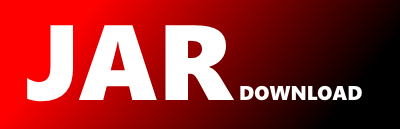
uk.co.caeldev.springsecuritymongo.domain.MongoClientDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-security-mongo Show documentation
Show all versions of spring-security-mongo Show documentation
A Commons Library to provide the repositories for mongo db
package uk.co.caeldev.springsecuritymongo.domain;
import org.springframework.data.annotation.Id;
import org.springframework.data.annotation.PersistenceConstructor;
import org.springframework.data.mongodb.core.mapping.Document;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.oauth2.provider.ClientDetails;
import java.util.*;
@Document
public class MongoClientDetails implements ClientDetails {
@Id
private String clientId;
private String clientSecret;
private Set scope = Collections.emptySet();
private Set resourceIds = Collections.emptySet();
private Set authorizedGrantTypes = Collections.emptySet();
private Set registeredRedirectUris;
private List authorities = Collections.emptyList();
private Integer accessTokenValiditySeconds;
private Integer refreshTokenValiditySeconds;
private Map additionalInformation = new LinkedHashMap();
private Set autoApproveScopes;
public MongoClientDetails() {
}
@PersistenceConstructor
public MongoClientDetails(final String clientId,
final String clientSecret,
final Set scope,
final Set resourceIds,
final Set authorizedGrantTypes,
final Set registeredRedirectUris,
final List authorities,
final Integer accessTokenValiditySeconds,
final Integer refreshTokenValiditySeconds,
final Map additionalInformation,
final Set autoApproveScopes) {
this.clientId = clientId;
this.clientSecret = clientSecret;
this.scope = scope;
this.resourceIds = resourceIds;
this.authorizedGrantTypes = authorizedGrantTypes;
this.registeredRedirectUris = registeredRedirectUris;
this.authorities = authorities;
this.accessTokenValiditySeconds = accessTokenValiditySeconds;
this.refreshTokenValiditySeconds = refreshTokenValiditySeconds;
this.additionalInformation = additionalInformation;
this.autoApproveScopes = autoApproveScopes;
}
public String getClientId() {
return clientId;
}
public String getClientSecret() {
return clientSecret;
}
public Set getScope() {
return scope;
}
public Set getResourceIds() {
return resourceIds;
}
public Set getAuthorizedGrantTypes() {
return authorizedGrantTypes;
}
public List getAuthorities() {
return authorities;
}
public Integer getAccessTokenValiditySeconds() {
return accessTokenValiditySeconds;
}
public Integer getRefreshTokenValiditySeconds() {
return refreshTokenValiditySeconds;
}
public Map getAdditionalInformation() {
return additionalInformation;
}
public void setAutoApproveScopes(final Set autoApproveScopes) {
this.autoApproveScopes = autoApproveScopes;
}
public Set getAutoApproveScopes() {
return autoApproveScopes;
}
@Override
public boolean isScoped() {
return this.scope != null && !this.scope.isEmpty();
}
@Override
public boolean isSecretRequired() {
return this.clientSecret != null;
}
@Override
public Set getRegisteredRedirectUri() {
return registeredRedirectUris;
}
@Override
public boolean isAutoApprove(final String scope) {
if (autoApproveScopes == null) {
return false;
}
for (String auto : autoApproveScopes) {
if (auto.equals("true") || scope.matches(auto)) {
return true;
}
}
return false;
}
@Override
public int hashCode() {
return Objects.hash(clientId, clientSecret, scope, resourceIds, authorizedGrantTypes, registeredRedirectUris, authorities, accessTokenValiditySeconds, refreshTokenValiditySeconds, additionalInformation, autoApproveScopes);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
final MongoClientDetails other = (MongoClientDetails) obj;
return Objects.equals(this.clientId, other.clientId)
&& Objects.equals(this.clientSecret, other.clientSecret)
&& Objects.equals(this.scope, other.scope)
&& Objects.equals(this.resourceIds, other.resourceIds)
&& Objects.equals(this.authorizedGrantTypes, other.authorizedGrantTypes)
&& Objects.equals(this.registeredRedirectUris, other.registeredRedirectUris)
&& Objects.equals(this.authorities, other.authorities)
&& Objects.equals(this.accessTokenValiditySeconds, other.accessTokenValiditySeconds)
&& Objects.equals(this.refreshTokenValiditySeconds, other.refreshTokenValiditySeconds)
&& Objects.equals(this.additionalInformation, other.additionalInformation)
&& Objects.equals(this.autoApproveScopes, other.autoApproveScopes);
}
@Override
public String toString() {
return "MongoClientDetails{" +
"clientId='" + clientId + '\'' +
", clientSecret='" + clientSecret + '\'' +
", scope=" + scope +
", resourceIds=" + resourceIds +
", authorizedGrantTypes=" + authorizedGrantTypes +
", registeredRedirectUris=" + registeredRedirectUris +
", authorities=" + authorities +
", accessTokenValiditySeconds=" + accessTokenValiditySeconds +
", refreshTokenValiditySeconds=" + refreshTokenValiditySeconds +
", additionalInformation=" + additionalInformation +
", autoApproveScopes=" + autoApproveScopes +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy