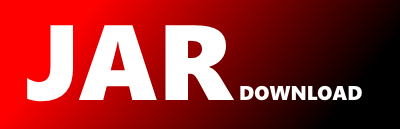
schemacrawler.schema.Table Maven / Gradle / Ivy
/*
========================================================================
SchemaCrawler
http://www.schemacrawler.com
Copyright (c) 2000-2021, Sualeh Fatehi .
All rights reserved.
------------------------------------------------------------------------
SchemaCrawler is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
SchemaCrawler and the accompanying materials are made available under
the terms of the Eclipse Public License v1.0, GNU General Public License
v3 or GNU Lesser General Public License v3.
You may elect to redistribute this code under any of these licenses.
The Eclipse Public License is available at:
http://www.eclipse.org/legal/epl-v10.html
The GNU General Public License v3 and the GNU Lesser General Public
License v3 are available at:
http://www.gnu.org/licenses/
========================================================================
*/
package schemacrawler.schema;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
/** Represents a table in the database. */
public interface Table extends DatabaseObject, TypedObject, DefinedObject {
/**
* Gets the list of all alternate keys of the table.
*
* @return Alternate keys of the table.
*/
Collection getAlternateKeys();
/**
* Gets the list of columns in ordinal order.
*
* @return Columns of the table
*/
List getColumns();
/**
* Gets the list of exported foreign keys. That is, only those whose primary key is referenced in
* another table.
*
* @return Exported foreign keys of the table.
*/
Collection getExportedForeignKeys();
/**
* Gets the list of all foreign keys of the table, including imported and exported foreign keys.
*
* @return Foreign keys of the table.
*/
Collection getForeignKeys();
/**
* Gets hidden columns.
*
* @return Columns of the table
*/
Collection getHiddenColumns();
/**
* Gets the list of imported foreign keys. That is, only those that reference a primary key
* another table.
*
* @return Imported foreign keys of the table.
*/
Collection getImportedForeignKeys();
/**
* Gets the list of indexes.
*
* @return Indexes of the table.
*/
Collection getIndexes();
/**
* Gets the primary key.
*
* @return Primary key
*/
PrimaryKey getPrimaryKey();
/**
* Gets the list of privileges.
*
* @return Privileges for the table.
*/
Collection> getPrivileges();
/**
* Gets the tables related to this one, based on the specified relationship type. Child tables are
* those who have a foreign key from this table. Parent tables are those to which this table has a
* foreign key.
*
* @param tableRelationshipType Table relationship type
* @return Related tables.
*/
Collection