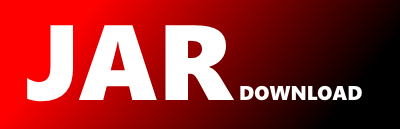
uk.org.retep.xmpp.muc.room.JoinExistingRoomLogic Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package uk.org.retep.xmpp.muc.room;
import javax.xml.bind.JAXBElement;
import uk.org.retep.util.messaging.MessageException;
import uk.org.retep.xmpp.JID;
import uk.org.retep.xmpp.XMPPException;
import uk.org.retep.xmpp.XMPPServer;
import uk.org.retep.xmpp.logic.AbstractXMPPLogic;
import uk.org.retep.xmpp.logic.XMPPLogic;
import uk.org.retep.xmpp.logic.XMPPProtocolLogic;
import uk.org.retep.xmpp.message.ErrorCondition;
import uk.org.retep.xmpp.util.MessageFactory;
import uk.org.retep.xmpp.message.Presence;
import uk.org.retep.xmpp.muc.MucRoom;
import uk.org.retep.xmpp.muc.MucRoomMember;
import uk.org.retep.xmpp.muc.MucService;
import uk.org.retep.xmpp.muc.beans.BasicMucRoomMember;
import static uk.org.retep.xmpp.XMPPConstants.*;
/**
* XEP-0045 7.1 - Joining a room
*
* This {@link XMPPLogic} is used when a room already exists
*
* @author peter
*/
public class JoinExistingRoomLogic
extends AbstractXMPPLogic
implements XMPPProtocolLogic
{
private static JoinExistingRoomLogic instance = new JoinExistingRoomLogic();
public static JoinExistingRoomLogic getInstance()
{
return instance;
}
private JoinExistingRoomLogic()
{
}
@Override
public Class> getProtocol()
{
return org.jabber.protocol.muc.X.class;
}
@Override
public XMPPLogic process( final XMPPServer server,
final MucService service,
final MucRoom room,
final Presence presence )
throws MessageException
{
// use presence.getTo() and not mucRoom.getJid() for the room JID
// to reduce cross-cluster locking
final JID chatRoomJid = presence.getTo();
// Who is entering the room
final MucRoomMember joiningMember = new BasicMucRoomMember(
chatRoomJid.getResource(),
presence.getFrom() );
// The requested nickName and run it through any rewrite policy
final String requestedNickName = joiningMember.getNickname();
final String nickName = service.getAuthenticator().enforceNicknamePolicy(
room,
chatRoomJid,
requestedNickName );
// Who is entering the room
final JID userJid = presence.getFrom();
try
{
// This will check for a nickname conflict, room full etc.
//Later reserved nicknames go here as well
final String oldNick = room.add( joiningMember,
service.getAuthenticator() );
// XEP-0172 support - if supplied store the persistentNickname in the MucRoomMember
final JAXBElement persistentNickname = MessageFactory.getJAXBElementNamespaceURI(
NS_NICK, presence.getContent() );
if( persistentNickname != null )
{
// Don't use joiningMember as that may not be the same instance
// in the room (i.e. nickname change)
final MucRoomMember realMember = room.getMember( nickName );
if( realMember == null )
{
// Should not occur unless the member has just left
throw new XMPPException(
ErrorCondition.INTERNAL_SERVER_ERROR );
}
else
{
realMember.setPersistentNickname( persistentNickname.getValue() );
}
}
if( oldNick != null )
{
return new NicknameChangedBroadcast( chatRoomJid,
joiningMember,
userJid,
requestedNickName,
nickName,
oldNick );
}
else
{
return new EnteredRoomBroadcast( chatRoomJid,
joiningMember,
userJid,
requestedNickName,
nickName );
}
}
catch( XMPPException xe )
{
final ErrorCondition errorCode = xe.getError();
getLog().error(
"%s: XMPPException[%s] - requested \"%s\" using \"%s\"",
chatRoomJid,
errorCode,
requestedNickName,
nickName );
if( errorCode == null )
{
throw xe;
}
else
{
final Presence response = MessageFactory.newResponse(
presence );
response.getContent().add( new org.jabber.protocol.muc.X() );
errorCode.addError( response );
response.setType( ERROR );
server.send( response );
return null;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy