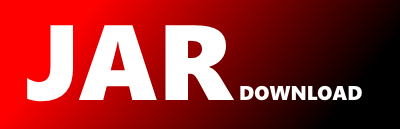
uk.org.retep.xmpp.muc.MucAuthenticator Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package uk.org.retep.xmpp.muc;
import uk.org.retep.util.messaging.MessageException;
import uk.org.retep.xmpp.JID;
import uk.org.retep.xmpp.message.ErrorCondition;
import uk.org.retep.xmpp.message.Message;
/**
* This interface acts as a hook to allow for external authentication to be
* performed with various protocols within the muc service.
*
* @author peter
*/
public interface MucAuthenticator
{
/**
* Can a user create a room
* @param roomName Requested room name
* @param jid JID of user attempting to create the room
* @return true if permitted, false if not
*/
boolean canUserCreateRoom( final String roomName, final JID jid );
/**
* Should this room be unlocked when created?
*
* This is not part of the XEP-0045 specification (that I can see) but it
* allows the option that a room is unlocked as soon as it's created.
*
* If in doubt then this should always return false
*
* @param roomName The created room name
* @return true to unlock the room, false to leave alone
*/
boolean unlockRoom( final String roomName );
/**
* Validate the sender to see if they are permitted to send a message
* to the room's members.
*
*
* This is a fail fast operation. If this returns anything other than
* ErrorCondition.OK then the user will be denied. However if this
* returns ErrorCondition.OK then processing will continue. In the default
* {@link uk.org.retep.xmpp.muc.visitor.MessageRelayVisitor} implementation
* this will then check the room's MucFeature set which then may fail.
*
*
*
* The default implementation simply returns {@link ErrorCondition#OK}
*
*
* @param sender
* @param room
* @param message
* @return
* @throws MessageException
*/
ErrorCondition canSenderRelayMessage( final MucRoomMember sender,
final MucRoom room,
final Message message )
throws MessageException;
/**
* Set the default room features for a room.
*
* The default implementation sets
* {@link uk.org.retep.xmpp.muc.MucFeature#MUC_OPEN},
* {@link uk.org.retep.xmpp.muc.MucFeature#MUC_UNMODERATED},
* {@link uk.org.retep.xmpp.muc.MucFeature#MUC_UNSECURED} and
* {@link uk.org.retep.xmpp.muc.MucFeature#MUC_NONANONYMOUS}.
*
* Other implementations can replace this with an alternate set of
* {@link uk.org.retep.xmpp.muc.MucFeature}'s.
*
* @param room
*/
void setDefaultRoomFeatures( final MucRoom room );
/**
* Enforce a nickname policy. This is called when a user either joins a room
* or attempts to change their nickname. You have the option of either:
*
*
* - Allowing the nickname (default) by returning the requestedNickname
* -
* Rewriting the nickname, returning the rewritten one,
* i.e. XEP-0045 7.3
*
* - throwing a {@link MessageException}
*
*
* @param room MucRoom
* @param jid The JID asking for the nickname
* @param requestedNickname The nickname the user is asking for
* @return The allowed nickname.
* @throws MessageException on failure. This can send an error response if
* required.
*/
String enforceNicknamePolicy( final MucRoom room,
final JID jid,
final String requestedNickname )
throws MessageException;
/**
* Can a user change their nickname via XEP-0045 7.3
*
* @param room {@link MucRoom}
* @param jid {@link JID} of the user
* @return true if they can change nickname (default), false if not.
* @throws MessageException
*/
boolean isNicknameChangeable( final MucRoom room, final JID jid )
throws MessageException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy