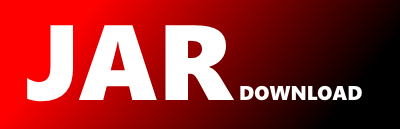
uk.gov.service.notify.LetterResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of notifications-java-client Show documentation
Show all versions of notifications-java-client Show documentation
Use this client to send emails, text messages and letters using the GOV.UK Notify API.
package uk.gov.service.notify;
import org.json.JSONObject;
import java.util.Optional;
import java.util.UUID;
public class LetterResponse {
private final UUID notificationId;
private final String reference;
private final String postage;
private final JSONObject data;
public LetterResponse(String response) {
data = new JSONObject(response);
notificationId = UUID.fromString(data.getString("id"));
reference = data.isNull("reference") ? null : data.getString("reference");
postage = data.isNull("postage") ? null : data.getString("postage");
}
public UUID getNotificationId() {
return notificationId;
}
public Optional getReference() {
return Optional.ofNullable(reference);
}
public JSONObject getData() {
return data;
}
public Optional getPostage() {
return Optional.ofNullable(postage);
}
@Override
public String toString() {
return "SendLetterResponse{" +
"notificationId=" + notificationId +
", reference=" + reference +
'}';
}
public String tryToGetString(JSONObject jsonObj, String key)
{
if (jsonObj.has(key))
{
if(jsonObj.opt(key).toString().equals("null"))
{
return null;
}
else
{
return jsonObj.opt(key).toString();
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy