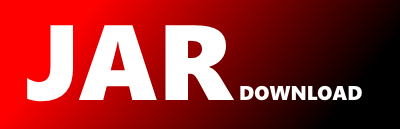
uk.gov.gchq.gaffer.commonutil.CollectionUtil Maven / Gradle / Ivy
/*
* Copyright 2016-2019 Crown Copyright
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package uk.gov.gchq.gaffer.commonutil;
import uk.gov.gchq.koryphe.serialisation.json.SimpleClassNameIdResolver;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public final class CollectionUtil {
private CollectionUtil() {
// Private constructor to prevent instantiation.
}
public static Iterable[] toIterableArray(final Collection extends Iterable> collection) {
if (null == collection) {
return null;
}
return collection.toArray(new Iterable[collection.size()]);
}
public static TreeSet treeSet(final T item) {
final TreeSet treeSet = new TreeSet<>();
if (null != item) {
treeSet.add(item);
}
return treeSet;
}
public static TreeSet treeSet(final T[] items) {
final TreeSet treeSet = new TreeSet<>();
if (null != items) {
for (final T item : items) {
if (null != item) {
treeSet.add(item);
}
}
}
return treeSet;
}
public static void toMapWithClassKeys(final Map mapAsStrings, final Map, V> map) throws ClassNotFoundException {
for (final Map.Entry entry : mapAsStrings.entrySet()) {
map.put(
(Class) Class.forName(SimpleClassNameIdResolver.getClassName(entry.getKey())),
entry.getValue()
);
}
}
public static Map, V> toMapWithClassKeys(final Map mapAsStrings) throws ClassNotFoundException {
final Map, V> map = new HashMap<>(mapAsStrings.size());
toMapWithClassKeys(mapAsStrings, map);
return map;
}
public static void toMapWithStringKeys(final Map, V> map, final Map mapAsStrings) {
for (final Map.Entry, V> entry : map.entrySet()) {
mapAsStrings.put(
entry.getKey().getName(),
entry.getValue()
);
}
}
public static Map toMapWithStringKeys(final Map, V> map) {
final Map mapAsStrings = new HashMap<>();
toMapWithStringKeys(map, mapAsStrings);
return mapAsStrings;
}
public static boolean containsAny(final Collection collection, final Object[] objects) {
boolean result = false;
if (null != collection && null != objects) {
for (final Object object : objects) {
if (collection.contains(object)) {
result = true;
break;
}
}
}
return result;
}
public static boolean anyMissing(final Collection collection, final Object[] objects) {
boolean result = false;
if (null == collection || collection.isEmpty()) {
if (null != objects && 0 < objects.length) {
result = true;
}
} else if (null != objects) {
for (final Object object : objects) {
if (!collection.contains(object)) {
result = true;
break;
}
}
}
return result;
}
/**
* Determine whether all of the items in the given {@link Collection} are unique.
*
* @param collection the collection to check
* @param the type of object contained in the collection
* @return {@code true} if all the items in the Collection are unique (as determined
* by {@link Object#equals(Object)}, otherwise {@code false}
*/
public static boolean distinct(final Collection collection) {
final Set set = new HashSet<>();
for (final T t : collection) {
if (!set.add(t)) {
return false;
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy