gives the *physical* excitation path (camera 1 or camera 2), while fluorophore
* type gives the *fluorophore's* type (Cy5, Cy3, Tetraspeck). For example, for
* biplane single-color 3D, you'd set the channel field if you emit separate
* localizations for both channels, but never the type field. For biplane
* two-color experiments, you'd set both channel and type if you fit in both
* channels independently, and only types if your fitter combines information
* for both channels. For spectrally separated measurements, channel and type
* are usually identical, but for spectral unmixing, they may differ.
*
*
* required int32 channel = 2;
* @return This builder for chaining.
*/
public Builder clearChannel() {
bitField0_ = (bitField0_ & ~0x00000002);
channel_ = 0;
onChanged();
return this;
}
private int frame_ ;
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return Whether the frame field is set.
*/
@java.lang.Override
public boolean hasFrame() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return The frame.
*/
@java.lang.Override
public int getFrame() {
return frame_;
}
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @param value The frame to set.
* @return This builder for chaining.
*/
public Builder setFrame(int value) {
frame_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Frame number (image number). The frames of each channel are numbered
* sequentially, starting from 1.
*
*
* required int32 frame = 3;
* @return This builder for chaining.
*/
public Builder clearFrame() {
bitField0_ = (bitField0_ & ~0x00000004);
frame_ = 0;
onChanged();
return this;
}
private int slice_ ;
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return Whether the slice field is set.
*/
@java.lang.Override
public boolean hasSlice() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return The slice.
*/
@java.lang.Override
public int getSlice() {
return slice_;
}
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @param value The slice to set.
* @return This builder for chaining.
*/
public Builder setSlice(int value) {
slice_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Z slice. If you are taking a series of images at different axial offsets,
* you can tag the localizations from each respective slice with this 1-based
* field. Please apply the offset between slices before saving to a TSF file:
* z=100 with slice=1 is considered same position as z=100 with slice=2.
*
*
* optional int32 slice = 4;
* @return This builder for chaining.
*/
public Builder clearSlice() {
bitField0_ = (bitField0_ & ~0x00000008);
slice_ = 0;
onChanged();
return this;
}
private int pos_ ;
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return Whether the pos field is set.
*/
@java.lang.Override
public boolean hasPos() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return The pos.
*/
@java.lang.Override
public int getPos() {
return pos_;
}
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @param value The pos to set.
* @return This builder for chaining.
*/
public Builder setPos(int value) {
pos_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Lateral position. If you are taking images at multiple x/y stage positions,
* you can tag the localizations from each position with this 1-based field.
* Positions are 1-based. Please apply the offset between positions before
* saving the TSF file:
* x=100 with pos=1 is considered same position as x=100 with pos=2.
*
*
* optional int32 pos = 5;
* @return This builder for chaining.
*/
public Builder clearPos() {
bitField0_ = (bitField0_ & ~0x00000010);
pos_ = 0;
onChanged();
return this;
}
private int fluorophoreType_ ;
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return Whether the fluorophoreType field is set.
*/
@java.lang.Override
public boolean hasFluorophoreType() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return The fluorophoreType.
*/
@java.lang.Override
public int getFluorophoreType() {
return fluorophoreType_;
}
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @param value The fluorophoreType to set.
* @return This builder for chaining.
*/
public Builder setFluorophoreType(int value) {
fluorophoreType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Fluorophore type. The number is 1-based. Please compare the note for
* channel for the distinction between type and channel.
*
*
* optional int32 fluorophore_type = 19;
* @return This builder for chaining.
*/
public Builder clearFluorophoreType() {
bitField0_ = (bitField0_ & ~0x00000020);
fluorophoreType_ = 0;
onChanged();
return this;
}
private int cluster_ ;
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return Whether the cluster field is set.
*/
@java.lang.Override
public boolean hasCluster() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return The cluster.
*/
@java.lang.Override
public int getCluster() {
return cluster_;
}
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @param value The cluster to set.
* @return This builder for chaining.
*/
public Builder setCluster(int value) {
cluster_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The identifier of the cluster that the localization belongs to, 1-based.
* A cluster is a logical group of multiple physical molecules. Typical
* examples are membrane rafts,
*
*
* optional int32 cluster = 20;
* @return This builder for chaining.
*/
public Builder clearCluster() {
bitField0_ = (bitField0_ & ~0x00000040);
cluster_ = 0;
onChanged();
return this;
}
private int locationUnits_ = 0;
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return Whether the locationUnits field is set.
*/
@java.lang.Override public boolean hasLocationUnits() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return The locationUnits.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits getLocationUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.forNumber(locationUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits.NM : result;
}
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @param value The locationUnits to set.
* @return This builder for chaining.
*/
public Builder setLocationUnits(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.LocationUnits value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
locationUnits_ = value.getNumber();
onChanged();
return this;
}
/**
*
* xyz coordinates of the spot in location_units
* after fitting and optional correction
*
*
* optional .TSF.LocationUnits location_units = 17;
* @return This builder for chaining.
*/
public Builder clearLocationUnits() {
bitField0_ = (bitField0_ & ~0x00000080);
locationUnits_ = 0;
onChanged();
return this;
}
private float x_ ;
/**
* required float x = 7;
* @return Whether the x field is set.
*/
@java.lang.Override
public boolean hasX() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* required float x = 7;
* @return The x.
*/
@java.lang.Override
public float getX() {
return x_;
}
/**
* required float x = 7;
* @param value The x to set.
* @return This builder for chaining.
*/
public Builder setX(float value) {
x_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* required float x = 7;
* @return This builder for chaining.
*/
public Builder clearX() {
bitField0_ = (bitField0_ & ~0x00000100);
x_ = 0F;
onChanged();
return this;
}
private float y_ ;
/**
* required float y = 8;
* @return Whether the y field is set.
*/
@java.lang.Override
public boolean hasY() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* required float y = 8;
* @return The y.
*/
@java.lang.Override
public float getY() {
return y_;
}
/**
* required float y = 8;
* @param value The y to set.
* @return This builder for chaining.
*/
public Builder setY(float value) {
y_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* required float y = 8;
* @return This builder for chaining.
*/
public Builder clearY() {
bitField0_ = (bitField0_ & ~0x00000200);
y_ = 0F;
onChanged();
return this;
}
private float z_ ;
/**
* optional float z = 9;
* @return Whether the z field is set.
*/
@java.lang.Override
public boolean hasZ() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional float z = 9;
* @return The z.
*/
@java.lang.Override
public float getZ() {
return z_;
}
/**
* optional float z = 9;
* @param value The z to set.
* @return This builder for chaining.
*/
public Builder setZ(float value) {
z_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* optional float z = 9;
* @return This builder for chaining.
*/
public Builder clearZ() {
bitField0_ = (bitField0_ & ~0x00000400);
z_ = 0F;
onChanged();
return this;
}
private int intensityUnits_ = 0;
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return Whether the intensityUnits field is set.
*/
@java.lang.Override public boolean hasIntensityUnits() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return The intensityUnits.
*/
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits getIntensityUnits() {
uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits result = uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.forNumber(intensityUnits_);
return result == null ? uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits.COUNTS : result;
}
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @param value The intensityUnits to set.
* @return This builder for chaining.
*/
public Builder setIntensityUnits(uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.IntensityUnits value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
intensityUnits_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Use intensity_units only if different from SpotList
* integrated spot density. This can either be determined from a fit or
* using any other methods. This number should be corrected for background
*
*
* optional .TSF.IntensityUnits intensity_units = 18;
* @return This builder for chaining.
*/
public Builder clearIntensityUnits() {
bitField0_ = (bitField0_ & ~0x00000800);
intensityUnits_ = 0;
onChanged();
return this;
}
private float intensity_ ;
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return Whether the intensity field is set.
*/
@java.lang.Override
public boolean hasIntensity() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return The intensity.
*/
@java.lang.Override
public float getIntensity() {
return intensity_;
}
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @param value The intensity to set.
* @return This builder for chaining.
*/
public Builder setIntensity(float value) {
intensity_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* integrated spot density
*
*
* required float intensity = 10;
* @return This builder for chaining.
*/
public Builder clearIntensity() {
bitField0_ = (bitField0_ & ~0x00001000);
intensity_ = 0F;
onChanged();
return this;
}
private float background_ ;
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return Whether the background field is set.
*/
@java.lang.Override
public boolean hasBackground() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return The background.
*/
@java.lang.Override
public float getBackground() {
return background_;
}
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @param value The background to set.
* @return This builder for chaining.
*/
public Builder setBackground(float value) {
background_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Background around the spot.
* This can be determined through a fit or other methods
* This number should not include the camera bias, i.e. it should be linearly
* proportional to the number of photons in the background
*
*
* optional float background = 11;
* @return This builder for chaining.
*/
public Builder clearBackground() {
bitField0_ = (bitField0_ & ~0x00002000);
background_ = 0F;
onChanged();
return this;
}
private float width_ ;
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return Whether the width field is set.
*/
@java.lang.Override
public boolean hasWidth() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return The width.
*/
@java.lang.Override
public float getWidth() {
return width_;
}
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @param value The width to set.
* @return This builder for chaining.
*/
public Builder setWidth(float value) {
width_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* Peak width at half height in location units
* for asymmetric peaks, calculate the width as the square root of the
* product of the widths of the long and short axes
*
*
* optional float width = 12;
* @return This builder for chaining.
*/
public Builder clearWidth() {
bitField0_ = (bitField0_ & ~0x00004000);
width_ = 0F;
onChanged();
return this;
}
private float a_ ;
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return Whether the a field is set.
*/
@java.lang.Override
public boolean hasA() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return The a.
*/
@java.lang.Override
public float getA() {
return a_;
}
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @param value The a to set.
* @return This builder for chaining.
*/
public Builder setA(float value) {
a_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* Shape of the peak: width of the long axis
* divided by width of the short axis
*
*
* optional float a = 13;
* @return This builder for chaining.
*/
public Builder clearA() {
bitField0_ = (bitField0_ & ~0x00008000);
a_ = 0F;
onChanged();
return this;
}
private float theta_ ;
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return Whether the theta field is set.
*/
@java.lang.Override
public boolean hasTheta() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return The theta.
*/
@java.lang.Override
public float getTheta() {
return theta_;
}
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @param value The theta to set.
* @return This builder for chaining.
*/
public Builder setTheta(float value) {
theta_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* Rotation of asymmetric peak, only used
* when fitmode == TWOAXISANDTHETA
*
*
* optional float theta = 14;
* @return This builder for chaining.
*/
public Builder clearTheta() {
bitField0_ = (bitField0_ & ~0x00010000);
theta_ = 0F;
onChanged();
return this;
}
private float xOriginal_ ;
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return Whether the xOriginal field is set.
*/
@java.lang.Override
public boolean hasXOriginal() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return The xOriginal.
*/
@java.lang.Override
public float getXOriginal() {
return xOriginal_;
}
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @param value The xOriginal to set.
* @return This builder for chaining.
*/
public Builder setXOriginal(float value) {
xOriginal_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* Original xyz coordinates from fitting before correction
*
*
* optional float x_original = 101;
* @return This builder for chaining.
*/
public Builder clearXOriginal() {
bitField0_ = (bitField0_ & ~0x00020000);
xOriginal_ = 0F;
onChanged();
return this;
}
private float yOriginal_ ;
/**
* optional float y_original = 102;
* @return Whether the yOriginal field is set.
*/
@java.lang.Override
public boolean hasYOriginal() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
* optional float y_original = 102;
* @return The yOriginal.
*/
@java.lang.Override
public float getYOriginal() {
return yOriginal_;
}
/**
* optional float y_original = 102;
* @param value The yOriginal to set.
* @return This builder for chaining.
*/
public Builder setYOriginal(float value) {
yOriginal_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
* optional float y_original = 102;
* @return This builder for chaining.
*/
public Builder clearYOriginal() {
bitField0_ = (bitField0_ & ~0x00040000);
yOriginal_ = 0F;
onChanged();
return this;
}
private float zOriginal_ ;
/**
* optional float z_original = 103;
* @return Whether the zOriginal field is set.
*/
@java.lang.Override
public boolean hasZOriginal() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
* optional float z_original = 103;
* @return The zOriginal.
*/
@java.lang.Override
public float getZOriginal() {
return zOriginal_;
}
/**
* optional float z_original = 103;
* @param value The zOriginal to set.
* @return This builder for chaining.
*/
public Builder setZOriginal(float value) {
zOriginal_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
* optional float z_original = 103;
* @return This builder for chaining.
*/
public Builder clearZOriginal() {
bitField0_ = (bitField0_ & ~0x00080000);
zOriginal_ = 0F;
onChanged();
return this;
}
private float xPrecision_ ;
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return Whether the xPrecision field is set.
*/
@java.lang.Override
public boolean hasXPrecision() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return The xPrecision.
*/
@java.lang.Override
public float getXPrecision() {
return xPrecision_;
}
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @param value The xPrecision to set.
* @return This builder for chaining.
*/
public Builder setXPrecision(float value) {
xPrecision_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* localization precision
*
*
* optional float x_precision = 104;
* @return This builder for chaining.
*/
public Builder clearXPrecision() {
bitField0_ = (bitField0_ & ~0x00100000);
xPrecision_ = 0F;
onChanged();
return this;
}
private float yPrecision_ ;
/**
* optional float y_precision = 105;
* @return Whether the yPrecision field is set.
*/
@java.lang.Override
public boolean hasYPrecision() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
* optional float y_precision = 105;
* @return The yPrecision.
*/
@java.lang.Override
public float getYPrecision() {
return yPrecision_;
}
/**
* optional float y_precision = 105;
* @param value The yPrecision to set.
* @return This builder for chaining.
*/
public Builder setYPrecision(float value) {
yPrecision_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
* optional float y_precision = 105;
* @return This builder for chaining.
*/
public Builder clearYPrecision() {
bitField0_ = (bitField0_ & ~0x00200000);
yPrecision_ = 0F;
onChanged();
return this;
}
private float zPrecision_ ;
/**
* optional float z_precision = 106;
* @return Whether the zPrecision field is set.
*/
@java.lang.Override
public boolean hasZPrecision() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
* optional float z_precision = 106;
* @return The zPrecision.
*/
@java.lang.Override
public float getZPrecision() {
return zPrecision_;
}
/**
* optional float z_precision = 106;
* @param value The zPrecision to set.
* @return This builder for chaining.
*/
public Builder setZPrecision(float value) {
zPrecision_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
* optional float z_precision = 106;
* @return This builder for chaining.
*/
public Builder clearZPrecision() {
bitField0_ = (bitField0_ & ~0x00400000);
zPrecision_ = 0F;
onChanged();
return this;
}
private int xPosition_ ;
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return Whether the xPosition field is set.
*/
@java.lang.Override
public boolean hasXPosition() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return The xPosition.
*/
@java.lang.Override
public int getXPosition() {
return xPosition_;
}
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @param value The xPosition to set.
* @return This builder for chaining.
*/
public Builder setXPosition(int value) {
xPosition_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* position in the original image (in pixels) used for fitting
*
*
* optional int32 x_position = 107;
* @return This builder for chaining.
*/
public Builder clearXPosition() {
bitField0_ = (bitField0_ & ~0x00800000);
xPosition_ = 0;
onChanged();
return this;
}
private int yPosition_ ;
/**
* optional int32 y_position = 108;
* @return Whether the yPosition field is set.
*/
@java.lang.Override
public boolean hasYPosition() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
* optional int32 y_position = 108;
* @return The yPosition.
*/
@java.lang.Override
public int getYPosition() {
return yPosition_;
}
/**
* optional int32 y_position = 108;
* @param value The yPosition to set.
* @return This builder for chaining.
*/
public Builder setYPosition(int value) {
yPosition_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
* optional int32 y_position = 108;
* @return This builder for chaining.
*/
public Builder clearYPosition() {
bitField0_ = (bitField0_ & ~0x01000000);
yPosition_ = 0;
onChanged();
return this;
}
private double error_ ;
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return Whether the error field is set.
*/
@java.lang.Override
public boolean hasError() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return The error.
*/
@java.lang.Override
public double getError() {
return error_;
}
/**
*
* Fit error
*
*
* optional double error = 1500;
* @param value The error to set.
* @return This builder for chaining.
*/
public Builder setError(double value) {
error_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* Fit error
*
*
* optional double error = 1500;
* @return This builder for chaining.
*/
public Builder clearError() {
bitField0_ = (bitField0_ & ~0x02000000);
error_ = 0D;
onChanged();
return this;
}
private float noise_ ;
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return Whether the noise field is set.
*/
@java.lang.Override
public boolean hasNoise() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return The noise.
*/
@java.lang.Override
public float getNoise() {
return noise_;
}
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @param value The noise to set.
* @return This builder for chaining.
*/
public Builder setNoise(float value) {
noise_ = value;
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
* Local noise estimate
*
*
* optional float noise = 1501;
* @return This builder for chaining.
*/
public Builder clearNoise() {
bitField0_ = (bitField0_ & ~0x04000000);
noise_ = 0F;
onChanged();
return this;
}
private int endFrame_ ;
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return Whether the endFrame field is set.
*/
@java.lang.Override
public boolean hasEndFrame() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return The endFrame.
*/
@java.lang.Override
public int getEndFrame() {
return endFrame_;
}
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @param value The endFrame to set.
* @return This builder for chaining.
*/
public Builder setEndFrame(int value) {
endFrame_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
* The end frame (if the spot represents an average across multiple frames)
*
*
* optional int32 end_frame = 1503;
* @return This builder for chaining.
*/
public Builder clearEndFrame() {
bitField0_ = (bitField0_ & ~0x08000000);
endFrame_ = 0;
onChanged();
return this;
}
private float originalValue_ ;
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return Whether the originalValue field is set.
*/
@java.lang.Override
public boolean hasOriginalValue() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return The originalValue.
*/
@java.lang.Override
public float getOriginalValue() {
return originalValue_;
}
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @param value The originalValue to set.
* @return This builder for chaining.
*/
public Builder setOriginalValue(float value) {
originalValue_ = value;
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
*
* The original pixel value at the fitting origin (x_position,y_position)
*
*
* optional float original_value = 1504;
* @return This builder for chaining.
*/
public Builder clearOriginalValue() {
bitField0_ = (bitField0_ & ~0x10000000);
originalValue_ = 0F;
onChanged();
return this;
}
private com.google.protobuf.Internal.FloatList paramStdDevs_ = emptyFloatList();
private void ensureParamStdDevsIsMutable() {
if (!((bitField0_ & 0x20000000) != 0)) {
paramStdDevs_ = mutableCopy(paramStdDevs_);
bitField0_ |= 0x20000000;
}
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return A list containing the paramStdDevs.
*/
public java.util.List
getParamStdDevsList() {
return ((bitField0_ & 0x20000000) != 0) ?
java.util.Collections.unmodifiableList(paramStdDevs_) : paramStdDevs_;
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return The count of paramStdDevs.
*/
public int getParamStdDevsCount() {
return paramStdDevs_.size();
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @param index The index of the element to return.
* @return The paramStdDevs at the given index.
*/
public float getParamStdDevs(int index) {
return paramStdDevs_.getFloat(index);
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @param index The index to set the value at.
* @param value The paramStdDevs to set.
* @return This builder for chaining.
*/
public Builder setParamStdDevs(
int index, float value) {
ensureParamStdDevsIsMutable();
paramStdDevs_.setFloat(index, value);
onChanged();
return this;
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @param value The paramStdDevs to add.
* @return This builder for chaining.
*/
public Builder addParamStdDevs(float value) {
ensureParamStdDevsIsMutable();
paramStdDevs_.addFloat(value);
onChanged();
return this;
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @param values The paramStdDevs to add.
* @return This builder for chaining.
*/
public Builder addAllParamStdDevs(
java.lang.Iterable extends java.lang.Float> values) {
ensureParamStdDevsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, paramStdDevs_);
onChanged();
return this;
}
/**
*
* The estimated standard deviations of each of the fitted parameters.
* The GDSC SMLM code uses a fixed array of size 7 for fitted parameters:
* [Background,Signal,Theta,X,Y,X-width,Y-width].
* Parameters that are not fit will have zero in the array.
*
*
* repeated float param_std_devs = 1505;
* @return This builder for chaining.
*/
public Builder clearParamStdDevs() {
paramStdDevs_ = emptyFloatList();
bitField0_ = (bitField0_ & ~0x20000000);
onChanged();
return this;
}
private float meanIntensity_ ;
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return Whether the meanIntensity field is set.
*/
@java.lang.Override
public boolean hasMeanIntensity() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return The meanIntensity.
*/
@java.lang.Override
public float getMeanIntensity() {
return meanIntensity_;
}
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @param value The meanIntensity to set.
* @return This builder for chaining.
*/
public Builder setMeanIntensity(float value) {
meanIntensity_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
* The mean intensity of the spot.
* This can be the peak spot intensity in the PSF which is simple to compute.
* It can be the integrated intensity over the main region of the spot
* divided by the area. E.g. This may be
* the intensity within the region with a value greater than half-maxima.
*
*
* optional float mean_intensity = 1506;
* @return This builder for chaining.
*/
public Builder clearMeanIntensity() {
bitField0_ = (bitField0_ & ~0x40000000);
meanIntensity_ = 0F;
onChanged();
return this;
}
private int category_ ;
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return Whether the category field is set.
*/
@java.lang.Override
public boolean hasCategory() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return The category.
*/
@java.lang.Override
public int getCategory() {
return category_;
}
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @param value The category to set.
* @return This builder for chaining.
*/
public Builder setCategory(int value) {
category_ = value;
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
*
* The category of the localisation.
* This may be used for example to sub-divide localisations in the same track
* (identified by the molecule) into categories based on diffusion speed.
*
*
* optional int32 category = 1507;
* @return This builder for chaining.
*/
public Builder clearCategory() {
bitField0_ = (bitField0_ & ~0x80000000);
category_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:TSF.Spot)
}
// @@protoc_insertion_point(class_scope:TSF.Spot)
private static final uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot();
}
public static uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Spot parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public uk.ac.sussex.gdsc.smlm.tsf.TSFProtos.Spot getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TSF_FluorophoreType_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_TSF_FluorophoreType_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TSF_ROI_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_TSF_ROI_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TSF_SpotList_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_TSF_SpotList_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TSF_Spot_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_TSF_Spot_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n$uk/ac/sussex/gdsc/smlm/tsf/tsf.proto\022\003" +
"TSF\"G\n\017FluorophoreType\022\n\n\002id\030\001 \002(\005\022\023\n\013de" +
"scription\030\002 \001(\t\022\023\n\013is_fiducial\030\003 \001(\010\"=\n\003" +
"ROI\022\t\n\001x\030\001 \002(\005\022\t\n\001y\030\002 \002(\005\022\017\n\007x_width\030\003 \002" +
"(\005\022\017\n\007y_width\030\004 \002(\005\"\361\005\n\010SpotList\022\031\n\016appl" +
"ication_id\030\001 \002(\005:\0011\022\014\n\004name\030\002 \001(\t\022\020\n\010fil" +
"epath\030\003 \001(\t\022\013\n\003uid\030\004 \001(\003\022\023\n\013nr_pixels_x\030" +
"\005 \001(\005\022\023\n\013nr_pixels_y\030\006 \001(\005\022\022\n\npixel_size" +
"\030\007 \001(\002\022\020\n\010nr_spots\030\010 \001(\003\022\020\n\010box_size\030\021 \001" +
"(\005\022\023\n\013nr_channels\030\022 \001(\005\022\021\n\tnr_frames\030\023 \001" +
"(\005\022\021\n\tnr_slices\030\024 \001(\005\022\016\n\006nr_pos\030\025 \001(\005\022/\n" +
"\021fluorophore_types\030\032 \003(\0132\024.TSF.Fluoropho" +
"reType\022*\n\016location_units\030\026 \001(\0162\022.TSF.Loc" +
"ationUnits\022,\n\017intensity_units\030\027 \001(\0162\023.TS" +
"F.IntensityUnits\022$\n\013theta_units\030\033 \001(\0162\017." +
"TSF.ThetaUnits\022\036\n\010fit_mode\030\030 \001(\0162\014.TSF.F" +
"itMode\022\027\n\010is_track\030\031 \001(\010:\005false\022\013\n\003ecf\030\034" +
" \003(\001\022\n\n\002qe\030\036 \003(\001\022\025\n\003roi\030\035 \001(\0132\010.TSF.ROI\022" +
"\017\n\006source\030\335\013 \001(\t\022\026\n\rconfiguration\030\336\013 \001(\t" +
"\022\r\n\004gain\030\337\013 \001(\001\022\026\n\rexposure_time\030\340\013 \001(\001\022" +
"\023\n\nread_noise\030\341\013 \001(\001\022\r\n\004bias\030\342\013 \001(\001\022%\n\013c" +
"amera_type\030\345\013 \001(\0162\017.TSF.CameraType\022\014\n\003PS" +
"F\030\346\013 \001(\t*\006\010\244\r\020\200\020J\006\010\343\013\020\344\013J\006\010\344\013\020\345\013R\006em_ccd" +
"R\ramplification\"\207\005\n\004Spot\022\020\n\010molecule\030\001 \002" +
"(\005\022\017\n\007channel\030\002 \002(\005\022\r\n\005frame\030\003 \002(\005\022\r\n\005sl" +
"ice\030\004 \001(\005\022\013\n\003pos\030\005 \001(\005\022\030\n\020fluorophore_ty" +
"pe\030\023 \001(\005\022\017\n\007cluster\030\024 \001(\005\022*\n\016location_un" +
"its\030\021 \001(\0162\022.TSF.LocationUnits\022\t\n\001x\030\007 \002(\002" +
"\022\t\n\001y\030\010 \002(\002\022\t\n\001z\030\t \001(\002\022,\n\017intensity_unit" +
"s\030\022 \001(\0162\023.TSF.IntensityUnits\022\021\n\tintensit" +
"y\030\n \002(\002\022\022\n\nbackground\030\013 \001(\002\022\r\n\005width\030\014 \001" +
"(\002\022\t\n\001a\030\r \001(\002\022\r\n\005theta\030\016 \001(\002\022\022\n\nx_origin" +
"al\030e \001(\002\022\022\n\ny_original\030f \001(\002\022\022\n\nz_origin" +
"al\030g \001(\002\022\023\n\013x_precision\030h \001(\002\022\023\n\013y_preci" +
"sion\030i \001(\002\022\023\n\013z_precision\030j \001(\002\022\022\n\nx_pos" +
"ition\030k \001(\005\022\022\n\ny_position\030l \001(\005\022\016\n\005error" +
"\030\334\013 \001(\001\022\016\n\005noise\030\335\013 \001(\002\022\022\n\tend_frame\030\337\013 " +
"\001(\005\022\027\n\016original_value\030\340\013 \001(\002\022\027\n\016param_st" +
"d_devs\030\341\013 \003(\002\022\027\n\016mean_intensity\030\342\013 \001(\002\022\021" +
"\n\010category\030\343\013 \001(\005*\006\010\244\r\020\200\020*8\n\007FitMode\022\013\n\007" +
"ONEAXIS\020\000\022\013\n\007TWOAXIS\020\001\022\023\n\017TWOAXISANDTHET" +
"A\020\002*&\n\nThetaUnits\022\013\n\007DEGREES\020\000\022\013\n\007RADIAN" +
"S\020\001*)\n\016IntensityUnits\022\n\n\006COUNTS\020\000\022\013\n\007PHO" +
"TONS\020\001*+\n\rLocationUnits\022\006\n\002NM\020\000\022\006\n\002UM\020\001\022" +
"\n\n\006PIXELS\020\002*+\n\nCameraType\022\007\n\003CCD\020\000\022\t\n\005EM" +
"CCD\020\001\022\t\n\005SCMOS\020\002B\'\n\032uk.ac.sussex.gdsc.sm" +
"lm.tsfB\tTSFProtos"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_TSF_FluorophoreType_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_TSF_FluorophoreType_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_TSF_FluorophoreType_descriptor,
new java.lang.String[] { "Id", "Description", "IsFiducial", });
internal_static_TSF_ROI_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_TSF_ROI_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_TSF_ROI_descriptor,
new java.lang.String[] { "X", "Y", "XWidth", "YWidth", });
internal_static_TSF_SpotList_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_TSF_SpotList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_TSF_SpotList_descriptor,
new java.lang.String[] { "ApplicationId", "Name", "Filepath", "Uid", "NrPixelsX", "NrPixelsY", "PixelSize", "NrSpots", "BoxSize", "NrChannels", "NrFrames", "NrSlices", "NrPos", "FluorophoreTypes", "LocationUnits", "IntensityUnits", "ThetaUnits", "FitMode", "IsTrack", "Ecf", "Qe", "Roi", "Source", "Configuration", "Gain", "ExposureTime", "ReadNoise", "Bias", "CameraType", "PSF", });
internal_static_TSF_Spot_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_TSF_Spot_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_TSF_Spot_descriptor,
new java.lang.String[] { "Molecule", "Channel", "Frame", "Slice", "Pos", "FluorophoreType", "Cluster", "LocationUnits", "X", "Y", "Z", "IntensityUnits", "Intensity", "Background", "Width", "A", "Theta", "XOriginal", "YOriginal", "ZOriginal", "XPrecision", "YPrecision", "ZPrecision", "XPosition", "YPosition", "Error", "Noise", "EndFrame", "OriginalValue", "ParamStdDevs", "MeanIntensity", "Category", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy