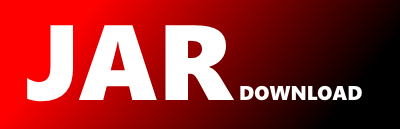
uk.ac.sussex.gdsc.smlm.utils.StdMath Maven / Gradle / Ivy
Show all versions of gdsc-smlm Show documentation
/*-
* #%L
* Genome Damage and Stability Centre SMLM Package
*
* Software for single molecule localisation microscopy (SMLM)
* %%
* Copyright (C) 2011 - 2023 Alex Herbert
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
package uk.ac.sussex.gdsc.smlm.utils;
import java.util.function.DoubleUnaryOperator;
/**
* Class for computing standard math functions.
*
* Provides an entry point to routines, or their equivalent, found in {@link java.lang.Math}.
*/
public final class StdMath {
/** Exponential function. */
private static final DoubleUnaryOperator EXP;
static {
// The Math.exp function in Java 8 is slower than FastMath.
// From Java 9 onwards the Math.exp function is faster.
if (System.getProperty("java.specification.version").startsWith("1.8")) {
EXP = org.apache.commons.math3.util.FastMath::exp;
} else {
EXP = Math::exp;
}
}
/** No public constructor. */
private StdMath() {}
/**
* Returns Euler's number e raised to the power of {@code x}.
*
* @param x the exponent to raise e to.
* @return the value e{@code x}, where e is the base of the natural
* logarithms.
* @see Math#exp(double)
*/
public static double exp(double x) {
return EXP.applyAsDouble(x);
}
}