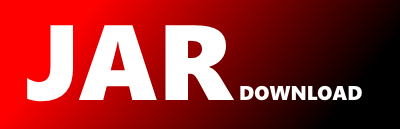
win.doyto.query.test.role.RoleQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doyto-query-test Show documentation
Show all versions of doyto-query-test Show documentation
The test package for DoytoQuery Library
// Generated by delombok at Sat Jan 27 04:38:03 UTC 2024
/*
* Copyright © 2019-2024 Forb Yuan
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package win.doyto.query.test.role;
import win.doyto.query.annotation.DomainPath;
import win.doyto.query.core.PageQuery;
import win.doyto.query.test.user.UserQuery;
import java.util.List;
/**
* UserQuery
*
* @author f0rb on 2020-04-01
*/
public class RoleQuery extends PageQuery {
private Integer id;
private List idIn;
@DomainPath({"role", "~", "user"})
private UserQuery user;
private String roleName;
private String roleNameLike;
private Boolean valid;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class RoleQueryBuilder> extends PageQuery.PageQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Integer id;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List idIn;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private UserQuery user;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String roleName;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String roleNameLike;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private Boolean valid;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B id(final Integer id) {
this.id = id;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B idIn(final List idIn) {
this.idIn = idIn;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B user(final UserQuery user) {
this.user = user;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B roleName(final String roleName) {
this.roleName = roleName;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B roleNameLike(final String roleNameLike) {
this.roleNameLike = roleNameLike;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B valid(final Boolean valid) {
this.valid = valid;
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "RoleQuery.RoleQueryBuilder(super=" + super.toString() + ", id=" + this.id + ", idIn=" + this.idIn + ", user=" + this.user + ", roleName=" + this.roleName + ", roleNameLike=" + this.roleNameLike + ", valid=" + this.valid + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class RoleQueryBuilderImpl extends RoleQuery.RoleQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private RoleQueryBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected RoleQuery.RoleQueryBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery build() {
return new RoleQuery(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected RoleQuery(final RoleQuery.RoleQueryBuilder, ?> b) {
super(b);
this.id = b.id;
this.idIn = b.idIn;
this.user = b.user;
this.roleName = b.roleName;
this.roleNameLike = b.roleNameLike;
this.valid = b.valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static RoleQuery.RoleQueryBuilder, ?> builder() {
return new RoleQuery.RoleQueryBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Integer getId() {
return this.id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getIdIn() {
return this.idIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public UserQuery getUser() {
return this.user;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRoleName() {
return this.roleName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRoleNameLike() {
return this.roleNameLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getValid() {
return this.valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final Integer id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdIn(final List idIn) {
this.idIn = idIn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUser(final UserQuery user) {
this.user = user;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRoleName(final String roleName) {
this.roleName = roleName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRoleNameLike(final String roleNameLike) {
this.roleNameLike = roleNameLike;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValid(final Boolean valid) {
this.valid = valid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery() {
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery(final Integer id, final List idIn, final UserQuery user, final String roleName, final String roleNameLike, final Boolean valid) {
this.id = id;
this.idIn = idIn;
this.user = user;
this.roleName = roleName;
this.roleNameLike = roleNameLike;
this.valid = valid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy