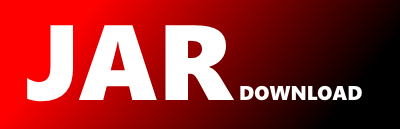
uk.co.thebadgerset.junit.maven.TKTestRunnerMojo Maven / Gradle / Ivy
/* Copyright Rupert Smith, 2005 to 2007, all rights reserved. */
/*
* Copyright 2007 Rupert Smith.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package uk.co.thebadgerset.junit.maven;
import java.io.File;
import java.lang.reflect.Method;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.*;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import uk.co.thebadgerset.junit.extensions.TKTestRunner;
/**
* CRC Card
* Responsibilities Collaborations
*
*
* @author Rupert Smith
*
* @goal tktest
* @phase test
* @execute phase="test"
* @requiresDependencyResolution test
*/
public class TKTestRunnerMojo extends AbstractMojo
{
private static final BitSet UNRESERVED = new BitSet(256);
/**
* The TKTest runner command lines. There are passed directly to the TKTestRunner main method.
*
* @parameter
*/
private Map commands = new LinkedHashMap();
/**
* The directory containing generated classes of the project being tested.
*
* @parameter expression="${project.build.outputDirectory}"
* @required
*/
private File classesDirectory;
/**
* The directory containing generated test classes of the project being tested.
*
* @parameter expression="${project.build.testOutputDirectory}"
* @required
*/
private File testClassesDirectory;
/**
* The classpath elements of the project being tested.
*
* @parameter expression="${project.testClasspathElements}"
* @required
* @readonly
*/
private List classpathElements;
/**
* List of System properties to pass to the tests.
*
* @parameter
*/
private Properties systemProperties;
/**
* Map of of plugin artifacts.
*
* @parameter expression="${plugin.artifactMap}"
* @required
* @readonly
*/
private Map pluginArtifactMap;
/**
* Map of of project artifacts.
*
* @parameter expression="${project.artifactMap}"
* @required
* @readonly
*/
private Map projectArtifactMap;
/**
* Option to specify the forking mode. Can be "never" (default), "once" or "always".
* "none" and "pertest" are also accepted for backwards compatibility.
*
* @parameter expression="${forkMode}" default-value="once"
*/
private String forkMode;
/**
* Option to specify the jvm (or path to the java executable) to use with
* the forking options. For the default we will assume that java is in the path.
*
* @parameter expression="${jvm}"
* default-value="java"
*/
private String jvm;
/**
* Implementation of the tktest goal.
*
* @throws MojoExecutionException
*/
public void execute() throws MojoExecutionException
{
getLog().info("Test Classpath :");
// no need to add classes/test classes directory here - they are in the classpath elements already
for (Iterator i = classpathElements.iterator(); i.hasNext();)
{
String classpathElement = (String)i.next();
getLog().info(" " + classpathElement);
}
try
{
// ClassLoader oldContextClassLoader = Thread.currentThread().getContextClassLoader();
ClassLoader testsClassLoader = ClassLoader.getSystemClassLoader();
ClassLoader tkRunnerClassLoader = createClassLoader(classpathElements, testsClassLoader, true);
Class tkRunnerClass = tkRunnerClassLoader.loadClass(TKTestRunner.class.getName());
//Object surefire = tkRunnerClass.newInstance();
Method run = tkRunnerClass.getMethod("main", new Class[] { String[].class });
Thread.currentThread().setContextClassLoader(testsClassLoader);
// Pass each of the test runner command lines in turn to the toolkit test runner.
for (String testName : commands.keySet())
{
String commandLine = commands.get(testName);
// Tokenize the command line on white space, into an array of string components.
String[] tokenizedCommandLine = commandLine.split("\\s");
// Run the tests.
run.invoke(tkRunnerClass, new Object[] { tokenizedCommandLine });
}
}
catch (Exception e)
{
getLog().error("There was an exception: " + e.getMessage(), e);
}
}
private static ClassLoader createClassLoader(List classPathUrls, ClassLoader parent, boolean childDelegation)
throws MalformedURLException
{
List urls = new ArrayList();
for (Iterator i = classPathUrls.iterator(); i.hasNext();)
{
String url = (String)i.next();
if (url != null)
{
File f = new File(url);
urls.add(f.toURL());
}
}
IsolatedClassLoader classLoader = new IsolatedClassLoader(parent, childDelegation);
for (Iterator iter = urls.iterator(); iter.hasNext();)
{
URL url = (URL)iter.next();
classLoader.addURL(url);
}
return classLoader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy