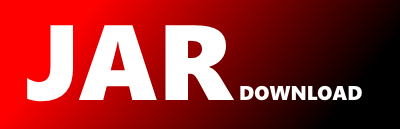
uk.co.it.modular.beans.GraphUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bean-utils Show documentation
Show all versions of bean-utils Show documentation
Utilities for manipulating and inspecting Java classes implementing the Java Beans standard
package uk.co.it.modular.beans;
import static uk.co.it.modular.beans.Graph.graph;
import java.util.List;
import java.util.Map;
/**
* Utility methods for inspecting Objects which expose properties which follow the Java Bean get/set standard
*
* @author Stewart Bissett
*/
public abstract class GraphUtils {
/**
* Return a list of the publicly exposes get/set properties on the Bean. For example:
*
*
* List<BeanProperty> properties = BeanUtils.propertyList(myObject);
*
* @param instance
* an object to get the properties list from
*/
public static List propertyList(final Object instance) {
return graph(instance).propertyList();
}
/**
* Return a map of the publicly exposes get/set properties on the Bean with the property name as the key and the initial character lowercased For example:
*
*
*
* Map<String, BeanProperty> propertyMap = BeanUtils.propertyMap(myObject);
*
* @param instance
* an object to get the properties for
*/
public static Map propertyMap(final Object instance) {
return graph(instance).propertyMap();
}
/**
* Test if the supplied instance has a Bean property with the given name
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanUtils.hasProperty(user, "surname")) == true;
*
* @param instance
* an object to test against
* @param name
* the property name
*/
public static boolean hasProperty(final Object instance, final String name) {
return graph(instance).hasProperty(name);
}
/**
* Test if the supplied instance has a Bean property with the given name
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanUtils.hasProperty(user, "surname")) == true;
*
* @param instance
* an object to test against
* @param name
* the property name
*/
public static boolean hasProperty(final Object instance, final BeanPropertyPredicate predicate) {
return graph(instance).hasProperty(predicate);
}
/**
* Get the requested property from the instance or return null
if the property is not present
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanProperty surname = BeanUtils.property(myUser, "surname");
*
* @param instance
* an object to get the property from
* @param name
* the property name
*/
public static BeanPropertyInstance propertyNamed(final Object instance, final String name) {
return graph(instance).propertyNamed(name);
}
/**
* Set the property value on the instance to the supplied value.
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanUtils.setProperty(myUser, "surname", "Smith");
*
* @param instance
* an object to get the property from
* @param name
* the property name
* @param value
* the value to set the property to
*/
public static boolean setProperty(final Object instance, final String name, final Object value) {
return graph(instance).setProperty(name, value);
}
/**
* Set the property value on the instance to the supplied value.
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanUtils.setProperty(myUser, "surname", "Smith");
*
* @param instance
* an object to get the property from
* @param name
* the property name
* @param value
* the value to set the property to
*/
public static boolean setProperty(final Object instance, final BeanPropertyPredicate predicate, final Object value) {
return graph(instance).setProperty(predicate, value);
}
/**
* Return the property value on the instance for the supplied property name or return null
if the property is not present on the instance
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* "Smith".equals(BeanUtils.propertyValue(myUser, "surname"));
*
* @param instance
* an object to get the property from
* @param name
* the property name
*/
public static Object propertyValue(final Object instance, final String propertyName) {
return graph(instance).propertyValue(propertyName);
}
/**
* Return the property value on the instance to the supplied value or return null
if the property was not present on the instance
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* "Smith".equals(BeanUtils.getPropertyValue(myUser, "surname", String.class));
*
* @param instance
* an object to get the property from
* @param name
* the property name
* @param name
* the type to return the property as
*/
public static T propertyValue(final Object instance, final String propertyName, final Class type) {
return graph(instance).propertyValue(propertyName, type);
}
/**
* Return the property type on the instance for the supplied property name or null
if the property doesn't exist
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* String.class.equals(BeanUtils.propertyType(myUser, "surname"));
*
* @param instance
* an object to get the property from
* @param name
* the property name
*/
public static Class> propertyType(final Object instance, final String propertyName) {
return graph(instance).propertyType(propertyName);
}
/**
* Test if the property on the supplied instance is of the supplied type.
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanUtils.isPropertyType(myUser, "surname", String.class) == true;
*
* @param instance
* an object to get the property from
* @param name
* the property name
* @param type
* the expected type of the property
*/
public static boolean isPropertyType(final Object instance, final String propertyName, final Class> type) {
return graph(instance).isPropertyType(propertyName, type);
}
/**
* Test if the property on the supplied instance is of the supplied type.
*
* For example, a class with a property getSurname() and setSurname(...):
*
*
* BeanUtils.isPropertyType(myUser, "surname", String.class) == true;
*
* @param instance
* an object to get the property from
* @param name
* the property name
* @param type
* the expected type of the property
*/
public static boolean isPropertyType(final Object instance, final BeanPropertyPredicate predicate, final Class> type) {
return graph(instance).isPropertyType(predicate, type);
}
/**
* Find all property instances which match the predicate in the supplied instance and it's assosciates. For example
*
*
* List relativesCalledBob = BeanUtils.find(myFamilyTree, BeanPredicates.stringProperty("name" "Bob"));
*
*/
public static List find(final Object instance, final BeanPropertyPredicate predicate) {
return graph(instance).find(predicate);
}
/**
* Apply the {@link BeanPropertyFunction} to all properties which match the predicate in the supplied instance and in any of the objects assosciates. For example
*
*
* BeanUtils.applyToGraph(myFamilyTree, deletePerson(), isDeceased()));
*
*/
public static void apply(final Object instance, final BeanPropertyFunction function, final BeanPropertyPredicate predicate) {
graph(instance).apply(function, predicate);
}
/**
* Apply the {@link BeanPropertyFunction} to all properties in the supplied instance and in any of the objects assosciates. For example
*
*
* BeanUtils.applyToGraph(myFamilyTree, pruneDeceased()));
*
*/
public static void apply(final Object instance, final BeanPropertyFunction function) {
graph(instance).apply(function);
}
/**
* Find the first instance of the property which matches the given predicate in the given instance and it's assosciates. For example
*
*
* BeanPropery property = BeanUtils.findPropertyInGraph(myFamilyTree, BeanPredicates.withProperty("name" "Bob"));
*
*/
public static BeanPropertyInstance findAny(final Object instance, final BeanPropertyPredicate predicate) {
return graph(instance).findAny(predicate);
}
/**
* Visit the supplied bean instance and notify the visitor for each bean property found. This method will recurse into the object graph by looping over
* collections and will visit all properties on assosciated objects and their assosciated objects, and so on.
*
* This method will at most visit each object once even when the object refers to iteself
*
*
*
* BeanUtils.visit(myUser, new BeanPropertyVisitor() {
*
* public void visit(final Object[] stack, final String path, final Object current, final BeanProperty property) {
* printer.println("Hello " + property);
* }
* });
*
* @param instance
* an object to get the property from
* @param visitor
* the visitor which will be notified of every bean property encountered
*/
public static void visit(final Object instance, final BeanVisitor visitor) {
graph(instance).visit(visitor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy