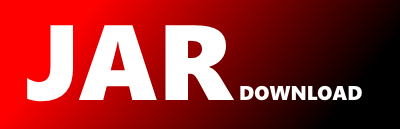
uk.gov.service.notify.TemplatePreview Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of notifications-java-client Show documentation
Show all versions of notifications-java-client Show documentation
Use this client to send emails, text messages and letters using the GOV.UK Notify API.
package uk.gov.service.notify;
import org.json.JSONObject;
import java.util.Optional;
import java.util.UUID;
public class TemplatePreview {
private UUID id;
private String templateType;
private int version;
private String body;
private String subject;
private String html;
public TemplatePreview(String content){
JSONObject responseBodyAsJson = new JSONObject(content);
build(responseBodyAsJson);
}
public TemplatePreview(org.json.JSONObject data){
build(data);
}
private void build(JSONObject data) {
id = UUID.fromString(data.getString("id"));
templateType = data.getString("type");
version = data.getInt("version");
body = data.getString("body");
subject = data.isNull("subject") ? null : data.getString("subject");
html = data.isNull("html") ? null : data.getString("html");
}
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public String getTemplateType() {
return templateType;
}
public void setTemplateType(String templateType) {
this.templateType = templateType;
}
public int getVersion() {
return version;
}
public void setVersion(int version) {
this.version = version;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public Optional getSubject() {
return Optional.ofNullable(subject);
}
public void setSubject(String subject) {
this.subject = subject;
}
public Optional getHtml() {
return Optional.ofNullable(html);
}
public void setHtml(String html) {
this.html = html;
}
@Override
public String toString() {
return "Template{" +
"id=" + id +
", templateType='" + templateType + '\'' +
", version=" + version +
", body='" + body + '\'' +
", subject='" + subject + '\'' +
", html='" + html + '\'' +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy