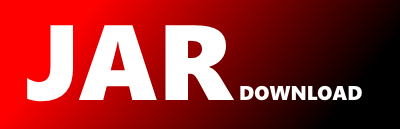
com.os.falcon.dwh.message.CSLevelDwhLog Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.os.falcon.dwh.message;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.os.falcon.dwh.event.constant.DWHEvents;
import com.os.falcon.dwh.message.item.AccountInfoItem;
import com.os.falcon.network.api.message.FMessage;
/**
*
* @author phamquan
*/
public class CSLevelDwhLog extends FMessage {
private AccountInfoItem accountInfo;
private int level;
private int maxPassedLevel;
private String difficulty;
private int duration; //second
private String where;
private String status;
private long createdDate;
public final static String DIFFUCULTY_EASY = "easy";
public final static String DIFFUCULTY_NORMAL = "normal";
public final static String DIFFUCULTY_HARD = "hard";
public final static String STATUS_REFAIL = "refail";
public final static String STATUS_REPASS = "repass";
public final static String STATUS_FAIL = "fail";
public final static String STATUS_PASS = "pass";
public CSLevelDwhLog() {
}
public CSLevelDwhLog(AccountInfoItem accountInfo, int level, int maxPassedLevel, String difficulty, int duration, String where, String status, long createdDate) {
this.accountInfo = accountInfo;
this.level = level;
this.maxPassedLevel = maxPassedLevel;
this.difficulty = difficulty;
this.duration = duration;
this.where = where;
this.status = status;
this.createdDate = createdDate;
}
public AccountInfoItem getAccountInfo() {
return accountInfo;
}
public void setAccountInfo(AccountInfoItem accountInfo) {
this.accountInfo = accountInfo;
}
public int getLevel() {
return level;
}
public void setLevel(int level) {
this.level = level;
}
public int getMaxPassedLevel() {
return maxPassedLevel;
}
public void setMaxPassedLevel(int maxPassedLevel) {
this.maxPassedLevel = maxPassedLevel;
}
public String getDifficulty() {
return difficulty;
}
public void setDifficulty(String difficulty) {
this.difficulty = difficulty;
}
public int getDuration() {
return duration;
}
public void setDuration(int duration) {
this.duration = duration;
}
public String getWhere() {
return where;
}
public void setWhere(String where) {
this.where = where;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public long getCreatedDate() {
return createdDate;
}
public void setCreatedDate(long createdDate) {
this.createdDate = createdDate;
}
@Override
@JsonIgnore
public String getEvent() {
return DWHEvents.CS_LEVEL_LOG;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy