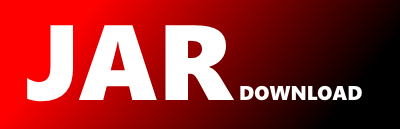
org.bitcoin.paymentchannel.Protos Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: paymentchannel.proto
package org.bitcoin.paymentchannel;
public final class Protos {
private Protos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface TwoWayChannelMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.TwoWayChannelMessage)
com.google.protobuf.MessageOrBuilder {
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
boolean hasType();
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType getType();
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
boolean hasClientVersion();
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
org.bitcoin.paymentchannel.Protos.ClientVersion getClientVersion();
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder getClientVersionOrBuilder();
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
boolean hasServerVersion();
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
org.bitcoin.paymentchannel.Protos.ServerVersion getServerVersion();
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder getServerVersionOrBuilder();
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
boolean hasInitiate();
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
org.bitcoin.paymentchannel.Protos.Initiate getInitiate();
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
org.bitcoin.paymentchannel.Protos.InitiateOrBuilder getInitiateOrBuilder();
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
boolean hasProvideRefund();
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
org.bitcoin.paymentchannel.Protos.ProvideRefund getProvideRefund();
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder getProvideRefundOrBuilder();
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
boolean hasReturnRefund();
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
org.bitcoin.paymentchannel.Protos.ReturnRefund getReturnRefund();
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder getReturnRefundOrBuilder();
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
boolean hasProvideContract();
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
org.bitcoin.paymentchannel.Protos.ProvideContract getProvideContract();
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder getProvideContractOrBuilder();
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
boolean hasUpdatePayment();
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
org.bitcoin.paymentchannel.Protos.UpdatePayment getUpdatePayment();
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder getUpdatePaymentOrBuilder();
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
boolean hasPaymentAck();
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
org.bitcoin.paymentchannel.Protos.PaymentAck getPaymentAck();
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder getPaymentAckOrBuilder();
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
boolean hasSettlement();
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
org.bitcoin.paymentchannel.Protos.Settlement getSettlement();
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
org.bitcoin.paymentchannel.Protos.SettlementOrBuilder getSettlementOrBuilder();
/**
* optional .paymentchannels.Error error = 10;
*/
boolean hasError();
/**
* optional .paymentchannels.Error error = 10;
*/
org.bitcoin.paymentchannel.Protos.Error getError();
/**
* optional .paymentchannels.Error error = 10;
*/
org.bitcoin.paymentchannel.Protos.ErrorOrBuilder getErrorOrBuilder();
}
/**
* Protobuf type {@code paymentchannels.TwoWayChannelMessage}
*
*
* This message is designed to be either sent raw over the network (e.g. length prefixed) or embedded inside another
* protocol that is being extended to support micropayments. In this file "primary" typically can be read as "client"
* and "secondary" as "server".
*
*/
public static final class TwoWayChannelMessage extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.TwoWayChannelMessage)
TwoWayChannelMessageOrBuilder {
// Use TwoWayChannelMessage.newBuilder() to construct.
private TwoWayChannelMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TwoWayChannelMessage(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TwoWayChannelMessage defaultInstance;
public static TwoWayChannelMessage getDefaultInstance() {
return defaultInstance;
}
public TwoWayChannelMessage getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TwoWayChannelMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType value = org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = value;
}
break;
}
case 18: {
org.bitcoin.paymentchannel.Protos.ClientVersion.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = clientVersion_.toBuilder();
}
clientVersion_ = input.readMessage(org.bitcoin.paymentchannel.Protos.ClientVersion.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientVersion_);
clientVersion_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.bitcoin.paymentchannel.Protos.ServerVersion.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = serverVersion_.toBuilder();
}
serverVersion_ = input.readMessage(org.bitcoin.paymentchannel.Protos.ServerVersion.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(serverVersion_);
serverVersion_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
org.bitcoin.paymentchannel.Protos.Initiate.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = initiate_.toBuilder();
}
initiate_ = input.readMessage(org.bitcoin.paymentchannel.Protos.Initiate.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(initiate_);
initiate_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = provideRefund_.toBuilder();
}
provideRefund_ = input.readMessage(org.bitcoin.paymentchannel.Protos.ProvideRefund.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(provideRefund_);
provideRefund_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 50: {
org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = returnRefund_.toBuilder();
}
returnRefund_ = input.readMessage(org.bitcoin.paymentchannel.Protos.ReturnRefund.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(returnRefund_);
returnRefund_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 58: {
org.bitcoin.paymentchannel.Protos.ProvideContract.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = provideContract_.toBuilder();
}
provideContract_ = input.readMessage(org.bitcoin.paymentchannel.Protos.ProvideContract.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(provideContract_);
provideContract_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = updatePayment_.toBuilder();
}
updatePayment_ = input.readMessage(org.bitcoin.paymentchannel.Protos.UpdatePayment.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(updatePayment_);
updatePayment_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 74: {
org.bitcoin.paymentchannel.Protos.Settlement.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
subBuilder = settlement_.toBuilder();
}
settlement_ = input.readMessage(org.bitcoin.paymentchannel.Protos.Settlement.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(settlement_);
settlement_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 82: {
org.bitcoin.paymentchannel.Protos.Error.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) == 0x00000400)) {
subBuilder = error_.toBuilder();
}
error_ = input.readMessage(org.bitcoin.paymentchannel.Protos.Error.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(error_);
error_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
case 90: {
org.bitcoin.paymentchannel.Protos.PaymentAck.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = paymentAck_.toBuilder();
}
paymentAck_ = input.readMessage(org.bitcoin.paymentchannel.Protos.PaymentAck.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(paymentAck_);
paymentAck_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_TwoWayChannelMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_TwoWayChannelMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.class, org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TwoWayChannelMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TwoWayChannelMessage(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code paymentchannels.TwoWayChannelMessage.MessageType}
*/
public enum MessageType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* CLIENT_VERSION = 1;
*/
CLIENT_VERSION(0, 1),
/**
* SERVER_VERSION = 2;
*/
SERVER_VERSION(1, 2),
/**
* INITIATE = 3;
*/
INITIATE(2, 3),
/**
* PROVIDE_REFUND = 4;
*/
PROVIDE_REFUND(3, 4),
/**
* RETURN_REFUND = 5;
*/
RETURN_REFUND(4, 5),
/**
* PROVIDE_CONTRACT = 6;
*/
PROVIDE_CONTRACT(5, 6),
/**
* CHANNEL_OPEN = 7;
*
*
* Note that there are no optional fields set for CHANNEL_OPEN, it is sent from the
* secondary to the primary to indicate that the provided contract was received,
* verified, and broadcast successfully and the primary can now provide UPDATE messages
* at will to begin paying secondary. If the channel is interrupted after the
* CHANNEL_OPEN message (ie closed without an explicit CLOSE or ERROR) the primary may
* reopen the channel by setting the contract transaction hash in its CLIENT_VERSION
* message.
*
*/
CHANNEL_OPEN(6, 7),
/**
* UPDATE_PAYMENT = 8;
*/
UPDATE_PAYMENT(7, 8),
/**
* PAYMENT_ACK = 11;
*
*
* Sent by the server to the client after an UPDATE_PAYMENT message is successfully processed.
*
*/
PAYMENT_ACK(8, 11),
/**
* CLOSE = 9;
*
*
* Either side can send this message. If the client sends it to the server, then the server
* takes the most recent signature it received in an UPDATE_PAYMENT and uses it to create a
* valid transaction, which it then broadcasts on the network.
* Once broadcast is complete, it sends back another CLOSE message with the settlement field set, containing
* the final state of the contract.
* The server is allowed to initiate settlement whenever it wants, in which case the client will
* asynchronously receive a CLOSE message with the settlement field set. The server is also allowed
* to send a CLOSE to mark the end of a connection without any settlement taking place, in which
* case this is just an equivalent to a TCP FIN packet. An explicit end-of-protocol markers can be
* useful when this protocol is embedded inside another.
*
*/
CLOSE(9, 9),
/**
* ERROR = 10;
*
*
* Used to indicate an error condition.
* Both parties should make an effort to send either an ERROR or a CLOSE immediately
* before closing the socket (unless they just received an ERROR or a CLOSE). This is important
* because the protocol may not run over TCP.
*
*/
ERROR(10, 10),
;
/**
* CLIENT_VERSION = 1;
*/
public static final int CLIENT_VERSION_VALUE = 1;
/**
* SERVER_VERSION = 2;
*/
public static final int SERVER_VERSION_VALUE = 2;
/**
* INITIATE = 3;
*/
public static final int INITIATE_VALUE = 3;
/**
* PROVIDE_REFUND = 4;
*/
public static final int PROVIDE_REFUND_VALUE = 4;
/**
* RETURN_REFUND = 5;
*/
public static final int RETURN_REFUND_VALUE = 5;
/**
* PROVIDE_CONTRACT = 6;
*/
public static final int PROVIDE_CONTRACT_VALUE = 6;
/**
* CHANNEL_OPEN = 7;
*
*
* Note that there are no optional fields set for CHANNEL_OPEN, it is sent from the
* secondary to the primary to indicate that the provided contract was received,
* verified, and broadcast successfully and the primary can now provide UPDATE messages
* at will to begin paying secondary. If the channel is interrupted after the
* CHANNEL_OPEN message (ie closed without an explicit CLOSE or ERROR) the primary may
* reopen the channel by setting the contract transaction hash in its CLIENT_VERSION
* message.
*
*/
public static final int CHANNEL_OPEN_VALUE = 7;
/**
* UPDATE_PAYMENT = 8;
*/
public static final int UPDATE_PAYMENT_VALUE = 8;
/**
* PAYMENT_ACK = 11;
*
*
* Sent by the server to the client after an UPDATE_PAYMENT message is successfully processed.
*
*/
public static final int PAYMENT_ACK_VALUE = 11;
/**
* CLOSE = 9;
*
*
* Either side can send this message. If the client sends it to the server, then the server
* takes the most recent signature it received in an UPDATE_PAYMENT and uses it to create a
* valid transaction, which it then broadcasts on the network.
* Once broadcast is complete, it sends back another CLOSE message with the settlement field set, containing
* the final state of the contract.
* The server is allowed to initiate settlement whenever it wants, in which case the client will
* asynchronously receive a CLOSE message with the settlement field set. The server is also allowed
* to send a CLOSE to mark the end of a connection without any settlement taking place, in which
* case this is just an equivalent to a TCP FIN packet. An explicit end-of-protocol markers can be
* useful when this protocol is embedded inside another.
*
*/
public static final int CLOSE_VALUE = 9;
/**
* ERROR = 10;
*
*
* Used to indicate an error condition.
* Both parties should make an effort to send either an ERROR or a CLOSE immediately
* before closing the socket (unless they just received an ERROR or a CLOSE). This is important
* because the protocol may not run over TCP.
*
*/
public static final int ERROR_VALUE = 10;
public final int getNumber() { return value; }
public static MessageType valueOf(int value) {
switch (value) {
case 1: return CLIENT_VERSION;
case 2: return SERVER_VERSION;
case 3: return INITIATE;
case 4: return PROVIDE_REFUND;
case 5: return RETURN_REFUND;
case 6: return PROVIDE_CONTRACT;
case 7: return CHANNEL_OPEN;
case 8: return UPDATE_PAYMENT;
case 11: return PAYMENT_ACK;
case 9: return CLOSE;
case 10: return ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MessageType findValueByNumber(int number) {
return MessageType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.getDescriptor().getEnumTypes().get(0);
}
private static final MessageType[] VALUES = values();
public static MessageType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private MessageType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:paymentchannels.TwoWayChannelMessage.MessageType)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType type_;
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
public org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType getType() {
return type_;
}
public static final int CLIENT_VERSION_FIELD_NUMBER = 2;
private org.bitcoin.paymentchannel.Protos.ClientVersion clientVersion_;
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public boolean hasClientVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public org.bitcoin.paymentchannel.Protos.ClientVersion getClientVersion() {
return clientVersion_;
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder getClientVersionOrBuilder() {
return clientVersion_;
}
public static final int SERVER_VERSION_FIELD_NUMBER = 3;
private org.bitcoin.paymentchannel.Protos.ServerVersion serverVersion_;
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public boolean hasServerVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public org.bitcoin.paymentchannel.Protos.ServerVersion getServerVersion() {
return serverVersion_;
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder getServerVersionOrBuilder() {
return serverVersion_;
}
public static final int INITIATE_FIELD_NUMBER = 4;
private org.bitcoin.paymentchannel.Protos.Initiate initiate_;
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public boolean hasInitiate() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public org.bitcoin.paymentchannel.Protos.Initiate getInitiate() {
return initiate_;
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public org.bitcoin.paymentchannel.Protos.InitiateOrBuilder getInitiateOrBuilder() {
return initiate_;
}
public static final int PROVIDE_REFUND_FIELD_NUMBER = 5;
private org.bitcoin.paymentchannel.Protos.ProvideRefund provideRefund_;
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public boolean hasProvideRefund() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public org.bitcoin.paymentchannel.Protos.ProvideRefund getProvideRefund() {
return provideRefund_;
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder getProvideRefundOrBuilder() {
return provideRefund_;
}
public static final int RETURN_REFUND_FIELD_NUMBER = 6;
private org.bitcoin.paymentchannel.Protos.ReturnRefund returnRefund_;
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public boolean hasReturnRefund() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public org.bitcoin.paymentchannel.Protos.ReturnRefund getReturnRefund() {
return returnRefund_;
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder getReturnRefundOrBuilder() {
return returnRefund_;
}
public static final int PROVIDE_CONTRACT_FIELD_NUMBER = 7;
private org.bitcoin.paymentchannel.Protos.ProvideContract provideContract_;
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public boolean hasProvideContract() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public org.bitcoin.paymentchannel.Protos.ProvideContract getProvideContract() {
return provideContract_;
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder getProvideContractOrBuilder() {
return provideContract_;
}
public static final int UPDATE_PAYMENT_FIELD_NUMBER = 8;
private org.bitcoin.paymentchannel.Protos.UpdatePayment updatePayment_;
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public boolean hasUpdatePayment() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public org.bitcoin.paymentchannel.Protos.UpdatePayment getUpdatePayment() {
return updatePayment_;
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder getUpdatePaymentOrBuilder() {
return updatePayment_;
}
public static final int PAYMENT_ACK_FIELD_NUMBER = 11;
private org.bitcoin.paymentchannel.Protos.PaymentAck paymentAck_;
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public boolean hasPaymentAck() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public org.bitcoin.paymentchannel.Protos.PaymentAck getPaymentAck() {
return paymentAck_;
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder getPaymentAckOrBuilder() {
return paymentAck_;
}
public static final int SETTLEMENT_FIELD_NUMBER = 9;
private org.bitcoin.paymentchannel.Protos.Settlement settlement_;
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public boolean hasSettlement() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public org.bitcoin.paymentchannel.Protos.Settlement getSettlement() {
return settlement_;
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public org.bitcoin.paymentchannel.Protos.SettlementOrBuilder getSettlementOrBuilder() {
return settlement_;
}
public static final int ERROR_FIELD_NUMBER = 10;
private org.bitcoin.paymentchannel.Protos.Error error_;
/**
* optional .paymentchannels.Error error = 10;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .paymentchannels.Error error = 10;
*/
public org.bitcoin.paymentchannel.Protos.Error getError() {
return error_;
}
/**
* optional .paymentchannels.Error error = 10;
*/
public org.bitcoin.paymentchannel.Protos.ErrorOrBuilder getErrorOrBuilder() {
return error_;
}
private void initFields() {
type_ = org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType.CLIENT_VERSION;
clientVersion_ = org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance();
serverVersion_ = org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance();
initiate_ = org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance();
provideRefund_ = org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance();
returnRefund_ = org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance();
provideContract_ = org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance();
updatePayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
paymentAck_ = org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance();
settlement_ = org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance();
error_ = org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (hasClientVersion()) {
if (!getClientVersion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasServerVersion()) {
if (!getServerVersion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasInitiate()) {
if (!getInitiate().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasProvideRefund()) {
if (!getProvideRefund().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasReturnRefund()) {
if (!getReturnRefund().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasProvideContract()) {
if (!getProvideContract().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasUpdatePayment()) {
if (!getUpdatePayment().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasSettlement()) {
if (!getSettlement().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, type_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, clientVersion_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, serverVersion_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, initiate_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, provideRefund_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, returnRefund_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, provideContract_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeMessage(8, updatePayment_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeMessage(9, settlement_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeMessage(10, error_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(11, paymentAck_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, clientVersion_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, serverVersion_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, initiate_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, provideRefund_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, returnRefund_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, provideContract_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, updatePayment_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, settlement_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, error_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, paymentAck_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.TwoWayChannelMessage}
*
*
* This message is designed to be either sent raw over the network (e.g. length prefixed) or embedded inside another
* protocol that is being extended to support micropayments. In this file "primary" typically can be read as "client"
* and "secondary" as "server".
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.TwoWayChannelMessage)
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_TwoWayChannelMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_TwoWayChannelMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.class, org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getClientVersionFieldBuilder();
getServerVersionFieldBuilder();
getInitiateFieldBuilder();
getProvideRefundFieldBuilder();
getReturnRefundFieldBuilder();
getProvideContractFieldBuilder();
getUpdatePaymentFieldBuilder();
getPaymentAckFieldBuilder();
getSettlementFieldBuilder();
getErrorFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
type_ = org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType.CLIENT_VERSION;
bitField0_ = (bitField0_ & ~0x00000001);
if (clientVersionBuilder_ == null) {
clientVersion_ = org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance();
} else {
clientVersionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (serverVersionBuilder_ == null) {
serverVersion_ = org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance();
} else {
serverVersionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (initiateBuilder_ == null) {
initiate_ = org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance();
} else {
initiateBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (provideRefundBuilder_ == null) {
provideRefund_ = org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance();
} else {
provideRefundBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (returnRefundBuilder_ == null) {
returnRefund_ = org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance();
} else {
returnRefundBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (provideContractBuilder_ == null) {
provideContract_ = org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance();
} else {
provideContractBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (updatePaymentBuilder_ == null) {
updatePayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
} else {
updatePaymentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (paymentAckBuilder_ == null) {
paymentAck_ = org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance();
} else {
paymentAckBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
if (settlementBuilder_ == null) {
settlement_ = org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance();
} else {
settlementBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (errorBuilder_ == null) {
error_ = org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_TwoWayChannelMessage_descriptor;
}
public org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage build() {
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage buildPartial() {
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage result = new org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (clientVersionBuilder_ == null) {
result.clientVersion_ = clientVersion_;
} else {
result.clientVersion_ = clientVersionBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (serverVersionBuilder_ == null) {
result.serverVersion_ = serverVersion_;
} else {
result.serverVersion_ = serverVersionBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (initiateBuilder_ == null) {
result.initiate_ = initiate_;
} else {
result.initiate_ = initiateBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (provideRefundBuilder_ == null) {
result.provideRefund_ = provideRefund_;
} else {
result.provideRefund_ = provideRefundBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (returnRefundBuilder_ == null) {
result.returnRefund_ = returnRefund_;
} else {
result.returnRefund_ = returnRefundBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (provideContractBuilder_ == null) {
result.provideContract_ = provideContract_;
} else {
result.provideContract_ = provideContractBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
if (updatePaymentBuilder_ == null) {
result.updatePayment_ = updatePayment_;
} else {
result.updatePayment_ = updatePaymentBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
if (paymentAckBuilder_ == null) {
result.paymentAck_ = paymentAck_;
} else {
result.paymentAck_ = paymentAckBuilder_.build();
}
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
if (settlementBuilder_ == null) {
result.settlement_ = settlement_;
} else {
result.settlement_ = settlementBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
if (errorBuilder_ == null) {
result.error_ = error_;
} else {
result.error_ = errorBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage other) {
if (other == org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasClientVersion()) {
mergeClientVersion(other.getClientVersion());
}
if (other.hasServerVersion()) {
mergeServerVersion(other.getServerVersion());
}
if (other.hasInitiate()) {
mergeInitiate(other.getInitiate());
}
if (other.hasProvideRefund()) {
mergeProvideRefund(other.getProvideRefund());
}
if (other.hasReturnRefund()) {
mergeReturnRefund(other.getReturnRefund());
}
if (other.hasProvideContract()) {
mergeProvideContract(other.getProvideContract());
}
if (other.hasUpdatePayment()) {
mergeUpdatePayment(other.getUpdatePayment());
}
if (other.hasPaymentAck()) {
mergePaymentAck(other.getPaymentAck());
}
if (other.hasSettlement()) {
mergeSettlement(other.getSettlement());
}
if (other.hasError()) {
mergeError(other.getError());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
if (hasClientVersion()) {
if (!getClientVersion().isInitialized()) {
return false;
}
}
if (hasServerVersion()) {
if (!getServerVersion().isInitialized()) {
return false;
}
}
if (hasInitiate()) {
if (!getInitiate().isInitialized()) {
return false;
}
}
if (hasProvideRefund()) {
if (!getProvideRefund().isInitialized()) {
return false;
}
}
if (hasReturnRefund()) {
if (!getReturnRefund().isInitialized()) {
return false;
}
}
if (hasProvideContract()) {
if (!getProvideContract().isInitialized()) {
return false;
}
}
if (hasUpdatePayment()) {
if (!getUpdatePayment().isInitialized()) {
return false;
}
}
if (hasSettlement()) {
if (!getSettlement().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType type_ = org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType.CLIENT_VERSION;
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
public org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType getType() {
return type_;
}
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
public Builder setType(org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value;
onChanged();
return this;
}
/**
* required .paymentchannels.TwoWayChannelMessage.MessageType type = 1;
*
*
* This is required so if a new message type is added in future, old software aborts trying
* to read the message as early as possible. If the message doesn't parse, the socket should
* be closed.
*
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = org.bitcoin.paymentchannel.Protos.TwoWayChannelMessage.MessageType.CLIENT_VERSION;
onChanged();
return this;
}
private org.bitcoin.paymentchannel.Protos.ClientVersion clientVersion_ = org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ClientVersion, org.bitcoin.paymentchannel.Protos.ClientVersion.Builder, org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder> clientVersionBuilder_;
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public boolean hasClientVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public org.bitcoin.paymentchannel.Protos.ClientVersion getClientVersion() {
if (clientVersionBuilder_ == null) {
return clientVersion_;
} else {
return clientVersionBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public Builder setClientVersion(org.bitcoin.paymentchannel.Protos.ClientVersion value) {
if (clientVersionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientVersion_ = value;
onChanged();
} else {
clientVersionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public Builder setClientVersion(
org.bitcoin.paymentchannel.Protos.ClientVersion.Builder builderForValue) {
if (clientVersionBuilder_ == null) {
clientVersion_ = builderForValue.build();
onChanged();
} else {
clientVersionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public Builder mergeClientVersion(org.bitcoin.paymentchannel.Protos.ClientVersion value) {
if (clientVersionBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
clientVersion_ != org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance()) {
clientVersion_ =
org.bitcoin.paymentchannel.Protos.ClientVersion.newBuilder(clientVersion_).mergeFrom(value).buildPartial();
} else {
clientVersion_ = value;
}
onChanged();
} else {
clientVersionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public Builder clearClientVersion() {
if (clientVersionBuilder_ == null) {
clientVersion_ = org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance();
onChanged();
} else {
clientVersionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public org.bitcoin.paymentchannel.Protos.ClientVersion.Builder getClientVersionBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getClientVersionFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
public org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder getClientVersionOrBuilder() {
if (clientVersionBuilder_ != null) {
return clientVersionBuilder_.getMessageOrBuilder();
} else {
return clientVersion_;
}
}
/**
* optional .paymentchannels.ClientVersion client_version = 2;
*
*
* Now one optional field for each message. Only the field specified by type should be read.
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ClientVersion, org.bitcoin.paymentchannel.Protos.ClientVersion.Builder, org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder>
getClientVersionFieldBuilder() {
if (clientVersionBuilder_ == null) {
clientVersionBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ClientVersion, org.bitcoin.paymentchannel.Protos.ClientVersion.Builder, org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder>(
getClientVersion(),
getParentForChildren(),
isClean());
clientVersion_ = null;
}
return clientVersionBuilder_;
}
private org.bitcoin.paymentchannel.Protos.ServerVersion serverVersion_ = org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ServerVersion, org.bitcoin.paymentchannel.Protos.ServerVersion.Builder, org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder> serverVersionBuilder_;
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public boolean hasServerVersion() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public org.bitcoin.paymentchannel.Protos.ServerVersion getServerVersion() {
if (serverVersionBuilder_ == null) {
return serverVersion_;
} else {
return serverVersionBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public Builder setServerVersion(org.bitcoin.paymentchannel.Protos.ServerVersion value) {
if (serverVersionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
serverVersion_ = value;
onChanged();
} else {
serverVersionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public Builder setServerVersion(
org.bitcoin.paymentchannel.Protos.ServerVersion.Builder builderForValue) {
if (serverVersionBuilder_ == null) {
serverVersion_ = builderForValue.build();
onChanged();
} else {
serverVersionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public Builder mergeServerVersion(org.bitcoin.paymentchannel.Protos.ServerVersion value) {
if (serverVersionBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
serverVersion_ != org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance()) {
serverVersion_ =
org.bitcoin.paymentchannel.Protos.ServerVersion.newBuilder(serverVersion_).mergeFrom(value).buildPartial();
} else {
serverVersion_ = value;
}
onChanged();
} else {
serverVersionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public Builder clearServerVersion() {
if (serverVersionBuilder_ == null) {
serverVersion_ = org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance();
onChanged();
} else {
serverVersionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public org.bitcoin.paymentchannel.Protos.ServerVersion.Builder getServerVersionBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getServerVersionFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
public org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder getServerVersionOrBuilder() {
if (serverVersionBuilder_ != null) {
return serverVersionBuilder_.getMessageOrBuilder();
} else {
return serverVersion_;
}
}
/**
* optional .paymentchannels.ServerVersion server_version = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ServerVersion, org.bitcoin.paymentchannel.Protos.ServerVersion.Builder, org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder>
getServerVersionFieldBuilder() {
if (serverVersionBuilder_ == null) {
serverVersionBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ServerVersion, org.bitcoin.paymentchannel.Protos.ServerVersion.Builder, org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder>(
getServerVersion(),
getParentForChildren(),
isClean());
serverVersion_ = null;
}
return serverVersionBuilder_;
}
private org.bitcoin.paymentchannel.Protos.Initiate initiate_ = org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Initiate, org.bitcoin.paymentchannel.Protos.Initiate.Builder, org.bitcoin.paymentchannel.Protos.InitiateOrBuilder> initiateBuilder_;
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public boolean hasInitiate() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public org.bitcoin.paymentchannel.Protos.Initiate getInitiate() {
if (initiateBuilder_ == null) {
return initiate_;
} else {
return initiateBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public Builder setInitiate(org.bitcoin.paymentchannel.Protos.Initiate value) {
if (initiateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
initiate_ = value;
onChanged();
} else {
initiateBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public Builder setInitiate(
org.bitcoin.paymentchannel.Protos.Initiate.Builder builderForValue) {
if (initiateBuilder_ == null) {
initiate_ = builderForValue.build();
onChanged();
} else {
initiateBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public Builder mergeInitiate(org.bitcoin.paymentchannel.Protos.Initiate value) {
if (initiateBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
initiate_ != org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance()) {
initiate_ =
org.bitcoin.paymentchannel.Protos.Initiate.newBuilder(initiate_).mergeFrom(value).buildPartial();
} else {
initiate_ = value;
}
onChanged();
} else {
initiateBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public Builder clearInitiate() {
if (initiateBuilder_ == null) {
initiate_ = org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance();
onChanged();
} else {
initiateBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public org.bitcoin.paymentchannel.Protos.Initiate.Builder getInitiateBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getInitiateFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
public org.bitcoin.paymentchannel.Protos.InitiateOrBuilder getInitiateOrBuilder() {
if (initiateBuilder_ != null) {
return initiateBuilder_.getMessageOrBuilder();
} else {
return initiate_;
}
}
/**
* optional .paymentchannels.Initiate initiate = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Initiate, org.bitcoin.paymentchannel.Protos.Initiate.Builder, org.bitcoin.paymentchannel.Protos.InitiateOrBuilder>
getInitiateFieldBuilder() {
if (initiateBuilder_ == null) {
initiateBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Initiate, org.bitcoin.paymentchannel.Protos.Initiate.Builder, org.bitcoin.paymentchannel.Protos.InitiateOrBuilder>(
getInitiate(),
getParentForChildren(),
isClean());
initiate_ = null;
}
return initiateBuilder_;
}
private org.bitcoin.paymentchannel.Protos.ProvideRefund provideRefund_ = org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ProvideRefund, org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder, org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder> provideRefundBuilder_;
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public boolean hasProvideRefund() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public org.bitcoin.paymentchannel.Protos.ProvideRefund getProvideRefund() {
if (provideRefundBuilder_ == null) {
return provideRefund_;
} else {
return provideRefundBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public Builder setProvideRefund(org.bitcoin.paymentchannel.Protos.ProvideRefund value) {
if (provideRefundBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
provideRefund_ = value;
onChanged();
} else {
provideRefundBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public Builder setProvideRefund(
org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder builderForValue) {
if (provideRefundBuilder_ == null) {
provideRefund_ = builderForValue.build();
onChanged();
} else {
provideRefundBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public Builder mergeProvideRefund(org.bitcoin.paymentchannel.Protos.ProvideRefund value) {
if (provideRefundBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
provideRefund_ != org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance()) {
provideRefund_ =
org.bitcoin.paymentchannel.Protos.ProvideRefund.newBuilder(provideRefund_).mergeFrom(value).buildPartial();
} else {
provideRefund_ = value;
}
onChanged();
} else {
provideRefundBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public Builder clearProvideRefund() {
if (provideRefundBuilder_ == null) {
provideRefund_ = org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance();
onChanged();
} else {
provideRefundBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder getProvideRefundBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getProvideRefundFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
public org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder getProvideRefundOrBuilder() {
if (provideRefundBuilder_ != null) {
return provideRefundBuilder_.getMessageOrBuilder();
} else {
return provideRefund_;
}
}
/**
* optional .paymentchannels.ProvideRefund provide_refund = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ProvideRefund, org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder, org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder>
getProvideRefundFieldBuilder() {
if (provideRefundBuilder_ == null) {
provideRefundBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ProvideRefund, org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder, org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder>(
getProvideRefund(),
getParentForChildren(),
isClean());
provideRefund_ = null;
}
return provideRefundBuilder_;
}
private org.bitcoin.paymentchannel.Protos.ReturnRefund returnRefund_ = org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ReturnRefund, org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder, org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder> returnRefundBuilder_;
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public boolean hasReturnRefund() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public org.bitcoin.paymentchannel.Protos.ReturnRefund getReturnRefund() {
if (returnRefundBuilder_ == null) {
return returnRefund_;
} else {
return returnRefundBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public Builder setReturnRefund(org.bitcoin.paymentchannel.Protos.ReturnRefund value) {
if (returnRefundBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
returnRefund_ = value;
onChanged();
} else {
returnRefundBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public Builder setReturnRefund(
org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder builderForValue) {
if (returnRefundBuilder_ == null) {
returnRefund_ = builderForValue.build();
onChanged();
} else {
returnRefundBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public Builder mergeReturnRefund(org.bitcoin.paymentchannel.Protos.ReturnRefund value) {
if (returnRefundBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
returnRefund_ != org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance()) {
returnRefund_ =
org.bitcoin.paymentchannel.Protos.ReturnRefund.newBuilder(returnRefund_).mergeFrom(value).buildPartial();
} else {
returnRefund_ = value;
}
onChanged();
} else {
returnRefundBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public Builder clearReturnRefund() {
if (returnRefundBuilder_ == null) {
returnRefund_ = org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance();
onChanged();
} else {
returnRefundBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder getReturnRefundBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getReturnRefundFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
public org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder getReturnRefundOrBuilder() {
if (returnRefundBuilder_ != null) {
return returnRefundBuilder_.getMessageOrBuilder();
} else {
return returnRefund_;
}
}
/**
* optional .paymentchannels.ReturnRefund return_refund = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ReturnRefund, org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder, org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder>
getReturnRefundFieldBuilder() {
if (returnRefundBuilder_ == null) {
returnRefundBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ReturnRefund, org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder, org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder>(
getReturnRefund(),
getParentForChildren(),
isClean());
returnRefund_ = null;
}
return returnRefundBuilder_;
}
private org.bitcoin.paymentchannel.Protos.ProvideContract provideContract_ = org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ProvideContract, org.bitcoin.paymentchannel.Protos.ProvideContract.Builder, org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder> provideContractBuilder_;
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public boolean hasProvideContract() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public org.bitcoin.paymentchannel.Protos.ProvideContract getProvideContract() {
if (provideContractBuilder_ == null) {
return provideContract_;
} else {
return provideContractBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public Builder setProvideContract(org.bitcoin.paymentchannel.Protos.ProvideContract value) {
if (provideContractBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
provideContract_ = value;
onChanged();
} else {
provideContractBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public Builder setProvideContract(
org.bitcoin.paymentchannel.Protos.ProvideContract.Builder builderForValue) {
if (provideContractBuilder_ == null) {
provideContract_ = builderForValue.build();
onChanged();
} else {
provideContractBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public Builder mergeProvideContract(org.bitcoin.paymentchannel.Protos.ProvideContract value) {
if (provideContractBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
provideContract_ != org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance()) {
provideContract_ =
org.bitcoin.paymentchannel.Protos.ProvideContract.newBuilder(provideContract_).mergeFrom(value).buildPartial();
} else {
provideContract_ = value;
}
onChanged();
} else {
provideContractBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public Builder clearProvideContract() {
if (provideContractBuilder_ == null) {
provideContract_ = org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance();
onChanged();
} else {
provideContractBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public org.bitcoin.paymentchannel.Protos.ProvideContract.Builder getProvideContractBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getProvideContractFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
public org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder getProvideContractOrBuilder() {
if (provideContractBuilder_ != null) {
return provideContractBuilder_.getMessageOrBuilder();
} else {
return provideContract_;
}
}
/**
* optional .paymentchannels.ProvideContract provide_contract = 7;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ProvideContract, org.bitcoin.paymentchannel.Protos.ProvideContract.Builder, org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder>
getProvideContractFieldBuilder() {
if (provideContractBuilder_ == null) {
provideContractBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.ProvideContract, org.bitcoin.paymentchannel.Protos.ProvideContract.Builder, org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder>(
getProvideContract(),
getParentForChildren(),
isClean());
provideContract_ = null;
}
return provideContractBuilder_;
}
private org.bitcoin.paymentchannel.Protos.UpdatePayment updatePayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.UpdatePayment, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder, org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder> updatePaymentBuilder_;
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public boolean hasUpdatePayment() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public org.bitcoin.paymentchannel.Protos.UpdatePayment getUpdatePayment() {
if (updatePaymentBuilder_ == null) {
return updatePayment_;
} else {
return updatePaymentBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public Builder setUpdatePayment(org.bitcoin.paymentchannel.Protos.UpdatePayment value) {
if (updatePaymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updatePayment_ = value;
onChanged();
} else {
updatePaymentBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public Builder setUpdatePayment(
org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder builderForValue) {
if (updatePaymentBuilder_ == null) {
updatePayment_ = builderForValue.build();
onChanged();
} else {
updatePaymentBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public Builder mergeUpdatePayment(org.bitcoin.paymentchannel.Protos.UpdatePayment value) {
if (updatePaymentBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080) &&
updatePayment_ != org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance()) {
updatePayment_ =
org.bitcoin.paymentchannel.Protos.UpdatePayment.newBuilder(updatePayment_).mergeFrom(value).buildPartial();
} else {
updatePayment_ = value;
}
onChanged();
} else {
updatePaymentBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public Builder clearUpdatePayment() {
if (updatePaymentBuilder_ == null) {
updatePayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
onChanged();
} else {
updatePaymentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder getUpdatePaymentBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getUpdatePaymentFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
public org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder getUpdatePaymentOrBuilder() {
if (updatePaymentBuilder_ != null) {
return updatePaymentBuilder_.getMessageOrBuilder();
} else {
return updatePayment_;
}
}
/**
* optional .paymentchannels.UpdatePayment update_payment = 8;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.UpdatePayment, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder, org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder>
getUpdatePaymentFieldBuilder() {
if (updatePaymentBuilder_ == null) {
updatePaymentBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.UpdatePayment, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder, org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder>(
getUpdatePayment(),
getParentForChildren(),
isClean());
updatePayment_ = null;
}
return updatePaymentBuilder_;
}
private org.bitcoin.paymentchannel.Protos.PaymentAck paymentAck_ = org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.PaymentAck, org.bitcoin.paymentchannel.Protos.PaymentAck.Builder, org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder> paymentAckBuilder_;
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public boolean hasPaymentAck() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public org.bitcoin.paymentchannel.Protos.PaymentAck getPaymentAck() {
if (paymentAckBuilder_ == null) {
return paymentAck_;
} else {
return paymentAckBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public Builder setPaymentAck(org.bitcoin.paymentchannel.Protos.PaymentAck value) {
if (paymentAckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
paymentAck_ = value;
onChanged();
} else {
paymentAckBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public Builder setPaymentAck(
org.bitcoin.paymentchannel.Protos.PaymentAck.Builder builderForValue) {
if (paymentAckBuilder_ == null) {
paymentAck_ = builderForValue.build();
onChanged();
} else {
paymentAckBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public Builder mergePaymentAck(org.bitcoin.paymentchannel.Protos.PaymentAck value) {
if (paymentAckBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
paymentAck_ != org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance()) {
paymentAck_ =
org.bitcoin.paymentchannel.Protos.PaymentAck.newBuilder(paymentAck_).mergeFrom(value).buildPartial();
} else {
paymentAck_ = value;
}
onChanged();
} else {
paymentAckBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public Builder clearPaymentAck() {
if (paymentAckBuilder_ == null) {
paymentAck_ = org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance();
onChanged();
} else {
paymentAckBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public org.bitcoin.paymentchannel.Protos.PaymentAck.Builder getPaymentAckBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getPaymentAckFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
public org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder getPaymentAckOrBuilder() {
if (paymentAckBuilder_ != null) {
return paymentAckBuilder_.getMessageOrBuilder();
} else {
return paymentAck_;
}
}
/**
* optional .paymentchannels.PaymentAck payment_ack = 11;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.PaymentAck, org.bitcoin.paymentchannel.Protos.PaymentAck.Builder, org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder>
getPaymentAckFieldBuilder() {
if (paymentAckBuilder_ == null) {
paymentAckBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.PaymentAck, org.bitcoin.paymentchannel.Protos.PaymentAck.Builder, org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder>(
getPaymentAck(),
getParentForChildren(),
isClean());
paymentAck_ = null;
}
return paymentAckBuilder_;
}
private org.bitcoin.paymentchannel.Protos.Settlement settlement_ = org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Settlement, org.bitcoin.paymentchannel.Protos.Settlement.Builder, org.bitcoin.paymentchannel.Protos.SettlementOrBuilder> settlementBuilder_;
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public boolean hasSettlement() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public org.bitcoin.paymentchannel.Protos.Settlement getSettlement() {
if (settlementBuilder_ == null) {
return settlement_;
} else {
return settlementBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public Builder setSettlement(org.bitcoin.paymentchannel.Protos.Settlement value) {
if (settlementBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
settlement_ = value;
onChanged();
} else {
settlementBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public Builder setSettlement(
org.bitcoin.paymentchannel.Protos.Settlement.Builder builderForValue) {
if (settlementBuilder_ == null) {
settlement_ = builderForValue.build();
onChanged();
} else {
settlementBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public Builder mergeSettlement(org.bitcoin.paymentchannel.Protos.Settlement value) {
if (settlementBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
settlement_ != org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance()) {
settlement_ =
org.bitcoin.paymentchannel.Protos.Settlement.newBuilder(settlement_).mergeFrom(value).buildPartial();
} else {
settlement_ = value;
}
onChanged();
} else {
settlementBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public Builder clearSettlement() {
if (settlementBuilder_ == null) {
settlement_ = org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance();
onChanged();
} else {
settlementBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public org.bitcoin.paymentchannel.Protos.Settlement.Builder getSettlementBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getSettlementFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
public org.bitcoin.paymentchannel.Protos.SettlementOrBuilder getSettlementOrBuilder() {
if (settlementBuilder_ != null) {
return settlementBuilder_.getMessageOrBuilder();
} else {
return settlement_;
}
}
/**
* optional .paymentchannels.Settlement settlement = 9;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Settlement, org.bitcoin.paymentchannel.Protos.Settlement.Builder, org.bitcoin.paymentchannel.Protos.SettlementOrBuilder>
getSettlementFieldBuilder() {
if (settlementBuilder_ == null) {
settlementBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Settlement, org.bitcoin.paymentchannel.Protos.Settlement.Builder, org.bitcoin.paymentchannel.Protos.SettlementOrBuilder>(
getSettlement(),
getParentForChildren(),
isClean());
settlement_ = null;
}
return settlementBuilder_;
}
private org.bitcoin.paymentchannel.Protos.Error error_ = org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Error, org.bitcoin.paymentchannel.Protos.Error.Builder, org.bitcoin.paymentchannel.Protos.ErrorOrBuilder> errorBuilder_;
/**
* optional .paymentchannels.Error error = 10;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .paymentchannels.Error error = 10;
*/
public org.bitcoin.paymentchannel.Protos.Error getError() {
if (errorBuilder_ == null) {
return error_;
} else {
return errorBuilder_.getMessage();
}
}
/**
* optional .paymentchannels.Error error = 10;
*/
public Builder setError(org.bitcoin.paymentchannel.Protos.Error value) {
if (errorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
error_ = value;
onChanged();
} else {
errorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .paymentchannels.Error error = 10;
*/
public Builder setError(
org.bitcoin.paymentchannel.Protos.Error.Builder builderForValue) {
if (errorBuilder_ == null) {
error_ = builderForValue.build();
onChanged();
} else {
errorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .paymentchannels.Error error = 10;
*/
public Builder mergeError(org.bitcoin.paymentchannel.Protos.Error value) {
if (errorBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
error_ != org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance()) {
error_ =
org.bitcoin.paymentchannel.Protos.Error.newBuilder(error_).mergeFrom(value).buildPartial();
} else {
error_ = value;
}
onChanged();
} else {
errorBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .paymentchannels.Error error = 10;
*/
public Builder clearError() {
if (errorBuilder_ == null) {
error_ = org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance();
onChanged();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .paymentchannels.Error error = 10;
*/
public org.bitcoin.paymentchannel.Protos.Error.Builder getErrorBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getErrorFieldBuilder().getBuilder();
}
/**
* optional .paymentchannels.Error error = 10;
*/
public org.bitcoin.paymentchannel.Protos.ErrorOrBuilder getErrorOrBuilder() {
if (errorBuilder_ != null) {
return errorBuilder_.getMessageOrBuilder();
} else {
return error_;
}
}
/**
* optional .paymentchannels.Error error = 10;
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Error, org.bitcoin.paymentchannel.Protos.Error.Builder, org.bitcoin.paymentchannel.Protos.ErrorOrBuilder>
getErrorFieldBuilder() {
if (errorBuilder_ == null) {
errorBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.Error, org.bitcoin.paymentchannel.Protos.Error.Builder, org.bitcoin.paymentchannel.Protos.ErrorOrBuilder>(
getError(),
getParentForChildren(),
isClean());
error_ = null;
}
return errorBuilder_;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.TwoWayChannelMessage)
}
static {
defaultInstance = new TwoWayChannelMessage(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.TwoWayChannelMessage)
}
public interface ClientVersionOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.ClientVersion)
com.google.protobuf.MessageOrBuilder {
/**
* required int32 major = 1;
*/
boolean hasMajor();
/**
* required int32 major = 1;
*/
int getMajor();
/**
* optional int32 minor = 2 [default = 0];
*/
boolean hasMinor();
/**
* optional int32 minor = 2 [default = 0];
*/
int getMinor();
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
boolean hasPreviousChannelContractHash();
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
com.google.protobuf.ByteString getPreviousChannelContractHash();
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
boolean hasTimeWindowSecs();
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
long getTimeWindowSecs();
}
/**
* Protobuf type {@code paymentchannels.ClientVersion}
*
*
* Sent by primary to secondary on opening the connection. If anything is received before this is
* sent, the socket is closed.
*
*/
public static final class ClientVersion extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.ClientVersion)
ClientVersionOrBuilder {
// Use ClientVersion.newBuilder() to construct.
private ClientVersion(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ClientVersion(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ClientVersion defaultInstance;
public static ClientVersion getDefaultInstance() {
return defaultInstance;
}
public ClientVersion getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ClientVersion(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
major_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
minor_ = input.readInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
previousChannelContractHash_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
timeWindowSecs_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ClientVersion_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ClientVersion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ClientVersion.class, org.bitcoin.paymentchannel.Protos.ClientVersion.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ClientVersion parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ClientVersion(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int MAJOR_FIELD_NUMBER = 1;
private int major_;
/**
* required int32 major = 1;
*/
public boolean hasMajor() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 major = 1;
*/
public int getMajor() {
return major_;
}
public static final int MINOR_FIELD_NUMBER = 2;
private int minor_;
/**
* optional int32 minor = 2 [default = 0];
*/
public boolean hasMinor() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 minor = 2 [default = 0];
*/
public int getMinor() {
return minor_;
}
public static final int PREVIOUS_CHANNEL_CONTRACT_HASH_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString previousChannelContractHash_;
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
public boolean hasPreviousChannelContractHash() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
public com.google.protobuf.ByteString getPreviousChannelContractHash() {
return previousChannelContractHash_;
}
public static final int TIME_WINDOW_SECS_FIELD_NUMBER = 4;
private long timeWindowSecs_;
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
public boolean hasTimeWindowSecs() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
public long getTimeWindowSecs() {
return timeWindowSecs_;
}
private void initFields() {
major_ = 0;
minor_ = 0;
previousChannelContractHash_ = com.google.protobuf.ByteString.EMPTY;
timeWindowSecs_ = 86340L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasMajor()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, major_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, minor_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, previousChannelContractHash_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt64(4, timeWindowSecs_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, major_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, minor_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, previousChannelContractHash_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, timeWindowSecs_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ClientVersion parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.ClientVersion prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.ClientVersion}
*
*
* Sent by primary to secondary on opening the connection. If anything is received before this is
* sent, the socket is closed.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.ClientVersion)
org.bitcoin.paymentchannel.Protos.ClientVersionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ClientVersion_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ClientVersion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ClientVersion.class, org.bitcoin.paymentchannel.Protos.ClientVersion.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.ClientVersion.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
major_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
minor_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
previousChannelContractHash_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
timeWindowSecs_ = 86340L;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ClientVersion_descriptor;
}
public org.bitcoin.paymentchannel.Protos.ClientVersion getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.ClientVersion build() {
org.bitcoin.paymentchannel.Protos.ClientVersion result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.ClientVersion buildPartial() {
org.bitcoin.paymentchannel.Protos.ClientVersion result = new org.bitcoin.paymentchannel.Protos.ClientVersion(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.major_ = major_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.minor_ = minor_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.previousChannelContractHash_ = previousChannelContractHash_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.timeWindowSecs_ = timeWindowSecs_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.ClientVersion) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.ClientVersion)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.ClientVersion other) {
if (other == org.bitcoin.paymentchannel.Protos.ClientVersion.getDefaultInstance()) return this;
if (other.hasMajor()) {
setMajor(other.getMajor());
}
if (other.hasMinor()) {
setMinor(other.getMinor());
}
if (other.hasPreviousChannelContractHash()) {
setPreviousChannelContractHash(other.getPreviousChannelContractHash());
}
if (other.hasTimeWindowSecs()) {
setTimeWindowSecs(other.getTimeWindowSecs());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMajor()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.ClientVersion parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.ClientVersion) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int major_ ;
/**
* required int32 major = 1;
*/
public boolean hasMajor() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 major = 1;
*/
public int getMajor() {
return major_;
}
/**
* required int32 major = 1;
*/
public Builder setMajor(int value) {
bitField0_ |= 0x00000001;
major_ = value;
onChanged();
return this;
}
/**
* required int32 major = 1;
*/
public Builder clearMajor() {
bitField0_ = (bitField0_ & ~0x00000001);
major_ = 0;
onChanged();
return this;
}
private int minor_ ;
/**
* optional int32 minor = 2 [default = 0];
*/
public boolean hasMinor() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 minor = 2 [default = 0];
*/
public int getMinor() {
return minor_;
}
/**
* optional int32 minor = 2 [default = 0];
*/
public Builder setMinor(int value) {
bitField0_ |= 0x00000002;
minor_ = value;
onChanged();
return this;
}
/**
* optional int32 minor = 2 [default = 0];
*/
public Builder clearMinor() {
bitField0_ = (bitField0_ & ~0x00000002);
minor_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString previousChannelContractHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
public boolean hasPreviousChannelContractHash() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
public com.google.protobuf.ByteString getPreviousChannelContractHash() {
return previousChannelContractHash_;
}
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
public Builder setPreviousChannelContractHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
previousChannelContractHash_ = value;
onChanged();
return this;
}
/**
* optional bytes previous_channel_contract_hash = 3;
*
*
* The hash of the multisig contract of a previous channel. This indicates that the primary
* wishes to reopen the given channel. If the server is willing to reopen it, it simply
* responds with a SERVER_VERSION and then immediately sends a CHANNEL_OPEN, it otherwise
* follows SERVER_VERSION with an Initiate representing a new channel
*
*/
public Builder clearPreviousChannelContractHash() {
bitField0_ = (bitField0_ & ~0x00000004);
previousChannelContractHash_ = getDefaultInstance().getPreviousChannelContractHash();
onChanged();
return this;
}
private long timeWindowSecs_ = 86340L;
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
public boolean hasTimeWindowSecs() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
public long getTimeWindowSecs() {
return timeWindowSecs_;
}
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
public Builder setTimeWindowSecs(long value) {
bitField0_ |= 0x00000008;
timeWindowSecs_ = value;
onChanged();
return this;
}
/**
* optional uint64 time_window_secs = 4 [default = 86340];
*
*
* How many seconds should the channel be open, only used when a new channel is created.
* Defaults to 24 h minus 60 seconds, 24*60*60 - 60
*
*/
public Builder clearTimeWindowSecs() {
bitField0_ = (bitField0_ & ~0x00000008);
timeWindowSecs_ = 86340L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.ClientVersion)
}
static {
defaultInstance = new ClientVersion(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.ClientVersion)
}
public interface ServerVersionOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.ServerVersion)
com.google.protobuf.MessageOrBuilder {
/**
* required int32 major = 1;
*/
boolean hasMajor();
/**
* required int32 major = 1;
*/
int getMajor();
/**
* optional int32 minor = 2 [default = 0];
*/
boolean hasMinor();
/**
* optional int32 minor = 2 [default = 0];
*/
int getMinor();
}
/**
* Protobuf type {@code paymentchannels.ServerVersion}
*
*
* Send by secondary to primary upon receiving the ClientVersion message. If it is willing to
* speak the given major version, it sends back the same major version and the minor version it
* speaks. If it is not, it may send back a lower major version representing the highest version
* it is willing to speak, or sends a NO_ACCEPTABLE_VERSION Error. If the secondary sends back a
* lower major version, the secondary should either expect to continue with that version, or
* should immediately close the connection with a NO_ACCEPTABLE_VERSION Error. Backwards
* incompatible changes to the protocol bump the major version. Extensions bump the minor version
*
*/
public static final class ServerVersion extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.ServerVersion)
ServerVersionOrBuilder {
// Use ServerVersion.newBuilder() to construct.
private ServerVersion(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ServerVersion(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ServerVersion defaultInstance;
public static ServerVersion getDefaultInstance() {
return defaultInstance;
}
public ServerVersion getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ServerVersion(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
major_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
minor_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ServerVersion_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ServerVersion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ServerVersion.class, org.bitcoin.paymentchannel.Protos.ServerVersion.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ServerVersion parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ServerVersion(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int MAJOR_FIELD_NUMBER = 1;
private int major_;
/**
* required int32 major = 1;
*/
public boolean hasMajor() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 major = 1;
*/
public int getMajor() {
return major_;
}
public static final int MINOR_FIELD_NUMBER = 2;
private int minor_;
/**
* optional int32 minor = 2 [default = 0];
*/
public boolean hasMinor() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 minor = 2 [default = 0];
*/
public int getMinor() {
return minor_;
}
private void initFields() {
major_ = 0;
minor_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasMajor()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, major_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, minor_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, major_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, minor_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ServerVersion parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.ServerVersion prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.ServerVersion}
*
*
* Send by secondary to primary upon receiving the ClientVersion message. If it is willing to
* speak the given major version, it sends back the same major version and the minor version it
* speaks. If it is not, it may send back a lower major version representing the highest version
* it is willing to speak, or sends a NO_ACCEPTABLE_VERSION Error. If the secondary sends back a
* lower major version, the secondary should either expect to continue with that version, or
* should immediately close the connection with a NO_ACCEPTABLE_VERSION Error. Backwards
* incompatible changes to the protocol bump the major version. Extensions bump the minor version
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.ServerVersion)
org.bitcoin.paymentchannel.Protos.ServerVersionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ServerVersion_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ServerVersion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ServerVersion.class, org.bitcoin.paymentchannel.Protos.ServerVersion.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.ServerVersion.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
major_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
minor_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ServerVersion_descriptor;
}
public org.bitcoin.paymentchannel.Protos.ServerVersion getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.ServerVersion build() {
org.bitcoin.paymentchannel.Protos.ServerVersion result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.ServerVersion buildPartial() {
org.bitcoin.paymentchannel.Protos.ServerVersion result = new org.bitcoin.paymentchannel.Protos.ServerVersion(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.major_ = major_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.minor_ = minor_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.ServerVersion) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.ServerVersion)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.ServerVersion other) {
if (other == org.bitcoin.paymentchannel.Protos.ServerVersion.getDefaultInstance()) return this;
if (other.hasMajor()) {
setMajor(other.getMajor());
}
if (other.hasMinor()) {
setMinor(other.getMinor());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMajor()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.ServerVersion parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.ServerVersion) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int major_ ;
/**
* required int32 major = 1;
*/
public boolean hasMajor() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 major = 1;
*/
public int getMajor() {
return major_;
}
/**
* required int32 major = 1;
*/
public Builder setMajor(int value) {
bitField0_ |= 0x00000001;
major_ = value;
onChanged();
return this;
}
/**
* required int32 major = 1;
*/
public Builder clearMajor() {
bitField0_ = (bitField0_ & ~0x00000001);
major_ = 0;
onChanged();
return this;
}
private int minor_ ;
/**
* optional int32 minor = 2 [default = 0];
*/
public boolean hasMinor() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 minor = 2 [default = 0];
*/
public int getMinor() {
return minor_;
}
/**
* optional int32 minor = 2 [default = 0];
*/
public Builder setMinor(int value) {
bitField0_ |= 0x00000002;
minor_ = value;
onChanged();
return this;
}
/**
* optional int32 minor = 2 [default = 0];
*/
public Builder clearMinor() {
bitField0_ = (bitField0_ & ~0x00000002);
minor_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.ServerVersion)
}
static {
defaultInstance = new ServerVersion(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.ServerVersion)
}
public interface InitiateOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.Initiate)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
boolean hasMultisigKey();
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
com.google.protobuf.ByteString getMultisigKey();
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
boolean hasMinAcceptedChannelSize();
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
long getMinAcceptedChannelSize();
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
boolean hasExpireTimeSecs();
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
long getExpireTimeSecs();
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
boolean hasMinPayment();
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
long getMinPayment();
}
/**
* Protobuf type {@code paymentchannels.Initiate}
*
*
* Sent from server to client once version nego is done.
*
*/
public static final class Initiate extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.Initiate)
InitiateOrBuilder {
// Use Initiate.newBuilder() to construct.
private Initiate(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Initiate(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Initiate defaultInstance;
public static Initiate getDefaultInstance() {
return defaultInstance;
}
public Initiate getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Initiate(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
multisigKey_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
minAcceptedChannelSize_ = input.readUInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
expireTimeSecs_ = input.readUInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
minPayment_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Initiate_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Initiate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.Initiate.class, org.bitcoin.paymentchannel.Protos.Initiate.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Initiate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Initiate(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int MULTISIG_KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString multisigKey_;
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
public boolean hasMultisigKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
public com.google.protobuf.ByteString getMultisigKey() {
return multisigKey_;
}
public static final int MIN_ACCEPTED_CHANNEL_SIZE_FIELD_NUMBER = 2;
private long minAcceptedChannelSize_;
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
public boolean hasMinAcceptedChannelSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
public long getMinAcceptedChannelSize() {
return minAcceptedChannelSize_;
}
public static final int EXPIRE_TIME_SECS_FIELD_NUMBER = 3;
private long expireTimeSecs_;
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
public boolean hasExpireTimeSecs() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
public long getExpireTimeSecs() {
return expireTimeSecs_;
}
public static final int MIN_PAYMENT_FIELD_NUMBER = 4;
private long minPayment_;
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
public boolean hasMinPayment() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
public long getMinPayment() {
return minPayment_;
}
private void initFields() {
multisigKey_ = com.google.protobuf.ByteString.EMPTY;
minAcceptedChannelSize_ = 0L;
expireTimeSecs_ = 0L;
minPayment_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasMultisigKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMinAcceptedChannelSize()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasExpireTimeSecs()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMinPayment()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, multisigKey_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(2, minAcceptedChannelSize_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, expireTimeSecs_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt64(4, minPayment_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, multisigKey_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, minAcceptedChannelSize_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, expireTimeSecs_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, minPayment_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Initiate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.Initiate prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.Initiate}
*
*
* Sent from server to client once version nego is done.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.Initiate)
org.bitcoin.paymentchannel.Protos.InitiateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Initiate_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Initiate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.Initiate.class, org.bitcoin.paymentchannel.Protos.Initiate.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.Initiate.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
multisigKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
minAcceptedChannelSize_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
expireTimeSecs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
minPayment_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Initiate_descriptor;
}
public org.bitcoin.paymentchannel.Protos.Initiate getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.Initiate build() {
org.bitcoin.paymentchannel.Protos.Initiate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.Initiate buildPartial() {
org.bitcoin.paymentchannel.Protos.Initiate result = new org.bitcoin.paymentchannel.Protos.Initiate(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.multisigKey_ = multisigKey_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.minAcceptedChannelSize_ = minAcceptedChannelSize_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.expireTimeSecs_ = expireTimeSecs_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.minPayment_ = minPayment_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.Initiate) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.Initiate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.Initiate other) {
if (other == org.bitcoin.paymentchannel.Protos.Initiate.getDefaultInstance()) return this;
if (other.hasMultisigKey()) {
setMultisigKey(other.getMultisigKey());
}
if (other.hasMinAcceptedChannelSize()) {
setMinAcceptedChannelSize(other.getMinAcceptedChannelSize());
}
if (other.hasExpireTimeSecs()) {
setExpireTimeSecs(other.getExpireTimeSecs());
}
if (other.hasMinPayment()) {
setMinPayment(other.getMinPayment());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMultisigKey()) {
return false;
}
if (!hasMinAcceptedChannelSize()) {
return false;
}
if (!hasExpireTimeSecs()) {
return false;
}
if (!hasMinPayment()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.Initiate parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.Initiate) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString multisigKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
public boolean hasMultisigKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
public com.google.protobuf.ByteString getMultisigKey() {
return multisigKey_;
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
public Builder setMultisigKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
multisigKey_ = value;
onChanged();
return this;
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is used only in the creation of the multisig contract, as outputs are
* created entirely by the secondary
*
*/
public Builder clearMultisigKey() {
bitField0_ = (bitField0_ & ~0x00000001);
multisigKey_ = getDefaultInstance().getMultisigKey();
onChanged();
return this;
}
private long minAcceptedChannelSize_ ;
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
public boolean hasMinAcceptedChannelSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
public long getMinAcceptedChannelSize() {
return minAcceptedChannelSize_;
}
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
public Builder setMinAcceptedChannelSize(long value) {
bitField0_ |= 0x00000002;
minAcceptedChannelSize_ = value;
onChanged();
return this;
}
/**
* required uint64 min_accepted_channel_size = 2;
*
*
* Once a channel is exhausted a new one must be set up. So secondary indicates the minimum
* size it's willing to accept here. This can be lower to trade off resources against
* security but shouldn't be so low the transactions get rejected by the network as spam.
* Zero isn't a sensible value to have here, so we make the field required.
*
*/
public Builder clearMinAcceptedChannelSize() {
bitField0_ = (bitField0_ & ~0x00000002);
minAcceptedChannelSize_ = 0L;
onChanged();
return this;
}
private long expireTimeSecs_ ;
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
public boolean hasExpireTimeSecs() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
public long getExpireTimeSecs() {
return expireTimeSecs_;
}
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
public Builder setExpireTimeSecs(long value) {
bitField0_ |= 0x00000004;
expireTimeSecs_ = value;
onChanged();
return this;
}
/**
* required uint64 expire_time_secs = 3;
*
*
* Rough UNIX time for when the channel expires. This is determined by the block header
* timestamps which can be very inaccurate when miners use the obsolete RollNTime hack.
* Channels could also be specified in terms of block heights but then how do you know the
* current chain height if you don't have internet access? Trust the server? Probably opens up
* attack vectors. We can assume the client has an independent clock, however. If the client
* considers this value too far off (eg more than a day), it may send an ERROR and close the
* channel.
*
*/
public Builder clearExpireTimeSecs() {
bitField0_ = (bitField0_ & ~0x00000004);
expireTimeSecs_ = 0L;
onChanged();
return this;
}
private long minPayment_ ;
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
public boolean hasMinPayment() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
public long getMinPayment() {
return minPayment_;
}
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
public Builder setMinPayment(long value) {
bitField0_ |= 0x00000008;
minPayment_ = value;
onChanged();
return this;
}
/**
* required uint64 min_payment = 4;
*
*
* The amount of money the server requires for the initial payment. The act of opening a channel
* always transfers some quantity of money to the server: it's impossible to have a channel with
* zero value transferred. This rule ensures that you can't get a channel that can't be settled
* due to having paid under the dust limit. Because the dust limit will float in future, the
* server tells the client what it thinks it is, and the client is supposed to sanity check this
* value.
*
*/
public Builder clearMinPayment() {
bitField0_ = (bitField0_ & ~0x00000008);
minPayment_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.Initiate)
}
static {
defaultInstance = new Initiate(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.Initiate)
}
public interface ProvideRefundOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.ProvideRefund)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
boolean hasMultisigKey();
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
com.google.protobuf.ByteString getMultisigKey();
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
boolean hasTx();
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
com.google.protobuf.ByteString getTx();
}
/**
* Protobuf type {@code paymentchannels.ProvideRefund}
*
*
* Sent from primary to secondary after Initiate to begin the refund transaction signing.
*
*/
public static final class ProvideRefund extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.ProvideRefund)
ProvideRefundOrBuilder {
// Use ProvideRefund.newBuilder() to construct.
private ProvideRefund(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ProvideRefund(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ProvideRefund defaultInstance;
public static ProvideRefund getDefaultInstance() {
return defaultInstance;
}
public ProvideRefund getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProvideRefund(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
multisigKey_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
tx_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideRefund_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideRefund_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ProvideRefund.class, org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ProvideRefund parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProvideRefund(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int MULTISIG_KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString multisigKey_;
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public boolean hasMultisigKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public com.google.protobuf.ByteString getMultisigKey() {
return multisigKey_;
}
public static final int TX_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString tx_;
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
public boolean hasTx() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
public com.google.protobuf.ByteString getTx() {
return tx_;
}
private void initFields() {
multisigKey_ = com.google.protobuf.ByteString.EMPTY;
tx_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasMultisigKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTx()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, multisigKey_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, tx_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, multisigKey_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, tx_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ProvideRefund parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.ProvideRefund prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.ProvideRefund}
*
*
* Sent from primary to secondary after Initiate to begin the refund transaction signing.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.ProvideRefund)
org.bitcoin.paymentchannel.Protos.ProvideRefundOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideRefund_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideRefund_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ProvideRefund.class, org.bitcoin.paymentchannel.Protos.ProvideRefund.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.ProvideRefund.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
multisigKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
tx_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideRefund_descriptor;
}
public org.bitcoin.paymentchannel.Protos.ProvideRefund getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.ProvideRefund build() {
org.bitcoin.paymentchannel.Protos.ProvideRefund result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.ProvideRefund buildPartial() {
org.bitcoin.paymentchannel.Protos.ProvideRefund result = new org.bitcoin.paymentchannel.Protos.ProvideRefund(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.multisigKey_ = multisigKey_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.tx_ = tx_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.ProvideRefund) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.ProvideRefund)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.ProvideRefund other) {
if (other == org.bitcoin.paymentchannel.Protos.ProvideRefund.getDefaultInstance()) return this;
if (other.hasMultisigKey()) {
setMultisigKey(other.getMultisigKey());
}
if (other.hasTx()) {
setTx(other.getTx());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMultisigKey()) {
return false;
}
if (!hasTx()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.ProvideRefund parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.ProvideRefund) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString multisigKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public boolean hasMultisigKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public com.google.protobuf.ByteString getMultisigKey() {
return multisigKey_;
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public Builder setMultisigKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
multisigKey_ = value;
onChanged();
return this;
}
/**
* required bytes multisig_key = 1;
*
*
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public Builder clearMultisigKey() {
bitField0_ = (bitField0_ & ~0x00000001);
multisigKey_ = getDefaultInstance().getMultisigKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString tx_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
public boolean hasTx() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
public com.google.protobuf.ByteString getTx() {
return tx_;
}
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
public Builder setTx(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
tx_ = value;
onChanged();
return this;
}
/**
* required bytes tx = 2;
*
*
* The serialized bytes of the return transaction in Satoshi format.
* * It must have exactly one input which spends the multisig output (see ProvideContract for
* details of exactly what that output must look like). This output must have a sequence
* number of 0.
* * It must have the lock time set to a time after the min_time_window_secs (from the
* Initiate message).
* * It must have exactly one output which goes back to the primary. This output's
* scriptPubKey will be reused to create payment transactions.
*
*/
public Builder clearTx() {
bitField0_ = (bitField0_ & ~0x00000002);
tx_ = getDefaultInstance().getTx();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.ProvideRefund)
}
static {
defaultInstance = new ProvideRefund(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.ProvideRefund)
}
public interface ReturnRefundOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.ReturnRefund)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes signature = 1;
*/
boolean hasSignature();
/**
* required bytes signature = 1;
*/
com.google.protobuf.ByteString getSignature();
}
/**
* Protobuf type {@code paymentchannels.ReturnRefund}
*
*
* Sent from secondary to primary after it has done initial verification of the refund
* transaction. Contains the primary's signature which is required to spend the multisig contract
* to the refund transaction. Must be signed using SIGHASH_NONE|SIGHASH_ANYONECANPAY (and include
* the postfix type byte) to allow the client to add any outputs/inputs it wants as long as the
* input's sequence and transaction's nLockTime remain set.
*
*/
public static final class ReturnRefund extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.ReturnRefund)
ReturnRefundOrBuilder {
// Use ReturnRefund.newBuilder() to construct.
private ReturnRefund(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ReturnRefund(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ReturnRefund defaultInstance;
public static ReturnRefund getDefaultInstance() {
return defaultInstance;
}
public ReturnRefund getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReturnRefund(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
signature_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ReturnRefund_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ReturnRefund_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ReturnRefund.class, org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ReturnRefund parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReturnRefund(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int SIGNATURE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString signature_;
/**
* required bytes signature = 1;
*/
public boolean hasSignature() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes signature = 1;
*/
public com.google.protobuf.ByteString getSignature() {
return signature_;
}
private void initFields() {
signature_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasSignature()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, signature_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, signature_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ReturnRefund parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.ReturnRefund prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.ReturnRefund}
*
*
* Sent from secondary to primary after it has done initial verification of the refund
* transaction. Contains the primary's signature which is required to spend the multisig contract
* to the refund transaction. Must be signed using SIGHASH_NONE|SIGHASH_ANYONECANPAY (and include
* the postfix type byte) to allow the client to add any outputs/inputs it wants as long as the
* input's sequence and transaction's nLockTime remain set.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.ReturnRefund)
org.bitcoin.paymentchannel.Protos.ReturnRefundOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ReturnRefund_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ReturnRefund_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ReturnRefund.class, org.bitcoin.paymentchannel.Protos.ReturnRefund.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.ReturnRefund.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
signature_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ReturnRefund_descriptor;
}
public org.bitcoin.paymentchannel.Protos.ReturnRefund getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.ReturnRefund build() {
org.bitcoin.paymentchannel.Protos.ReturnRefund result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.ReturnRefund buildPartial() {
org.bitcoin.paymentchannel.Protos.ReturnRefund result = new org.bitcoin.paymentchannel.Protos.ReturnRefund(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.signature_ = signature_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.ReturnRefund) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.ReturnRefund)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.ReturnRefund other) {
if (other == org.bitcoin.paymentchannel.Protos.ReturnRefund.getDefaultInstance()) return this;
if (other.hasSignature()) {
setSignature(other.getSignature());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasSignature()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.ReturnRefund parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.ReturnRefund) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString signature_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes signature = 1;
*/
public boolean hasSignature() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes signature = 1;
*/
public com.google.protobuf.ByteString getSignature() {
return signature_;
}
/**
* required bytes signature = 1;
*/
public Builder setSignature(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
signature_ = value;
onChanged();
return this;
}
/**
* required bytes signature = 1;
*/
public Builder clearSignature() {
bitField0_ = (bitField0_ & ~0x00000001);
signature_ = getDefaultInstance().getSignature();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.ReturnRefund)
}
static {
defaultInstance = new ReturnRefund(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.ReturnRefund)
}
public interface ProvideContractOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.ProvideContract)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
boolean hasTx();
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
com.google.protobuf.ByteString getTx();
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
boolean hasInitialPayment();
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
org.bitcoin.paymentchannel.Protos.UpdatePayment getInitialPayment();
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder getInitialPaymentOrBuilder();
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
boolean hasClientKey();
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
com.google.protobuf.ByteString getClientKey();
}
/**
* Protobuf type {@code paymentchannels.ProvideContract}
*
*
* Sent from the primary to the secondary to complete initialization.
*
*/
public static final class ProvideContract extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.ProvideContract)
ProvideContractOrBuilder {
// Use ProvideContract.newBuilder() to construct.
private ProvideContract(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ProvideContract(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ProvideContract defaultInstance;
public static ProvideContract getDefaultInstance() {
return defaultInstance;
}
public ProvideContract getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProvideContract(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
tx_ = input.readBytes();
break;
}
case 18: {
org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = initialPayment_.toBuilder();
}
initialPayment_ = input.readMessage(org.bitcoin.paymentchannel.Protos.UpdatePayment.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(initialPayment_);
initialPayment_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
bitField0_ |= 0x00000004;
clientKey_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideContract_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideContract_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ProvideContract.class, org.bitcoin.paymentchannel.Protos.ProvideContract.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ProvideContract parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProvideContract(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TX_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString tx_;
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
public boolean hasTx() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
public com.google.protobuf.ByteString getTx() {
return tx_;
}
public static final int INITIAL_PAYMENT_FIELD_NUMBER = 2;
private org.bitcoin.paymentchannel.Protos.UpdatePayment initialPayment_;
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public boolean hasInitialPayment() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public org.bitcoin.paymentchannel.Protos.UpdatePayment getInitialPayment() {
return initialPayment_;
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder getInitialPaymentOrBuilder() {
return initialPayment_;
}
public static final int CLIENT_KEY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString clientKey_;
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public boolean hasClientKey() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public com.google.protobuf.ByteString getClientKey() {
return clientKey_;
}
private void initFields() {
tx_ = com.google.protobuf.ByteString.EMPTY;
initialPayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
clientKey_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTx()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasInitialPayment()) {
memoizedIsInitialized = 0;
return false;
}
if (!getInitialPayment().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, tx_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, initialPayment_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, clientKey_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, tx_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, initialPayment_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, clientKey_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.ProvideContract parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.ProvideContract prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.ProvideContract}
*
*
* Sent from the primary to the secondary to complete initialization.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.ProvideContract)
org.bitcoin.paymentchannel.Protos.ProvideContractOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideContract_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideContract_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.ProvideContract.class, org.bitcoin.paymentchannel.Protos.ProvideContract.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.ProvideContract.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getInitialPaymentFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
tx_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (initialPaymentBuilder_ == null) {
initialPayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
} else {
initialPaymentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
clientKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_ProvideContract_descriptor;
}
public org.bitcoin.paymentchannel.Protos.ProvideContract getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.ProvideContract build() {
org.bitcoin.paymentchannel.Protos.ProvideContract result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.ProvideContract buildPartial() {
org.bitcoin.paymentchannel.Protos.ProvideContract result = new org.bitcoin.paymentchannel.Protos.ProvideContract(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.tx_ = tx_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (initialPaymentBuilder_ == null) {
result.initialPayment_ = initialPayment_;
} else {
result.initialPayment_ = initialPaymentBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.clientKey_ = clientKey_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.ProvideContract) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.ProvideContract)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.ProvideContract other) {
if (other == org.bitcoin.paymentchannel.Protos.ProvideContract.getDefaultInstance()) return this;
if (other.hasTx()) {
setTx(other.getTx());
}
if (other.hasInitialPayment()) {
mergeInitialPayment(other.getInitialPayment());
}
if (other.hasClientKey()) {
setClientKey(other.getClientKey());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTx()) {
return false;
}
if (!hasInitialPayment()) {
return false;
}
if (!getInitialPayment().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.ProvideContract parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.ProvideContract) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString tx_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
public boolean hasTx() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
public com.google.protobuf.ByteString getTx() {
return tx_;
}
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
public Builder setTx(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
tx_ = value;
onChanged();
return this;
}
/**
* required bytes tx = 1;
*
*
* The serialized bytes of the transaction in Satoshi format.
* For version 1:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a 2-of-2 multisig output with the first pubkey being the
* primary's and the second being the secondary's (ie the script must be exactly "OP_2
* ProvideRefund.multisig_key Initiate.multisig_key OP_2 OP_CHECKMULTISIG")
* For version 2:
* * It must be signed and completely valid and ready for broadcast (ie it includes the
* necessary fees) TODO: tell the client how much fee it needs
* * Its first output must be a CHECKLOCKTIMEVERIFY output with the first pubkey being the
* primary's and the second being the secondary's.
*
*/
public Builder clearTx() {
bitField0_ = (bitField0_ & ~0x00000001);
tx_ = getDefaultInstance().getTx();
onChanged();
return this;
}
private org.bitcoin.paymentchannel.Protos.UpdatePayment initialPayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.UpdatePayment, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder, org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder> initialPaymentBuilder_;
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public boolean hasInitialPayment() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public org.bitcoin.paymentchannel.Protos.UpdatePayment getInitialPayment() {
if (initialPaymentBuilder_ == null) {
return initialPayment_;
} else {
return initialPaymentBuilder_.getMessage();
}
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public Builder setInitialPayment(org.bitcoin.paymentchannel.Protos.UpdatePayment value) {
if (initialPaymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
initialPayment_ = value;
onChanged();
} else {
initialPaymentBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public Builder setInitialPayment(
org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder builderForValue) {
if (initialPaymentBuilder_ == null) {
initialPayment_ = builderForValue.build();
onChanged();
} else {
initialPaymentBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public Builder mergeInitialPayment(org.bitcoin.paymentchannel.Protos.UpdatePayment value) {
if (initialPaymentBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
initialPayment_ != org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance()) {
initialPayment_ =
org.bitcoin.paymentchannel.Protos.UpdatePayment.newBuilder(initialPayment_).mergeFrom(value).buildPartial();
} else {
initialPayment_ = value;
}
onChanged();
} else {
initialPaymentBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public Builder clearInitialPayment() {
if (initialPaymentBuilder_ == null) {
initialPayment_ = org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
onChanged();
} else {
initialPaymentBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder getInitialPaymentBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getInitialPaymentFieldBuilder().getBuilder();
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
public org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder getInitialPaymentOrBuilder() {
if (initialPaymentBuilder_ != null) {
return initialPaymentBuilder_.getMessageOrBuilder();
} else {
return initialPayment_;
}
}
/**
* required .paymentchannels.UpdatePayment initial_payment = 2;
*
*
* To open the channel, an initial payment of the server-specified dust limit value must be
* provided. This ensures that the channel is never in an un-settleable state due to either
* no payment tx having been provided at all, or a payment that is smaller than the dust
* limit being provided.
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.UpdatePayment, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder, org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder>
getInitialPaymentFieldBuilder() {
if (initialPaymentBuilder_ == null) {
initialPaymentBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.bitcoin.paymentchannel.Protos.UpdatePayment, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder, org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder>(
getInitialPayment(),
getParentForChildren(),
isClean());
initialPayment_ = null;
}
return initialPaymentBuilder_;
}
private com.google.protobuf.ByteString clientKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public boolean hasClientKey() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public com.google.protobuf.ByteString getClientKey() {
return clientKey_;
}
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public Builder setClientKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
clientKey_ = value;
onChanged();
return this;
}
/**
* optional bytes client_key = 3;
*
*
* This field is added in protocol version 2 to send the client public key to the server.
* In version 1 it isn't used.
* This must be a raw pubkey in regular ECDSA form. Both compressed and non-compressed forms
* are accepted. It is only used in the creation of the multisig contract.
*
*/
public Builder clearClientKey() {
bitField0_ = (bitField0_ & ~0x00000004);
clientKey_ = getDefaultInstance().getClientKey();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.ProvideContract)
}
static {
defaultInstance = new ProvideContract(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.ProvideContract)
}
public interface UpdatePaymentOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.UpdatePayment)
com.google.protobuf.MessageOrBuilder {
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
boolean hasClientChangeValue();
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
long getClientChangeValue();
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
boolean hasSignature();
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
com.google.protobuf.ByteString getSignature();
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
boolean hasInfo();
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
com.google.protobuf.ByteString getInfo();
}
/**
* Protobuf type {@code paymentchannels.UpdatePayment}
*
*
* This message can only be used by the primary after it has received a CHANNEL_OPEN message. It
* creates a new payment transaction. Note that we don't resubmit the entire TX, this is to avoid
* (re)parsing bugs and overhead. The payment transaction is created by the primary by:
* * Adding an input which spends the multisig contract
* * Setting this input's scriptSig to the given signature and a new signature created by the
* primary (the primary should ensure the signature provided correctly spends the multisig
* contract)
* * Adding an output who's scriptPubKey is the same as the refund output (the only output) in
* the refund transaction
* * Setting this output's value to client_change_value (which must be lower than the most recent
* client_change_value and lower than the multisig contract's output value)
* * Adding any number of additional outputs as desired (leaving sufficient fee, if necessary)
* * Adding any number of additional inputs as desired (eg to add more fee)
*
*/
public static final class UpdatePayment extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.UpdatePayment)
UpdatePaymentOrBuilder {
// Use UpdatePayment.newBuilder() to construct.
private UpdatePayment(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private UpdatePayment(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final UpdatePayment defaultInstance;
public static UpdatePayment getDefaultInstance() {
return defaultInstance;
}
public UpdatePayment getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UpdatePayment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
clientChangeValue_ = input.readUInt64();
break;
}
case 18: {
bitField0_ |= 0x00000002;
signature_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
info_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_UpdatePayment_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_UpdatePayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.UpdatePayment.class, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public UpdatePayment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UpdatePayment(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int CLIENT_CHANGE_VALUE_FIELD_NUMBER = 1;
private long clientChangeValue_;
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
public boolean hasClientChangeValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
public long getClientChangeValue() {
return clientChangeValue_;
}
public static final int SIGNATURE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString signature_;
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
public boolean hasSignature() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
public com.google.protobuf.ByteString getSignature() {
return signature_;
}
public static final int INFO_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString info_;
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
public com.google.protobuf.ByteString getInfo() {
return info_;
}
private void initFields() {
clientChangeValue_ = 0L;
signature_ = com.google.protobuf.ByteString.EMPTY;
info_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasClientChangeValue()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSignature()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(1, clientChangeValue_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, signature_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, info_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, clientChangeValue_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, signature_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, info_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.UpdatePayment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.UpdatePayment prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.UpdatePayment}
*
*
* This message can only be used by the primary after it has received a CHANNEL_OPEN message. It
* creates a new payment transaction. Note that we don't resubmit the entire TX, this is to avoid
* (re)parsing bugs and overhead. The payment transaction is created by the primary by:
* * Adding an input which spends the multisig contract
* * Setting this input's scriptSig to the given signature and a new signature created by the
* primary (the primary should ensure the signature provided correctly spends the multisig
* contract)
* * Adding an output who's scriptPubKey is the same as the refund output (the only output) in
* the refund transaction
* * Setting this output's value to client_change_value (which must be lower than the most recent
* client_change_value and lower than the multisig contract's output value)
* * Adding any number of additional outputs as desired (leaving sufficient fee, if necessary)
* * Adding any number of additional inputs as desired (eg to add more fee)
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.UpdatePayment)
org.bitcoin.paymentchannel.Protos.UpdatePaymentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_UpdatePayment_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_UpdatePayment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.UpdatePayment.class, org.bitcoin.paymentchannel.Protos.UpdatePayment.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.UpdatePayment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
clientChangeValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
signature_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
info_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_UpdatePayment_descriptor;
}
public org.bitcoin.paymentchannel.Protos.UpdatePayment getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.UpdatePayment build() {
org.bitcoin.paymentchannel.Protos.UpdatePayment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.UpdatePayment buildPartial() {
org.bitcoin.paymentchannel.Protos.UpdatePayment result = new org.bitcoin.paymentchannel.Protos.UpdatePayment(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.clientChangeValue_ = clientChangeValue_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.signature_ = signature_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.info_ = info_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.UpdatePayment) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.UpdatePayment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.UpdatePayment other) {
if (other == org.bitcoin.paymentchannel.Protos.UpdatePayment.getDefaultInstance()) return this;
if (other.hasClientChangeValue()) {
setClientChangeValue(other.getClientChangeValue());
}
if (other.hasSignature()) {
setSignature(other.getSignature());
}
if (other.hasInfo()) {
setInfo(other.getInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasClientChangeValue()) {
return false;
}
if (!hasSignature()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.UpdatePayment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.UpdatePayment) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long clientChangeValue_ ;
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
public boolean hasClientChangeValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
public long getClientChangeValue() {
return clientChangeValue_;
}
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
public Builder setClientChangeValue(long value) {
bitField0_ |= 0x00000001;
clientChangeValue_ = value;
onChanged();
return this;
}
/**
* required uint64 client_change_value = 1;
*
*
* The value which is sent back to the primary. The rest of the multisig output is left for
* the secondary to do with as they wish.
*
*/
public Builder clearClientChangeValue() {
bitField0_ = (bitField0_ & ~0x00000001);
clientChangeValue_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString signature_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
public boolean hasSignature() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
public com.google.protobuf.ByteString getSignature() {
return signature_;
}
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
public Builder setSignature(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
signature_ = value;
onChanged();
return this;
}
/**
* required bytes signature = 2;
*
*
* A SIGHASH_SINGLE|SIGHASH_ANYONECANPAY signature (including the postfix type byte) which
* spends the primary's part of the multisig contract's output. This signature only covers
* the primary's refund output and thus the secondary is free to do what they wish with their
* part of the multisig output.
*
*/
public Builder clearSignature() {
bitField0_ = (bitField0_ & ~0x00000002);
signature_ = getDefaultInstance().getSignature();
onChanged();
return this;
}
private com.google.protobuf.ByteString info_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
public com.google.protobuf.ByteString getInfo() {
return info_;
}
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
public Builder setInfo(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
info_ = value;
onChanged();
return this;
}
/**
* optional bytes info = 3;
*
*
* Information about this update. Used to extend this protocol.
*
*/
public Builder clearInfo() {
bitField0_ = (bitField0_ & ~0x00000004);
info_ = getDefaultInstance().getInfo();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.UpdatePayment)
}
static {
defaultInstance = new UpdatePayment(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.UpdatePayment)
}
public interface PaymentAckOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.PaymentAck)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
boolean hasInfo();
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
com.google.protobuf.ByteString getInfo();
}
/**
* Protobuf type {@code paymentchannels.PaymentAck}
*
*
* This message is sent as an acknowledgement of an UpdatePayment message
*
*/
public static final class PaymentAck extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.PaymentAck)
PaymentAckOrBuilder {
// Use PaymentAck.newBuilder() to construct.
private PaymentAck(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private PaymentAck(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final PaymentAck defaultInstance;
public static PaymentAck getDefaultInstance() {
return defaultInstance;
}
public PaymentAck getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PaymentAck(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
info_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_PaymentAck_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_PaymentAck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.PaymentAck.class, org.bitcoin.paymentchannel.Protos.PaymentAck.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public PaymentAck parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PaymentAck(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int INFO_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString info_;
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
public com.google.protobuf.ByteString getInfo() {
return info_;
}
private void initFields() {
info_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, info_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, info_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.PaymentAck parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.PaymentAck prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.PaymentAck}
*
*
* This message is sent as an acknowledgement of an UpdatePayment message
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.PaymentAck)
org.bitcoin.paymentchannel.Protos.PaymentAckOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_PaymentAck_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_PaymentAck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.PaymentAck.class, org.bitcoin.paymentchannel.Protos.PaymentAck.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.PaymentAck.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
info_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_PaymentAck_descriptor;
}
public org.bitcoin.paymentchannel.Protos.PaymentAck getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.PaymentAck build() {
org.bitcoin.paymentchannel.Protos.PaymentAck result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.PaymentAck buildPartial() {
org.bitcoin.paymentchannel.Protos.PaymentAck result = new org.bitcoin.paymentchannel.Protos.PaymentAck(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.info_ = info_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.PaymentAck) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.PaymentAck)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.PaymentAck other) {
if (other == org.bitcoin.paymentchannel.Protos.PaymentAck.getDefaultInstance()) return this;
if (other.hasInfo()) {
setInfo(other.getInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.PaymentAck parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.PaymentAck) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString info_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
public com.google.protobuf.ByteString getInfo() {
return info_;
}
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
public Builder setInfo(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
info_ = value;
onChanged();
return this;
}
/**
* optional bytes info = 1;
*
*
* Information about this update. Used to extend this protocol
*
*/
public Builder clearInfo() {
bitField0_ = (bitField0_ & ~0x00000001);
info_ = getDefaultInstance().getInfo();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.PaymentAck)
}
static {
defaultInstance = new PaymentAck(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.PaymentAck)
}
public interface SettlementOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.Settlement)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
boolean hasTx();
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
com.google.protobuf.ByteString getTx();
}
/**
* Protobuf type {@code paymentchannels.Settlement}
*/
public static final class Settlement extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.Settlement)
SettlementOrBuilder {
// Use Settlement.newBuilder() to construct.
private Settlement(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Settlement(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Settlement defaultInstance;
public static Settlement getDefaultInstance() {
return defaultInstance;
}
public Settlement getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Settlement(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 26: {
bitField0_ |= 0x00000001;
tx_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Settlement_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Settlement_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.Settlement.class, org.bitcoin.paymentchannel.Protos.Settlement.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Settlement parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Settlement(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TX_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString tx_;
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
public boolean hasTx() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
public com.google.protobuf.ByteString getTx() {
return tx_;
}
private void initFields() {
tx_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTx()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(3, tx_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, tx_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Settlement parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.Settlement prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.Settlement}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.Settlement)
org.bitcoin.paymentchannel.Protos.SettlementOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Settlement_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Settlement_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.Settlement.class, org.bitcoin.paymentchannel.Protos.Settlement.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.Settlement.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
tx_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Settlement_descriptor;
}
public org.bitcoin.paymentchannel.Protos.Settlement getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.Settlement build() {
org.bitcoin.paymentchannel.Protos.Settlement result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.Settlement buildPartial() {
org.bitcoin.paymentchannel.Protos.Settlement result = new org.bitcoin.paymentchannel.Protos.Settlement(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.tx_ = tx_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.Settlement) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.Settlement)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.Settlement other) {
if (other == org.bitcoin.paymentchannel.Protos.Settlement.getDefaultInstance()) return this;
if (other.hasTx()) {
setTx(other.getTx());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTx()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.Settlement parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.Settlement) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString tx_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
public boolean hasTx() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
public com.google.protobuf.ByteString getTx() {
return tx_;
}
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
public Builder setTx(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
tx_ = value;
onChanged();
return this;
}
/**
* required bytes tx = 3;
*
*
* A copy of the fully signed final contract that settles the channel. The client can verify
* the transaction is correct and then commit it to their wallet.
*
*/
public Builder clearTx() {
bitField0_ = (bitField0_ & ~0x00000001);
tx_ = getDefaultInstance().getTx();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.Settlement)
}
static {
defaultInstance = new Settlement(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.Settlement)
}
public interface ErrorOrBuilder extends
// @@protoc_insertion_point(interface_extends:paymentchannels.Error)
com.google.protobuf.MessageOrBuilder {
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
boolean hasCode();
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
org.bitcoin.paymentchannel.Protos.Error.ErrorCode getCode();
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
boolean hasExplanation();
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
java.lang.String getExplanation();
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
com.google.protobuf.ByteString
getExplanationBytes();
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
boolean hasExpectedValue();
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
long getExpectedValue();
}
/**
* Protobuf type {@code paymentchannels.Error}
*
*
* An Error can be sent by either party at any time
* Both parties should make an effort to send either an ERROR or a CLOSE immediately before
* closing the socket (unless they just received an ERROR or a CLOSE)
*
*/
public static final class Error extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:paymentchannels.Error)
ErrorOrBuilder {
// Use Error.newBuilder() to construct.
private Error(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Error(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Error defaultInstance;
public static Error getDefaultInstance() {
return defaultInstance;
}
public Error getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Error(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
org.bitcoin.paymentchannel.Protos.Error.ErrorCode value = org.bitcoin.paymentchannel.Protos.Error.ErrorCode.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
code_ = value;
}
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
explanation_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
expectedValue_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Error_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Error_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.Error.class, org.bitcoin.paymentchannel.Protos.Error.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Error parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Error(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code paymentchannels.Error.ErrorCode}
*/
public enum ErrorCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* TIMEOUT = 1;
*
*
* Protocol timeout occurred (one party hung).
*
*/
TIMEOUT(0, 1),
/**
* SYNTAX_ERROR = 2;
*
*
* Generic error indicating some message was not properly
*
*/
SYNTAX_ERROR(1, 2),
/**
* NO_ACCEPTABLE_VERSION = 3;
*
*
* formatted or was out of order.
*
*/
NO_ACCEPTABLE_VERSION(2, 3),
/**
* BAD_TRANSACTION = 4;
*
*
* A provided transaction was not in the proper structure
*
*/
BAD_TRANSACTION(3, 4),
/**
* TIME_WINDOW_UNACCEPTABLE = 5;
*
*
* (wrong inputs/outputs, sequence, lock time, signature,
* etc)
*
*/
TIME_WINDOW_UNACCEPTABLE(4, 5),
/**
* CHANNEL_VALUE_TOO_LARGE = 6;
*
*
* for the primary
*
*/
CHANNEL_VALUE_TOO_LARGE(5, 6),
/**
* MIN_PAYMENT_TOO_LARGE = 7;
*
*
* too large for the primary
*
*/
MIN_PAYMENT_TOO_LARGE(6, 7),
/**
* OTHER = 8;
*/
OTHER(7, 8),
;
/**
* TIMEOUT = 1;
*
*
* Protocol timeout occurred (one party hung).
*
*/
public static final int TIMEOUT_VALUE = 1;
/**
* SYNTAX_ERROR = 2;
*
*
* Generic error indicating some message was not properly
*
*/
public static final int SYNTAX_ERROR_VALUE = 2;
/**
* NO_ACCEPTABLE_VERSION = 3;
*
*
* formatted or was out of order.
*
*/
public static final int NO_ACCEPTABLE_VERSION_VALUE = 3;
/**
* BAD_TRANSACTION = 4;
*
*
* A provided transaction was not in the proper structure
*
*/
public static final int BAD_TRANSACTION_VALUE = 4;
/**
* TIME_WINDOW_UNACCEPTABLE = 5;
*
*
* (wrong inputs/outputs, sequence, lock time, signature,
* etc)
*
*/
public static final int TIME_WINDOW_UNACCEPTABLE_VALUE = 5;
/**
* CHANNEL_VALUE_TOO_LARGE = 6;
*
*
* for the primary
*
*/
public static final int CHANNEL_VALUE_TOO_LARGE_VALUE = 6;
/**
* MIN_PAYMENT_TOO_LARGE = 7;
*
*
* too large for the primary
*
*/
public static final int MIN_PAYMENT_TOO_LARGE_VALUE = 7;
/**
* OTHER = 8;
*/
public static final int OTHER_VALUE = 8;
public final int getNumber() { return value; }
public static ErrorCode valueOf(int value) {
switch (value) {
case 1: return TIMEOUT;
case 2: return SYNTAX_ERROR;
case 3: return NO_ACCEPTABLE_VERSION;
case 4: return BAD_TRANSACTION;
case 5: return TIME_WINDOW_UNACCEPTABLE;
case 6: return CHANNEL_VALUE_TOO_LARGE;
case 7: return MIN_PAYMENT_TOO_LARGE;
case 8: return OTHER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ErrorCode findValueByNumber(int number) {
return ErrorCode.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.Error.getDescriptor().getEnumTypes().get(0);
}
private static final ErrorCode[] VALUES = values();
public static ErrorCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ErrorCode(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:paymentchannels.Error.ErrorCode)
}
private int bitField0_;
public static final int CODE_FIELD_NUMBER = 1;
private org.bitcoin.paymentchannel.Protos.Error.ErrorCode code_;
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
public org.bitcoin.paymentchannel.Protos.Error.ErrorCode getCode() {
return code_;
}
public static final int EXPLANATION_FIELD_NUMBER = 2;
private java.lang.Object explanation_;
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public boolean hasExplanation() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public java.lang.String getExplanation() {
java.lang.Object ref = explanation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
explanation_ = s;
}
return s;
}
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public com.google.protobuf.ByteString
getExplanationBytes() {
java.lang.Object ref = explanation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
explanation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXPECTED_VALUE_FIELD_NUMBER = 3;
private long expectedValue_;
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
public boolean hasExpectedValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
public long getExpectedValue() {
return expectedValue_;
}
private void initFields() {
code_ = org.bitcoin.paymentchannel.Protos.Error.ErrorCode.OTHER;
explanation_ = "";
expectedValue_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, code_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getExplanationBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, expectedValue_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, code_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getExplanationBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, expectedValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Error parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Error parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.bitcoin.paymentchannel.Protos.Error parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.bitcoin.paymentchannel.Protos.Error prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code paymentchannels.Error}
*
*
* An Error can be sent by either party at any time
* Both parties should make an effort to send either an ERROR or a CLOSE immediately before
* closing the socket (unless they just received an ERROR or a CLOSE)
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:paymentchannels.Error)
org.bitcoin.paymentchannel.Protos.ErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Error_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Error_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoin.paymentchannel.Protos.Error.class, org.bitcoin.paymentchannel.Protos.Error.Builder.class);
}
// Construct using org.bitcoin.paymentchannel.Protos.Error.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
code_ = org.bitcoin.paymentchannel.Protos.Error.ErrorCode.OTHER;
bitField0_ = (bitField0_ & ~0x00000001);
explanation_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
expectedValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoin.paymentchannel.Protos.internal_static_paymentchannels_Error_descriptor;
}
public org.bitcoin.paymentchannel.Protos.Error getDefaultInstanceForType() {
return org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance();
}
public org.bitcoin.paymentchannel.Protos.Error build() {
org.bitcoin.paymentchannel.Protos.Error result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.bitcoin.paymentchannel.Protos.Error buildPartial() {
org.bitcoin.paymentchannel.Protos.Error result = new org.bitcoin.paymentchannel.Protos.Error(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.code_ = code_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.explanation_ = explanation_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.expectedValue_ = expectedValue_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoin.paymentchannel.Protos.Error) {
return mergeFrom((org.bitcoin.paymentchannel.Protos.Error)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoin.paymentchannel.Protos.Error other) {
if (other == org.bitcoin.paymentchannel.Protos.Error.getDefaultInstance()) return this;
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasExplanation()) {
bitField0_ |= 0x00000002;
explanation_ = other.explanation_;
onChanged();
}
if (other.hasExpectedValue()) {
setExpectedValue(other.getExpectedValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoin.paymentchannel.Protos.Error parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoin.paymentchannel.Protos.Error) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.bitcoin.paymentchannel.Protos.Error.ErrorCode code_ = org.bitcoin.paymentchannel.Protos.Error.ErrorCode.OTHER;
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
public org.bitcoin.paymentchannel.Protos.Error.ErrorCode getCode() {
return code_;
}
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
public Builder setCode(org.bitcoin.paymentchannel.Protos.Error.ErrorCode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
code_ = value;
onChanged();
return this;
}
/**
* optional .paymentchannels.Error.ErrorCode code = 1 [default = OTHER];
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000001);
code_ = org.bitcoin.paymentchannel.Protos.Error.ErrorCode.OTHER;
onChanged();
return this;
}
private java.lang.Object explanation_ = "";
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public boolean hasExplanation() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public java.lang.String getExplanation() {
java.lang.Object ref = explanation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
explanation_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public com.google.protobuf.ByteString
getExplanationBytes() {
java.lang.Object ref = explanation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
explanation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public Builder setExplanation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
explanation_ = value;
onChanged();
return this;
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public Builder clearExplanation() {
bitField0_ = (bitField0_ & ~0x00000002);
explanation_ = getDefaultInstance().getExplanation();
onChanged();
return this;
}
/**
* optional string explanation = 2;
*
*
* NOT SAFE FOR HTML WITHOUT ESCAPING
*
*/
public Builder setExplanationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
explanation_ = value;
onChanged();
return this;
}
private long expectedValue_ ;
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
public boolean hasExpectedValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
public long getExpectedValue() {
return expectedValue_;
}
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
public Builder setExpectedValue(long value) {
bitField0_ |= 0x00000004;
expectedValue_ = value;
onChanged();
return this;
}
/**
* optional uint64 expected_value = 3;
*
*
* Can be set by the client when erroring to the server if a value was out of range. Can help with debugging.
*
*/
public Builder clearExpectedValue() {
bitField0_ = (bitField0_ & ~0x00000004);
expectedValue_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:paymentchannels.Error)
}
static {
defaultInstance = new Error(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:paymentchannels.Error)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_TwoWayChannelMessage_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_TwoWayChannelMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_ClientVersion_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_ClientVersion_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_ServerVersion_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_ServerVersion_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_Initiate_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_Initiate_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_ProvideRefund_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_ProvideRefund_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_ReturnRefund_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_ReturnRefund_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_ProvideContract_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_ProvideContract_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_UpdatePayment_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_UpdatePayment_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_PaymentAck_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_PaymentAck_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_Settlement_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_Settlement_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_paymentchannels_Error_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_paymentchannels_Error_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024paymentchannel.proto\022\017paymentchannels\"" +
"\260\006\n\024TwoWayChannelMessage\022?\n\004type\030\001 \002(\01621" +
".paymentchannels.TwoWayChannelMessage.Me" +
"ssageType\0226\n\016client_version\030\002 \001(\0132\036.paym" +
"entchannels.ClientVersion\0226\n\016server_vers" +
"ion\030\003 \001(\0132\036.paymentchannels.ServerVersio" +
"n\022+\n\010initiate\030\004 \001(\0132\031.paymentchannels.In" +
"itiate\0226\n\016provide_refund\030\005 \001(\0132\036.payment" +
"channels.ProvideRefund\0224\n\rreturn_refund\030" +
"\006 \001(\0132\035.paymentchannels.ReturnRefund\022:\n\020",
"provide_contract\030\007 \001(\0132 .paymentchannels" +
".ProvideContract\0226\n\016update_payment\030\010 \001(\013" +
"2\036.paymentchannels.UpdatePayment\0220\n\013paym" +
"ent_ack\030\013 \001(\0132\033.paymentchannels.PaymentA" +
"ck\022/\n\nsettlement\030\t \001(\0132\033.paymentchannels" +
".Settlement\022%\n\005error\030\n \001(\0132\026.paymentchan" +
"nels.Error\"\315\001\n\013MessageType\022\022\n\016CLIENT_VER" +
"SION\020\001\022\022\n\016SERVER_VERSION\020\002\022\014\n\010INITIATE\020\003" +
"\022\022\n\016PROVIDE_REFUND\020\004\022\021\n\rRETURN_REFUND\020\005\022" +
"\024\n\020PROVIDE_CONTRACT\020\006\022\020\n\014CHANNEL_OPEN\020\007\022",
"\022\n\016UPDATE_PAYMENT\020\010\022\017\n\013PAYMENT_ACK\020\013\022\t\n\005" +
"CLOSE\020\t\022\t\n\005ERROR\020\n\"y\n\rClientVersion\022\r\n\005m" +
"ajor\030\001 \002(\005\022\020\n\005minor\030\002 \001(\005:\0010\022&\n\036previous" +
"_channel_contract_hash\030\003 \001(\014\022\037\n\020time_win" +
"dow_secs\030\004 \001(\004:\00586340\"0\n\rServerVersion\022\r" +
"\n\005major\030\001 \002(\005\022\020\n\005minor\030\002 \001(\005:\0010\"r\n\010Initi" +
"ate\022\024\n\014multisig_key\030\001 \002(\014\022!\n\031min_accepte" +
"d_channel_size\030\002 \002(\004\022\030\n\020expire_time_secs" +
"\030\003 \002(\004\022\023\n\013min_payment\030\004 \002(\004\"1\n\rProvideRe" +
"fund\022\024\n\014multisig_key\030\001 \002(\014\022\n\n\002tx\030\002 \002(\014\"!",
"\n\014ReturnRefund\022\021\n\tsignature\030\001 \002(\014\"j\n\017Pro" +
"videContract\022\n\n\002tx\030\001 \002(\014\0227\n\017initial_paym" +
"ent\030\002 \002(\0132\036.paymentchannels.UpdatePaymen" +
"t\022\022\n\nclient_key\030\003 \001(\014\"M\n\rUpdatePayment\022\033" +
"\n\023client_change_value\030\001 \002(\004\022\021\n\tsignature" +
"\030\002 \002(\014\022\014\n\004info\030\003 \001(\014\"\032\n\nPaymentAck\022\014\n\004in" +
"fo\030\001 \001(\014\"\030\n\nSettlement\022\n\n\002tx\030\003 \002(\014\"\251\002\n\005E" +
"rror\0225\n\004code\030\001 \001(\0162 .paymentchannels.Err" +
"or.ErrorCode:\005OTHER\022\023\n\013explanation\030\002 \001(\t" +
"\022\026\n\016expected_value\030\003 \001(\004\"\273\001\n\tErrorCode\022\013",
"\n\007TIMEOUT\020\001\022\020\n\014SYNTAX_ERROR\020\002\022\031\n\025NO_ACCE" +
"PTABLE_VERSION\020\003\022\023\n\017BAD_TRANSACTION\020\004\022\034\n" +
"\030TIME_WINDOW_UNACCEPTABLE\020\005\022\033\n\027CHANNEL_V" +
"ALUE_TOO_LARGE\020\006\022\031\n\025MIN_PAYMENT_TOO_LARG" +
"E\020\007\022\t\n\005OTHER\020\010B$\n\032org.bitcoin.paymentcha" +
"nnelB\006Protos"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_paymentchannels_TwoWayChannelMessage_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_paymentchannels_TwoWayChannelMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_TwoWayChannelMessage_descriptor,
new java.lang.String[] { "Type", "ClientVersion", "ServerVersion", "Initiate", "ProvideRefund", "ReturnRefund", "ProvideContract", "UpdatePayment", "PaymentAck", "Settlement", "Error", });
internal_static_paymentchannels_ClientVersion_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_paymentchannels_ClientVersion_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_ClientVersion_descriptor,
new java.lang.String[] { "Major", "Minor", "PreviousChannelContractHash", "TimeWindowSecs", });
internal_static_paymentchannels_ServerVersion_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_paymentchannels_ServerVersion_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_ServerVersion_descriptor,
new java.lang.String[] { "Major", "Minor", });
internal_static_paymentchannels_Initiate_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_paymentchannels_Initiate_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_Initiate_descriptor,
new java.lang.String[] { "MultisigKey", "MinAcceptedChannelSize", "ExpireTimeSecs", "MinPayment", });
internal_static_paymentchannels_ProvideRefund_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_paymentchannels_ProvideRefund_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_ProvideRefund_descriptor,
new java.lang.String[] { "MultisigKey", "Tx", });
internal_static_paymentchannels_ReturnRefund_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_paymentchannels_ReturnRefund_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_ReturnRefund_descriptor,
new java.lang.String[] { "Signature", });
internal_static_paymentchannels_ProvideContract_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_paymentchannels_ProvideContract_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_ProvideContract_descriptor,
new java.lang.String[] { "Tx", "InitialPayment", "ClientKey", });
internal_static_paymentchannels_UpdatePayment_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_paymentchannels_UpdatePayment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_UpdatePayment_descriptor,
new java.lang.String[] { "ClientChangeValue", "Signature", "Info", });
internal_static_paymentchannels_PaymentAck_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_paymentchannels_PaymentAck_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_PaymentAck_descriptor,
new java.lang.String[] { "Info", });
internal_static_paymentchannels_Settlement_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_paymentchannels_Settlement_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_Settlement_descriptor,
new java.lang.String[] { "Tx", });
internal_static_paymentchannels_Error_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_paymentchannels_Error_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_paymentchannels_Error_descriptor,
new java.lang.String[] { "Code", "Explanation", "ExpectedValue", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy