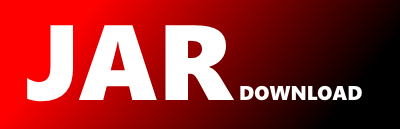
win.doyto.query.test.perm.PermissionQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doyto-query-test Show documentation
Show all versions of doyto-query-test Show documentation
The test package for DoytoQuery Library
// Generated by delombok at Sat Jan 27 04:38:03 UTC 2024
/*
* Copyright © 2019-2024 Forb Yuan
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package win.doyto.query.test.perm;
import win.doyto.query.annotation.DomainPath;
import win.doyto.query.core.PageQuery;
import win.doyto.query.test.role.RoleQuery;
import win.doyto.query.test.user.UserQuery;
/**
* PermissionQuery
*
* @author f0rb on 2019-05-28
*/
public class PermissionQuery extends PageQuery {
@DomainPath({"perm", "~", "role"})
private RoleQuery role;
private RoleQuery roleQuery;
@DomainPath({"perm", "~", "role", "~", "user"})
private UserQuery user;
private String permNameStart;
private Boolean valid;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class PermissionQueryBuilder> extends PageQuery.PageQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private RoleQuery role;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private RoleQuery roleQuery;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private UserQuery user;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String permNameStart;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean valid;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B role(final RoleQuery role) {
this.role = role;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B roleQuery(final RoleQuery roleQuery) {
this.roleQuery = roleQuery;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B user(final UserQuery user) {
this.user = user;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B permNameStart(final String permNameStart) {
this.permNameStart = permNameStart;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B valid(final Boolean valid) {
this.valid = valid;
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "PermissionQuery.PermissionQueryBuilder(super=" + super.toString() + ", role=" + this.role + ", roleQuery=" + this.roleQuery + ", user=" + this.user + ", permNameStart=" + this.permNameStart + ", valid=" + this.valid + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class PermissionQueryBuilderImpl extends PermissionQuery.PermissionQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private PermissionQueryBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected PermissionQuery.PermissionQueryBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PermissionQuery build() {
return new PermissionQuery(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected PermissionQuery(final PermissionQuery.PermissionQueryBuilder, ?> b) {
super(b);
this.role = b.role;
this.roleQuery = b.roleQuery;
this.user = b.user;
this.permNameStart = b.permNameStart;
this.valid = b.valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static PermissionQuery.PermissionQueryBuilder, ?> builder() {
return new PermissionQuery.PermissionQueryBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery getRole() {
return this.role;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery getRoleQuery() {
return this.roleQuery;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public UserQuery getUser() {
return this.user;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPermNameStart() {
return this.permNameStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getValid() {
return this.valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRole(final RoleQuery role) {
this.role = role;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRoleQuery(final RoleQuery roleQuery) {
this.roleQuery = roleQuery;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUser(final UserQuery user) {
this.user = user;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPermNameStart(final String permNameStart) {
this.permNameStart = permNameStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValid(final Boolean valid) {
this.valid = valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PermissionQuery() {
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PermissionQuery(final RoleQuery role, final RoleQuery roleQuery, final UserQuery user, final String permNameStart, final Boolean valid) {
this.role = role;
this.roleQuery = roleQuery;
this.user = user;
this.permNameStart = permNameStart;
this.valid = valid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy