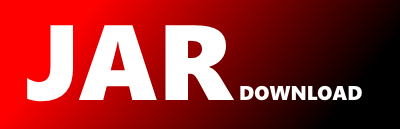
hvalspik.dse.DseContainerBuilder Maven / Gradle / Ivy
The newest version!
package hvalspik.dse;
import java.util.HashSet;
import java.util.Set;
import hvalspik.container.AbstractContainerBuilder;
import hvalspik.container.spec.PortMappingSpec;
import hvalspik.container.spec.SpecBuilder;
import hvalspik.sources.ContainerSource;
import hvalspik.sources.NamedContainerSource;
import hvalspik.wait.LogCheckWait;
import hvalspik.wait.PortListeningWait;
public final class DseContainerBuilder
extends AbstractContainerBuilder {
private static final Set PORTS = new HashSet<>();
private static final Set SEARCH_PORTS = new HashSet<>();
private static final Set SPARK_PORTS = new HashSet<>();
private static final Set GRAPH_PORTS = new HashSet<>();
private static final ContainerSource DEFAULT_SOURCE = NamedContainerSource.named("luketillman/datastax-enterprise:5.0.4");
private static final String COMMAND_SEARCH = "-s";
private static final String COMMAND_SPARK = "-k";
private static final String COMMAND_GRAPH = "-g";
static {
PORTS.add(9042);
PORTS.add(9160);
SEARCH_PORTS.add(8983);
SEARCH_PORTS.add(8984);
SPARK_PORTS.add(4040);
SPARK_PORTS.add(7077);
SPARK_PORTS.add(7080);
SPARK_PORTS.add(7081);
SPARK_PORTS.add(8090);
SPARK_PORTS.add(9999);
SPARK_PORTS.add(18080);
GRAPH_PORTS.add(8182);
}
private boolean withSearch = false;
private boolean withSpark = false;
private boolean withGraph = false;
private DseContainerBuilder(ContainerSource source, SpecBuilder specBuilder) {
super(source, specBuilder);
}
public static DseContainerBuilder defaultSource() {
return forSource(DEFAULT_SOURCE);
}
public static DseContainerBuilder forVersion(String version) {
return forSource(NamedContainerSource.named("harisekhon/hbase:" + version));
}
public static DseContainerBuilder forSource(ContainerSource containerSource) {
SpecBuilder delegate = SpecBuilder.create();
return new DseContainerBuilder(containerSource, delegate);
}
public DseContainerBuilder withSearch() {
withSearch = true;
return this;
}
public DseContainerBuilder withSpark() {
withSpark = true;
return this;
}
public DseContainerBuilder withGraph() {
withGraph = true;
return this;
}
@Override
public DseContainer build() {
PORTS.forEach(port -> getSpecBuilder().port(PortMappingSpec.random(port)).waitFor(PortListeningWait.portListening(port)));
Set commands = new HashSet<>();
withOption(withSearch, SEARCH_PORTS, COMMAND_SEARCH, commands);
withOption(withSpark, SPARK_PORTS, COMMAND_SPARK, commands);
withOption(withGraph, GRAPH_PORTS, COMMAND_GRAPH, commands);
getSpecBuilder().waitFor(LogCheckWait.logMessage("DSE startup complete"));
if (!commands.isEmpty()) {
getSpecBuilder().command(commands.toArray(new String[0]));
}
return new DseContainer(getName(), getSource(), getSpecBuilder().build(), getToCopy(),
getEventHandlers());
}
private void withOption(boolean enabled, Set ports, String command, Set commands) {
if (!enabled) {
return;
}
commands.add(command);
ports.forEach(port -> getSpecBuilder().port(PortMappingSpec.random(port)).waitFor(PortListeningWait.portListening(port)));
}
@Override
protected DseContainerBuilder instance() {
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy