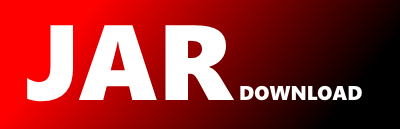
uk.co.it.modular.beans.BeanUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bean-utils Show documentation
Show all versions of bean-utils Show documentation
Utilities for manipulating and inspecting Java classes implementing the Java Beans standard
The newest version!
package uk.co.it.modular.beans;
import java.util.List;
import java.util.Map;
import static uk.co.it.modular.beans.Bean.bean;
import static uk.co.it.modular.beans.Type.type;
/**
* Utility methods for inspecting Objects which expose properties which follow the Java Bean get/set standard
*
* @author Stewart Bissett
*/
public abstract class BeanUtils {
/**
* Return a list of the publicly exposes get/set properties on the Bean. For example:
*
*
*
* List<BeanPropertyInstance> properties = BeanUtils.propertyList(myObject)
*
*
* @param instance an object to get the properties list from
*/
public static List propertyList(final Object instance) {
return bean(instance).propertyList();
}
/**
* Return a list of the publicly exposes get/set properties on a class. For example:
*
*
*
* List<BeanProperty> properties = BeanUtils.propertyList(MyObject.class)
*
*
* @param type a class to get the properties list from
*/
public static List propertyList(final Class> type) {
return type(type).propertyList();
}
/**
* Return a map of the publicly exposes get/set properties on the Bean with the property name as the key and the initial character lowercased For example:
*
*
*
* Map<String, BeanPropertyInstance> propertyMap = BeanUtils.propertyMap(myObject)
*
*
* @param instance an object to get the properties for
*/
public static Map propertyMap(final Object instance) {
return bean(instance).propertyMap();
}
/**
* Return a map of the publicly exposes get/set properties on the type with the property name as the key and the initial character lowercased For example:
*
*
*
* Map<String, BeanProperty> propertyMap = BeanUtils.propertyMap(MyObject.class)
*
*
* @param type a type to get the properties for
*/
public static Map propertyMap(final Class> type) {
return type(type).propertyMap();
}
/**
* Test if the supplied instance has a Bean property with the given name. For example
*
*
* if ( BeanUtils.hasProperty(aUser, "surname"))) {
* // Do Something;
* }
*
*
* @param instance an object to test against
* @param name the property name
*/
public static boolean hasProperty(final Object instance, final String name) {
return bean(instance).hasProperty(name);
}
/**
* Test if the supplied instance has a Bean property which matches the given predicate. For example:
*
*
*
* if ( BeanUtils.hasProperty(aUser, BeanPredicates.named("surname")))) {
* // Do Something;
* }
*
*
* @param instance an object to test against
* @param name the property name
*/
public static boolean hasProperty(final Object instance, final BeanPropertyPredicate predicate) {
return bean(instance).hasProperty(predicate);
}
/**
* Test if the supplied type has a property with the given name. For example:
*
*
* if ( BeanUtils.hasProperty(MyObject.class, "surname"))) {
* // Do Something;
* }
*
*
* @param type an type to test against
* @param name the property name
*/
public static boolean hasProperty(final Class> type, final String name) {
return type(type).hasProperty(name);
}
/**
* Get the requested property from the instance or return null
if the property is not present. For example:
*
*
* BeanPropertyInstance surname = BeanUtils.propertyNamed(aUser, "surname")
*
*
* @param instance an object to get the property from
* @param name the property name
*/
public static BeanProperty propertyNamed(final Object instance, final String name) {
return bean(instance).propertyNamed(name);
}
/**
* Get the requested property from the type or return null
if the property is not present. For example:
*
*
* BeanProperty surname = BeanUtils.propertyNamed(MyObject.class, "surname")
*
*
* @param type a type to get the property from
* @param name the property name
*/
public static TypeProperty propertyNamed(final Class> type, final String name) {
return type(type).propertyNamed(name);
}
/**
* Get the requested property from the type or return null
if the property is not present. For example:
*
*
* BeanPropertyInstance surname = BeanUtils.get(aUser, "surname")
*
*
* @param instance an instance to get the property from
* @param name the property name
*/
public static BeanProperty get(final Object instance, final String name) {
return bean(instance).get(name);
}
/**
* Get the property which matches the predicate from the instance or return null
if not matching property is found. For example:
*
*
* BeanPropertyInstance surname = BeanUtils.get(aUser, BeanPredicates.named("surname"))
*
*
* @param instance an instance to get the property from
* @param predicate a predicate to match the property
*/
public static BeanProperty get(final Object instance, final BeanPropertyPredicate predicate) {
return bean(instance).get(predicate);
}
/**
* Get the requested property from the type or return null
if the property is not present. For example:
*
*
* BeanProperty surname = BeanUtils.get(MyObject.class, "surname")
*
*
* @param type a type to get the property from
* @param name the property name
*/
public static TypeProperty get(final Class> type, final String name) {
return type(type).get(name);
}
/**
* Set the requested property on the given instance. For example:
*
*
* BeanUtils.setProperty(aUser, "surname", "Smith")
*
*
* @param instance an instance to get the property from
* @param name the property name
* @param value the property value
*/
public static boolean setProperty(final Object instance, final String name, final Object value) {
return bean(instance).setProperty(name, value);
}
/**
* Set the property which matches the predicate on the given instance. For example:
*
*
* BeanUtils.setProperty(aUser, BeanPredicates.named("surname"), "Smith")
*
*
* @param instance an instance to get the property from
* @param predicate a predicate to match the properties
* @param value the property value
*/
public static boolean setProperty(final Object instance, final BeanPropertyPredicate predicate, final Object value) {
return bean(instance).setProperty(predicate, value);
}
/**
* Return the property value on the instance for the supplied property name or return null
if the property is not present on the instance. For example:
*
*
* Object value = BeanUtils.propertyValue(aUser, "surname"))
*
*
* @param instance an object to get the property from
* @param name the property name
*/
public static Object propertyValue(final Object instance, final String propertyName) {
return bean(instance).propertyValue(propertyName);
}
/**
* Return the first property value on the instance which matches the predicate or return null
if the no matching properties are not present on the instance. For
* example:
*
*
* Object value = BeanUtils.propertyValue(aUser, BeanPredicates.named("surname")))
*
*
* @param instance an object to get the property from
* @param name the property name
*/
public static Object propertyValue(final Object instance, final BeanPropertyPredicate predicated) {
return bean(instance).propertyValue(predicated);
}
/**
* Return the property value on the instance for the supplied property name or return null
if the property is not present on the instance. For example:
*
*
* String value = BeanUtils.propertyValue(aUser, "surname", String.class))
*
*
* @param instance an object to get the property from
* @param name the property name
* @param type the type to return the property value as
*/
public static T propertyValue(final Object instance, final String propertyName, final Class type) {
return bean(instance).propertyValue(propertyName, type);
}
/**
* Return the first property value on the instance which matches the predicate or return null
if the no matching properties are not present on the instance. For
* example:
*
*
* String value = BeanUtils.propertyValue(aUser, BeanPredicates.named("surname")), String.class)
*
*
* @param instance an object to get the property from
* @param name the property name
* @param type the type to return the property value as
*/
public static T propertyValue(final Object instance, final BeanPropertyPredicate predicate, final Class type) {
return bean(instance).propertyValue(predicate, type);
}
/**
* Return the property type on the instance for the supplied property name or null
if the property doesn't exist. For example:
*
*
* if (String.class.equals(BeanUtils.propertyType(aUser, "surname"))) {
* // Do something
* }
*
*
* @param instance an object to get the property from
* @param name the property name
*/
public static Class> propertyType(final Object instance, final String propertyName) {
return bean(instance).propertyType(propertyName);
}
/**
* Return the property type on the type for the supplied property name or null
if the property doesn't exist. For example:
*
*
* if (String.class.equals(BeanUtils.propertyType(MyObject.class, "surname"))) {
* // Do something
* }
*
*
* @param type a type to get the property from
* @param name the property name
*/
public static Class> propertyType(final Class> type, final String propertyName) {
return type(type).propertyType(propertyName);
}
/**
* Return the property type on the instance for the first property which matches the supplied predicate or null
if no matching properties are found. For
* example:
*
*
* if (String.class.equals(BeanUtils.propertyType(aUser, BeanPredicates.named("surname")))) {
* // Do something
* }
*
*
* @param instance an object to get the property from
* @param name the property name
*/
public static Class> propertyType(final Object instance, final BeanPropertyPredicate predicate) {
return bean(instance).propertyType(predicate);
}
/**
* Test if the property on the supplied instance is of the supplied type. For example:
*
*
* if (BeanUtils.isPropertyType(aUser, "surname", String.class)) {
* // Do something
* }
*
* @param instance an object to get the property from
* @param name the property name
* @param type the expected type of the property
*/
public static boolean isPropertyType(final Object instance, final String propertyName, final Class> type) {
return bean(instance).isPropertyType(propertyName, type);
}
/**
* Test if the property which matches the predicate on the supplied instance is of the supplied type. For example:
*
*
* if (BeanUtils.isPropertyType(aUser, "surname", String.class)) {
* // Do something
* }
*
* @param instance an object to get the property from
* @param predicate a predicate to match the properties
* @param type the expected type of the property
*/
public static boolean isPropertyType(final Object instance, final BeanPropertyPredicate predicate, final Class> type) {
return bean(instance).isPropertyType(predicate, type);
}
/**
* Test if the property on the type is of the supplied type. For example:
*
*
* if (BeanUtils.isPropertyType(MyObject.class, "surname", String.class)) {
* // Do something
* }
*
* @param type a type to get the property from
* @param name the property name
* @param type the expected type of the property
*/
public static boolean isPropertyType(final Class> type, final String propertyName, final Class> expectedType) {
return type(type).isPropertyType(propertyName, expectedType);
}
/**
* Find all property properties which match the predicate. For example
*
*
* for ( BeanPropertyInstance property : BeanUtils.find(aUser, BeanPredicates.ofType(String.class)) {
* property.setValue(null);
* }
*
*
* @param instance an object to get the property from
* @param predicate a predicate to match the properties
*/
public static List find(final Object instance, final BeanPropertyPredicate predicate) {
return bean(instance).find(predicate);
}
/**
* Find the first instance of the property which matches the given predicate in the instance. For example
*
*
* BeanPropertyInstance property = BeanUtils.findAny(aUser, BeanPredicates.withValue("name" "Bob"))
*
*
* @param instance an object to get the property from
* @param predicate a predicate to match the properties
*/
public static BeanProperty findAny(final Object instance, final BeanPropertyPredicate predicate) {
return bean(instance).findAny(predicate);
}
/**
* Apply the {@link BeanPropertyFunction} to all properties which match the predicate in the supplied instances. For example
*
*
* BeanUtils.apply(aUser, BeanPredicates.ofType(String.class), BeanFunctions.setValue(null))
*
*
* @param instance an object to get the property from
* @param function the function to apply to the matching properties
* @param predicate a predicate to match the properties
*/
public static void apply(final Object instance, final BeanPropertyFunction function, final BeanPropertyPredicate predicate) {
bean(instance).apply(function, predicate);
}
/**
* Apply the {@link BeanPropertyFunction} to all properties on the supplied instance. For example
*
*
* BeanUtils.apply(aUser, BeanFunctions.setValue(null))
*
*
* @param instance an object to get the property from
* @param function the function to apply to the matching properties
*/
public static void apply(final Object instance, final BeanPropertyFunction function) {
bean(instance).apply(function);
}
/**
* Visit the supplied bean instance and notify the visitor for each bean property found. For example:
*
*
* BeanUtils.visit(aUser, new BeanPropertyVisitor() {
*
* public void visit(final BeanPropertyInstance property, final Object current, final BeanPropertyPath path, final Object[] stack) {
* System.out.println(path.fullPath());
* }
* });
*
*
* @param instance an object to get the property from
* @param visitor the visitor which will be notified of every bean property encountered
*/
public static void visit(final Object instance, final BeanVisitor visitor) {
bean(instance).visit(visitor);
}
/**
* Visit the supplied class and notify the visitor for each bean property found. For example:
*
*
* BeanUtils.visit(MyObject.class, new BeanPropertyVisitor() {
*
* public void visit(final BeanProperty property) {
* System.out.println(property.getName());
* }
* });
*
*
* @param type the type to get the property from
* @param visitor the visitor which will be notified of every bean property encountered
*/
public static void visit(final Class> type, final TypeVisitor visitor) {
type(type).visit(visitor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy