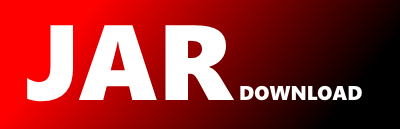
uk.org.retep.util.collections.map.TimestampedHashMap Maven / Gradle / Ivy
/*
* Copyright (c) 1998-2010, Peter T Mount
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* - Neither the name of the retep.org.uk nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
*
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER
* OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package uk.org.retep.util.collections.map;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.concurrent.TimeUnit;
/**
*
* @author peter
*/
public class TimestampedHashMap
extends TreeMap
implements TimestampedMap
{
static final long serialVersionUID = -7193127234818772335L;
private TimeUnit timeUnit;
private int maxSize;
public TimestampedHashMap()
{
this( 0, TimeUnit.MILLISECONDS );
}
public TimestampedHashMap( TimeUnit unit )
{
this( 0, unit );
}
public TimestampedHashMap( int maxSize )
{
this( maxSize, TimeUnit.MILLISECONDS );
}
public TimestampedHashMap( int maxSize, TimeUnit unit )
{
super();
this.timeUnit = unit;
this.maxSize = maxSize;
}
public int getMaxSize()
{
return maxSize;
}
public void setMaxSize( int maxSize )
{
this.maxSize = maxSize;
if( maxSize > 0 )
{
while( size() > maxSize )
{
removeOldestEntry();
}
}
}
public TimeUnit getTimeUnit()
{
return timeUnit;
}
public void setTimeUnit( TimeUnit unit )
{
timeUnit = unit;
for( Timestamp t : keySet() )
{
TimeUnit oldUnit = t.getTimeUnit();
t.setTimeStamp( timeUnit.convert( t.getTimeStamp(), oldUnit ) );
t.setTimeUnit( timeUnit );
}
}
public final Set> entrySet( Calendar start,
Calendar end )
{
return entrySet( start.getTimeInMillis(), end.getTimeInMillis() );
}
public final Set> entrySet( Date start, Date end )
{
return entrySet( start.getTime(), end.getTime() );
}
public final Set> entrySet( long start, long end )
{
return entrySet( start, end, TimeUnit.MILLISECONDS );
}
public final Set> entrySet( long start, long end,
TimeUnit unit )
{
return entrySet( start, unit, end, unit );
}
public Set> entrySet(
long start, TimeUnit startUnit,
long end, TimeUnit endUnit )
{
Set> ret = new HashSet>();
for( Map.Entry e : entrySet() )
{
if( e.getKey().between( start, startUnit, end, endUnit ) )
{
ret.add( e );
}
}
return ret;
}
// ======================================================================
public final void put( Calendar timestamp, E value )
{
put( timestamp.getTimeInMillis(), TimeUnit.MILLISECONDS, value );
}
public final void put( Date timestamp, E value )
{
put( timestamp.getTime(), TimeUnit.MILLISECONDS, value );
}
public final void put( long timestamp, E value )
{
put( timestamp, getTimeUnit(), value );
}
public void put( long timestamp, TimeUnit unit, E value )
{
put( new Timestamp( timestamp, unit ), value );
}
@Override
public E put( Timestamp t, E value )
{
E ret = super.put( t, value );
if( maxSize > 0 && size() > maxSize )
{
removeOldestEntry();
}
return ret;
}
//======================================================================
public Map.Entry removeOldestEntry()
{
Iterator> it = entrySet().iterator();
if( it.hasNext() )
{
Map.Entry e = it.next();
it.remove();
return e;
}
return null;
}
//======================================================================
public final E get( Calendar timestamp )
{
return get( timestamp.getTimeInMillis() );
}
public final E get( Date timestamp )
{
return get( timestamp.getTime() );
}
public final E get( long timestamp )
{
return get( getTimeUnit().convert( timestamp, TimeUnit.MILLISECONDS ) );
}
public E get( long timestamp, TimeUnit unit )
{
return get( new Timestamp( timestamp, unit ) );
}
//======================================================================
public final Collection get( Calendar start, Calendar end )
{
return get( start.getTimeInMillis(), end.getTimeInMillis() );
}
public final Collection get( Date start, Date end )
{
return get( start.getTime(), end.getTime() );
}
public final Collection get( long start, long end )
{
return get( start, end, TimeUnit.MILLISECONDS );
}
public final Collection get( long start, long end, TimeUnit unit )
{
return get( start, unit, end, unit );
}
public Collection get( long start, TimeUnit startUnit, long end,
TimeUnit endUnit )
{
List ret = new ArrayList();
for( Map.Entry e : entrySet() )
{
if( e.getKey().between( start, startUnit, end, endUnit ) )
{
ret.add( e.getValue() );
}
}
return ret;
}
}