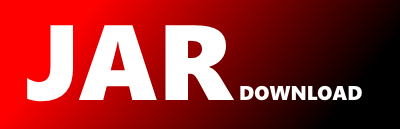
com.etsy.net.UnixDomainSocketClient Maven / Gradle / Ivy
package com.etsy.net;
import java.io.IOException;
import java.io.InputStream;
public class UnixDomainSocketClient extends UnixDomainSocket {
/**
* Creates a Unix domain socket and connects it to the server specified by
* the socket file.
*
* @param socketFile
* Name of the socket file
*
* @exception IOException
* If unable to construct the socket
*/
public UnixDomainSocketClient(String socketFile, int socketType)
throws IOException {
super.socketFile = socketFile;
super.socketType = socketType;
if ((nativeSocketFileHandle = nativeOpen(socketFile, socketType)) == -1)
throw new IOException("Unable to open Unix domain socket");
// Initialize the socket input and output streams
if (socketType == JUDS.SOCK_STREAM)
in = new UnixDomainSocketInputStream();
out = new UnixDomainSocketOutputStream();
}
/**
* Returns an input stream for this socket.
*
* @exception UnsupportedOperationException
* if getInputStream
is invoked for an
* UnixDomainSocketClient
of type
* JUDS.SOCK_DGRAM
.
* @return An input stream for writing bytes to this socket
*/
@Override
public InputStream getInputStream() {
if (socketType == JUDS.SOCK_STREAM)
return (InputStream) in;
else
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy