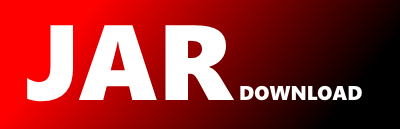
uk.gov.nationalarchives.Tables.scala Maven / Gradle / Ivy
package uk.gov.nationalarchives
// AUTO-GENERATED Slick data model
/** Stand-alone Slick data model for immediate use */
object Tables extends Tables {
val profile: slick.jdbc.JdbcProfile = slick.jdbc.PostgresProfile
}
/** Slick data model trait for extension, choice of backend or usage in the cake pattern. (Make sure to initialize this late.) */
trait Tables {
val profile: slick.jdbc.JdbcProfile
import profile.api._
import slick.model.ForeignKeyAction
// NOTE: GetResult mappers for plain SQL are only generated for
// tables where Slick knows how to map the types of all columns.
import slick.jdbc.{GetResult => GR}
/** DDL for all tables. Call .create to execute. */
lazy val schema: profile.SchemaDescription = Array(Allowedpuids.schema, Avmetadata.schema, Body.schema, Consignment.schema, Consignmentmetadata.schema, Consignmentproperty.schema, Consignmentstatus.schema, Disallowedpuids.schema, Displayproperties.schema, Ffidmetadata.schema, Ffidmetadatamatches.schema, File.schema, Filemetadata.schema, Fileproperty.schema, Filepropertydependencies.schema, Filepropertyvalues.schema, Filestatus.schema, FlywaySchemaHistory.schema, Series.schema).reduceLeft(_ ++ _)
/** Entity class storing rows of table Allowedpuids
* @param puid Database column PUID SqlType(text)
* @param `puid description` Database column PUID Description SqlType(text)
* @param `created date` Database column Created Date SqlType(timestamptz)
* @param `modified date` Database column Modified Date SqlType(timestamptz), Default(None)
* @param consignmenttype Database column ConsignmentType SqlType(text) */
case class AllowedpuidsRow(puid: String, `puid description`: String, `created date`: java.sql.Timestamp, `modified date`: Option[java.sql.Timestamp] = None, consignmenttype: String)
/** GetResult implicit for fetching AllowedpuidsRow objects using plain SQL queries */
implicit def GetResultAllowedpuidsRow(implicit e0: GR[String], e1: GR[java.sql.Timestamp], e2: GR[Option[java.sql.Timestamp]]): GR[AllowedpuidsRow] = GR{
prs => import prs._
(AllowedpuidsRow.apply _).tupled((<<[String], <<[String], <<[java.sql.Timestamp], <[java.sql.Timestamp], <<[String]))
}
/** Table description of table AllowedPuids. Objects of this class serve as prototypes for rows in queries. */
class Allowedpuids(_tableTag: Tag) extends profile.api.Table[AllowedpuidsRow](_tableTag, "AllowedPuids") {
def * = ((puid, `puid description`, `created date`, `modified date`, consignmenttype)).mapTo[AllowedpuidsRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(puid), Rep.Some(`puid description`), Rep.Some(`created date`), `modified date`, Rep.Some(consignmenttype))).shaped.<>({r=>import r._; _1.map(_=> (AllowedpuidsRow.apply _).tupled((_1.get, _2.get, _3.get, _4, _5.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column PUID SqlType(text) */
val puid: Rep[String] = column[String]("PUID")
/** Database column PUID Description SqlType(text) */
val `puid description`: Rep[String] = column[String]("PUID Description")
/** Database column Created Date SqlType(timestamptz) */
val `created date`: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Created Date")
/** Database column Modified Date SqlType(timestamptz), Default(None) */
val `modified date`: Rep[Option[java.sql.Timestamp]] = column[Option[java.sql.Timestamp]]("Modified Date", O.Default(None))
/** Database column ConsignmentType SqlType(text) */
val consignmenttype: Rep[String] = column[String]("ConsignmentType")
}
/** Collection-like TableQuery object for table Allowedpuids */
lazy val Allowedpuids = new TableQuery(tag => new Allowedpuids(tag))
/** Entity class storing rows of table Avmetadata
* @param fileid Database column FileId SqlType(uuid)
* @param software Database column Software SqlType(text)
* @param softwareversion Database column SoftwareVersion SqlType(text)
* @param databaseversion Database column DatabaseVersion SqlType(text)
* @param result Database column Result SqlType(text)
* @param datetime Database column Datetime SqlType(timestamptz) */
case class AvmetadataRow(fileid: java.util.UUID, software: String, softwareversion: String, databaseversion: String, result: String, datetime: java.sql.Timestamp)
/** GetResult implicit for fetching AvmetadataRow objects using plain SQL queries */
implicit def GetResultAvmetadataRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[java.sql.Timestamp]): GR[AvmetadataRow] = GR{
prs => import prs._
(AvmetadataRow.apply _).tupled((<<[java.util.UUID], <<[String], <<[String], <<[String], <<[String], <<[java.sql.Timestamp]))
}
/** Table description of table AVMetadata. Objects of this class serve as prototypes for rows in queries. */
class Avmetadata(_tableTag: Tag) extends profile.api.Table[AvmetadataRow](_tableTag, "AVMetadata") {
def * = ((fileid, software, softwareversion, databaseversion, result, datetime)).mapTo[AvmetadataRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(fileid), Rep.Some(software), Rep.Some(softwareversion), Rep.Some(databaseversion), Rep.Some(result), Rep.Some(datetime))).shaped.<>({r=>import r._; _1.map(_=> (AvmetadataRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5.get, _6.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column FileId SqlType(uuid) */
val fileid: Rep[java.util.UUID] = column[java.util.UUID]("FileId")
/** Database column Software SqlType(text) */
val software: Rep[String] = column[String]("Software")
/** Database column SoftwareVersion SqlType(text) */
val softwareversion: Rep[String] = column[String]("SoftwareVersion")
/** Database column DatabaseVersion SqlType(text) */
val databaseversion: Rep[String] = column[String]("DatabaseVersion")
/** Database column Result SqlType(text) */
val result: Rep[String] = column[String]("Result")
/** Database column Datetime SqlType(timestamptz) */
val datetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Datetime")
/** Foreign key referencing File (database name AVMetadata_Consignment_fkey) */
lazy val fileFk = foreignKey("AVMetadata_Consignment_fkey", fileid, File)(r => r.fileid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Avmetadata */
lazy val Avmetadata = new TableQuery(tag => new Avmetadata(tag))
/** Entity class storing rows of table Body
* @param bodyid Database column BodyId SqlType(uuid), PrimaryKey
* @param name Database column Name SqlType(text)
* @param description Database column Description SqlType(text), Default(None)
* @param tdrcode Database column TdrCode SqlType(text) */
case class BodyRow(bodyid: java.util.UUID, name: String, description: Option[String] = None, tdrcode: String)
/** GetResult implicit for fetching BodyRow objects using plain SQL queries */
implicit def GetResultBodyRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[Option[String]]): GR[BodyRow] = GR{
prs => import prs._
(BodyRow.apply _).tupled((<<[java.util.UUID], <<[String], <[String], <<[String]))
}
/** Table description of table Body. Objects of this class serve as prototypes for rows in queries. */
class Body(_tableTag: Tag) extends profile.api.Table[BodyRow](_tableTag, "Body") {
def * = ((bodyid, name, description, tdrcode)).mapTo[BodyRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(bodyid), Rep.Some(name), description, Rep.Some(tdrcode))).shaped.<>({r=>import r._; _1.map(_=> (BodyRow.apply _).tupled((_1.get, _2.get, _3, _4.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column BodyId SqlType(uuid), PrimaryKey */
val bodyid: Rep[java.util.UUID] = column[java.util.UUID]("BodyId", O.PrimaryKey)
/** Database column Name SqlType(text) */
val name: Rep[String] = column[String]("Name")
/** Database column Description SqlType(text), Default(None) */
val description: Rep[Option[String]] = column[Option[String]]("Description", O.Default(None))
/** Database column TdrCode SqlType(text) */
val tdrcode: Rep[String] = column[String]("TdrCode")
}
/** Collection-like TableQuery object for table Body */
lazy val Body = new TableQuery(tag => new Body(tag))
/** Entity class storing rows of table Consignment
* @param consignmentid Database column ConsignmentId SqlType(uuid), PrimaryKey
* @param seriesid Database column SeriesId SqlType(uuid), Default(None)
* @param userid Database column UserId SqlType(uuid)
* @param datetime Database column Datetime SqlType(timestamptz)
* @param parentfolder Database column ParentFolder SqlType(text), Default(None)
* @param transferinitiateddatetime Database column TransferInitiatedDatetime SqlType(timestamptz), Default(None)
* @param transferinitiatedby Database column TransferInitiatedBy SqlType(uuid), Default(None)
* @param exportdatetime Database column ExportDatetime SqlType(timestamptz), Default(None)
* @param exportlocation Database column ExportLocation SqlType(text), Default(None)
* @param consignmentsequence Database column ConsignmentSequence SqlType(int8)
* @param consignmentreference Database column ConsignmentReference SqlType(text)
* @param consignmenttype Database column ConsignmentType SqlType(text)
* @param bodyid Database column BodyId SqlType(uuid)
* @param exportversion Database column ExportVersion SqlType(text), Default(None)
* @param includetoplevelfolder Database column IncludeTopLevelFolder SqlType(bool), Default(None)
* @param seriesname Database column SeriesName SqlType(text), Default(None)
* @param transferringbodyname Database column TransferringBodyName SqlType(text), Default(None)
* @param transferringbodytdrcode Database column TransferringBodyTdrCode SqlType(text), Default(None) */
case class ConsignmentRow(consignmentid: java.util.UUID, seriesid: Option[java.util.UUID] = None, userid: java.util.UUID, datetime: java.sql.Timestamp, parentfolder: Option[String] = None, transferinitiateddatetime: Option[java.sql.Timestamp] = None, transferinitiatedby: Option[java.util.UUID] = None, exportdatetime: Option[java.sql.Timestamp] = None, exportlocation: Option[String] = None, consignmentsequence: Long, consignmentreference: String, consignmenttype: String, bodyid: java.util.UUID, exportversion: Option[String] = None, includetoplevelfolder: Option[Boolean] = None, seriesname: Option[String] = None, transferringbodyname: Option[String] = None, transferringbodytdrcode: Option[String] = None)
/** GetResult implicit for fetching ConsignmentRow objects using plain SQL queries */
implicit def GetResultConsignmentRow(implicit e0: GR[java.util.UUID], e1: GR[Option[java.util.UUID]], e2: GR[java.sql.Timestamp], e3: GR[Option[String]], e4: GR[Option[java.sql.Timestamp]], e5: GR[Long], e6: GR[String], e7: GR[Option[Boolean]]): GR[ConsignmentRow] = GR{
prs => import prs._
(ConsignmentRow.apply _).tupled((<<[java.util.UUID], <[java.util.UUID], <<[java.util.UUID], <<[java.sql.Timestamp], <[String], <[java.sql.Timestamp], <[java.util.UUID], <[java.sql.Timestamp], <[String], <<[Long], <<[String], <<[String], <<[java.util.UUID], <[String], <[Boolean], <[String], <[String], <[String]))
}
/** Table description of table Consignment. Objects of this class serve as prototypes for rows in queries. */
class Consignment(_tableTag: Tag) extends profile.api.Table[ConsignmentRow](_tableTag, "Consignment") {
def * = ((consignmentid, seriesid, userid, datetime, parentfolder, transferinitiateddatetime, transferinitiatedby, exportdatetime, exportlocation, consignmentsequence, consignmentreference, consignmenttype, bodyid, exportversion, includetoplevelfolder, seriesname, transferringbodyname, transferringbodytdrcode)).mapTo[ConsignmentRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(consignmentid), seriesid, Rep.Some(userid), Rep.Some(datetime), parentfolder, transferinitiateddatetime, transferinitiatedby, exportdatetime, exportlocation, Rep.Some(consignmentsequence), Rep.Some(consignmentreference), Rep.Some(consignmenttype), Rep.Some(bodyid), exportversion, includetoplevelfolder, seriesname, transferringbodyname, transferringbodytdrcode)).shaped.<>({r=>import r._; _1.map(_=> (ConsignmentRow.apply _).tupled((_1.get, _2, _3.get, _4.get, _5, _6, _7, _8, _9, _10.get, _11.get, _12.get, _13.get, _14, _15, _16, _17, _18)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column ConsignmentId SqlType(uuid), PrimaryKey */
val consignmentid: Rep[java.util.UUID] = column[java.util.UUID]("ConsignmentId", O.PrimaryKey)
/** Database column SeriesId SqlType(uuid), Default(None) */
val seriesid: Rep[Option[java.util.UUID]] = column[Option[java.util.UUID]]("SeriesId", O.Default(None))
/** Database column UserId SqlType(uuid) */
val userid: Rep[java.util.UUID] = column[java.util.UUID]("UserId")
/** Database column Datetime SqlType(timestamptz) */
val datetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Datetime")
/** Database column ParentFolder SqlType(text), Default(None) */
val parentfolder: Rep[Option[String]] = column[Option[String]]("ParentFolder", O.Default(None))
/** Database column TransferInitiatedDatetime SqlType(timestamptz), Default(None) */
val transferinitiateddatetime: Rep[Option[java.sql.Timestamp]] = column[Option[java.sql.Timestamp]]("TransferInitiatedDatetime", O.Default(None))
/** Database column TransferInitiatedBy SqlType(uuid), Default(None) */
val transferinitiatedby: Rep[Option[java.util.UUID]] = column[Option[java.util.UUID]]("TransferInitiatedBy", O.Default(None))
/** Database column ExportDatetime SqlType(timestamptz), Default(None) */
val exportdatetime: Rep[Option[java.sql.Timestamp]] = column[Option[java.sql.Timestamp]]("ExportDatetime", O.Default(None))
/** Database column ExportLocation SqlType(text), Default(None) */
val exportlocation: Rep[Option[String]] = column[Option[String]]("ExportLocation", O.Default(None))
/** Database column ConsignmentSequence SqlType(int8) */
val consignmentsequence: Rep[Long] = column[Long]("ConsignmentSequence")
/** Database column ConsignmentReference SqlType(text) */
val consignmentreference: Rep[String] = column[String]("ConsignmentReference")
/** Database column ConsignmentType SqlType(text) */
val consignmenttype: Rep[String] = column[String]("ConsignmentType")
/** Database column BodyId SqlType(uuid) */
val bodyid: Rep[java.util.UUID] = column[java.util.UUID]("BodyId")
/** Database column ExportVersion SqlType(text), Default(None) */
val exportversion: Rep[Option[String]] = column[Option[String]]("ExportVersion", O.Default(None))
/** Database column IncludeTopLevelFolder SqlType(bool), Default(None) */
val includetoplevelfolder: Rep[Option[Boolean]] = column[Option[Boolean]]("IncludeTopLevelFolder", O.Default(None))
/** Database column SeriesName SqlType(text), Default(None) */
val seriesname: Rep[Option[String]] = column[Option[String]]("SeriesName", O.Default(None))
/** Database column TransferringBodyName SqlType(text), Default(None) */
val transferringbodyname: Rep[Option[String]] = column[Option[String]]("TransferringBodyName", O.Default(None))
/** Database column TransferringBodyTdrCode SqlType(text), Default(None) */
val transferringbodytdrcode: Rep[Option[String]] = column[Option[String]]("TransferringBodyTdrCode", O.Default(None))
/** Foreign key referencing Body (database name Consignment_Body_fkey) */
lazy val bodyFk = foreignKey("Consignment_Body_fkey", bodyid, Body)(r => r.bodyid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Foreign key referencing Series (database name Consignment_Series_fkey) */
lazy val seriesFk = foreignKey("Consignment_Series_fkey", seriesid, Series)(r => Rep.Some(r.seriesid), onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Uniqueness Index over (consignmentreference) (database name UniqueConsignmentReference) */
val index1 = index("UniqueConsignmentReference", consignmentreference, unique=true)
/** Uniqueness Index over (consignmentsequence) (database name UniqueConsignmentSequence) */
val index2 = index("UniqueConsignmentSequence", consignmentsequence, unique=true)
/** Index over (consignmentid,consignmentreference) (database name consignment_consignmentid_consignmentreference_idx) */
val index3 = index("consignment_consignmentid_consignmentreference_idx", (consignmentid, consignmentreference))
}
/** Collection-like TableQuery object for table Consignment */
lazy val Consignment = new TableQuery(tag => new Consignment(tag))
/** Entity class storing rows of table Consignmentmetadata
* @param metadataid Database column MetadataId SqlType(uuid), PrimaryKey
* @param consignmentid Database column ConsignmentId SqlType(uuid)
* @param propertyname Database column PropertyName SqlType(text)
* @param value Database column Value SqlType(text)
* @param datetime Database column Datetime SqlType(timestamptz)
* @param userid Database column UserId SqlType(uuid) */
case class ConsignmentmetadataRow(metadataid: java.util.UUID, consignmentid: java.util.UUID, propertyname: String, value: String, datetime: java.sql.Timestamp, userid: java.util.UUID)
/** GetResult implicit for fetching ConsignmentmetadataRow objects using plain SQL queries */
implicit def GetResultConsignmentmetadataRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[java.sql.Timestamp]): GR[ConsignmentmetadataRow] = GR{
prs => import prs._
(ConsignmentmetadataRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[String], <<[String], <<[java.sql.Timestamp], <<[java.util.UUID]))
}
/** Table description of table ConsignmentMetadata. Objects of this class serve as prototypes for rows in queries. */
class Consignmentmetadata(_tableTag: Tag) extends profile.api.Table[ConsignmentmetadataRow](_tableTag, "ConsignmentMetadata") {
def * = ((metadataid, consignmentid, propertyname, value, datetime, userid)).mapTo[ConsignmentmetadataRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(metadataid), Rep.Some(consignmentid), Rep.Some(propertyname), Rep.Some(value), Rep.Some(datetime), Rep.Some(userid))).shaped.<>({r=>import r._; _1.map(_=> (ConsignmentmetadataRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5.get, _6.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column MetadataId SqlType(uuid), PrimaryKey */
val metadataid: Rep[java.util.UUID] = column[java.util.UUID]("MetadataId", O.PrimaryKey)
/** Database column ConsignmentId SqlType(uuid) */
val consignmentid: Rep[java.util.UUID] = column[java.util.UUID]("ConsignmentId")
/** Database column PropertyName SqlType(text) */
val propertyname: Rep[String] = column[String]("PropertyName")
/** Database column Value SqlType(text) */
val value: Rep[String] = column[String]("Value")
/** Database column Datetime SqlType(timestamptz) */
val datetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Datetime")
/** Database column UserId SqlType(uuid) */
val userid: Rep[java.util.UUID] = column[java.util.UUID]("UserId")
/** Foreign key referencing Consignment (database name ConMetadata_Consignment_fkey) */
lazy val consignmentFk = foreignKey("ConMetadata_Consignment_fkey", consignmentid, Consignment)(r => r.consignmentid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Foreign key referencing Consignmentproperty (database name ConMetadata_PropertyName_fkey) */
lazy val consignmentpropertyFk = foreignKey("ConMetadata_PropertyName_fkey", propertyname, Consignmentproperty)(r => r.name, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Consignmentmetadata */
lazy val Consignmentmetadata = new TableQuery(tag => new Consignmentmetadata(tag))
/** Entity class storing rows of table Consignmentproperty
* @param name Database column Name SqlType(text), PrimaryKey
* @param description Database column Description SqlType(text), Default(None) */
case class ConsignmentpropertyRow(name: String, description: Option[String] = None)
/** GetResult implicit for fetching ConsignmentpropertyRow objects using plain SQL queries */
implicit def GetResultConsignmentpropertyRow(implicit e0: GR[String], e1: GR[Option[String]]): GR[ConsignmentpropertyRow] = GR{
prs => import prs._
(ConsignmentpropertyRow.apply _).tupled((<<[String], <[String]))
}
/** Table description of table ConsignmentProperty. Objects of this class serve as prototypes for rows in queries. */
class Consignmentproperty(_tableTag: Tag) extends profile.api.Table[ConsignmentpropertyRow](_tableTag, "ConsignmentProperty") {
def * = ((name, description)).mapTo[ConsignmentpropertyRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(name), description)).shaped.<>({r=>import r._; _1.map(_=> (ConsignmentpropertyRow.apply _).tupled((_1.get, _2)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column Name SqlType(text), PrimaryKey */
val name: Rep[String] = column[String]("Name", O.PrimaryKey)
/** Database column Description SqlType(text), Default(None) */
val description: Rep[Option[String]] = column[Option[String]]("Description", O.Default(None))
}
/** Collection-like TableQuery object for table Consignmentproperty */
lazy val Consignmentproperty = new TableQuery(tag => new Consignmentproperty(tag))
/** Entity class storing rows of table Consignmentstatus
* @param consignmentstatusid Database column ConsignmentStatusId SqlType(uuid), PrimaryKey
* @param consignmentid Database column ConsignmentId SqlType(uuid)
* @param statustype Database column StatusType SqlType(text)
* @param value Database column Value SqlType(text)
* @param createddatetime Database column CreatedDatetime SqlType(timestamptz)
* @param modifieddatetime Database column ModifiedDatetime SqlType(timestamptz), Default(None) */
case class ConsignmentstatusRow(consignmentstatusid: java.util.UUID, consignmentid: java.util.UUID, statustype: String, value: String, createddatetime: java.sql.Timestamp, modifieddatetime: Option[java.sql.Timestamp] = None)
/** GetResult implicit for fetching ConsignmentstatusRow objects using plain SQL queries */
implicit def GetResultConsignmentstatusRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[java.sql.Timestamp], e3: GR[Option[java.sql.Timestamp]]): GR[ConsignmentstatusRow] = GR{
prs => import prs._
(ConsignmentstatusRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[String], <<[String], <<[java.sql.Timestamp], <[java.sql.Timestamp]))
}
/** Table description of table ConsignmentStatus. Objects of this class serve as prototypes for rows in queries. */
class Consignmentstatus(_tableTag: Tag) extends profile.api.Table[ConsignmentstatusRow](_tableTag, "ConsignmentStatus") {
def * = ((consignmentstatusid, consignmentid, statustype, value, createddatetime, modifieddatetime)).mapTo[ConsignmentstatusRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(consignmentstatusid), Rep.Some(consignmentid), Rep.Some(statustype), Rep.Some(value), Rep.Some(createddatetime), modifieddatetime)).shaped.<>({r=>import r._; _1.map(_=> (ConsignmentstatusRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5.get, _6)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column ConsignmentStatusId SqlType(uuid), PrimaryKey */
val consignmentstatusid: Rep[java.util.UUID] = column[java.util.UUID]("ConsignmentStatusId", O.PrimaryKey)
/** Database column ConsignmentId SqlType(uuid) */
val consignmentid: Rep[java.util.UUID] = column[java.util.UUID]("ConsignmentId")
/** Database column StatusType SqlType(text) */
val statustype: Rep[String] = column[String]("StatusType")
/** Database column Value SqlType(text) */
val value: Rep[String] = column[String]("Value")
/** Database column CreatedDatetime SqlType(timestamptz) */
val createddatetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("CreatedDatetime")
/** Database column ModifiedDatetime SqlType(timestamptz), Default(None) */
val modifieddatetime: Rep[Option[java.sql.Timestamp]] = column[Option[java.sql.Timestamp]]("ModifiedDatetime", O.Default(None))
/** Foreign key referencing Consignment (database name ConsignmentStatus_Consignment_fkey) */
lazy val consignmentFk = foreignKey("ConsignmentStatus_Consignment_fkey", consignmentid, Consignment)(r => r.consignmentid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Uniqueness Index over (consignmentid,statustype) (database name consignment_status) */
val index1 = index("consignment_status", (consignmentid, statustype), unique=true)
}
/** Collection-like TableQuery object for table Consignmentstatus */
lazy val Consignmentstatus = new TableQuery(tag => new Consignmentstatus(tag))
/** Entity class storing rows of table Disallowedpuids
* @param puid Database column PUID SqlType(text)
* @param `puid description` Database column PUID Description SqlType(text)
* @param `created date` Database column Created Date SqlType(timestamptz)
* @param `modified date` Database column Modified Date SqlType(timestamptz), Default(None)
* @param reason Database column Reason SqlType(text)
* @param active Database column Active SqlType(bool), Default(true) */
case class DisallowedpuidsRow(puid: String, `puid description`: String, `created date`: java.sql.Timestamp, `modified date`: Option[java.sql.Timestamp] = None, reason: String, active: Boolean = true)
/** GetResult implicit for fetching DisallowedpuidsRow objects using plain SQL queries */
implicit def GetResultDisallowedpuidsRow(implicit e0: GR[String], e1: GR[java.sql.Timestamp], e2: GR[Option[java.sql.Timestamp]], e3: GR[Boolean]): GR[DisallowedpuidsRow] = GR{
prs => import prs._
(DisallowedpuidsRow.apply _).tupled((<<[String], <<[String], <<[java.sql.Timestamp], <[java.sql.Timestamp], <<[String], <<[Boolean]))
}
/** Table description of table DisallowedPuids. Objects of this class serve as prototypes for rows in queries. */
class Disallowedpuids(_tableTag: Tag) extends profile.api.Table[DisallowedpuidsRow](_tableTag, "DisallowedPuids") {
def * = ((puid, `puid description`, `created date`, `modified date`, reason, active)).mapTo[DisallowedpuidsRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(puid), Rep.Some(`puid description`), Rep.Some(`created date`), `modified date`, Rep.Some(reason), Rep.Some(active))).shaped.<>({r=>import r._; _1.map(_=> (DisallowedpuidsRow.apply _).tupled((_1.get, _2.get, _3.get, _4, _5.get, _6.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column PUID SqlType(text) */
val puid: Rep[String] = column[String]("PUID")
/** Database column PUID Description SqlType(text) */
val `puid description`: Rep[String] = column[String]("PUID Description")
/** Database column Created Date SqlType(timestamptz) */
val `created date`: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Created Date")
/** Database column Modified Date SqlType(timestamptz), Default(None) */
val `modified date`: Rep[Option[java.sql.Timestamp]] = column[Option[java.sql.Timestamp]]("Modified Date", O.Default(None))
/** Database column Reason SqlType(text) */
val reason: Rep[String] = column[String]("Reason")
/** Database column Active SqlType(bool), Default(true) */
val active: Rep[Boolean] = column[Boolean]("Active", O.Default(true))
}
/** Collection-like TableQuery object for table Disallowedpuids */
lazy val Disallowedpuids = new TableQuery(tag => new Disallowedpuids(tag))
/** Entity class storing rows of table Displayproperties
* @param propertyname Database column PropertyName SqlType(text), Default(None)
* @param attribute Database column Attribute SqlType(text), Default(None)
* @param value Database column Value SqlType(text), Default(None)
* @param attributetype Database column AttributeType SqlType(text), Default(None) */
case class DisplaypropertiesRow(propertyname: Option[String] = None, attribute: Option[String] = None, value: Option[String] = None, attributetype: Option[String] = None)
/** GetResult implicit for fetching DisplaypropertiesRow objects using plain SQL queries */
implicit def GetResultDisplaypropertiesRow(implicit e0: GR[Option[String]]): GR[DisplaypropertiesRow] = GR{
prs => import prs._
(DisplaypropertiesRow.apply _).tupled((<[String], <[String], <[String], <[String]))
}
/** Table description of table DisplayProperties. Objects of this class serve as prototypes for rows in queries. */
class Displayproperties(_tableTag: Tag) extends profile.api.Table[DisplaypropertiesRow](_tableTag, "DisplayProperties") {
def * = ((propertyname, attribute, value, attributetype)).mapTo[DisplaypropertiesRow]
/** Database column PropertyName SqlType(text), Default(None) */
val propertyname: Rep[Option[String]] = column[Option[String]]("PropertyName", O.Default(None))
/** Database column Attribute SqlType(text), Default(None) */
val attribute: Rep[Option[String]] = column[Option[String]]("Attribute", O.Default(None))
/** Database column Value SqlType(text), Default(None) */
val value: Rep[Option[String]] = column[Option[String]]("Value", O.Default(None))
/** Database column AttributeType SqlType(text), Default(None) */
val attributetype: Rep[Option[String]] = column[Option[String]]("AttributeType", O.Default(None))
/** Foreign key referencing Fileproperty (database name DisplayProperties_FileProperty_PropertyName_fkey) */
lazy val filepropertyFk = foreignKey("DisplayProperties_FileProperty_PropertyName_fkey", propertyname, Fileproperty)(r => Rep.Some(r.name), onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Displayproperties */
lazy val Displayproperties = new TableQuery(tag => new Displayproperties(tag))
/** Entity class storing rows of table Ffidmetadata
* @param ffidmetadataid Database column FFIDMetadataId SqlType(uuid), PrimaryKey
* @param fileid Database column FileId SqlType(uuid)
* @param software Database column Software SqlType(text)
* @param softwareversion Database column SoftwareVersion SqlType(text)
* @param datetime Database column Datetime SqlType(timestamptz)
* @param binarysignaturefileversion Database column BinarySignatureFileVersion SqlType(text)
* @param containersignaturefileversion Database column ContainerSignatureFileVersion SqlType(text)
* @param method Database column Method SqlType(text) */
case class FfidmetadataRow(ffidmetadataid: java.util.UUID, fileid: java.util.UUID, software: String, softwareversion: String, datetime: java.sql.Timestamp, binarysignaturefileversion: String, containersignaturefileversion: String, method: String)
/** GetResult implicit for fetching FfidmetadataRow objects using plain SQL queries */
implicit def GetResultFfidmetadataRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[java.sql.Timestamp]): GR[FfidmetadataRow] = GR{
prs => import prs._
(FfidmetadataRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[String], <<[String], <<[java.sql.Timestamp], <<[String], <<[String], <<[String]))
}
/** Table description of table FFIDMetadata. Objects of this class serve as prototypes for rows in queries. */
class Ffidmetadata(_tableTag: Tag) extends profile.api.Table[FfidmetadataRow](_tableTag, "FFIDMetadata") {
def * = ((ffidmetadataid, fileid, software, softwareversion, datetime, binarysignaturefileversion, containersignaturefileversion, method)).mapTo[FfidmetadataRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(ffidmetadataid), Rep.Some(fileid), Rep.Some(software), Rep.Some(softwareversion), Rep.Some(datetime), Rep.Some(binarysignaturefileversion), Rep.Some(containersignaturefileversion), Rep.Some(method))).shaped.<>({r=>import r._; _1.map(_=> (FfidmetadataRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5.get, _6.get, _7.get, _8.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column FFIDMetadataId SqlType(uuid), PrimaryKey */
val ffidmetadataid: Rep[java.util.UUID] = column[java.util.UUID]("FFIDMetadataId", O.PrimaryKey)
/** Database column FileId SqlType(uuid) */
val fileid: Rep[java.util.UUID] = column[java.util.UUID]("FileId")
/** Database column Software SqlType(text) */
val software: Rep[String] = column[String]("Software")
/** Database column SoftwareVersion SqlType(text) */
val softwareversion: Rep[String] = column[String]("SoftwareVersion")
/** Database column Datetime SqlType(timestamptz) */
val datetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Datetime")
/** Database column BinarySignatureFileVersion SqlType(text) */
val binarysignaturefileversion: Rep[String] = column[String]("BinarySignatureFileVersion")
/** Database column ContainerSignatureFileVersion SqlType(text) */
val containersignaturefileversion: Rep[String] = column[String]("ContainerSignatureFileVersion")
/** Database column Method SqlType(text) */
val method: Rep[String] = column[String]("Method")
/** Foreign key referencing File (database name FFIDMetadata_Consignment_fkey) */
lazy val fileFk = foreignKey("FFIDMetadata_Consignment_fkey", fileid, File)(r => r.fileid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Index over (fileid,ffidmetadataid) (database name ffidmetadata_fileid_ffidmetadataid_idx) */
val index1 = index("ffidmetadata_fileid_ffidmetadataid_idx", (fileid, ffidmetadataid))
}
/** Collection-like TableQuery object for table Ffidmetadata */
lazy val Ffidmetadata = new TableQuery(tag => new Ffidmetadata(tag))
/** Entity class storing rows of table Ffidmetadatamatches
* @param ffidmetadataid Database column FFIDMetadataId SqlType(uuid)
* @param extension Database column Extension SqlType(text), Default(None)
* @param identificationbasis Database column IdentificationBasis SqlType(text)
* @param puid Database column PUID SqlType(text), Default(None)
* @param extensionmismatch Database column ExtensionMismatch SqlType(bool), Default(None)
* @param formatname Database column FormatName SqlType(text), Default(None) */
case class FfidmetadatamatchesRow(ffidmetadataid: java.util.UUID, extension: Option[String] = None, identificationbasis: String, puid: Option[String] = None, extensionmismatch: Option[Boolean] = None, formatname: Option[String] = None)
/** GetResult implicit for fetching FfidmetadatamatchesRow objects using plain SQL queries */
implicit def GetResultFfidmetadatamatchesRow(implicit e0: GR[java.util.UUID], e1: GR[Option[String]], e2: GR[String], e3: GR[Option[Boolean]]): GR[FfidmetadatamatchesRow] = GR{
prs => import prs._
(FfidmetadatamatchesRow.apply _).tupled((<<[java.util.UUID], <[String], <<[String], <[String], <[Boolean], <[String]))
}
/** Table description of table FFIDMetadataMatches. Objects of this class serve as prototypes for rows in queries. */
class Ffidmetadatamatches(_tableTag: Tag) extends profile.api.Table[FfidmetadatamatchesRow](_tableTag, "FFIDMetadataMatches") {
def * = ((ffidmetadataid, extension, identificationbasis, puid, extensionmismatch, formatname)).mapTo[FfidmetadatamatchesRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(ffidmetadataid), extension, Rep.Some(identificationbasis), puid, extensionmismatch, formatname)).shaped.<>({r=>import r._; _1.map(_=> (FfidmetadatamatchesRow.apply _).tupled((_1.get, _2, _3.get, _4, _5, _6)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column FFIDMetadataId SqlType(uuid) */
val ffidmetadataid: Rep[java.util.UUID] = column[java.util.UUID]("FFIDMetadataId")
/** Database column Extension SqlType(text), Default(None) */
val extension: Rep[Option[String]] = column[Option[String]]("Extension", O.Default(None))
/** Database column IdentificationBasis SqlType(text) */
val identificationbasis: Rep[String] = column[String]("IdentificationBasis")
/** Database column PUID SqlType(text), Default(None) */
val puid: Rep[Option[String]] = column[Option[String]]("PUID", O.Default(None))
/** Database column ExtensionMismatch SqlType(bool), Default(None) */
val extensionmismatch: Rep[Option[Boolean]] = column[Option[Boolean]]("ExtensionMismatch", O.Default(None))
/** Database column FormatName SqlType(text), Default(None) */
val formatname: Rep[Option[String]] = column[Option[String]]("FormatName", O.Default(None))
/** Foreign key referencing Ffidmetadata (database name FFIDMetadataMatches_Metadata_fkey) */
lazy val ffidmetadataFk = foreignKey("FFIDMetadataMatches_Metadata_fkey", ffidmetadataid, Ffidmetadata)(r => r.ffidmetadataid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Ffidmetadatamatches */
lazy val Ffidmetadatamatches = new TableQuery(tag => new Ffidmetadatamatches(tag))
/** Entity class storing rows of table File
* @param fileid Database column FileId SqlType(uuid), PrimaryKey
* @param consignmentid Database column ConsignmentId SqlType(uuid)
* @param userid Database column UserId SqlType(uuid)
* @param datetime Database column Datetime SqlType(timestamptz)
* @param checksummatches Database column ChecksumMatches SqlType(bool), Default(None)
* @param filetype Database column FileType SqlType(text), Default(None)
* @param filename Database column FileName SqlType(text), Default(None)
* @param parentid Database column ParentId SqlType(uuid), Default(None)
* @param filereference Database column FileReference SqlType(text), Default(None)
* @param parentreference Database column ParentReference SqlType(text), Default(None) */
case class FileRow(fileid: java.util.UUID, consignmentid: java.util.UUID, userid: java.util.UUID, datetime: java.sql.Timestamp, checksummatches: Option[Boolean] = None, filetype: Option[String] = None, filename: Option[String] = None, parentid: Option[java.util.UUID] = None, filereference: Option[String] = None, parentreference: Option[String] = None)
/** GetResult implicit for fetching FileRow objects using plain SQL queries */
implicit def GetResultFileRow(implicit e0: GR[java.util.UUID], e1: GR[java.sql.Timestamp], e2: GR[Option[Boolean]], e3: GR[Option[String]], e4: GR[Option[java.util.UUID]]): GR[FileRow] = GR{
prs => import prs._
(FileRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[java.util.UUID], <<[java.sql.Timestamp], <[Boolean], <[String], <[String], <[java.util.UUID], <[String], <[String]))
}
/** Table description of table File. Objects of this class serve as prototypes for rows in queries. */
class File(_tableTag: Tag) extends profile.api.Table[FileRow](_tableTag, "File") {
def * = ((fileid, consignmentid, userid, datetime, checksummatches, filetype, filename, parentid, filereference, parentreference)).mapTo[FileRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(fileid), Rep.Some(consignmentid), Rep.Some(userid), Rep.Some(datetime), checksummatches, filetype, filename, parentid, filereference, parentreference)).shaped.<>({r=>import r._; _1.map(_=> (FileRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5, _6, _7, _8, _9, _10)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column FileId SqlType(uuid), PrimaryKey */
val fileid: Rep[java.util.UUID] = column[java.util.UUID]("FileId", O.PrimaryKey)
/** Database column ConsignmentId SqlType(uuid) */
val consignmentid: Rep[java.util.UUID] = column[java.util.UUID]("ConsignmentId")
/** Database column UserId SqlType(uuid) */
val userid: Rep[java.util.UUID] = column[java.util.UUID]("UserId")
/** Database column Datetime SqlType(timestamptz) */
val datetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Datetime")
/** Database column ChecksumMatches SqlType(bool), Default(None) */
val checksummatches: Rep[Option[Boolean]] = column[Option[Boolean]]("ChecksumMatches", O.Default(None))
/** Database column FileType SqlType(text), Default(None) */
val filetype: Rep[Option[String]] = column[Option[String]]("FileType", O.Default(None))
/** Database column FileName SqlType(text), Default(None) */
val filename: Rep[Option[String]] = column[Option[String]]("FileName", O.Default(None))
/** Database column ParentId SqlType(uuid), Default(None) */
val parentid: Rep[Option[java.util.UUID]] = column[Option[java.util.UUID]]("ParentId", O.Default(None))
/** Database column FileReference SqlType(text), Default(None) */
val filereference: Rep[Option[String]] = column[Option[String]]("FileReference", O.Default(None))
/** Database column ParentReference SqlType(text), Default(None) */
val parentreference: Rep[Option[String]] = column[Option[String]]("ParentReference", O.Default(None))
/** Foreign key referencing Consignment (database name File_Consignment_fkey) */
lazy val consignmentFk = foreignKey("File_Consignment_fkey", consignmentid, Consignment)(r => r.consignmentid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Uniqueness Index over (filereference) (database name FileReference_unique) */
val index1 = index("FileReference_unique", filereference, unique=true)
/** Index over (consignmentid,fileid,filetype,filename,parentid,userid,datetime,checksummatches) (database name file_allfields_idx) */
val index2 = index("file_allfields_idx", (consignmentid, fileid, filetype, filename, parentid, userid, datetime, checksummatches))
/** Index over (consignmentid,fileid) (database name file_consignmentid_fileid_index) */
val index3 = index("file_consignmentid_fileid_index", (consignmentid, fileid))
/** Index over (consignmentid,filetype,fileid) (database name file_consignmentid_filetype_fileid_idx) */
val index4 = index("file_consignmentid_filetype_fileid_idx", (consignmentid, filetype, fileid))
}
/** Collection-like TableQuery object for table File */
lazy val File = new TableQuery(tag => new File(tag))
/** Entity class storing rows of table Filemetadata
* @param metadataid Database column MetadataId SqlType(uuid), PrimaryKey
* @param fileid Database column FileId SqlType(uuid)
* @param value Database column Value SqlType(text)
* @param datetime Database column Datetime SqlType(timestamptz)
* @param userid Database column UserId SqlType(uuid)
* @param propertyname Database column PropertyName SqlType(text) */
case class FilemetadataRow(metadataid: java.util.UUID, fileid: java.util.UUID, value: String, datetime: java.sql.Timestamp, userid: java.util.UUID, propertyname: String)
/** GetResult implicit for fetching FilemetadataRow objects using plain SQL queries */
implicit def GetResultFilemetadataRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[java.sql.Timestamp]): GR[FilemetadataRow] = GR{
prs => import prs._
(FilemetadataRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[String], <<[java.sql.Timestamp], <<[java.util.UUID], <<[String]))
}
/** Table description of table FileMetadata. Objects of this class serve as prototypes for rows in queries. */
class Filemetadata(_tableTag: Tag) extends profile.api.Table[FilemetadataRow](_tableTag, "FileMetadata") {
def * = ((metadataid, fileid, value, datetime, userid, propertyname)).mapTo[FilemetadataRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(metadataid), Rep.Some(fileid), Rep.Some(value), Rep.Some(datetime), Rep.Some(userid), Rep.Some(propertyname))).shaped.<>({r=>import r._; _1.map(_=> (FilemetadataRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5.get, _6.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column MetadataId SqlType(uuid), PrimaryKey */
val metadataid: Rep[java.util.UUID] = column[java.util.UUID]("MetadataId", O.PrimaryKey)
/** Database column FileId SqlType(uuid) */
val fileid: Rep[java.util.UUID] = column[java.util.UUID]("FileId")
/** Database column Value SqlType(text) */
val value: Rep[String] = column[String]("Value")
/** Database column Datetime SqlType(timestamptz) */
val datetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("Datetime")
/** Database column UserId SqlType(uuid) */
val userid: Rep[java.util.UUID] = column[java.util.UUID]("UserId")
/** Database column PropertyName SqlType(text) */
val propertyname: Rep[String] = column[String]("PropertyName")
/** Foreign key referencing File (database name FileMetadata_File_FileId_fkey) */
lazy val fileFk = foreignKey("FileMetadata_File_FileId_fkey", fileid, File)(r => r.fileid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Foreign key referencing Fileproperty (database name FileMetadata_FileProperty_PropertyName_fkey) */
lazy val filepropertyFk = foreignKey("FileMetadata_FileProperty_PropertyName_fkey", propertyname, Fileproperty)(r => r.name, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Index over (fileid,propertyname,value,metadataid,datetime,userid) (database name filemetadata_allfields_idx) */
val index1 = index("filemetadata_allfields_idx", (fileid, propertyname, value, metadataid, datetime, userid))
/** Index over (fileid,propertyname) (database name filemetadata_fileid_propertyname_idx) */
val index2 = index("filemetadata_fileid_propertyname_idx", (fileid, propertyname))
}
/** Collection-like TableQuery object for table Filemetadata */
lazy val Filemetadata = new TableQuery(tag => new Filemetadata(tag))
/** Entity class storing rows of table Fileproperty
* @param name Database column Name SqlType(text), PrimaryKey
* @param description Database column Description SqlType(text), Default(None)
* @param fullname Database column FullName SqlType(text), Default(None)
* @param createddatetime Database column CreatedDatetime SqlType(timestamptz)
* @param modifieddatetime Database column ModifiedDatetime SqlType(timestamptz), Default(None)
* @param propertytype Database column PropertyType SqlType(text), Default(None)
* @param datatype Database column Datatype SqlType(text), Default(None)
* @param editable Database column Editable SqlType(bool), Default(None)
* @param multivalue Database column MultiValue SqlType(bool), Default(None)
* @param propertygroup Database column PropertyGroup SqlType(text), Default(None)
* @param uiordinal Database column UIOrdinal SqlType(int4), Default(None)
* @param exportordinal Database column ExportOrdinal SqlType(int2), Default(None)
* @param allowexport Database column AllowExport SqlType(bool), Default(false) */
case class FilepropertyRow(name: String, description: Option[String] = None, fullname: Option[String] = None, createddatetime: java.sql.Timestamp, modifieddatetime: Option[java.sql.Timestamp] = None, propertytype: Option[String] = None, datatype: Option[String] = None, editable: Option[Boolean] = None, multivalue: Option[Boolean] = None, propertygroup: Option[String] = None, uiordinal: Option[Int] = None, exportordinal: Option[Short] = None, allowexport: Boolean = false)
/** GetResult implicit for fetching FilepropertyRow objects using plain SQL queries */
implicit def GetResultFilepropertyRow(implicit e0: GR[String], e1: GR[Option[String]], e2: GR[java.sql.Timestamp], e3: GR[Option[java.sql.Timestamp]], e4: GR[Option[Boolean]], e5: GR[Option[Int]], e6: GR[Option[Short]], e7: GR[Boolean]): GR[FilepropertyRow] = GR{
prs => import prs._
(FilepropertyRow.apply _).tupled((<<[String], <[String], <[String], <<[java.sql.Timestamp], <[java.sql.Timestamp], <[String], <[String], <[Boolean], <[Boolean], <[String], <[Int], <[Short], <<[Boolean]))
}
/** Table description of table FileProperty. Objects of this class serve as prototypes for rows in queries. */
class Fileproperty(_tableTag: Tag) extends profile.api.Table[FilepropertyRow](_tableTag, "FileProperty") {
def * = ((name, description, fullname, createddatetime, modifieddatetime, propertytype, datatype, editable, multivalue, propertygroup, uiordinal, exportordinal, allowexport)).mapTo[FilepropertyRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(name), description, fullname, Rep.Some(createddatetime), modifieddatetime, propertytype, datatype, editable, multivalue, propertygroup, uiordinal, exportordinal, Rep.Some(allowexport))).shaped.<>({r=>import r._; _1.map(_=> (FilepropertyRow.apply _).tupled((_1.get, _2, _3, _4.get, _5, _6, _7, _8, _9, _10, _11, _12, _13.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column Name SqlType(text), PrimaryKey */
val name: Rep[String] = column[String]("Name", O.PrimaryKey)
/** Database column Description SqlType(text), Default(None) */
val description: Rep[Option[String]] = column[Option[String]]("Description", O.Default(None))
/** Database column FullName SqlType(text), Default(None) */
val fullname: Rep[Option[String]] = column[Option[String]]("FullName", O.Default(None))
/** Database column CreatedDatetime SqlType(timestamptz) */
val createddatetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("CreatedDatetime")
/** Database column ModifiedDatetime SqlType(timestamptz), Default(None) */
val modifieddatetime: Rep[Option[java.sql.Timestamp]] = column[Option[java.sql.Timestamp]]("ModifiedDatetime", O.Default(None))
/** Database column PropertyType SqlType(text), Default(None) */
val propertytype: Rep[Option[String]] = column[Option[String]]("PropertyType", O.Default(None))
/** Database column Datatype SqlType(text), Default(None) */
val datatype: Rep[Option[String]] = column[Option[String]]("Datatype", O.Default(None))
/** Database column Editable SqlType(bool), Default(None) */
val editable: Rep[Option[Boolean]] = column[Option[Boolean]]("Editable", O.Default(None))
/** Database column MultiValue SqlType(bool), Default(None) */
val multivalue: Rep[Option[Boolean]] = column[Option[Boolean]]("MultiValue", O.Default(None))
/** Database column PropertyGroup SqlType(text), Default(None) */
val propertygroup: Rep[Option[String]] = column[Option[String]]("PropertyGroup", O.Default(None))
/** Database column UIOrdinal SqlType(int4), Default(None) */
val uiordinal: Rep[Option[Int]] = column[Option[Int]]("UIOrdinal", O.Default(None))
/** Database column ExportOrdinal SqlType(int2), Default(None) */
val exportordinal: Rep[Option[Short]] = column[Option[Short]]("ExportOrdinal", O.Default(None))
/** Database column AllowExport SqlType(bool), Default(false) */
val allowexport: Rep[Boolean] = column[Boolean]("AllowExport", O.Default(false))
/** Index over (allowexport) (database name fileproperty_allowexport_idx) */
val index1 = index("fileproperty_allowexport_idx", allowexport)
/** Index over (exportordinal) (database name fileproperty_exportordinal_idx) */
val index2 = index("fileproperty_exportordinal_idx", exportordinal)
}
/** Collection-like TableQuery object for table Fileproperty */
lazy val Fileproperty = new TableQuery(tag => new Fileproperty(tag))
/** Entity class storing rows of table Filepropertydependencies
* @param groupid Database column GroupId SqlType(int4)
* @param propertyname Database column PropertyName SqlType(text)
* @param default Database column Default SqlType(text), Default(None) */
case class FilepropertydependenciesRow(groupid: Int, propertyname: String, default: Option[String] = None)
/** GetResult implicit for fetching FilepropertydependenciesRow objects using plain SQL queries */
implicit def GetResultFilepropertydependenciesRow(implicit e0: GR[Int], e1: GR[String], e2: GR[Option[String]]): GR[FilepropertydependenciesRow] = GR{
prs => import prs._
(FilepropertydependenciesRow.apply _).tupled((<<[Int], <<[String], <[String]))
}
/** Table description of table FilePropertyDependencies. Objects of this class serve as prototypes for rows in queries. */
class Filepropertydependencies(_tableTag: Tag) extends profile.api.Table[FilepropertydependenciesRow](_tableTag, "FilePropertyDependencies") {
def * = ((groupid, propertyname, default)).mapTo[FilepropertydependenciesRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(groupid), Rep.Some(propertyname), default)).shaped.<>({r=>import r._; _1.map(_=> (FilepropertydependenciesRow.apply _).tupled((_1.get, _2.get, _3)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column GroupId SqlType(int4) */
val groupid: Rep[Int] = column[Int]("GroupId")
/** Database column PropertyName SqlType(text) */
val propertyname: Rep[String] = column[String]("PropertyName")
/** Database column Default SqlType(text), Default(None) */
val default: Rep[Option[String]] = column[Option[String]]("Default", O.Default(None))
/** Foreign key referencing Fileproperty (database name FilePropertyDependencies_FileProperty_PropertyName_fkey) */
lazy val filepropertyFk = foreignKey("FilePropertyDependencies_FileProperty_PropertyName_fkey", propertyname, Fileproperty)(r => r.name, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Filepropertydependencies */
lazy val Filepropertydependencies = new TableQuery(tag => new Filepropertydependencies(tag))
/** Entity class storing rows of table Filepropertyvalues
* @param propertyname Database column PropertyName SqlType(text)
* @param propertyvalue Database column PropertyValue SqlType(text)
* @param default Database column Default SqlType(bool), Default(None)
* @param dependencies Database column Dependencies SqlType(int4), Default(None)
* @param secondaryvalue Database column SecondaryValue SqlType(int4), Default(None)
* @param ordinal Database column Ordinal SqlType(int4), Default(None) */
case class FilepropertyvaluesRow(propertyname: String, propertyvalue: String, default: Option[Boolean] = None, dependencies: Option[Int] = None, secondaryvalue: Option[Int] = None, ordinal: Option[Int] = None)
/** GetResult implicit for fetching FilepropertyvaluesRow objects using plain SQL queries */
implicit def GetResultFilepropertyvaluesRow(implicit e0: GR[String], e1: GR[Option[Boolean]], e2: GR[Option[Int]]): GR[FilepropertyvaluesRow] = GR{
prs => import prs._
(FilepropertyvaluesRow.apply _).tupled((<<[String], <<[String], <[Boolean], <[Int], <[Int], <[Int]))
}
/** Table description of table FilePropertyValues. Objects of this class serve as prototypes for rows in queries. */
class Filepropertyvalues(_tableTag: Tag) extends profile.api.Table[FilepropertyvaluesRow](_tableTag, "FilePropertyValues") {
def * = ((propertyname, propertyvalue, default, dependencies, secondaryvalue, ordinal)).mapTo[FilepropertyvaluesRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(propertyname), Rep.Some(propertyvalue), default, dependencies, secondaryvalue, ordinal)).shaped.<>({r=>import r._; _1.map(_=> (FilepropertyvaluesRow.apply _).tupled((_1.get, _2.get, _3, _4, _5, _6)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column PropertyName SqlType(text) */
val propertyname: Rep[String] = column[String]("PropertyName")
/** Database column PropertyValue SqlType(text) */
val propertyvalue: Rep[String] = column[String]("PropertyValue")
/** Database column Default SqlType(bool), Default(None) */
val default: Rep[Option[Boolean]] = column[Option[Boolean]]("Default", O.Default(None))
/** Database column Dependencies SqlType(int4), Default(None) */
val dependencies: Rep[Option[Int]] = column[Option[Int]]("Dependencies", O.Default(None))
/** Database column SecondaryValue SqlType(int4), Default(None) */
val secondaryvalue: Rep[Option[Int]] = column[Option[Int]]("SecondaryValue", O.Default(None))
/** Database column Ordinal SqlType(int4), Default(None) */
val ordinal: Rep[Option[Int]] = column[Option[Int]]("Ordinal", O.Default(None))
/** Foreign key referencing Fileproperty (database name FilePropertyValues_FileProperty_PropertyName_fkey) */
lazy val filepropertyFk = foreignKey("FilePropertyValues_FileProperty_PropertyName_fkey", propertyname, Fileproperty)(r => r.name, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Filepropertyvalues */
lazy val Filepropertyvalues = new TableQuery(tag => new Filepropertyvalues(tag))
/** Entity class storing rows of table Filestatus
* @param filestatusid Database column FileStatusId SqlType(uuid), PrimaryKey
* @param fileid Database column FileId SqlType(uuid)
* @param statustype Database column StatusType SqlType(text)
* @param value Database column Value SqlType(text)
* @param createddatetime Database column CreatedDatetime SqlType(timestamptz) */
case class FilestatusRow(filestatusid: java.util.UUID, fileid: java.util.UUID, statustype: String, value: String, createddatetime: java.sql.Timestamp)
/** GetResult implicit for fetching FilestatusRow objects using plain SQL queries */
implicit def GetResultFilestatusRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[java.sql.Timestamp]): GR[FilestatusRow] = GR{
prs => import prs._
(FilestatusRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[String], <<[String], <<[java.sql.Timestamp]))
}
/** Table description of table FileStatus. Objects of this class serve as prototypes for rows in queries. */
class Filestatus(_tableTag: Tag) extends profile.api.Table[FilestatusRow](_tableTag, "FileStatus") {
def * = ((filestatusid, fileid, statustype, value, createddatetime)).mapTo[FilestatusRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(filestatusid), Rep.Some(fileid), Rep.Some(statustype), Rep.Some(value), Rep.Some(createddatetime))).shaped.<>({r=>import r._; _1.map(_=> (FilestatusRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column FileStatusId SqlType(uuid), PrimaryKey */
val filestatusid: Rep[java.util.UUID] = column[java.util.UUID]("FileStatusId", O.PrimaryKey)
/** Database column FileId SqlType(uuid) */
val fileid: Rep[java.util.UUID] = column[java.util.UUID]("FileId")
/** Database column StatusType SqlType(text) */
val statustype: Rep[String] = column[String]("StatusType")
/** Database column Value SqlType(text) */
val value: Rep[String] = column[String]("Value")
/** Database column CreatedDatetime SqlType(timestamptz) */
val createddatetime: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("CreatedDatetime")
/** Foreign key referencing File (database name FileStatus_File_fkey) */
lazy val fileFk = foreignKey("FileStatus_File_fkey", fileid, File)(r => r.fileid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
/** Index over (fileid,statustype,value,filestatusid,createddatetime) (database name filestatus_allfields_idx) */
val index1 = index("filestatus_allfields_idx", (fileid, statustype, value, filestatusid, createddatetime))
/** Index over (fileid,statustype) (database name filestatus_fileid_statustype_idx) */
val index2 = index("filestatus_fileid_statustype_idx", (fileid, statustype))
}
/** Collection-like TableQuery object for table Filestatus */
lazy val Filestatus = new TableQuery(tag => new Filestatus(tag))
/** Entity class storing rows of table FlywaySchemaHistory
* @param installedRank Database column installed_rank SqlType(int4), PrimaryKey
* @param version Database column version SqlType(varchar), Length(50,true), Default(None)
* @param description Database column description SqlType(varchar), Length(200,true)
* @param `type` Database column type SqlType(varchar), Length(20,true)
* @param script Database column script SqlType(varchar), Length(1000,true)
* @param checksum Database column checksum SqlType(int4), Default(None)
* @param installedBy Database column installed_by SqlType(varchar), Length(100,true)
* @param installedOn Database column installed_on SqlType(timestamp)
* @param executionTime Database column execution_time SqlType(int4)
* @param success Database column success SqlType(bool) */
case class FlywaySchemaHistoryRow(installedRank: Int, version: Option[String] = None, description: String, `type`: String, script: String, checksum: Option[Int] = None, installedBy: String, installedOn: java.sql.Timestamp, executionTime: Int, success: Boolean)
/** GetResult implicit for fetching FlywaySchemaHistoryRow objects using plain SQL queries */
implicit def GetResultFlywaySchemaHistoryRow(implicit e0: GR[Int], e1: GR[Option[String]], e2: GR[String], e3: GR[Option[Int]], e4: GR[java.sql.Timestamp], e5: GR[Boolean]): GR[FlywaySchemaHistoryRow] = GR{
prs => import prs._
(FlywaySchemaHistoryRow.apply _).tupled((<<[Int], <[String], <<[String], <<[String], <<[String], <[Int], <<[String], <<[java.sql.Timestamp], <<[Int], <<[Boolean]))
}
/** Table description of table flyway_schema_history. Objects of this class serve as prototypes for rows in queries.
* NOTE: The following names collided with Scala keywords and were escaped: type */
class FlywaySchemaHistory(_tableTag: Tag) extends profile.api.Table[FlywaySchemaHistoryRow](_tableTag, "flyway_schema_history") {
def * = ((installedRank, version, description, `type`, script, checksum, installedBy, installedOn, executionTime, success)).mapTo[FlywaySchemaHistoryRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(installedRank), version, Rep.Some(description), Rep.Some(`type`), Rep.Some(script), checksum, Rep.Some(installedBy), Rep.Some(installedOn), Rep.Some(executionTime), Rep.Some(success))).shaped.<>({r=>import r._; _1.map(_=> (FlywaySchemaHistoryRow.apply _).tupled((_1.get, _2, _3.get, _4.get, _5.get, _6, _7.get, _8.get, _9.get, _10.get)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column installed_rank SqlType(int4), PrimaryKey */
val installedRank: Rep[Int] = column[Int]("installed_rank", O.PrimaryKey)
/** Database column version SqlType(varchar), Length(50,true), Default(None) */
val version: Rep[Option[String]] = column[Option[String]]("version", O.Length(50,varying=true), O.Default(None))
/** Database column description SqlType(varchar), Length(200,true) */
val description: Rep[String] = column[String]("description", O.Length(200,varying=true))
/** Database column type SqlType(varchar), Length(20,true)
* NOTE: The name was escaped because it collided with a Scala keyword. */
val `type`: Rep[String] = column[String]("type", O.Length(20,varying=true))
/** Database column script SqlType(varchar), Length(1000,true) */
val script: Rep[String] = column[String]("script", O.Length(1000,varying=true))
/** Database column checksum SqlType(int4), Default(None) */
val checksum: Rep[Option[Int]] = column[Option[Int]]("checksum", O.Default(None))
/** Database column installed_by SqlType(varchar), Length(100,true) */
val installedBy: Rep[String] = column[String]("installed_by", O.Length(100,varying=true))
/** Database column installed_on SqlType(timestamp) */
val installedOn: Rep[java.sql.Timestamp] = column[java.sql.Timestamp]("installed_on")
/** Database column execution_time SqlType(int4) */
val executionTime: Rep[Int] = column[Int]("execution_time")
/** Database column success SqlType(bool) */
val success: Rep[Boolean] = column[Boolean]("success")
/** Index over (success) (database name flyway_schema_history_s_idx) */
val index1 = index("flyway_schema_history_s_idx", success)
}
/** Collection-like TableQuery object for table FlywaySchemaHistory */
lazy val FlywaySchemaHistory = new TableQuery(tag => new FlywaySchemaHistory(tag))
/** Entity class storing rows of table Series
* @param seriesid Database column SeriesId SqlType(uuid), PrimaryKey
* @param bodyid Database column BodyId SqlType(uuid)
* @param name Database column Name SqlType(text)
* @param code Database column Code SqlType(text)
* @param description Database column Description SqlType(text), Default(None) */
case class SeriesRow(seriesid: java.util.UUID, bodyid: java.util.UUID, name: String, code: String, description: Option[String] = None)
/** GetResult implicit for fetching SeriesRow objects using plain SQL queries */
implicit def GetResultSeriesRow(implicit e0: GR[java.util.UUID], e1: GR[String], e2: GR[Option[String]]): GR[SeriesRow] = GR{
prs => import prs._
(SeriesRow.apply _).tupled((<<[java.util.UUID], <<[java.util.UUID], <<[String], <<[String], <[String]))
}
/** Table description of table Series. Objects of this class serve as prototypes for rows in queries. */
class Series(_tableTag: Tag) extends profile.api.Table[SeriesRow](_tableTag, "Series") {
def * = ((seriesid, bodyid, name, code, description)).mapTo[SeriesRow]
/** Maps whole row to an option. Useful for outer joins. */
def ? = ((Rep.Some(seriesid), Rep.Some(bodyid), Rep.Some(name), Rep.Some(code), description)).shaped.<>({r=>import r._; _1.map(_=> (SeriesRow.apply _).tupled((_1.get, _2.get, _3.get, _4.get, _5)))}, (_:Any) => throw new Exception("Inserting into ? projection not supported."))
/** Database column SeriesId SqlType(uuid), PrimaryKey */
val seriesid: Rep[java.util.UUID] = column[java.util.UUID]("SeriesId", O.PrimaryKey)
/** Database column BodyId SqlType(uuid) */
val bodyid: Rep[java.util.UUID] = column[java.util.UUID]("BodyId")
/** Database column Name SqlType(text) */
val name: Rep[String] = column[String]("Name")
/** Database column Code SqlType(text) */
val code: Rep[String] = column[String]("Code")
/** Database column Description SqlType(text), Default(None) */
val description: Rep[Option[String]] = column[Option[String]]("Description", O.Default(None))
/** Foreign key referencing Body (database name Series_Body_fkey) */
lazy val bodyFk = foreignKey("Series_Body_fkey", bodyid, Body)(r => r.bodyid, onUpdate=ForeignKeyAction.NoAction, onDelete=ForeignKeyAction.NoAction)
}
/** Collection-like TableQuery object for table Series */
lazy val Series = new TableQuery(tag => new Series(tag))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy