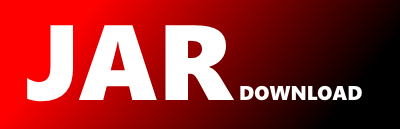
uk.emarte.regurgitator.extensions.web.HttpCallYmlLoader Maven / Gradle / Ivy
/*
* Copyright (C) 2017 Miles Talmey.
* Distributed under the MIT License (license terms are at http://opensource.org/licenses/MIT).
*/
package uk.emarte.regurgitator.extensions.web;
import uk.emarte.regurgitator.core.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import static java.lang.Integer.parseInt;
import static uk.emarte.regurgitator.core.CoreConfigConstants.STEPS;
import static uk.emarte.regurgitator.core.Log.getLog;
import static uk.emarte.regurgitator.core.YmlConfigUtil.*;
import static uk.emarte.regurgitator.extensions.web.ExtensionsWebConfigConstants.*;
public class HttpCallYmlLoader implements YmlLoader {
private static final Log log = getLog(HttpCallYmlLoader.class);
private static final YmlLoaderUtil> loaderUtil = new YmlLoaderUtil<>();
@Override
public Step load(Yaml yaml, Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy