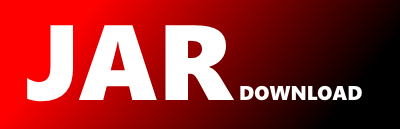
win.doyto.query.test.user.UserViewQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doyto-query-test Show documentation
Show all versions of doyto-query-test Show documentation
The test package for DoytoQuery Library
// Generated by delombok at Sat Jan 27 04:38:03 UTC 2024
/*
* Copyright © 2019-2024 Forb Yuan
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package win.doyto.query.test.user;
import win.doyto.query.core.RelationalQuery;
import win.doyto.query.test.menu.MenuQuery;
import win.doyto.query.test.perm.PermissionQuery;
import win.doyto.query.test.role.RoleQuery;
/**
* UserViewQuery
*
* @author f0rb on 2022-03-26
*/
public class UserViewQuery extends UserQuery implements RelationalQuery {
private RoleQuery withRoles;
private PermissionQuery withPerms;
private MenuQuery withMenus;
private UserQuery withCreateUser;
private RoleQuery withCreateRoles;
@Override
public Class getDomainClass() {
return UserView.class;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static abstract class UserViewQueryBuilder> extends UserQuery.UserQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private RoleQuery withRoles;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private PermissionQuery withPerms;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private MenuQuery withMenus;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private UserQuery withCreateUser;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private RoleQuery withCreateRoles;
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected abstract B self();
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public abstract C build();
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B withRoles(final RoleQuery withRoles) {
this.withRoles = withRoles;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B withPerms(final PermissionQuery withPerms) {
this.withPerms = withPerms;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B withMenus(final MenuQuery withMenus) {
this.withMenus = withMenus;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B withCreateUser(final UserQuery withCreateUser) {
this.withCreateUser = withCreateUser;
return self();
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public B withCreateRoles(final RoleQuery withCreateRoles) {
this.withCreateRoles = withCreateRoles;
return self();
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "UserViewQuery.UserViewQueryBuilder(super=" + super.toString() + ", withRoles=" + this.withRoles + ", withPerms=" + this.withPerms + ", withMenus=" + this.withMenus + ", withCreateUser=" + this.withCreateUser + ", withCreateRoles=" + this.withCreateRoles + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private static final class UserViewQueryBuilderImpl extends UserViewQuery.UserViewQueryBuilder {
@java.lang.SuppressWarnings("all")
@lombok.Generated
private UserViewQueryBuilderImpl() {
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected UserViewQuery.UserViewQueryBuilderImpl self() {
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public UserViewQuery build() {
return new UserViewQuery(this);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected UserViewQuery(final UserViewQuery.UserViewQueryBuilder, ?> b) {
super(b);
this.withRoles = b.withRoles;
this.withPerms = b.withPerms;
this.withMenus = b.withMenus;
this.withCreateUser = b.withCreateUser;
this.withCreateRoles = b.withCreateRoles;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static UserViewQuery.UserViewQueryBuilder, ?> builder() {
return new UserViewQuery.UserViewQueryBuilderImpl();
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery getWithRoles() {
return this.withRoles;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PermissionQuery getWithPerms() {
return this.withPerms;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MenuQuery getWithMenus() {
return this.withMenus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public UserQuery getWithCreateUser() {
return this.withCreateUser;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RoleQuery getWithCreateRoles() {
return this.withCreateRoles;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWithRoles(final RoleQuery withRoles) {
this.withRoles = withRoles;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWithPerms(final PermissionQuery withPerms) {
this.withPerms = withPerms;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWithMenus(final MenuQuery withMenus) {
this.withMenus = withMenus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWithCreateUser(final UserQuery withCreateUser) {
this.withCreateUser = withCreateUser;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWithCreateRoles(final RoleQuery withCreateRoles) {
this.withCreateRoles = withCreateRoles;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public UserViewQuery(final RoleQuery withRoles, final PermissionQuery withPerms, final MenuQuery withMenus, final UserQuery withCreateUser, final RoleQuery withCreateRoles) {
this.withRoles = withRoles;
this.withPerms = withPerms;
this.withMenus = withMenus;
this.withCreateUser = withCreateUser;
this.withCreateRoles = withCreateRoles;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public UserViewQuery() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy