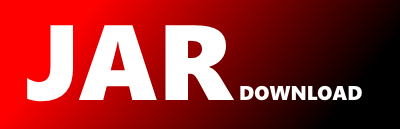
hvalspik.maven.DeployableUtils Maven / Gradle / Ivy
package hvalspik.maven;
import java.io.File;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Map;
import org.jboss.shrinkwrap.resolver.api.NoResolvedResultException;
import org.jboss.shrinkwrap.resolver.api.maven.Maven;
import org.jboss.shrinkwrap.resolver.api.maven.MavenResolverSystem;
import org.jboss.shrinkwrap.resolver.api.maven.MavenVersionRangeResult;
import org.jboss.shrinkwrap.resolver.api.maven.coordinate.MavenCoordinate;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.vdurmont.semver4j.Semver;
import com.vdurmont.semver4j.Semver.SemverType;
public final class DeployableUtils {
private static final String SNAPSHOT = "SNAPSHOT";
private static final Logger LOG = LoggerFactory.getLogger(DeployableUtils.class);
private static final SemverOrder ORDER = new SemverOrder();
public static Deployable downloadLatest(String groupId, String artifactId, DeployableType type) {
return downloadLatestDependency(groupId, artifactId, "0.0", type);
}
public static Deployable downloadLatestDependency(String groupId, String artifactId, String minVersion, DeployableType type) {
String version = getLatestVersion(groupId, artifactId, minVersion, type);
return downloadDependency(groupId, artifactId, version, type);
}
public static Deployable downloadDependency(String groupId, String artifactId, String version, DeployableType type) {
LOG.debug("Loading: {}/{}", groupId, artifactId);
String mavenVersion = formatMavenId(groupId, artifactId, version, type);
File resolvedFile = resolveVersion(mavenVersion);
if (resolvedFile == null) {
return null;
}
LOG.debug("Loaded: {}", resolvedFile.toString());
return new DeployableImpl(type, groupId, artifactId, version, resolvedFile);
}
private static File resolveVersion(String warVersion) {
try {
return getResolver().resolve(warVersion).withoutTransitivity().asSingleFile();
} catch (NoResolvedResultException e) {
LOG.warn("Could not find version", e);
return null;
}
}
private static String getLatestVersion(String groupId, String artifactId, String minVersion, DeployableType type) {
LOG.debug("Looking for version: {}/{}", groupId, artifactId);
String searchVersion = String.format("(%s,)", minVersion);
MavenVersionRangeResult versionRangeResult = getResolver().resolveVersionRange(formatMavenId(groupId, artifactId, searchVersion, type));
if (!versionRangeResult.getHighestVersion().getVersion().contains(SNAPSHOT)) {
return versionRangeResult.getHighestVersion().getVersion();
}
Map versionMap = new HashMap<>();
versionRangeResult.getVersions().stream().forEach(c -> {
versionMap.put(new Semver(c.getVersion(), SemverType.LOOSE), c);
});
Semver version = versionMap.keySet().stream()
.filter(v -> !v.getOriginalValue().contains(SNAPSHOT))
.max(ORDER).get();
LOG.debug("Found version: {}/{} -> {}", groupId, artifactId, version);
return versionMap.get(version).getVersion();
}
private static String formatMavenId(String groupId, String artifactId, String version, DeployableType type) {
return String.format("%s:%s:%s:%s", groupId, artifactId, type.getType(), version);
}
private static MavenResolverSystem getResolver() {
return Maven.resolver();
}
private static final class SemverOrder implements Comparator {
@Override
public int compare(Semver o1, Semver o2) {
return o1.compareTo(o2);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy