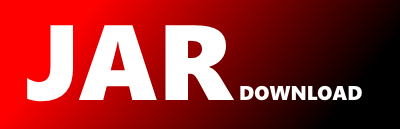
uk.gov.gchq.gaffer.commonutil.stream.GafferCollectors Maven / Gradle / Ivy
/*
* Copyright 2017-2019 Crown Copyright
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package uk.gov.gchq.gaffer.commonutil.stream;
import uk.gov.gchq.gaffer.commonutil.iterable.LimitedInMemorySortedIterable;
import java.util.Comparator;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
/**
* Java 8 {@link java.util.stream.Collector}s for Gaffer, based on the {@link java.util.stream.Collectors}
* class.
*
* Please note that using a {@link java.util.stream.Collector} to gather together
* the items contained in a {@link java.util.stream.Stream} will result in those
* items being loaded into memory.
*/
public final class GafferCollectors {
private GafferCollectors() {
// Empty
}
/**
* Returns a {@link java.util.stream.Collector} that accumulates the input
* items into a {@link java.util.LinkedHashSet}.
*
* @param the type of the input items
* @return a {@link java.util.stream.Collector} which collects all the input
* elements into a {@link java.util.LinkedHashSet}
*/
public static Collector> toLinkedHashSet() {
return new GafferCollectorImpl<>(
(Supplier>) LinkedHashSet::new,
Set::add,
(left, right) -> {
left.addAll(right);
return left;
},
set -> set
);
}
/**
*
* Returns a {@link java.util.stream.Collector} that accumulates the input
* items into a {@link LimitedInMemorySortedIterable}.
*
*
* The usage of a {@link LimitedInMemorySortedIterable}
* ensures that only relevant results are stored in memory, as the output is
* built up incrementally.
*
*
* @param comparator the {@link java.util.Comparator} to use when comparing
* items
* @param limit the maximum number of items to collect
* @param deduplicate true if the results should be deduplicated based the items hashcode/equals methods
* @param the type of input items
* @return a {@link java.util.stream.Collector} which collects all the input
* elements into a {@link LimitedInMemorySortedIterable}
*/
public static Collector, LimitedInMemorySortedIterable> toLimitedInMemorySortedIterable(final Comparator comparator, final Integer limit, final boolean deduplicate) {
return new GafferCollectorImpl<>(
() -> new LimitedInMemorySortedIterable<>(comparator, limit, deduplicate),
LimitedInMemorySortedIterable::add,
(left, right) -> {
left.addAll(right);
return left;
}
);
}
/**
* Simple implementation class for {@code GafferCollector}.
*
* @param the type of elements to be collected
* @param the type of the result
*/
static class GafferCollectorImpl implements Collector {
private final Supplier supplier;
private final BiConsumer accumulator;
private final BinaryOperator combiner;
private final Function finisher;
GafferCollectorImpl(final Supplier supplier,
final BiConsumer accumulator,
final BinaryOperator combiner,
final Function finisher) {
this.supplier = supplier;
this.accumulator = accumulator;
this.combiner = combiner;
this.finisher = finisher;
}
GafferCollectorImpl(final Supplier supplier,
final BiConsumer accumulator,
final BinaryOperator combiner) {
this(supplier, accumulator, combiner, i -> (R) i);
}
@Override
public BiConsumer accumulator() {
return accumulator;
}
@Override
public Supplier supplier() {
return supplier;
}
@Override
public BinaryOperator combiner() {
return combiner;
}
@Override
public Function finisher() {
return finisher;
}
@Override
public Set characteristics() {
return new HashSet<>();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy