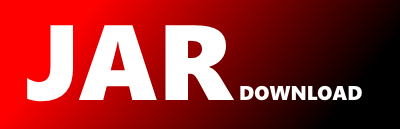
schemacrawler.tools.options.Config Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of schemacrawler Show documentation
Show all versions of schemacrawler Show documentation
SchemaCrawler is an open-source Java API that makes working with database metadata as easy as working with plain old Java objects. SchemaCrawler is also a database schema discovery and comprehension, and schema documentation tool. You can search for database schema objects using regular expressions, and output the schema and data in a readable text format. The output is designed to be diff-ed against other database schemas.
/*
========================================================================
SchemaCrawler
http://www.schemacrawler.com
Copyright (c) 2000-2024, Sualeh Fatehi .
All rights reserved.
------------------------------------------------------------------------
SchemaCrawler is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
SchemaCrawler and the accompanying materials are made available under
the terms of the Eclipse Public License v1.0, GNU General Public License
v3 or GNU Lesser General Public License v3.
You may elect to redistribute this code under any of these licenses.
The Eclipse Public License is available at:
http://www.eclipse.org/legal/epl-v10.html
The GNU General Public License v3 and the GNU Lesser General Public
License v3 are available at:
http://www.gnu.org/licenses/
========================================================================
*/
package schemacrawler.tools.options;
import static schemacrawler.utility.EnumUtility.enumValue;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.logging.Level;
import java.util.logging.Logger;
import static java.util.Objects.requireNonNull;
import static us.fatehi.utility.Utility.isBlank;
import schemacrawler.inclusionrule.InclusionRule;
import schemacrawler.inclusionrule.RegularExpressionRule;
import schemacrawler.schemacrawler.Options;
import us.fatehi.utility.ObjectToString;
import us.fatehi.utility.string.StringFormat;
/** Configuration properties. */
public final class Config implements Options {
public static final Logger LOGGER = Logger.getLogger(Config.class.getName());
private final Map configMap;
/** Creates an empty config. */
public Config() {
configMap = new HashMap<>();
}
public Config(final Config config) {
this();
merge(config);
}
/**
* Copies config into a map.
*
* @param configMap Config to copy
*/
public Config(final Map map) {
this();
if (map != null) {
configMap.putAll(map);
}
}
public boolean containsKey(final String key) {
return configMap.containsKey(key);
}
/**
* Gets the value of a property as a boolean.
*
* @param propertyName Property name
* @return Boolean value
*/
public boolean getBooleanValue(final String propertyName) {
return getBooleanValue(propertyName, false);
}
public boolean getBooleanValue(final String propertyName, final boolean defaultValue) {
return Boolean.parseBoolean(getStringValue(propertyName, Boolean.toString(defaultValue)));
}
/**
* Gets the value of a property as an enum.
*
* @param propertyName Property name
* @return Enum value
*/
public > E getEnumValue(final String propertyName, final E defaultValue) {
requireNonNull(defaultValue, "No default value provided");
// Check if this can be looked up
if (!configMap.containsKey(propertyName)) {
return defaultValue;
}
// Attempt to get as an object
final Object objValue = configMap.get(propertyName);
if (objValue != null && (objValue.getClass() == defaultValue.getClass())) {
return (E) objValue;
}
// Otherwise attempt to match as a string
final String value = getStringValue(propertyName, defaultValue.name());
return enumValue(value, defaultValue);
}
/**
* Gets the value of a property as an integer.
*
* @param propertyName Property name
* @return Integer value
*/
public int getIntegerValue(final String propertyName, final int defaultValue) {
try {
return Integer.parseInt(getStringValue(propertyName, String.valueOf(defaultValue)));
} catch (final NumberFormatException e) {
LOGGER.log(
Level.FINEST,
e,
new StringFormat("Could not parse integer value for property <%s>", propertyName));
return defaultValue;
}
}
public T getObject(final String key, final T defaultValue) throws ClassCastException {
return (T) configMap.getOrDefault(key, defaultValue);
}
public Optional getOptionalInclusionRule(
final String includePatternProperty, final String excludePatternProperty) {
final String includePattern = getStringValue(includePatternProperty, null);
final String excludePattern = getStringValue(excludePatternProperty, null);
if (isBlank(includePattern) && isBlank(excludePattern)) {
return Optional.empty();
}
return Optional.of(new RegularExpressionRule(includePattern, excludePattern));
}
/**
* Gets the value of a property as a string.
*
* @param propertyName Property name
* @param defaultValue Default value
* @return String value
*/
public String getStringValue(final String propertyName, final String defaultValue) {
final Object value = configMap.get(propertyName);
if (value == null) {
return defaultValue;
}
return value.toString();
}
public Map getSubMap(final String propertyName) {
if (isBlank(propertyName)) {
return new HashMap<>(configMap);
}
final Map subMap = new HashMap<>();
for (final Entry configEntry : configMap.entrySet()) {
final String fullKey = configEntry.getKey();
if (fullKey == null || !fullKey.startsWith(propertyName)) {
continue;
}
final String key = fullKey.substring(propertyName.length() + 1);
final Object value = configEntry.getValue();
subMap.put(key, value);
}
return subMap;
}
public void merge(final Config config) {
if (config == null) {
return;
}
configMap.putAll(config.configMap);
}
/**
* Put an key-value pair into the config.
*
* @param key Key of the config map.
* @param value Value of the config map.
* @return Object that was put into the config, or null.
* @see java.util.Map#put(java.lang.Object, java.lang.Object)
*/
public Object put(final String key, final Object value) {
if (value == null) {
return configMap.remove(key);
}
if (!isBlank(key)) {
return configMap.put(key, value);
}
return null;
}
public int size() {
return configMap.size();
}
@Override
public String toString() {
return ObjectToString.toString(configMap);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy