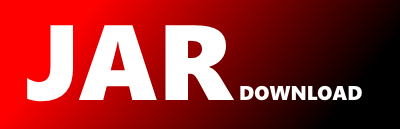
uk.org.retep.xmpp.muc.logic.DiscoveryLogic Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package uk.org.retep.xmpp.muc.logic;
import java.util.Collection;
import java.util.List;
import uk.org.retep.util.messaging.MessageException;
import uk.org.retep.xmpp.JID;
import uk.org.retep.xmpp.XMPPServer;
import uk.org.retep.xmpp.builder.IqBuilder;
import uk.org.retep.xmpp.logic.UnexpectedMessageLogic;
import uk.org.retep.xmpp.logic.XMPPLogic;
import uk.org.retep.xmpp.logic.XMPPProtocolLogic;
import uk.org.retep.xmpp.message.Iq;
import uk.org.retep.xmpp.message.Message;
import uk.org.retep.xmpp.util.MessageFactory;
import uk.org.retep.xmpp.message.Presence;
import uk.org.retep.xmpp.message.Stanza;
import uk.org.retep.xmpp.muc.MucRoom;
import uk.org.retep.xmpp.muc.MucRoomSet;
import uk.org.retep.xmpp.muc.MucService;
/**
* XEP-0045 Discovery
*
* @author peter
*/
public enum DiscoveryLogic
implements XMPPProtocolLogic
{
/**
* XEP-0045 6.1 Discovering Component Support for MUC
*/
MUC_SUPPORT
{
@Override
public Class> getProtocol()
{
return org.jabber.protocol.disco_info.Query.class;
}
@Override
public XMPPLogic process( final XMPPServer server,
final MucService service,
final MucRoomSet roomSet,
final Iq iq )
throws MessageException
{
final org.jabber.protocol.disco_info.QueryBuilder queryBuilder = new org.jabber.protocol.disco_info.QueryBuilder();
queryBuilder.addIdentity( "Chat Service", "conference", "text", null );
queryBuilder.addFeature( null, "http://jabber.org/protocol/muc" );
server.send( iq.getResponseBuilder().addContent( queryBuilder ) );
return null;
}
},
/**
* XEP-0045 6.2 Discovering Rooms
*/
ROOMS
{
@Override
public Class> getProtocol()
{
return org.jabber.protocol.disco_items.Query.class;
}
@Override
public XMPPLogic process( final XMPPServer server,
final MucService service,
final MucRoomSet ignore,
final Iq iq )
throws MessageException
{
final MucRoomSet roomSet = service.getRoomSet();
final JID baseJid = roomSet.getJid().copyWithoutNodeOrResource();
final org.jabber.protocol.disco_items.QueryBuilder queryBuilder = new org.jabber.protocol.disco_items.QueryBuilder();
// See commented out section below of a bug in XEP-0045 RETEPXMPP-1
//String firstName = null;
//String lastName = null;
final Collection names = roomSet.getRoomNames();
for( String name : names )
{
// This is ok as if the room is deleted between the get and
// sending the response, they would get an error - which is a normal
// usecase anyhow - this is a snapshot not definitive list
final MucRoom room = roomSet.get( name );
if( room != null )
{
// See commented out section below of a bug in XEP-0045
//if( firstName == null )
//{
// firstName = name;
//}
//lastName = name;
queryBuilder.addItem(
null,
// TODO confusingly name in Item is actually the subject? needs confirming
room.getSubject(),
baseJid.copyWithoutNodeOrResource().setNode( name ),
null );
}
}
/*
* FIXME RETEPXMPP-1 According to XEP-0045 6.3 this should happen.
* However the XML Schema only permits Items inside Query so we need
* to check with xmpp.org and get the schema fixed
* if( names.size() < roomSet.getSize() )
* {
* final Set set = new Set();
*
* final First first = new First();
* first.setIndex( 0 );
* first.setValue( firstName );
* set.setFirst( first );
* set.setLast( lastName );
* set.setCount( roomSet.getSize() );
* col.add( set );
* }
*/
server.send( iq.getResponseBuilder().addContent( queryBuilder ) );
return null;
}
};
@Override
public XMPPLogic process( final XMPPServer server,
final MucService service,
final MucRoomSet set,
final Message message )
throws MessageException
{
return UnexpectedMessageLogic.INSTANCE;
}
@Override
public XMPPLogic process( final XMPPServer server,
final MucService service,
final MucRoomSet set,
final Presence presence )
throws MessageException
{
return UnexpectedMessageLogic.INSTANCE;
}
@Override
public XMPPLogic process( final XMPPServer server,
final MucService service,
final MucRoomSet room,
final Stanza stanza )
throws MessageException
{
return UnexpectedMessageLogic.INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy