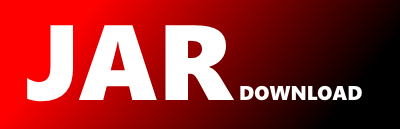
uk.gov.ida.saml.hub.domain.AuthnRequestFromRelyingParty Maven / Gradle / Ivy
package uk.gov.ida.saml.hub.domain;
import org.joda.time.DateTime;
import org.opensaml.xmlsec.signature.Signature;
import java.net.URI;
import java.util.Optional;
public class AuthnRequestFromRelyingParty extends VerifySamlMessage {
private Optional forceAuthentication;
private Optional assertionConsumerServiceUrl;
private Optional assertionConsumerServiceIndex;
private Optional signature;
private Optional verifyServiceProviderVersion;
protected AuthnRequestFromRelyingParty() {
}
public AuthnRequestFromRelyingParty(
String id,
String issuer,
DateTime issueInstant,
URI destination,
Optional forceAuthentication,
Optional assertionConsumerServiceUrl,
Optional assertionConsumerServiceIndex,
Optional signature,
Optional verifyServiceProviderVersion
) {
super(id, issuer, issueInstant, destination);
this.forceAuthentication = forceAuthentication;
this.assertionConsumerServiceUrl = assertionConsumerServiceUrl;
this.assertionConsumerServiceIndex = assertionConsumerServiceIndex;
this.signature = signature;
this.verifyServiceProviderVersion = verifyServiceProviderVersion;
}
public Optional getForceAuthentication() {
return forceAuthentication;
}
public Optional getAssertionConsumerServiceIndex() {
return assertionConsumerServiceIndex;
}
public Optional getSignature() {
return signature;
}
public Optional getAssertionConsumerServiceUrl() {
return assertionConsumerServiceUrl;
}
public Optional getVerifyServiceProviderVersion() {
return verifyServiceProviderVersion;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof AuthnRequestFromRelyingParty)) return false;
AuthnRequestFromRelyingParty that = (AuthnRequestFromRelyingParty) o;
if (forceAuthentication != null ? !forceAuthentication.equals(that.forceAuthentication) : that.forceAuthentication != null)
return false;
if (assertionConsumerServiceUrl != null ? !assertionConsumerServiceUrl.equals(that.assertionConsumerServiceUrl) : that.assertionConsumerServiceUrl != null)
return false;
if (assertionConsumerServiceIndex != null ? !assertionConsumerServiceIndex.equals(that.assertionConsumerServiceIndex) : that.assertionConsumerServiceIndex != null)
return false;
if (signature != null ? !signature.equals(that.signature) : that.signature != null) return false;
return verifyServiceProviderVersion != null ? verifyServiceProviderVersion.equals(that.verifyServiceProviderVersion) : that.verifyServiceProviderVersion == null;
}
@Override
public int hashCode() {
int result = forceAuthentication != null ? forceAuthentication.hashCode() : 0;
result = 31 * result + (assertionConsumerServiceUrl != null ? assertionConsumerServiceUrl.hashCode() : 0);
result = 31 * result + (assertionConsumerServiceIndex != null ? assertionConsumerServiceIndex.hashCode() : 0);
result = 31 * result + (signature != null ? signature.hashCode() : 0);
result = 31 * result + (verifyServiceProviderVersion != null ? verifyServiceProviderVersion.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "AuthnRequestFromRelyingParty{" +
"forceAuthentication=" + forceAuthentication +
", assertionConsumerServiceUrl=" + assertionConsumerServiceUrl +
", assertionConsumerServiceIndex=" + assertionConsumerServiceIndex +
", signature=" + signature +
", verifyServiceProviderVersion=" + verifyServiceProviderVersion +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy