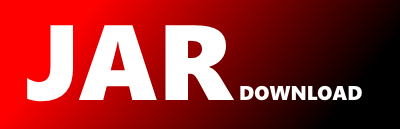
win.doyto.query.core.QuerySuffix Maven / Gradle / Ivy
// Generated by delombok at Sat Aug 10 11:56:00 CST 2019
package win.doyto.query.core;
import org.apache.commons.lang3.StringUtils;
import java.util.*;
import java.util.function.Function;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import static win.doyto.query.core.Constant.*;
/**
* QuerySuffix
*
* @author f0rb
*/
@SuppressWarnings("squid:S00115")
enum QuerySuffix {
Not("!="), NotLike("NOT LIKE"), Start("LIKE"), Like("LIKE"), NotIn("NOT IN", Ex.collection), In("IN", Ex.collection), NotNull("IS NOT NULL", Ex.empty), Null("IS NULL", Ex.empty), Gt(">"), Ge(">="), Lt("<"), Le("<="), NONE("=");
private static final Pattern SUFFIX_PTN;
private static final Map> sqlFuncMap = new EnumMap<>(QuerySuffix.class);
static {
List suffixList = Arrays.stream(values()).filter(querySuffix -> querySuffix != NONE).map(Enum::name).collect(Collectors.toList());
String suffixPtn = StringUtils.join(suffixList, "|");
SUFFIX_PTN = Pattern.compile("(" + suffixPtn + ")$");
Arrays.stream(values()).forEach(querySuffix -> sqlFuncMap.put(querySuffix, querySuffix::buildAndSql));
}
private final String op;
private final Ex ex;
QuerySuffix(String op) {
this(op, Ex.placeHolder);
}
QuerySuffix(String op, Ex ex) {
this.op = op;
this.ex = ex;
}
static QuerySuffix resolve(String fieldName) {
Matcher matcher = SUFFIX_PTN.matcher(fieldName);
return matcher.find() ? valueOf(matcher.group()) : NONE;
}
static String buildAndSql(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy