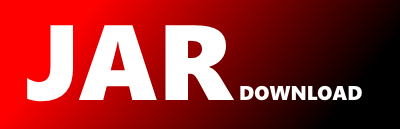
pacman.controllers.MASController Maven / Gradle / Ivy
Show all versions of pacman-main Show documentation
package pacman.controllers;
import pacman.game.Constants.GHOST;
import pacman.game.Constants.MOVE;
import pacman.game.Game;
import java.util.EnumMap;
/**
* Created by Piers on 11/11/2015.
*
* Makes the game PO for each controller
*/
public class MASController extends Controller> {
private final boolean po;
public MASController(boolean po, EnumMap controllers) {
this.po = po;
this.controllers = controllers;
}
private EnumMap myMoves = new EnumMap(GHOST.class);
protected EnumMap controllers = new EnumMap<>(GHOST.class);
public MASController(EnumMap controllers) {
this(true, controllers);
}
@Override
public final EnumMap getMove(Game game, long timeDue) {
myMoves.clear();
for (GHOST ghost : GHOST.values()) {
myMoves.put(
ghost,
controllers.get(ghost).getMove(
(po) ? game.copy(ghost) : game.copy(),
timeDue));
}
return myMoves;
}
/**
* This is a shallow copy used to alter the PO status to force it to a desired value
* @param po Should the copy enforce PO on the ghosts
* @return The copy created
*/
public final MASController copy(boolean po){
return new MASController(po, controllers);
}
}