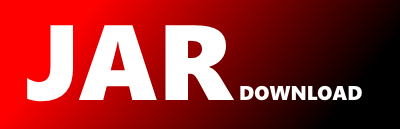
uk.kludje.annotation.processor.interface.txt Maven / Gradle / Ivy
/*Generated file; do not edit*/
package ${pack};
/**
* TLDR; IMPLEMENT {@code ${functionSignature} throws Throwable} USING A LAMBDA; INVOKE {@code ${parentSig}}.
*
* A functional interface for handling checked exceptions.
* See parent type for interface intent.
*
* {@code ${parentSig}} invokes {@code ${functionSignature}}
* and throws anything thrown by {@code ${functionSignature}}.
*
* General example using {@code java.lang.Runnable} that reduces 11 lines to a single line.
* Call {@code someMethodThatThrowsException} in {@code throwsNothing}:
*
* public void someMethodThatThrowsException() throws IOException {
* // I/O functionality
* }
*
* public void throwsNothing(Runnable r) {
* r.run();
* }
*
*
* Before:
*
* public void yourMethod() throws IOException {
* try {
* throwsNothing(() -> {
* try {
* someMethodThatThrowsException();
* } catch (IOException e) {
* throw new SomeWrapperException(e);
* }
* });
* } catch(SomeWrapperException w) {
* throw (IOException) w.getCause();
* }
* }
*
*
* After:
*
* public void yourMethod() throws IOException {
* throwsNothing((URunnable) this::someMethodThatThrowsException);
* }
*
*
* Method {@code public static ${generics} ${simpleName}${parentGenerics} as${simpleName}(${simpleName}${parentGenerics} t)}
* provides a convenience method for manufacturing types as an alternative to casting.
*/
@java.lang.FunctionalInterface
@javax.annotation.Generated("${generator}")
@SuppressWarnings("javadoc")
public interface ${simpleName}${generics} extends ${parent}${parentGenerics} {
${parentSigGenerics} default ${parentSig} {
try {
${invocation};
} catch (Throwable throwable) {
throw uk.kludje.Exceptions.throwChecked(throwable);
}
}
${functionSignature} throws Throwable;
public static ${generics} ${simpleName}${parentGenerics} as${simpleName}(${simpleName}${parentGenerics} t) {
return t;
}
}