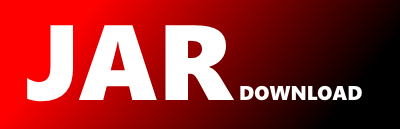
win.doyto.query.test.TestEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doyto-query-test Show documentation
Show all versions of doyto-query-test Show documentation
The test package for DoytoQuery Library
// Generated by delombok at Sat Jan 27 04:38:03 UTC 2024
/*
* Copyright © 2019-2024 Forb Yuan
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package win.doyto.query.test;
import win.doyto.query.annotation.Column;
import win.doyto.query.annotation.Entity;
import win.doyto.query.annotation.Transient;
import win.doyto.query.core.OptimisticLock;
import win.doyto.query.entity.AbstractPersistable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* TestEntity
*
* @author f0rb 2019-05-12
*/
@Entity(name = TestEntity.TABLE)
public class TestEntity extends AbstractPersistable implements OptimisticLock {
public static final String TABLE = "t_user";
private String username;
private String password;
private String mobile;
private String email;
private String nickname;
private TestEnum userLevel;
private String memo;
private Integer score;
private Boolean valid;
private Integer version;
@Transient
private Date createTime;
@Column(name = "version")
@Override
public Integer currentVersion() {
return version;
}
private static final int INIT_SIZE = 5;
public static List initUserEntities() {
List userEntities = new ArrayList<>(INIT_SIZE);
for (int i = 1; i < INIT_SIZE; i++) {
TestEntity testEntity = new TestEntity();
testEntity.setId(i);
testEntity.setUsername("username" + i);
testEntity.setPassword("password" + i);
testEntity.setEmail("test" + i + "@163.com");
testEntity.setMobile("1777888888" + i);
testEntity.setUserLevel(TestEnum.NORMAL);
testEntity.setValid(i % 2 == 0);
testEntity.setScore(i * 10);
userEntities.add(testEntity);
}
TestEntity testEntity = new TestEntity();
testEntity.setId(INIT_SIZE);
testEntity.setUsername("f0rb");
testEntity.setNickname("自在");
testEntity.setPassword("123456");
testEntity.setEmail("[email protected]");
testEntity.setMobile("17778888880");
testEntity.setUserLevel(TestEnum.VIP);
testEntity.setValid(true);
testEntity.setMemo("master");
testEntity.setScore(100);
userEntities.add(testEntity);
return userEntities;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class TestEntityBuilder> extends AbstractPersistable.AbstractPersistableBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String username;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String password;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String mobile;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String email;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String nickname;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestEnum userLevel;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String memo;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer score;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean valid;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer version;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Date createTime;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B username(final String username) {
this.username = username;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B password(final String password) {
this.password = password;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B mobile(final String mobile) {
this.mobile = mobile;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B email(final String email) {
this.email = email;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B nickname(final String nickname) {
this.nickname = nickname;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userLevel(final TestEnum userLevel) {
this.userLevel = userLevel;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B memo(final String memo) {
this.memo = memo;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B score(final Integer score) {
this.score = score;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B valid(final Boolean valid) {
this.valid = valid;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B version(final Integer version) {
this.version = version;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B createTime(final Date createTime) {
this.createTime = createTime;
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "TestEntity.TestEntityBuilder(super=" + super.toString() + ", username=" + this.username + ", password=" + this.password + ", mobile=" + this.mobile + ", email=" + this.email + ", nickname=" + this.nickname + ", userLevel=" + this.userLevel + ", memo=" + this.memo + ", score=" + this.score + ", valid=" + this.valid + ", version=" + this.version + ", createTime=" + this.createTime + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class TestEntityBuilderImpl extends TestEntity.TestEntityBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestEntityBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected TestEntity.TestEntityBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestEntity build() {
return new TestEntity(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected TestEntity(final TestEntity.TestEntityBuilder, ?> b) {
super(b);
this.username = b.username;
this.password = b.password;
this.mobile = b.mobile;
this.email = b.email;
this.nickname = b.nickname;
this.userLevel = b.userLevel;
this.memo = b.memo;
this.score = b.score;
this.valid = b.valid;
this.version = b.version;
this.createTime = b.createTime;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static TestEntity.TestEntityBuilder, ?> builder() {
return new TestEntity.TestEntityBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsername() {
return this.username;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPassword() {
return this.password;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMobile() {
return this.mobile;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNickname() {
return this.nickname;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestEnum getUserLevel() {
return this.userLevel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMemo() {
return this.memo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getScore() {
return this.score;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getValid() {
return this.valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getVersion() {
return this.version;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Date getCreateTime() {
return this.createTime;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsername(final String username) {
this.username = username;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPassword(final String password) {
this.password = password;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMobile(final String mobile) {
this.mobile = mobile;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNickname(final String nickname) {
this.nickname = nickname;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserLevel(final TestEnum userLevel) {
this.userLevel = userLevel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMemo(final String memo) {
this.memo = memo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScore(final Integer score) {
this.score = score;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValid(final Boolean valid) {
this.valid = valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVersion(final Integer version) {
this.version = version;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreateTime(final Date createTime) {
this.createTime = createTime;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "TestEntity(username=" + this.getUsername() + ", password=" + this.getPassword() + ", mobile=" + this.getMobile() + ", email=" + this.getEmail() + ", nickname=" + this.getNickname() + ", userLevel=" + this.getUserLevel() + ", memo=" + this.getMemo() + ", score=" + this.getScore() + ", valid=" + this.getValid() + ", version=" + this.getVersion() + ", createTime=" + this.getCreateTime() + ")";
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof TestEntity)) return false;
final TestEntity other = (TestEntity) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$score = this.getScore();
final java.lang.Object other$score = other.getScore();
if (this$score == null ? other$score != null : !this$score.equals(other$score)) return false;
final java.lang.Object this$valid = this.getValid();
final java.lang.Object other$valid = other.getValid();
if (this$valid == null ? other$valid != null : !this$valid.equals(other$valid)) return false;
final java.lang.Object this$version = this.getVersion();
final java.lang.Object other$version = other.getVersion();
if (this$version == null ? other$version != null : !this$version.equals(other$version)) return false;
final java.lang.Object this$username = this.getUsername();
final java.lang.Object other$username = other.getUsername();
if (this$username == null ? other$username != null : !this$username.equals(other$username)) return false;
final java.lang.Object this$password = this.getPassword();
final java.lang.Object other$password = other.getPassword();
if (this$password == null ? other$password != null : !this$password.equals(other$password)) return false;
final java.lang.Object this$mobile = this.getMobile();
final java.lang.Object other$mobile = other.getMobile();
if (this$mobile == null ? other$mobile != null : !this$mobile.equals(other$mobile)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$nickname = this.getNickname();
final java.lang.Object other$nickname = other.getNickname();
if (this$nickname == null ? other$nickname != null : !this$nickname.equals(other$nickname)) return false;
final java.lang.Object this$userLevel = this.getUserLevel();
final java.lang.Object other$userLevel = other.getUserLevel();
if (this$userLevel == null ? other$userLevel != null : !this$userLevel.equals(other$userLevel)) return false;
final java.lang.Object this$memo = this.getMemo();
final java.lang.Object other$memo = other.getMemo();
if (this$memo == null ? other$memo != null : !this$memo.equals(other$memo)) return false;
final java.lang.Object this$createTime = this.getCreateTime();
final java.lang.Object other$createTime = other.getCreateTime();
if (this$createTime == null ? other$createTime != null : !this$createTime.equals(other$createTime)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof TestEntity;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final java.lang.Object $score = this.getScore();
result = result * PRIME + ($score == null ? 43 : $score.hashCode());
final java.lang.Object $valid = this.getValid();
result = result * PRIME + ($valid == null ? 43 : $valid.hashCode());
final java.lang.Object $version = this.getVersion();
result = result * PRIME + ($version == null ? 43 : $version.hashCode());
final java.lang.Object $username = this.getUsername();
result = result * PRIME + ($username == null ? 43 : $username.hashCode());
final java.lang.Object $password = this.getPassword();
result = result * PRIME + ($password == null ? 43 : $password.hashCode());
final java.lang.Object $mobile = this.getMobile();
result = result * PRIME + ($mobile == null ? 43 : $mobile.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $nickname = this.getNickname();
result = result * PRIME + ($nickname == null ? 43 : $nickname.hashCode());
final java.lang.Object $userLevel = this.getUserLevel();
result = result * PRIME + ($userLevel == null ? 43 : $userLevel.hashCode());
final java.lang.Object $memo = this.getMemo();
result = result * PRIME + ($memo == null ? 43 : $memo.hashCode());
final java.lang.Object $createTime = this.getCreateTime();
result = result * PRIME + ($createTime == null ? 43 : $createTime.hashCode());
return result;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestEntity() {
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestEntity(final String username, final String password, final String mobile, final String email, final String nickname, final TestEnum userLevel, final String memo, final Integer score, final Boolean valid, final Integer version, final Date createTime) {
this.username = username;
this.password = password;
this.mobile = mobile;
this.email = email;
this.nickname = nickname;
this.userLevel = userLevel;
this.memo = memo;
this.score = score;
this.valid = valid;
this.version = version;
this.createTime = createTime;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy