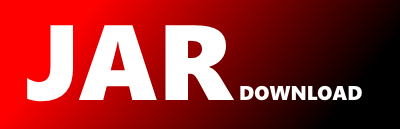
schemacrawler.crawl.MutableJdbcDriverInfo Maven / Gradle / Ivy
/*
========================================================================
SchemaCrawler
http://www.schemacrawler.com
Copyright (c) 2000-2020, Sualeh Fatehi .
All rights reserved.
------------------------------------------------------------------------
SchemaCrawler is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
SchemaCrawler and the accompanying materials are made available under
the terms of the Eclipse Public License v1.0, GNU General Public License
v3 or GNU Lesser General Public License v3.
You may elect to redistribute this code under any of these licenses.
The Eclipse Public License is available at:
http://www.eclipse.org/legal/epl-v10.html
The GNU General Public License v3 and the GNU Lesser General Public
License v3 are available at:
http://www.gnu.org/licenses/
========================================================================
*/
package schemacrawler.crawl;
import static java.util.Comparator.naturalOrder;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import schemacrawler.schema.JdbcDriverInfo;
import schemacrawler.schema.JdbcDriverProperty;
/**
* JDBC driver information. Created from metadata returned by a JDBC call, and
* other sources of information.
*
* @author Sualeh Fatehi [email protected]
*/
final class MutableJdbcDriverInfo
implements JdbcDriverInfo
{
private static final long serialVersionUID = 8030156654422512161L;
private final Set jdbcDriverProperties =
new HashSet<>();
private String connectionUrl = "";
private String driverClassName = "";
private String driverName = "";
private String driverVersion = "";
private boolean jdbcCompliant;
/**
* {@inheritDoc}
*/
@Override
public String getConnectionUrl()
{
return connectionUrl;
}
void setConnectionUrl(final String connectionUrl)
{
this.connectionUrl = connectionUrl;
}
/**
* {@inheritDoc}
*/
@Override
public String getDriverClassName()
{
return driverClassName;
}
/**
* {@inheritDoc}
*/
@Override
public Collection getDriverProperties()
{
final List properties =
new ArrayList<>(jdbcDriverProperties);
properties.sort(naturalOrder());
return properties;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isJdbcCompliant()
{
return jdbcCompliant;
}
void setJdbcCompliant(final boolean jdbcCompliant)
{
this.jdbcCompliant = jdbcCompliant;
}
/**
* {@inheritDoc}
*/
@Override
public String getProductName()
{
return driverName;
}
/**
* {@inheritDoc}
*/
@Override
public String getProductVersion()
{
return driverVersion;
}
/**
* {@inheritDoc}
*/
@Override
public String toString()
{
final StringBuilder info = new StringBuilder(1024);
info
.append("-- driver: ")
.append(getProductName())
.append(' ')
.append(getProductVersion())
.append(System.lineSeparator());
info
.append("-- driver class: ")
.append(getDriverClassName())
.append(System.lineSeparator());
info
.append("-- url: ")
.append(getConnectionUrl())
.append(System.lineSeparator());
info
.append("-- jdbc compliant: ")
.append(isJdbcCompliant());
return info.toString();
}
/**
* Adds a JDBC driver property.
*
* @param jdbcDriverProperty
* JDBC driver property
*/
void addJdbcDriverProperty(final ImmutableJdbcDriverProperty jdbcDriverProperty)
{
jdbcDriverProperties.add(jdbcDriverProperty);
}
void setDriverName(final String driverName)
{
this.driverName = driverName;
}
void setDriverVersion(final String driverVersion)
{
this.driverVersion = driverVersion;
}
void setJdbcDriverClassName(final String jdbcDriverClassName)
{
driverClassName = jdbcDriverClassName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy