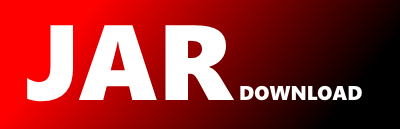
win.doyto.query.test.TestQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doyto-query-test Show documentation
Show all versions of doyto-query-test Show documentation
The test package for DoytoQuery Library
// Generated by delombok at Sat Jan 27 04:38:03 UTC 2024
/*
* Copyright © 2019-2024 Forb Yuan
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package win.doyto.query.test;
import win.doyto.query.annotation.DomainPath;
import win.doyto.query.annotation.QueryField;
import win.doyto.query.annotation.Subquery;
import win.doyto.query.core.PageQuery;
import win.doyto.query.test.perm.PermissionQuery;
import win.doyto.query.test.user.UserEntity;
import java.util.Date;
import java.util.List;
/**
* TestQuery
*
* @author f0rb
*/
@SuppressWarnings("java:S116")
public class TestQuery extends PageQuery {
@DomainPath({"user", "role", "perm"})
private PermissionQuery perm;
private List idIn;
private List idNotIn;
private Integer id;
private Integer idNot;
private Integer idNe;
private Integer idLt;
private Integer idLe;
private String username;
private String usernameEq;
@QueryField(and = "(username = ? OR email = ? OR mobile = ?)")
private String account;
private AccountOr account2;
private String email;
private String usernameOrEmailOrMobile;
private String usernameOrEmailOrMobileLike;
private String usernameLikeOrEmailLikeOrMobileLike;
private String usernameLike;
private String usernameNotLike;
private String usernameContain;
private String usernameNotContain;
private String usernameStart;
private String usernameNotStart;
private String usernameEnd;
private String usernameNotEnd;
private String testLikeEq;
private String usernameOrUserCodeLike;
private Boolean memoNull;
private boolean memoNotNull;
private TestEnum userLevel;
private TestEnum userLevelNot;
private List userLevelIn;
private List userLevelNotIn;
private List statusIn;
private Date createTimeGt;
private Date createTimeGe;
private Date createTimeLt;
private Date createTimeLe;
private Boolean valid;
@Subquery(select = "score", from = UserEntity.class)
private TestQuery scoreGtAny;
@Subquery(select = "score", from = UserEntity.class)
private TestQuery scoreLtAll;
@Subquery(select = "avg(score)", from = UserEntity.class)
private TestQuery scoreGt1;
@Subquery(select = "avgScore", from = UserEntity.class)
private TestQuery scoreGt3;
private TestQuery scoreGt$avgScoreFromUser;
private Double scoreGt; // score > ?
private Double scoreGt2; // unsupported
@Subquery(select = "score", from = UserEntity.class)
private TestQuery scoreIn;
@DomainPath(value = "user", foreignField = "createUserId")
private TestQuery userExists;
@DomainPath(value = "user", foreignField = "createUserId")
private TestQuery userNotExists;
// for MongoDB
private Boolean statusExists;
private String nation;
private String nationEq;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class TestQueryBuilder> extends PageQuery.PageQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private PermissionQuery perm;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List idIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List idNotIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer id;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer idNot;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer idNe;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer idLt;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer idLe;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String username;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameEq;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String account;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private AccountOr account2;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String email;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameOrEmailOrMobile;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameOrEmailOrMobileLike;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameLikeOrEmailLikeOrMobileLike;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameLike;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameNotLike;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameContain;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameNotContain;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameStart;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameNotStart;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameEnd;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameNotEnd;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String testLikeEq;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String usernameOrUserCodeLike;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean memoNull;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private boolean memoNotNull;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestEnum userLevel;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestEnum userLevelNot;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List userLevelIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List userLevelNotIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List statusIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Date createTimeGt;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Date createTimeGe;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Date createTimeLt;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Date createTimeLe;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean valid;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery scoreGtAny;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery scoreLtAll;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery scoreGt1;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery scoreGt3;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery scoreGt$avgScoreFromUser;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Double scoreGt;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Double scoreGt2;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery scoreIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery userExists;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQuery userNotExists;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean statusExists;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String nation;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String nationEq;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B perm(final PermissionQuery perm) {
this.perm = perm;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idIn(final List idIn) {
this.idIn = idIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idNotIn(final List idNotIn) {
this.idNotIn = idNotIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B id(final Integer id) {
this.id = id;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idNot(final Integer idNot) {
this.idNot = idNot;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idNe(final Integer idNe) {
this.idNe = idNe;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idLt(final Integer idLt) {
this.idLt = idLt;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idLe(final Integer idLe) {
this.idLe = idLe;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B username(final String username) {
this.username = username;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameEq(final String usernameEq) {
this.usernameEq = usernameEq;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B account(final String account) {
this.account = account;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B account2(final AccountOr account2) {
this.account2 = account2;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B email(final String email) {
this.email = email;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameOrEmailOrMobile(final String usernameOrEmailOrMobile) {
this.usernameOrEmailOrMobile = usernameOrEmailOrMobile;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameOrEmailOrMobileLike(final String usernameOrEmailOrMobileLike) {
this.usernameOrEmailOrMobileLike = usernameOrEmailOrMobileLike;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameLikeOrEmailLikeOrMobileLike(final String usernameLikeOrEmailLikeOrMobileLike) {
this.usernameLikeOrEmailLikeOrMobileLike = usernameLikeOrEmailLikeOrMobileLike;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameLike(final String usernameLike) {
this.usernameLike = usernameLike;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameNotLike(final String usernameNotLike) {
this.usernameNotLike = usernameNotLike;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameContain(final String usernameContain) {
this.usernameContain = usernameContain;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameNotContain(final String usernameNotContain) {
this.usernameNotContain = usernameNotContain;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameStart(final String usernameStart) {
this.usernameStart = usernameStart;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameNotStart(final String usernameNotStart) {
this.usernameNotStart = usernameNotStart;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameEnd(final String usernameEnd) {
this.usernameEnd = usernameEnd;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameNotEnd(final String usernameNotEnd) {
this.usernameNotEnd = usernameNotEnd;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B testLikeEq(final String testLikeEq) {
this.testLikeEq = testLikeEq;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B usernameOrUserCodeLike(final String usernameOrUserCodeLike) {
this.usernameOrUserCodeLike = usernameOrUserCodeLike;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B memoNull(final Boolean memoNull) {
this.memoNull = memoNull;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B memoNotNull(final boolean memoNotNull) {
this.memoNotNull = memoNotNull;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userLevel(final TestEnum userLevel) {
this.userLevel = userLevel;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userLevelNot(final TestEnum userLevelNot) {
this.userLevelNot = userLevelNot;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userLevelIn(final List userLevelIn) {
this.userLevelIn = userLevelIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userLevelNotIn(final List userLevelNotIn) {
this.userLevelNotIn = userLevelNotIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B statusIn(final List statusIn) {
this.statusIn = statusIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B createTimeGt(final Date createTimeGt) {
this.createTimeGt = createTimeGt;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B createTimeGe(final Date createTimeGe) {
this.createTimeGe = createTimeGe;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B createTimeLt(final Date createTimeLt) {
this.createTimeLt = createTimeLt;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B createTimeLe(final Date createTimeLe) {
this.createTimeLe = createTimeLe;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B valid(final Boolean valid) {
this.valid = valid;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreGtAny(final TestQuery scoreGtAny) {
this.scoreGtAny = scoreGtAny;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreLtAll(final TestQuery scoreLtAll) {
this.scoreLtAll = scoreLtAll;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreGt1(final TestQuery scoreGt1) {
this.scoreGt1 = scoreGt1;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreGt3(final TestQuery scoreGt3) {
this.scoreGt3 = scoreGt3;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreGt$avgScoreFromUser(final TestQuery scoreGt$avgScoreFromUser) {
this.scoreGt$avgScoreFromUser = scoreGt$avgScoreFromUser;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreGt(final Double scoreGt) {
this.scoreGt = scoreGt;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreGt2(final Double scoreGt2) {
this.scoreGt2 = scoreGt2;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B scoreIn(final TestQuery scoreIn) {
this.scoreIn = scoreIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userExists(final TestQuery userExists) {
this.userExists = userExists;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B userNotExists(final TestQuery userNotExists) {
this.userNotExists = userNotExists;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B statusExists(final Boolean statusExists) {
this.statusExists = statusExists;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B nation(final String nation) {
this.nation = nation;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B nationEq(final String nationEq) {
this.nationEq = nationEq;
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "TestQuery.TestQueryBuilder(super=" + super.toString() + ", perm=" + this.perm + ", idIn=" + this.idIn + ", idNotIn=" + this.idNotIn + ", id=" + this.id + ", idNot=" + this.idNot + ", idNe=" + this.idNe + ", idLt=" + this.idLt + ", idLe=" + this.idLe + ", username=" + this.username + ", usernameEq=" + this.usernameEq + ", account=" + this.account + ", account2=" + this.account2 + ", email=" + this.email + ", usernameOrEmailOrMobile=" + this.usernameOrEmailOrMobile + ", usernameOrEmailOrMobileLike=" + this.usernameOrEmailOrMobileLike + ", usernameLikeOrEmailLikeOrMobileLike=" + this.usernameLikeOrEmailLikeOrMobileLike + ", usernameLike=" + this.usernameLike + ", usernameNotLike=" + this.usernameNotLike + ", usernameContain=" + this.usernameContain + ", usernameNotContain=" + this.usernameNotContain + ", usernameStart=" + this.usernameStart + ", usernameNotStart=" + this.usernameNotStart + ", usernameEnd=" + this.usernameEnd + ", usernameNotEnd=" + this.usernameNotEnd + ", testLikeEq=" + this.testLikeEq + ", usernameOrUserCodeLike=" + this.usernameOrUserCodeLike + ", memoNull=" + this.memoNull + ", memoNotNull=" + this.memoNotNull + ", userLevel=" + this.userLevel + ", userLevelNot=" + this.userLevelNot + ", userLevelIn=" + this.userLevelIn + ", userLevelNotIn=" + this.userLevelNotIn + ", statusIn=" + this.statusIn + ", createTimeGt=" + this.createTimeGt + ", createTimeGe=" + this.createTimeGe + ", createTimeLt=" + this.createTimeLt + ", createTimeLe=" + this.createTimeLe + ", valid=" + this.valid + ", scoreGtAny=" + this.scoreGtAny + ", scoreLtAll=" + this.scoreLtAll + ", scoreGt1=" + this.scoreGt1 + ", scoreGt3=" + this.scoreGt3 + ", scoreGt$avgScoreFromUser=" + this.scoreGt$avgScoreFromUser + ", scoreGt=" + this.scoreGt + ", scoreGt2=" + this.scoreGt2 + ", scoreIn=" + this.scoreIn + ", userExists=" + this.userExists + ", userNotExists=" + this.userNotExists + ", statusExists=" + this.statusExists + ", nation=" + this.nation + ", nationEq=" + this.nationEq + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class TestQueryBuilderImpl extends TestQuery.TestQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private TestQueryBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected TestQuery.TestQueryBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery build() {
return new TestQuery(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected TestQuery(final TestQuery.TestQueryBuilder, ?> b) {
super(b);
this.perm = b.perm;
this.idIn = b.idIn;
this.idNotIn = b.idNotIn;
this.id = b.id;
this.idNot = b.idNot;
this.idNe = b.idNe;
this.idLt = b.idLt;
this.idLe = b.idLe;
this.username = b.username;
this.usernameEq = b.usernameEq;
this.account = b.account;
this.account2 = b.account2;
this.email = b.email;
this.usernameOrEmailOrMobile = b.usernameOrEmailOrMobile;
this.usernameOrEmailOrMobileLike = b.usernameOrEmailOrMobileLike;
this.usernameLikeOrEmailLikeOrMobileLike = b.usernameLikeOrEmailLikeOrMobileLike;
this.usernameLike = b.usernameLike;
this.usernameNotLike = b.usernameNotLike;
this.usernameContain = b.usernameContain;
this.usernameNotContain = b.usernameNotContain;
this.usernameStart = b.usernameStart;
this.usernameNotStart = b.usernameNotStart;
this.usernameEnd = b.usernameEnd;
this.usernameNotEnd = b.usernameNotEnd;
this.testLikeEq = b.testLikeEq;
this.usernameOrUserCodeLike = b.usernameOrUserCodeLike;
this.memoNull = b.memoNull;
this.memoNotNull = b.memoNotNull;
this.userLevel = b.userLevel;
this.userLevelNot = b.userLevelNot;
this.userLevelIn = b.userLevelIn;
this.userLevelNotIn = b.userLevelNotIn;
this.statusIn = b.statusIn;
this.createTimeGt = b.createTimeGt;
this.createTimeGe = b.createTimeGe;
this.createTimeLt = b.createTimeLt;
this.createTimeLe = b.createTimeLe;
this.valid = b.valid;
this.scoreGtAny = b.scoreGtAny;
this.scoreLtAll = b.scoreLtAll;
this.scoreGt1 = b.scoreGt1;
this.scoreGt3 = b.scoreGt3;
this.scoreGt$avgScoreFromUser = b.scoreGt$avgScoreFromUser;
this.scoreGt = b.scoreGt;
this.scoreGt2 = b.scoreGt2;
this.scoreIn = b.scoreIn;
this.userExists = b.userExists;
this.userNotExists = b.userNotExists;
this.statusExists = b.statusExists;
this.nation = b.nation;
this.nationEq = b.nationEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static TestQuery.TestQueryBuilder, ?> builder() {
return new TestQuery.TestQueryBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PermissionQuery getPerm() {
return this.perm;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getIdIn() {
return this.idIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getIdNotIn() {
return this.idNotIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getId() {
return this.id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getIdNot() {
return this.idNot;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getIdNe() {
return this.idNe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getIdLt() {
return this.idLt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getIdLe() {
return this.idLe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsername() {
return this.username;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameEq() {
return this.usernameEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAccount() {
return this.account;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AccountOr getAccount2() {
return this.account2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameOrEmailOrMobile() {
return this.usernameOrEmailOrMobile;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameOrEmailOrMobileLike() {
return this.usernameOrEmailOrMobileLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameLikeOrEmailLikeOrMobileLike() {
return this.usernameLikeOrEmailLikeOrMobileLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameLike() {
return this.usernameLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameNotLike() {
return this.usernameNotLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameContain() {
return this.usernameContain;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameNotContain() {
return this.usernameNotContain;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameStart() {
return this.usernameStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameNotStart() {
return this.usernameNotStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameEnd() {
return this.usernameEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameNotEnd() {
return this.usernameNotEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTestLikeEq() {
return this.testLikeEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsernameOrUserCodeLike() {
return this.usernameOrUserCodeLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getMemoNull() {
return this.memoNull;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean isMemoNotNull() {
return this.memoNotNull;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestEnum getUserLevel() {
return this.userLevel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestEnum getUserLevelNot() {
return this.userLevelNot;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getUserLevelIn() {
return this.userLevelIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getUserLevelNotIn() {
return this.userLevelNotIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getStatusIn() {
return this.statusIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Date getCreateTimeGt() {
return this.createTimeGt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Date getCreateTimeGe() {
return this.createTimeGe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Date getCreateTimeLt() {
return this.createTimeLt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Date getCreateTimeLe() {
return this.createTimeLe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getValid() {
return this.valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getScoreGtAny() {
return this.scoreGtAny;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getScoreLtAll() {
return this.scoreLtAll;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getScoreGt1() {
return this.scoreGt1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getScoreGt3() {
return this.scoreGt3;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getScoreGt$avgScoreFromUser() {
return this.scoreGt$avgScoreFromUser;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Double getScoreGt() {
return this.scoreGt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Double getScoreGt2() {
return this.scoreGt2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getScoreIn() {
return this.scoreIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getUserExists() {
return this.userExists;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery getUserNotExists() {
return this.userNotExists;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getStatusExists() {
return this.statusExists;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNation() {
return this.nation;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNationEq() {
return this.nationEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPerm(final PermissionQuery perm) {
this.perm = perm;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdIn(final List idIn) {
this.idIn = idIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdNotIn(final List idNotIn) {
this.idNotIn = idNotIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final Integer id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdNot(final Integer idNot) {
this.idNot = idNot;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdNe(final Integer idNe) {
this.idNe = idNe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdLt(final Integer idLt) {
this.idLt = idLt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdLe(final Integer idLe) {
this.idLe = idLe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsername(final String username) {
this.username = username;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameEq(final String usernameEq) {
this.usernameEq = usernameEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccount(final String account) {
this.account = account;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccount2(final AccountOr account2) {
this.account2 = account2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameOrEmailOrMobile(final String usernameOrEmailOrMobile) {
this.usernameOrEmailOrMobile = usernameOrEmailOrMobile;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameOrEmailOrMobileLike(final String usernameOrEmailOrMobileLike) {
this.usernameOrEmailOrMobileLike = usernameOrEmailOrMobileLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameLikeOrEmailLikeOrMobileLike(final String usernameLikeOrEmailLikeOrMobileLike) {
this.usernameLikeOrEmailLikeOrMobileLike = usernameLikeOrEmailLikeOrMobileLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameLike(final String usernameLike) {
this.usernameLike = usernameLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameNotLike(final String usernameNotLike) {
this.usernameNotLike = usernameNotLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameContain(final String usernameContain) {
this.usernameContain = usernameContain;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameNotContain(final String usernameNotContain) {
this.usernameNotContain = usernameNotContain;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameStart(final String usernameStart) {
this.usernameStart = usernameStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameNotStart(final String usernameNotStart) {
this.usernameNotStart = usernameNotStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameEnd(final String usernameEnd) {
this.usernameEnd = usernameEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameNotEnd(final String usernameNotEnd) {
this.usernameNotEnd = usernameNotEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTestLikeEq(final String testLikeEq) {
this.testLikeEq = testLikeEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsernameOrUserCodeLike(final String usernameOrUserCodeLike) {
this.usernameOrUserCodeLike = usernameOrUserCodeLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMemoNull(final Boolean memoNull) {
this.memoNull = memoNull;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMemoNotNull(final boolean memoNotNull) {
this.memoNotNull = memoNotNull;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserLevel(final TestEnum userLevel) {
this.userLevel = userLevel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserLevelNot(final TestEnum userLevelNot) {
this.userLevelNot = userLevelNot;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserLevelIn(final List userLevelIn) {
this.userLevelIn = userLevelIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserLevelNotIn(final List userLevelNotIn) {
this.userLevelNotIn = userLevelNotIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatusIn(final List statusIn) {
this.statusIn = statusIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreateTimeGt(final Date createTimeGt) {
this.createTimeGt = createTimeGt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreateTimeGe(final Date createTimeGe) {
this.createTimeGe = createTimeGe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreateTimeLt(final Date createTimeLt) {
this.createTimeLt = createTimeLt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreateTimeLe(final Date createTimeLe) {
this.createTimeLe = createTimeLe;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValid(final Boolean valid) {
this.valid = valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreGtAny(final TestQuery scoreGtAny) {
this.scoreGtAny = scoreGtAny;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreLtAll(final TestQuery scoreLtAll) {
this.scoreLtAll = scoreLtAll;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreGt1(final TestQuery scoreGt1) {
this.scoreGt1 = scoreGt1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreGt3(final TestQuery scoreGt3) {
this.scoreGt3 = scoreGt3;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreGt$avgScoreFromUser(final TestQuery scoreGt$avgScoreFromUser) {
this.scoreGt$avgScoreFromUser = scoreGt$avgScoreFromUser;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreGt(final Double scoreGt) {
this.scoreGt = scoreGt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreGt2(final Double scoreGt2) {
this.scoreGt2 = scoreGt2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setScoreIn(final TestQuery scoreIn) {
this.scoreIn = scoreIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserExists(final TestQuery userExists) {
this.userExists = userExists;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserNotExists(final TestQuery userNotExists) {
this.userNotExists = userNotExists;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatusExists(final Boolean statusExists) {
this.statusExists = statusExists;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNation(final String nation) {
this.nation = nation;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNationEq(final String nationEq) {
this.nationEq = nationEq;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery() {
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TestQuery(final PermissionQuery perm, final List idIn, final List idNotIn, final Integer id, final Integer idNot, final Integer idNe, final Integer idLt, final Integer idLe, final String username, final String usernameEq, final String account, final AccountOr account2, final String email, final String usernameOrEmailOrMobile, final String usernameOrEmailOrMobileLike, final String usernameLikeOrEmailLikeOrMobileLike, final String usernameLike, final String usernameNotLike, final String usernameContain, final String usernameNotContain, final String usernameStart, final String usernameNotStart, final String usernameEnd, final String usernameNotEnd, final String testLikeEq, final String usernameOrUserCodeLike, final Boolean memoNull, final boolean memoNotNull, final TestEnum userLevel, final TestEnum userLevelNot, final List userLevelIn, final List userLevelNotIn, final List statusIn, final Date createTimeGt, final Date createTimeGe, final Date createTimeLt, final Date createTimeLe, final Boolean valid, final TestQuery scoreGtAny, final TestQuery scoreLtAll, final TestQuery scoreGt1, final TestQuery scoreGt3, final TestQuery scoreGt$avgScoreFromUser, final Double scoreGt, final Double scoreGt2, final TestQuery scoreIn, final TestQuery userExists, final TestQuery userNotExists, final Boolean statusExists, final String nation, final String nationEq) {
this.perm = perm;
this.idIn = idIn;
this.idNotIn = idNotIn;
this.id = id;
this.idNot = idNot;
this.idNe = idNe;
this.idLt = idLt;
this.idLe = idLe;
this.username = username;
this.usernameEq = usernameEq;
this.account = account;
this.account2 = account2;
this.email = email;
this.usernameOrEmailOrMobile = usernameOrEmailOrMobile;
this.usernameOrEmailOrMobileLike = usernameOrEmailOrMobileLike;
this.usernameLikeOrEmailLikeOrMobileLike = usernameLikeOrEmailLikeOrMobileLike;
this.usernameLike = usernameLike;
this.usernameNotLike = usernameNotLike;
this.usernameContain = usernameContain;
this.usernameNotContain = usernameNotContain;
this.usernameStart = usernameStart;
this.usernameNotStart = usernameNotStart;
this.usernameEnd = usernameEnd;
this.usernameNotEnd = usernameNotEnd;
this.testLikeEq = testLikeEq;
this.usernameOrUserCodeLike = usernameOrUserCodeLike;
this.memoNull = memoNull;
this.memoNotNull = memoNotNull;
this.userLevel = userLevel;
this.userLevelNot = userLevelNot;
this.userLevelIn = userLevelIn;
this.userLevelNotIn = userLevelNotIn;
this.statusIn = statusIn;
this.createTimeGt = createTimeGt;
this.createTimeGe = createTimeGe;
this.createTimeLt = createTimeLt;
this.createTimeLe = createTimeLe;
this.valid = valid;
this.scoreGtAny = scoreGtAny;
this.scoreLtAll = scoreLtAll;
this.scoreGt1 = scoreGt1;
this.scoreGt3 = scoreGt3;
this.scoreGt$avgScoreFromUser = scoreGt$avgScoreFromUser;
this.scoreGt = scoreGt;
this.scoreGt2 = scoreGt2;
this.scoreIn = scoreIn;
this.userExists = userExists;
this.userNotExists = userNotExists;
this.statusExists = statusExists;
this.nation = nation;
this.nationEq = nationEq;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy