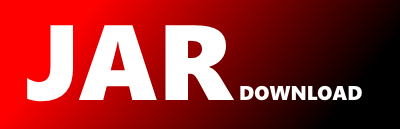
uk.org.retep.xmpp.muc.beans.BasicMucService Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package uk.org.retep.xmpp.muc.beans;
import uk.org.retep.xmpp.JID;
import uk.org.retep.xmpp.logic.XMPPLogic;
import uk.org.retep.xmpp.message.Message;
import uk.org.retep.xmpp.muc.MucAuthenticator;
import uk.org.retep.xmpp.muc.MucLogger;
import uk.org.retep.xmpp.muc.MucRoom;
import uk.org.retep.xmpp.muc.MucRoomSet;
import uk.org.retep.xmpp.muc.MucService;
import uk.org.retep.xmpp.muc.MucValidator;
import uk.org.retep.xmpp.presence.PresenceManager;
/**
* Data object attached to an XMPPComponent and passed to the various XMPPLogic
* instances. It maintains the links between various objects required for
* a functioning MucService.
*
* @author peter
*/
public class BasicMucService
implements MucService
{
private final JID jid;
private MucAuthenticator authenticator;
private MucValidator validator;
private MucLogger logger;
private MucRoomSet roomSet;
private XMPPLogic roomAbsentLogic;
private XMPPLogic roomLogic;
private XMPPLogic serverLogic;
private PresenceManager presenceManager;
public BasicMucService( final JID jid )
{
this.jid = jid;
}
public JID getJid()
{
return jid;
}
/**
* The {@link MucAuthenticator} to handle security
* @return the {@link MucAuthenticator} to handle security
*/
public MucAuthenticator getAuthenticator()
{
return authenticator;
}
/**
* Sets the {@link MucAuthenticator} to handle security
* @param authenticator the {@link MucAuthenticator} to handle security
*/
public void setAuthenticator( final MucAuthenticator authenticator )
{
this.authenticator = authenticator;
}
public MucValidator getValidator()
{
return validator;
}
public void setValidator( final MucValidator validator )
{
this.validator = validator;
}
/**
* The {@link MucRoomSet} implementation managing the {@link MucRoom}'s
*
* @return the {@link MucRoomSet} implementation managing the {@link MucRoom}'s
*/
public MucRoomSet getRoomSet()
{
return roomSet;
}
/**
* Set the {@link MucRoomSet} implementation managing the {@link MucRoom}'s
* @param roomSet the {@link MucRoomSet} implementation managing the {@link MucRoom}'s
*/
public void setRoomSet( final MucRoomSet roomSet )
{
this.roomSet = roomSet;
}
/**
* The {@link XMPPLogic} instance containing the logic of this
* service.
*
* @return the {@link XMPPLogic} instance containing the logic
* of this service.
*/
public XMPPLogic getRoomAbsentLogic()
{
return roomAbsentLogic;
}
/**
* Set the {@link XMPPLogic} instance containing the logic of this
* service.
* @param roomAbsentLanguage the {@link XMPPLogic} instance containing the
* logic of this service.
*/
public void setRoomAbsentLogic(
final XMPPLogic roomAbsentLanguage )
{
this.roomAbsentLogic = roomAbsentLanguage;
}
/**
* The {@link XMPPLogic} instance containing the logic of this
* service.
*
* @return the {@link XMPPLogic} instance containing the logic
* of this service.
*/
public XMPPLogic getRoomLogic()
{
return roomLogic;
}
/**
* Set the {@link XMPPLogic} instance containing the logic of this
* service.
* @param roomLogic the {@link XMPPLogic} instance containing the
* logic of this service.
*/
public void setRoomLogic( final XMPPLogic roomLogic )
{
this.roomLogic = roomLogic;
}
/**
* The {@link XMPPLogic} instance containing the logic of this
* service.
*
* @return the {@link XMPPLogic} instance containing the logic
* of this service.
*/
public XMPPLogic getServerLogic()
{
return serverLogic;
}
/**
* Set the {@link XMPPLogic} instance containing the logic of this
* service.
* @param serverLogic the {@link XMPPLogic} instance containing the
* logic of this service.
*/
public void setServerLogic(
final XMPPLogic serverLogic )
{
this.serverLogic = serverLogic;
}
public void setLogger( final MucLogger logger )
{
this.logger = logger;
}
@Override
public void logMessage( final MucRoom room, final Message message )
{
if( logger != null )
{
logger.logMessage( room, message );
}
}
@Override
public PresenceManager getPresenceManager()
{
return presenceManager;
}
public void setPresenceManager( final PresenceManager presenceManager )
{
this.presenceManager = presenceManager;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy